Common Pitfalls in Customizing HttpMessageConverters in Spring
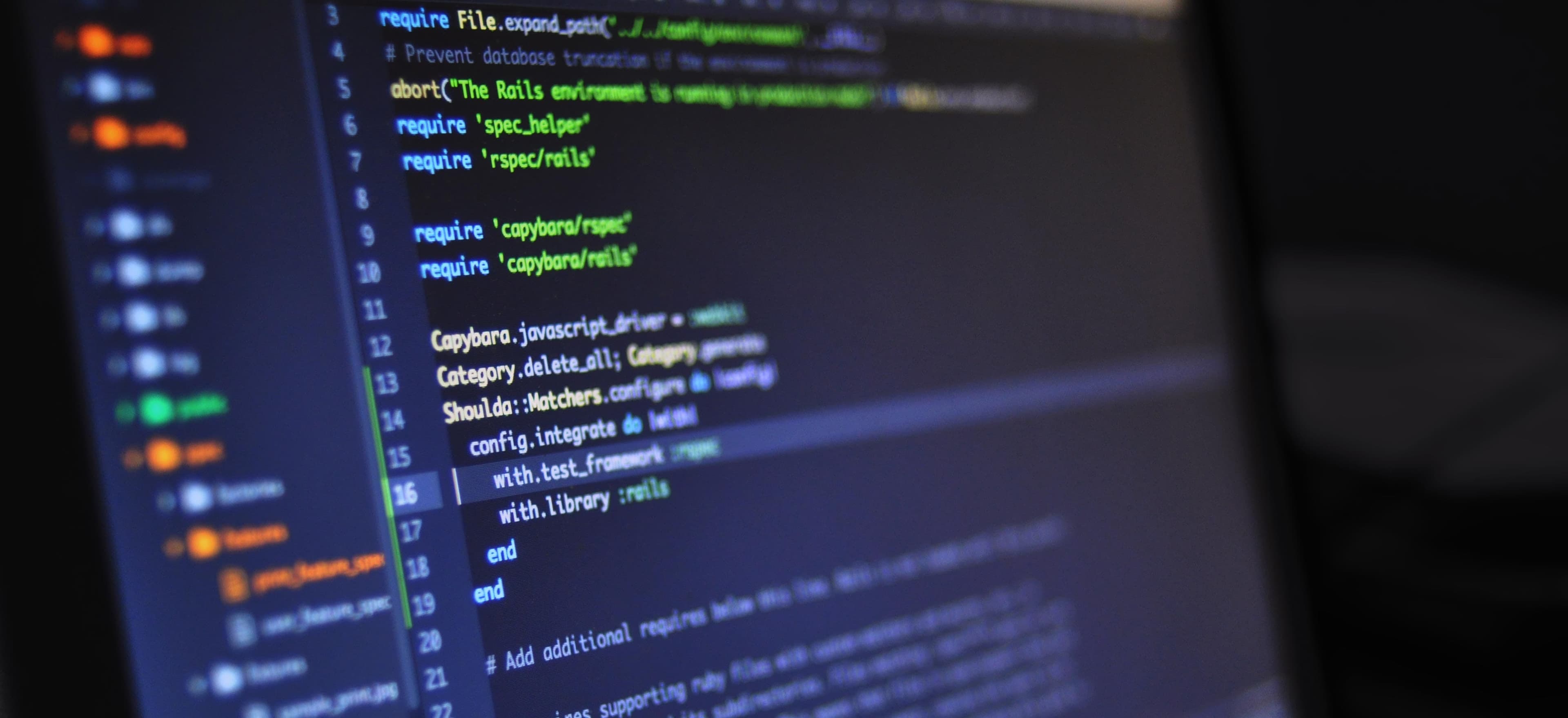
- Published on
Common Pitfalls in Customizing HttpMessageConverters in Spring
Customizing HttpMessageConverters
in Spring is often a powerful approach to manipulating the serialization and deserialization process of HTTP requests and responses. However, this fundamental capability comes with its own set of pitfalls that developers should be cautious of. In this blog post, we’ll explore these challenges while providing clear, actionable guidance on how to avoid them.
Understanding HttpMessageConverters
Spring MVC comes equipped with several built-in HttpMessageConverters
that handle data formats like JSON, XML, and others. When you customize these converters, you’re essentially altering how data is converted between Java objects and HTTP messages.
Why Customize HttpMessageConverters?
- Special Requirements: Sometimes, you have to deal with specific data formats or structures that aren’t readily supported by default converters.
- Performance Optimization: Custom converters may offer optimizations tailored to your specific requirements, reducing overhead and enhancing performance.
- Business Logic: Implementing business-specific serialization logic directly within converters can help keep your controllers cleaner and more focused.
Common Pitfalls and How to Avoid Them
1. Overriding Default Behavior
One of the most frequent mistakes is inadvertently overriding Spring’s default HttpMessageConverters
. If your converter has conflicting logic or is incorrectly registered, it might interfere with the default converters.
Solution
Always consider whether you truly need to override existing converters. If you do, make sure to chain to the default converters when necessary. Here’s how:
import org.springframework.context.annotation.Bean;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import java.util.List;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
converters.add(new MappingJackson2HttpMessageConverter());
customConverter(converters);
}
private void customConverter(List<HttpMessageConverter<?>> converters) {
// Add your custom HttpMessageConverter here
}
}
This code snippet shows how to extend the existing configuration while retaining default behavior.
2. Not Considering Media Types
Many developers do not account for the content types returned by their converters. Failure to specify media types can lead to unexpected behavior, especially with requests and responses.
Solution
Ensure that your custom converters explicitly specify the media types they handle:
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.http.HttpOutputMessage;
import org.springframework.http.HttpInputMessage;
import org.springframework.http.MediaType;
import org.springframework.http.converter.JsonHttpMessageConverter;
public class CustomJsonMessageConverter extends JsonHttpMessageConverter {
public CustomJsonMessageConverter() {
// Specify media types
setSupportedMediaTypes(List.of(MediaType.APPLICATION_JSON));
}
@Override
protected void writeInternal(Object object, HttpOutputMessage outputMessage) throws IOException {
super.writeInternal(object, outputMessage);
}
@Override
protected Object readInternal(Class<?> clazz, HttpInputMessage inputMessage) throws IOException {
return super.readInternal(clazz, inputMessage);
}
}
This code demonstrates a custom JSON message converter with a focus on media types, which contributes to proper request handling.
3. Ignoring the Order of Message Converters
The order of HttpMessageConverters
can significantly affect how the system serializes and deserializes messages. If a converter that can handle a specific media type is placed after another converter, it may never be utilized.
Solution
You can control the order of the converters by modifying the list. Be mindful of placements based on their capabilities:
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
converters.add(0, new CustomJsonMessageConverter()); // High priority
converters.add(new MappingJackson2HttpMessageConverter()); // Lower priority
}
In this example, CustomJsonMessageConverter
is placed at the top, ensuring it takes precedence for JSON requests.
4. Not Handling Errors Appropriately
Custom converters may fail to handle errors during parsing or serialization. Failing to manage these errors could lead to unhelpful HTTP responses and poor user experience.
Solution
Wrap the core logic within try-catch blocks to handle exceptions gracefully. You can customize the response as follows:
@Override
protected Object readInternal(Class<?> clazz, HttpInputMessage inputMessage) throws IOException {
try {
return super.readInternal(clazz, inputMessage);
} catch (IOException e) {
// Handle IOException
throw new CustomException("Error occurred while reading", e);
}
}
This ensures that your application won’t crash due to unexpected parsing issues and allows you to return meaningful error messages.
5. Not Testing Your Custom Converter
Failing to test the custom message converters adequately is a common mistake. Even minor changes could significantly impact serialization/deserialization outcomes.
Solution
Unit tests are essential for your converters. Use frameworks like JUnit or Mockito to validate your converter logic.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CustomJsonMessageConverterTest {
@Test
void testSerialization() throws Exception {
CustomJsonMessageConverter converter = new CustomJsonMessageConverter();
String json = converter.write(...); // Assuming write method exists
assertEquals(expectedJson, json);
}
}
Building tests around your converters ensures they function as expected in various scenarios, minimizing risk.
To Wrap Things Up
Customizing HttpMessageConverters
in Spring can greatly enhance your web application’s flexibility and performance. However, as we've seen, it comes with its own set of challenges. By avoiding these common pitfalls, you set a solid foundation for building rich, efficient API endpoints.
For additional resources, consider checking out the official Spring documentation on content negotiation and Baeldung's guide on custom message converters for a deeper dive into practical implementations.
Remember, thoughtful customization can make a significant difference. Avoid these pitfalls, focus on clear logic, and your custom message converters will serve your application well. Happy coding!
Checkout our other articles