The Pitfalls of Test-Driven Development You Need to Avoid
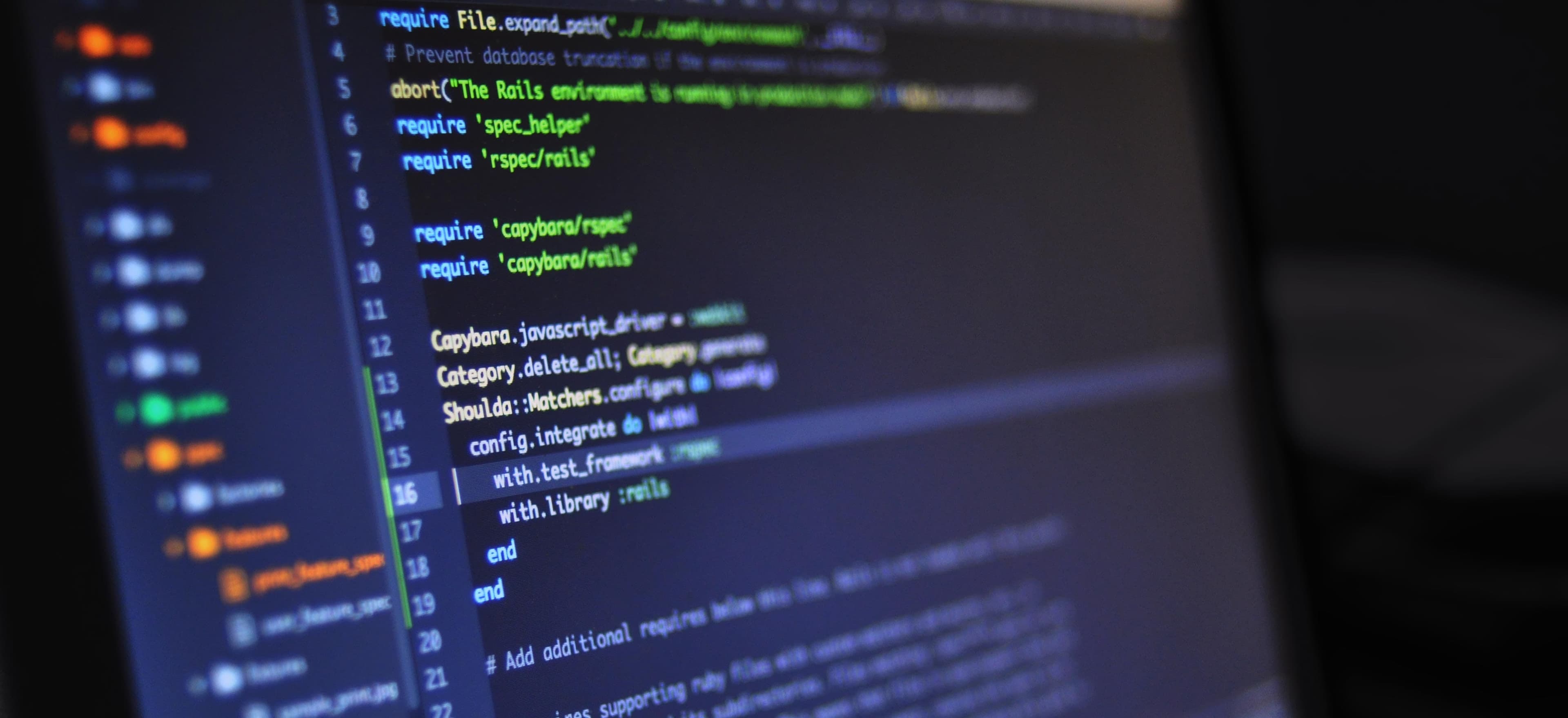
- Published on
The Pitfalls of Test-Driven Development You Need to Avoid
Test-Driven Development (TDD) has gained popularity as a key methodology within the software development landscape. The allure of TDD lies in its promise of higher-quality code, enhanced design flexibility, and fewer bugs in production. However, like any methodology, TDD is not without its pitfalls. This blog post explores the common challenges developers face when implementing TDD and how to avoid them.
What is Test-Driven Development?
Before diving into the pitfalls, let’s briefly explain what TDD is. In TDD, developers write tests for a new feature before even writing the corresponding code. The cycle typically follows this pattern:
- Write a Test: Start by writing a failing test that defines a function or improvement.
- Run the Test: Execute the test to confirm that it fails (as expected).
- Write Code: Write the minimal code necessary to pass the test.
- Run the Test Again: Ensure the new code passes the test.
- Refactor: Optimize the code while ensuring the tests still pass.
This cycle is often referred to as the "Red-Green-Refactor" cycle.
Pitfall 1: Writing Too Many Tests
One of the first pitfalls new TDD practitioners encounter is writing an excessive number of tests. While having tests is crucial, it’s easy to overdo it.
Why it matters: Too many tests can lead to maintenance headaches. Each test you write also needs to be maintained, and overflowing test suites can slow down the development process and drown out meaningful feedback.
Solution: Focus on writing tests that matter. Prioritize testing critical components and edge cases. For example, if you’re developing a login feature, ensure you test for valid credentials, invalid credentials, and account lockout scenarios, but avoid redundant tests that don’t add new information.
@Test
public void testUserLogin() {
// Arrange
User user = new User("username", "password");
AuthenticationService authService = new AuthenticationService();
// Act
boolean result = authService.login(user);
// Assert
assertTrue(result, "User should log in successfully with valid credentials.");
}
Pitfall 2: Ignoring Refactoring
Refactoring is at the heart of TDD, yet many developers overlook it. The urge to keep moving forward with new features can lead to neglecting the code that’s already been written.
Why it matters: If you don't refactor your code regularly, you risk accumulating technical debt. Code can become convoluted and difficult to maintain, defeating the purpose of TDD in the first place.
Solution: Regularly schedule refactoring sessions in your development routine. You can introduce a “refactor Friday” to encourage the practice within your team.
// Before refactoring
public String formatUserDetails(User user) {
return user.getUsername() + " - " + user.getEmail() + " - " + user.getPhone();
}
// After refactoring
public String formatUserDetails(User user) {
return String.join(" - ", user.getUsername(), user.getEmail(), user.getPhone());
}
Pitfall 3: Focusing Solely on Unit Tests
TDD commonly emphasizes unit tests. While they are essential, solely focusing on them can obstruct your ability to fully capture system requirements.
Why it matters: Unit tests verify isolated components, but they may overlook interactions between those components. Relying only on unit tests can lead to integration issues that mightn't manifest until late in the development cycle.
Solution: Complement your TDD approach with integration tests. These tests assess how different pieces of your system work together and catch issues that unit tests may miss.
@Test
public void testUserIntegration() {
// Arrange
UserService userService = new UserService();
AuthService authService = new AuthService(userService);
// Act
String token = authService.login("username", "password");
// Assert
assertNotNull(token, "A valid token should be returned upon successful login.");
}
Pitfall 4: Skipping Test Maintenance
As your codebase evolves, so do its tests. Neglecting the maintenance of tests can lead to a situation known as "test rot".
Why it matters: Outdated tests can produce false positives or negatives, leading to confusion and incorrect assumptions about code quality.
Solution: Regularly review your tests. When code changes, ensure you update the corresponding tests. Consider implementing a code review process specifically for tests to maintain quality.
Pitfall 5: Overly Complicated Test Cases
Overcomplicating test cases can make them harder to read and maintain. It’s tempting to squeeze multiple assertions or intricate logic into a single test.
Why it matters: Complex test cases can obscure their purpose and make diagnosing failures difficult. Simple tests are usually more reliable and easier to understand.
Solution: Keep test cases focused and straightforward. If a test appears convoluted, consider splitting it into multiple tests.
// Poorly designed test case
@Test
public void testMultipleConditions() {
assertEquals(5, add(2, 3));
assertEquals(6, add(2, 4));
assertTrue(isValid("username"));
}
// Better design
@Test
public void testAddTwoNumbers() {
assertEquals(5, add(2, 3));
}
@Test
public void testIsValidUser() {
assertTrue(isValid("username"));
}
To Wrap Things Up
Test-Driven Development is a powerful tool for developers, but it’s important to navigate its pitfalls wisely. By avoiding excessive tests, prioritizing refactoring, including integration testing, maintaining tests, and keeping cases simple, you can harness the full benefits of TDD while mitigating its drawbacks.
Moreover, remember that TDD might not be a universal solution. It works best in certain scenarios, and understanding when to implement it—and when to apply different methodologies—is key to effective software development.
For a deeper insight into testing methodologies, consider reading articles on Agile Testing and the testing pyramid concept.
Following these guidelines will ensure your TDD journey is smooth and fruitful, ultimately leading to cleaner code and a more robust application. Happy coding!
Checkout our other articles