Mastering JPQL and HQL Tests Without Deployment Hassles
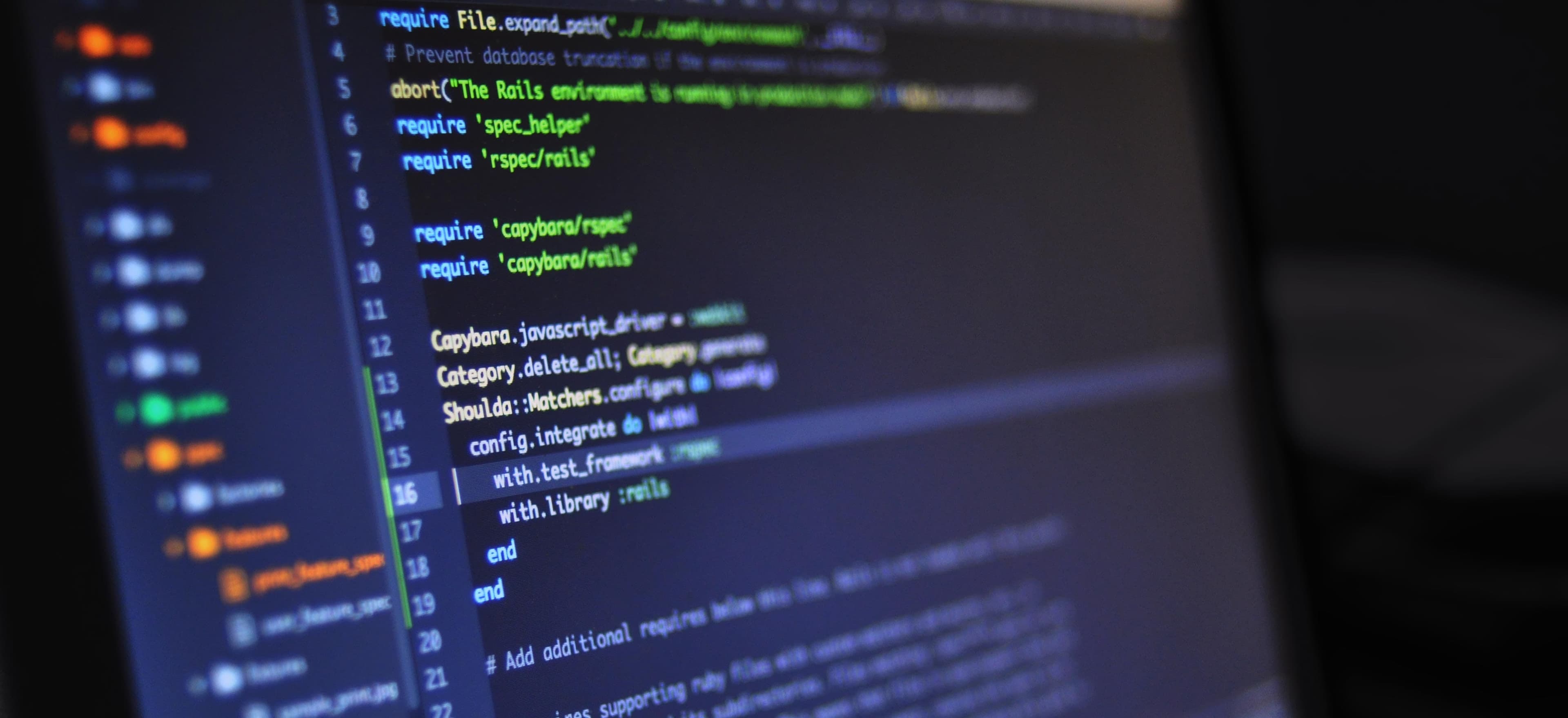
- Published on
Mastering JPQL and HQL Tests Without Deployment Hassles
Java Persistence Query Language (JPQL) and Hibernate Query Language (HQL) are integral when dealing with relational databases in Java applications. They offer a powerful way to query data without being tightly coupled to SQL, promoting the principle of portability while providing a more object-oriented approach to data manipulation.
However, the typical development process can become cumbersome, especially when testing queries. You may find yourself deploying the whole application just to verify if a query works as intended. In this blog post, we will explore best practices for mastering JPQL and HQL tests without the hassle of deployment.
Understanding JPQL and HQL
Before diving into testing best practices, let's first clarify the relationship between JPQL, HQL, and SQL.
-
JPQL: This is a query language defined by the Java Persistence API (JPA). It is designed to operate over entity objects instead of traditional tables.
-
HQL: Hibernate Query Language, a superset of JPQL, allows for the querying of entities in a Hibernate-specific manner.
Both JPQL and HQL provide developers the ability to execute queries independently of the underlying database schema.
Why Test JPQL and HQL Queries?
Testing queries is critical for various reasons:
- Performance: Efficient queries can significantly improve application performance.
- Correctness: Ensuring the query logic is sound prevents bugs and unexpected behavior.
- Refactoring: As your code evolves, so do queries. Testing helps keep them in check.
Setting Up Your Testing Environment
For effective testing, it is essential to set up a lightweight environment. A commonly used library for this is H2 Database, an in-memory SQL database that allows rapid testing without external dependencies.
Adding H2 to Your Project
You can easily include H2 in your Maven pom.xml
:
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>test</scope>
</dependency>
For Gradle, add this to your build.gradle
:
testImplementation 'com.h2database:h2'
Writing Unit Tests for JPQL and HQL
Here, we'll use JUnit 5 as our testing framework. Ensure you import relevant JPA and Hibernate libraries in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
Example Entity Class
First, let’s start with a simple entity class named Book
.
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
private Author author;
// Constructors, getters, and setters
}
This Book
class represents a book entity with an id
, a title
, and a reference to an Author
entity.
Example Query Method
Let’s build a repository interface for managing Book
entities:
public interface BookRepository extends JpaRepository<Book, Long> {
@Query("SELECT b FROM Book b WHERE b.title = :title")
List<Book> findByTitle(@Param("title") String title);
}
This query retrieves books based on their title. It leverages JPQL's potential to work with entities instead of raw SQL.
Writing a Test Case
Now, let's create a test class to verify our query.
@ExtendWith(SpringExtension.class)
@DataJpaTest
public class BookRepositoryTests {
@Autowired
private BookRepository bookRepository;
@Autowired
private TestEntityManager entityManager;
@Test
public void testFindByTitle() {
// Given
Author author = new Author("J.K. Rowling");
entityManager.persist(author);
Book book = new Book("Harry Potter and the Philosopher's Stone", author);
entityManager.persist(book);
entityManager.flush();
// When
List<Book> foundBooks = bookRepository.findByTitle("Harry Potter and the Philosopher's Stone");
// Then
assertEquals(1, foundBooks.size());
assertEquals("Harry Potter and the Philosopher's Stone", foundBooks.get(0).getTitle());
}
}
Code Explanation
- Test Entity Creation: We create an
Author
entity and persist it. Then, we create aBook
entity related to that author and flush it to the database. - Query Execution: We call the
findByTitle
method of our repository. - Assertions: Finally, we assert that we found exactly one book, and its title matches what we queried.
Running Tests Without Deployment
By leveraging an in-memory database and Spring Data JPA's testing support, you can run these unit tests quickly without deploying the entire application. This setup allows you to validate your queries in isolation, focusing solely on correctness and efficiency.
Testing Edge Cases
It is also crucial to test edge cases to ensure robust solutions. You might want to check:
@Test
public void testFindByNonExistentTitle() {
List<Book> foundBooks = bookRepository.findByTitle("Non Existent Book");
assertTrue(foundBooks.isEmpty());
}
This checks that searching for a non-existent book returns an empty list, further strengthening your repository's reliability.
The Bottom Line
In conclusion, effective testing of JPQL and HQL queries can streamline your development process significantly. By employing an in-memory database, you can validate functionality quickly, freeing you from cumbersome deployment cycles. You can focus on optimizing queries and enhancing performance without the overhead of full-scale deployment.
For a deeper understanding of JPQL and its capabilities, feel free to check out the Java EE 8 Tutorial for more insights and advanced topics. Happy coding!
Checkout our other articles