Common Pitfalls When Using LangChain4j for JSON Outputs
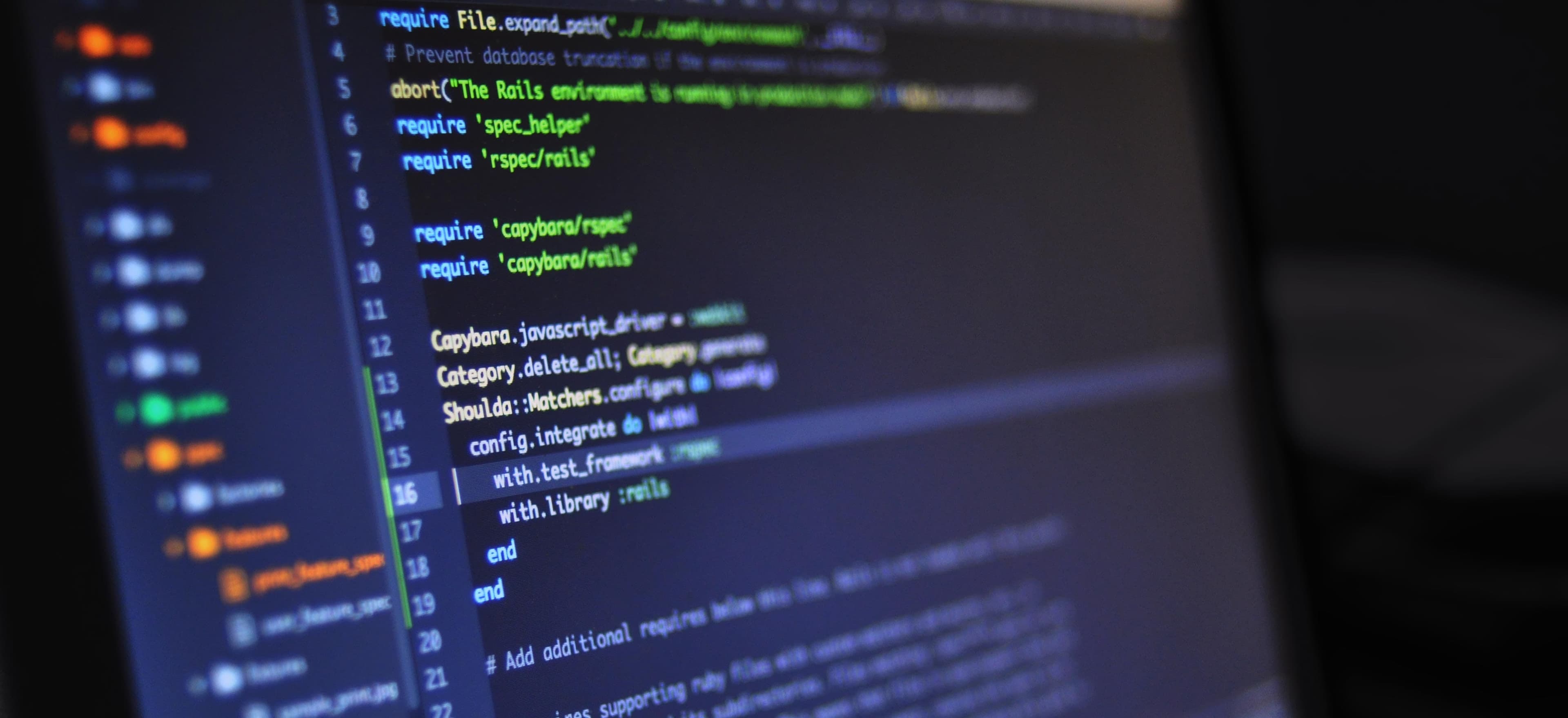
- Published on
Common Pitfalls When Using LangChain4j for JSON Outputs
As the landscape of Natural Language Processing (NLP) continues to evolve, developers increasingly seek efficient ways to integrate language models into their applications. One such exciting framework is LangChain4j, which offers a powerful toolkit for building applications with string-oriented components like prompts and outputs. In this blog post, we'll delve into some common pitfalls when using LangChain4j, particularly focusing on generating JSON outputs.
Why Use LangChain4j for JSON Outputs?
LangChain4j is cemented in the Java ecosystem, allowing developers to harness the power of language models while writing efficient and manageable code. When generating JSON outputs, LangChain4j provides exceptional versatility that can streamline development. However, pitfalls lurk in its usage.
Before we dive into the common pitfalls, let’s ensure you have the LangChain4j library. You can include it in your Maven project as follows:
<dependency>
<groupId>com.langchain4j</groupId>
<artifactId>langchain4j-core</artifactId>
<version>0.1.0</version> <!-- Check for the latest version -->
</dependency>
Common Pitfalls in LangChain4j When Working with JSON
1. Improper Data Type Handling
The Pitfall
One common mistake is neglecting data type validation. When assembling your JSON responses, Java's strong typing can work against you.
Why It Matters
If you're not careful, you may end up with a NullPointerException
or a malformed JSON object.
Example Code
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataProcessor {
private ObjectMapper mapper = new ObjectMapper();
public String processAndConvertToJson(String key, Object value) {
if (key == null || value == null) {
throw new IllegalArgumentException("Key and value must not be null");
}
Map<String, Object> data = new HashMap<>();
data.put(key, value);
try {
return mapper.writeValueAsString(data);
} catch (JsonProcessingException e) {
throw new RuntimeException("Failed to process JSON", e);
}
}
}
Commentary
In this example, the processAndConvertToJson
method checks for null inputs. Such validations are crucial to prevent runtime exceptions, allowing smoother execution of your application.
2. Ignoring Character Encoding
The Pitfall
Another common issue arises from character encoding. If your JSON output includes special characters, you might run into instances where encoding mishandles those characters.
Why It Matters
Improperly encoded data can lead to reading issues on the client side, affecting user experience and potentially leading to data corruption.
Example Code
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
public String createJsonOutput(String name) {
try {
String encodedName = URLEncoder.encode(name, StandardCharsets.UTF_8.toString());
return "{\"name\": \"" + encodedName + "\"}";
} catch (UnsupportedEncodingException e) {
throw new RuntimeException("Encoding not supported", e);
}
}
Commentary
Here, we utilize URLEncoder
to ensure any special characters in the name don't disrupt the JSON format. This keeps your output safe and predictable.
3. Outdated Library Versions
The Pitfall
Many developers forget to keep their libraries updated. Using outdated versions of LangChain4j can lead to unexpected results, especially with JSON outputs where improvements and optimizations may have been introduced in recent releases.
Why It Matters
Staying updated ensures you benefit from fixes, new features, and performance improvements.
Additional Resources
To stay updated on the latest LangChain4j developments, check their official documentation or GitHub repository.
4. Lack of Error Handling
The Pitfall
Inadequate error handling can lead to poor user experience. When processing JSON outputs, unexpected situations can arise, like malformed data, and failing to anticipate these can cripple your application.
Why It Matters
Error handling is critical for reliability. Users should receive informative feedback rather than vague error messages.
Example Code
public String safeProcessAndConvertToJson(String key, Object value) {
try {
return processAndConvertToJson(key, value);
} catch (IllegalArgumentException e) {
return "{\"error\": \"Invalid input: " + e.getMessage() + "\"}";
} catch (RuntimeException e) {
return "{\"error\": \"Internal server error\"}";
}
}
Commentary
In this example, we encapsulate our JSON processing logic in a try-catch block. The JSON error outputs provide clearer feedback, helping developers debug and enhancing user experience.
5. Not Utilizing Asynchronous Processing
The Pitfall
When dealing with high-volume data outputs, blocking the main thread can slow down your application. Some developers overlook asynchronous APIs that LangChain4j provides.
Why It Matters
Utilizing asynchronous processing can greatly improve the responsiveness of your application, providing a smoother and faster user interaction.
Example Code
import java.util.concurrent.CompletableFuture;
public CompletableFuture<String> asyncConvertToJson(String key, Object value) {
return CompletableFuture.supplyAsync(() -> processAndConvertToJson(key, value));
}
Commentary
In this code snippet, we employ CompletableFuture
to perform JSON processing in a non-blocking manner. This allows other tasks to continue executing while waiting for the JSON output, significantly enhancing the usability of your application.
A Final Look
The use of LangChain4j offers substantial potential for developing robust Java applications powered by language models. However, while generating JSON outputs, developers must navigate several common pitfalls.
Improper data handling, ignoring character encoding, outdated library versions, insufficient error handling, and the lack of asynchronous processing can lead to inefficiencies and negative user experiences. Being aware of these pitfalls will allow you to galvanize your application’s performance and reliability.
By adhering to best practices and consistent coding standards, you can harness the full potential of LangChain4j for effective JSON outputs. Happy coding!
Checkout our other articles