Common Mistakes New Hazelcast Users Make and How to Avoid Them
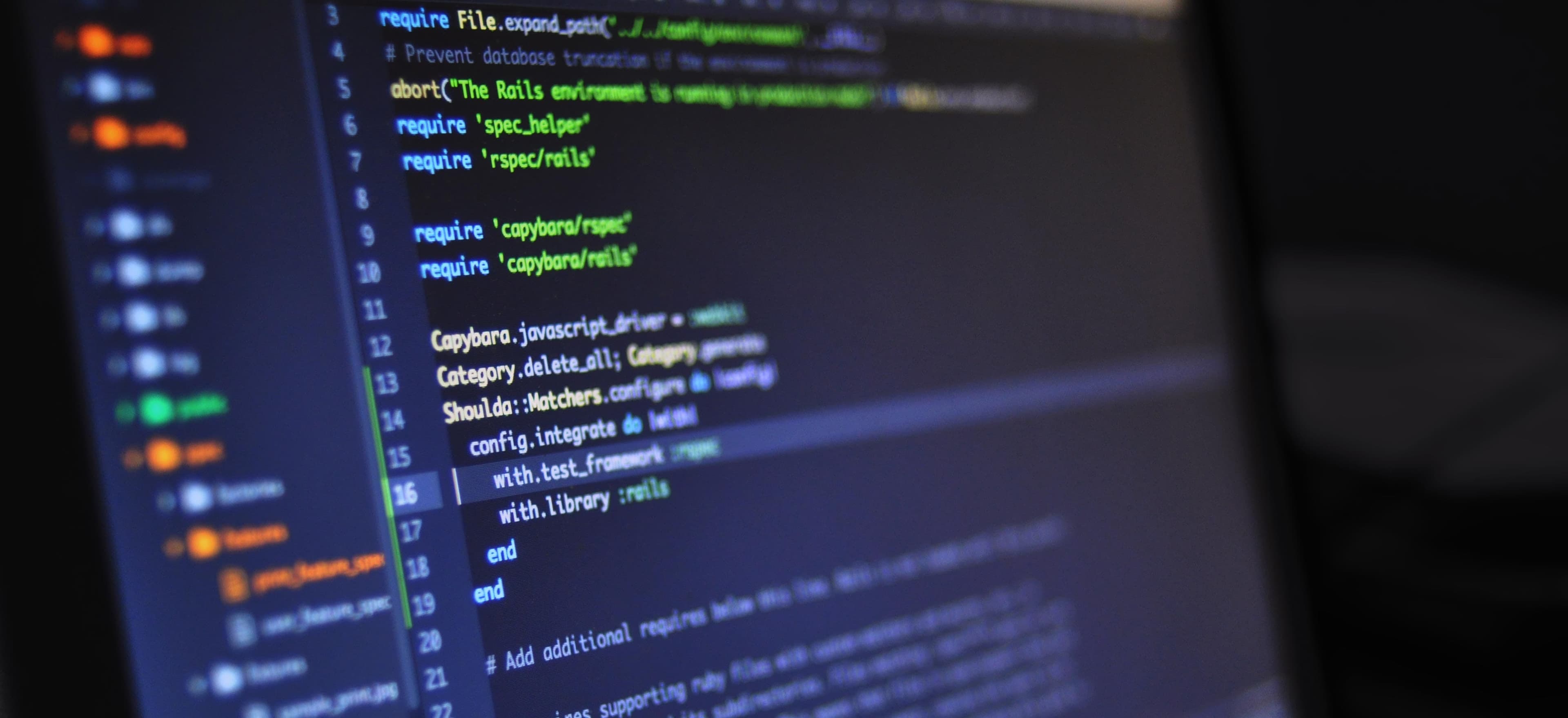
- Published on
Common Mistakes New Hazelcast Users Make and How to Avoid Them
Hazelcast is a powerful in-memory data grid (IMDG) solution, commonly used for distributed computing and data caching. While its flexibility and capabilities are remarkable, new users can often find themselves falling into common pitfalls. This article will explore these mistakes and provide actionable advice to help you avoid them and leverage Hazelcast effectively for your applications.
Understanding Hazelcast Basics
Before diving into the specific mistakes, it's essential to understand what Hazelcast is and how it works. Hazelcast allows you to create distributed data structures, perform distributed computing, and offers reliable data storage with features like clustering, data partitioning, and replication. Its seamless integration with Java makes it a popular choice among developers aiming to build scalable applications.
Mistake 1: Not Understanding the Data Partitioning
The Issue
One of the most significant advantages of Hazelcast is its ability to partition data across different nodes. However, many new users neglect to grasp how partitioning actually works and how to utilize it effectively.
The Solution
When you store data in Hazelcast, it's distributed based on the hash of the key. This balancing act is crucial for performance. If your data is not sufficiently randomized, you might end up with hotspots—nodes that become heavily loaded while others sit idle.
Example Code Snippet
IMap<String, String> map = hazelcastInstance.getMap("my-distributed-map");
// Storing data
for (int i = 0; i < 100; i++) {
map.put("key-" + i, "value-" + i);
}
Why this is important: Ensure your keys have a good randomness to avoid issues with partitioning. Choosing unique and well-distributed keys for your data will enhance performance and efficiency.
Mistake 2: Ignoring Backup Policies
The Issue
New users often overlook Hazelcast's backup features. Failing to configure backups can lead to data loss in case of node failure.
The Solution
Always set up backup copies of your data. Hazelcast allows you to define the backup count when creating a map, which can provide redundancy.
Example Code Snippet
Config config = new Config();
MapConfig mapConfig = new MapConfig("my-distributed-map")
.setBackupCount(1);
config.addMapConfig(mapConfig);
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance(config);
Why this is important: Backups ensure your data is resilient and can be recovered in the event of node failure. Define your backup count according to how critical your data is.
Mistake 3: Not Tuning the Memory Settings
The Issue
Hazelcast's memory usage parameters can significantly affect performance. New users frequently accept the default memory settings, which may not align with their workload.
The Solution
Fine-tune the JVM settings and configure Hazelcast memory settings according to your application’s needs. This includes heap size, off-heap storage, and eviction policies.
Example Code Snippet
Config config = new Config();
config.getSerializationConfig()
.setPortableVersion(1);
Why this is important: Proper memory tuning can help avoid out-of-memory errors and improve application performance under heavy load. Customize these settings during testing and production.
Mistake 4: Misconfiguring Network Settings
The Issue
Networking issues can impede Hazelcast's effectiveness. New users often overlook parameters like network.port
and clustering settings.
The Solution
Ensure that the network settings are correctly configured to allow nodes to communicate effectively. Be aware of the network interfaces and firewalls in use.
Example Code Snippet
Config config = new Config();
NetworkConfig networkConfig = config.getNetworkConfig();
networkConfig.setPublicAddress("192.168.1.100")
.setPort(5701);
Why this is important: Proper network configuration ensures that all Hazelcast nodes can join the cluster and communicate without interruption.
Mistake 5: Lack of Monitoring and Management Tools
The Issue
Many new Hazelcast users neglect to use monitoring tools. Failing to monitor cluster health can lead to issues going unresolved for extended periods.
The Solution
Leverage Hazelcast Management Center to keep an eye on the performance of your cluster. Regular monitoring can help you catch scaling and resource issues proactively.
Example Code Snippet (Connection)
ManagementCenterConfig managementCenterConfig = new ManagementCenterConfig();
managementCenterConfig.setEnabled(true);
managementCenterConfig.setUrl("http://localhost:8080/mancenter");
Config config = new Config();
config.setManagementCenterConfig(managementCenterConfig);
Why this is important: Effective monitoring allows for problem detection before they impact your application. Use these tools to keep your cluster healthy.
Mistake 6: Skipping Documentation
The Issue
New developers often dive into coding without fully reviewing Hazelcast's documentation. This can lead to misunderstandings and missed opportunities to utilize powerful features.
The Solution
Make it a habit to consult the Hazelcast Documentation before implementation. Understanding the various features available can enhance your usage.
Why this is important: Familiarizing yourself with the documentation allows you to utilize Hazelcast's capabilities effectively. You will learn about new features, improvements, and best practices.
A Final Look
Switching to Hazelcast can be a game-changer for your application's performance and scalability. However, not understanding its intricacies can lead to avoidable mistakes. By gaining a deeper understanding of partitioning, backups, memory tuning, network settings, monitoring, and thorough documentation reading, you can avoid the common pitfalls many new users face.
As you embark on your journey with Hazelcast, always remember: it’s not just about the code you write but the choices you make around architecture and configuration that will set the foundation for your success.
For more insights into best practices and advanced topics related to Hazelcast, consider following the Hazelcast Official Blog for continuous learning and updates.
By avoiding these common mistakes, you’ll not only improve your own skills but also contribute to the development of stable and scalable applications in your organization. Happy coding!
Checkout our other articles