Common Pitfalls in Hibernate DDL Schema Generation
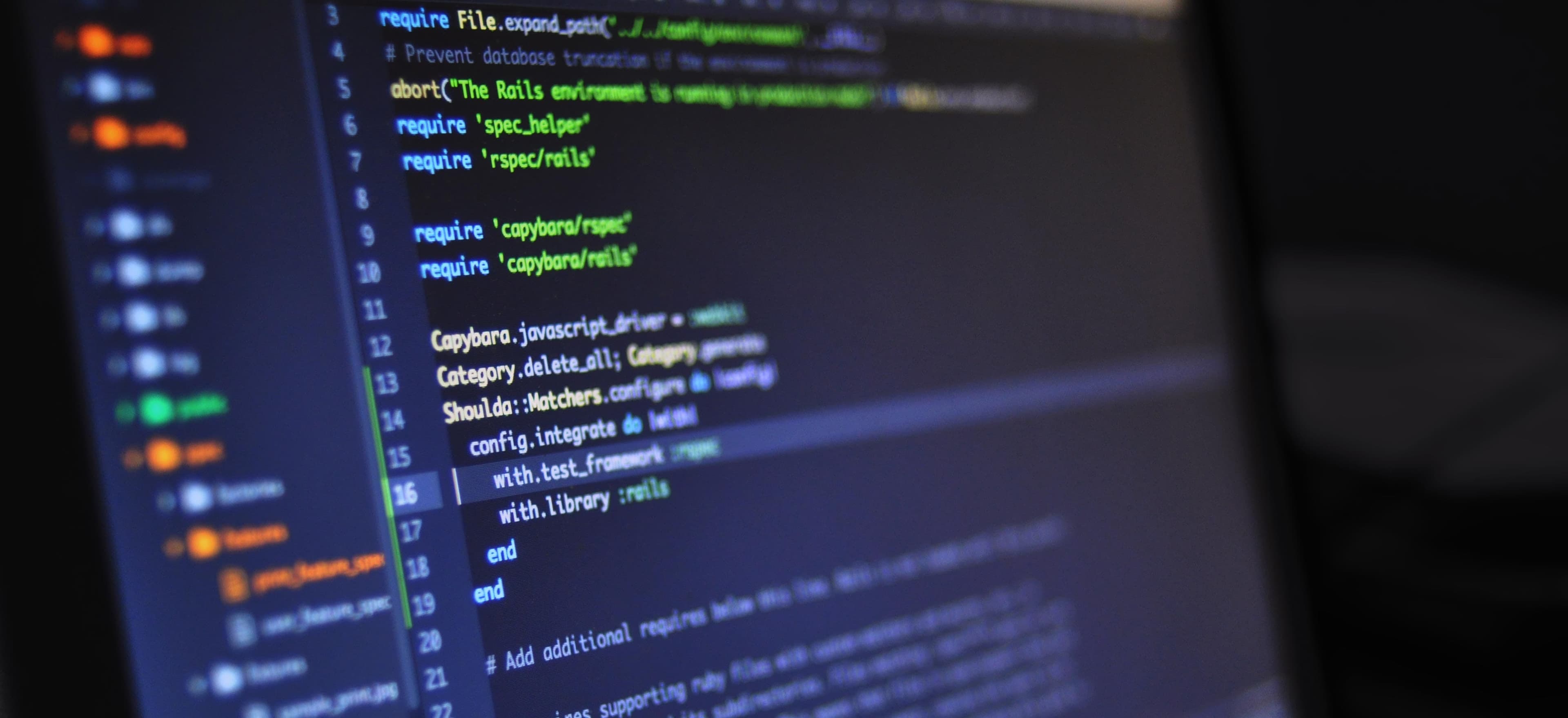
- Published on
Common Pitfalls in Hibernate DDL Schema Generation
Hibernate's Data Definition Language (DDL) schema generation can greatly simplify the development process, allowing developers to automate the creation and management of database tables according to their object-oriented models. However, while it provides significant benefits, pitfalls can lead to frustrating errors and inefficient database architecture. In this post, we will explore common pitfalls in Hibernate DDL schema generation, including best practices to avoid them.
Understanding Hibernate DDL Schema Generation
Hibernate allows users to automatically generate database schemas through configuration parameters and annotations in entity classes. This can be particularly useful during development, as it helps maintain consistency between the database schema and the application model.
Typical usage involves properties in the hibernate.cfg.xml
or in the application.properties
file depending on the configuration style:
<property name="hibernate.hbm2ddl.auto">update</property>
This line would update the database schema based on the changes in entity classes without the risk of dropping tables, allowing for iterative development.
Common Pitfalls
1. Not Configuring the DDL Auto Property Correctly
One of the most common mistakes is misconfiguring the hibernate.hbm2ddl.auto
property. The options available include:
- create: Drops existing tables and creates new ones.
- create-drop: Creates tables on session factory creation and drops them on shutdown.
- update: Updates the schema based on the entity classes.
- validate: Validates the schema against the entity model.
- none: No action; this is generally not advised.
Improper understanding can lead to data loss or unintentional database changes.
Example Configuration:
hibernate.hbm2ddl.auto=update
Why? This option is ideal for development but should be approached with caution in production, as updates could unintentionally alter the schema.
2. Ignoring Naming Strategies
By default, Hibernate uses PhysicalNamingStrategyStandardImpl
which translates class and field names to tables and columns directly. However, many organizations have naming conventions (e.g., snake_case for table names).
Example of Custom Naming Strategy:
import org.hibernate.boot.model.naming.Identifier;
import org.hibernate.boot.model.naming.PhysicalNamingStrategy;
import org.hibernate.engine.jdbc.env.spi.JdbcEnvironment;
public class CustomNamingStrategy implements PhysicalNamingStrategy {
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return new Identifier(name.getText().toLowerCase(), name.isQuoted());
}
}
Why? This allows developers to ensure that the generated schema adheres to corporate standards, preventing issues down the line.
3. Overlooking Cascade Types in Relationships
When defining relationships, like ManyToOne or OneToMany, it's crucial to specify the cascade type properly. Failure to do so can lead to orphaned records or unintended deletions.
Example of Entity with Cascade Types:
@Entity
public class Course {
@OneToMany(mappedBy = "course", cascade = CascadeType.ALL)
private List<Student> students;
}
Why? By using CascadeType.ALL
, when a course is deleted, all its associated students are also deleted, maintaining data integrity.
4. Failing to Annotate Entity Fields Properly
Annotations direct Hibernate on how to treat class properties. Forgetting to annotate fields can lead to unexpected behaviors.
Example:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, length = 100)
private String username;
@Column(unique = true)
private String email;
}
Why? Here, @Column
annotations define constraints like nullable
and unique
, which will enforce database-level validations and improve data integrity.
5. Mistaking Relationships and Fetch Types
When establishing relationships, developers often overlook fetch types, which can lead to performance issues.
Example of Eager vs Lazy Fetch:
@Entity
public class Teacher {
@OneToMany(mappedBy = "teacher", fetch = FetchType.LAZY)
private List<Course> courses;
}
Why? Using FetchType.LAZY
loads data on demand rather than at once, optimizing performance, especially when dealing with large datasets.
6. Ignoring Database Constraints
Hibernate schema generation can create constraints based on entity mappings, but lapses in defining fields (such as foreign keys) can lead to loss of data integrity.
Example of Foreign Key Definition:
@Entity
public class Order {
@ManyToOne
@JoinColumn(name = "user_id", referencedColumnName = "id", nullable = false)
private User user;
}
Why? This ensures that an order cannot exist without being linked to a user, maintaining relational integrity.
7. Deployment Configurations
Different configurations may be required for development versus production. The DDL generation settings used in development (e.g., hibernate.hbm2ddl.auto=update
) should not be applied in production environments, where data integrity is critical.
Best Practice: Set the property to none or validate in production:
hibernate.hbm2ddl.auto=validate
Why? This ensures no unintended changes occur, preserving the integrity of the production database.
8. Ignoring Versioning for Concurrency Control
In a collaborative development environment, version control for entities is essential. Failing to implement versioning can lead to lost updates.
Example with Version Field:
@Entity
public class Product {
@Id
private Long id;
@Version
private Long version;
private String name;
}
Why? The @Version
annotation allows Hibernate to manage and detect conflicting updates gracefully.
Lessons Learned
Hibernate's DDL schema generation can significantly ease development and ensure database consistency, but it's critical to avoid common pitfalls. Configuration management, relationship handling, field annotations, and environmental distinctions play pivotal roles in ensuring a robust database framework.
While this post has outlined key mistakes and how to sidestep them, continuous learning, reading Hibernate documentation, and engaging with the community can provide valuable insights. For deeper exploration into Hibernate mappings and specifications, consider reading the official Hibernate Documentation.
By adhering to these insights and improving your understanding of Hibernate's capabilities, you can create a scalable, maintainable, and efficient database management system that serves your application's needs effectively.