Mastering Java: Displaying Adjacent Lines in File Matching
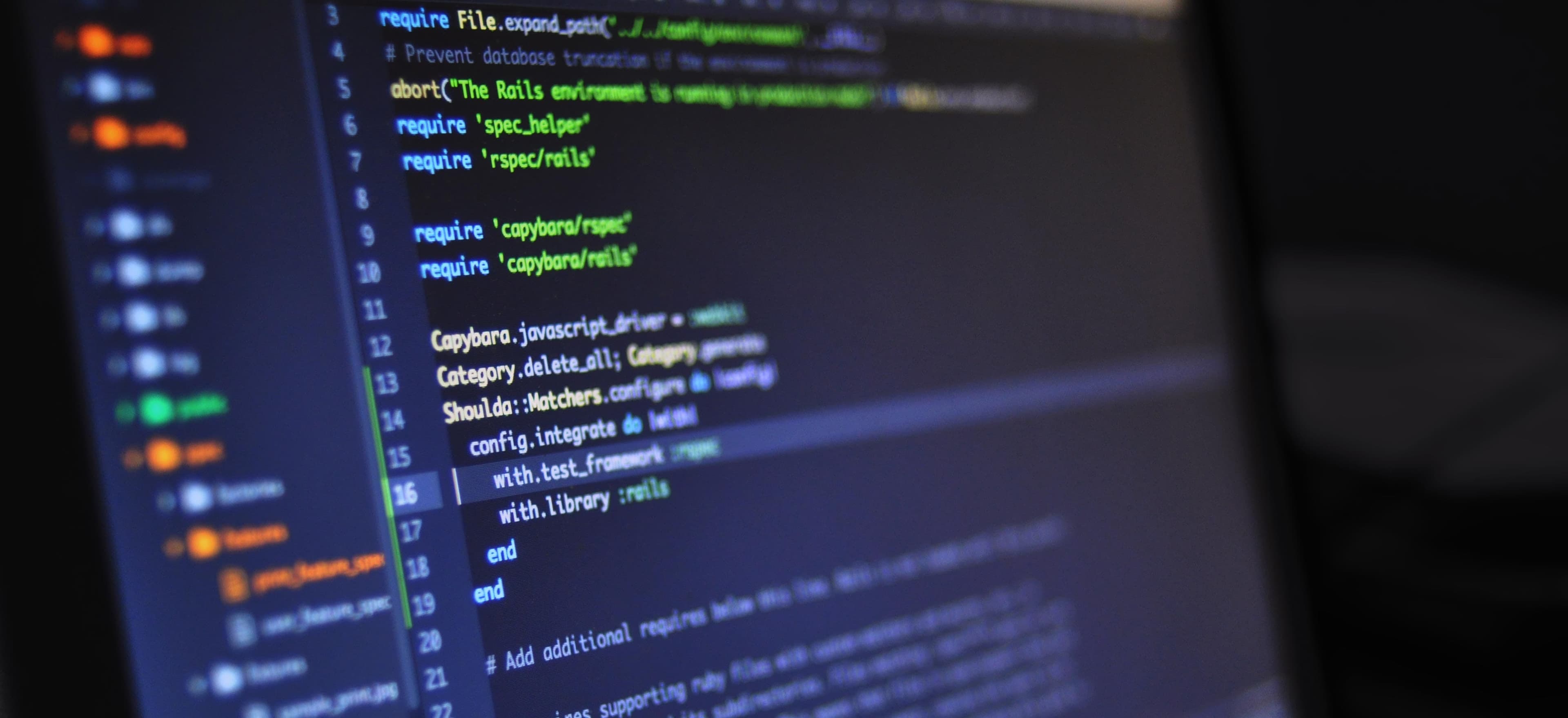
- Published on
Mastering Java: Displaying Adjacent Lines in File Matching
Java, as a robust and versatile programming language, offers an array of capabilities, especially for file handling. One common task developers encounter is searching through text files for specific data and displaying information based on certain conditions. In this blog post, we will focus on how to read a file in Java, search for specific lines that match a given pattern, and display adjacent lines.
Table of Contents
- Understanding the Problem
- Setting Up Your Java Environment
- Code Implementation
- 3.1 Reading the File
- 3.2 Finding Matches and Displaying Adjacent Lines
- Testing Our Code
- Conclusion
Understanding the Problem
Imagine you have a log file or a text data file containing thousands of lines, and you need to find lines of interest (based on user-defined criteria). For example, if you are sifting through logs for errors, it is often important to see what happened immediately before and after the error message. This is where displaying adjacent lines comes in handy.
Setting Up Your Java Environment
Before we proceed, ensure that you have Java installed on your machine. You can download it from the official Java SE Development Kit. You will also need an IDE (Integrated Development Environment) such as IntelliJ IDEA or Eclipse to run your code smoothly.
Project Structure
- Create a new Java project in your IDE.
- In the
src
folder, create a new class namedAdjacentLinesFinder.java
.
Code Implementation
3.1 Reading the File
First, we need to create a method to read the contents of the file. Here we will use BufferedReader
for efficient reading.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class AdjacentLinesFinder {
public static List<String> readFile(String filePath) throws IOException {
List<String> lines = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
lines.add(line);
}
}
return lines;
}
}
Explanation:
- We utilize
BufferedReader
, which offers efficient reading of characters, arrays, and lines. - The method reads the file line-by-line until it reaches the end.
- Each line is added to a
List<String>
for further processing.
3.2 Finding Matches and Displaying Adjacent Lines
Now, we will implement the core functionality where we find the lines that match a given keyword and display the lines adjacent to them.
public void findAndDisplayAdjacentLines(List<String> lines, String keyword) {
for (int i = 0; i < lines.size(); i++) {
if (lines.get(i).contains(keyword)) {
System.out.println("Matched Line: " + lines.get(i));
if (i > 0) {
System.out.println("Previous Line: " + lines.get(i - 1));
}
if (i < lines.size() - 1) {
System.out.println("Next Line: " + lines.get(i + 1));
}
System.out.println(); // Empty line for better readability
}
}
}
Explanation:
- The method iterates over each line in the input list.
- If a line contains the specified keyword, it prints that line.
- It checks and prints the previous line (if exists) and the next line accordingly.
- Using
System.out.println()
facilitates easy debugging and provides clear, console output.
Bringing It All Together
Here is how we would call the above methods inside the main
function:
public static void main(String[] args) {
String filePath = "path/to/your/file.txt"; // Change to your file path
String keyword = "ERROR"; // Sample keyword to search for
try {
List<String> lines = readFile(filePath);
AdjacentLinesFinder finder = new AdjacentLinesFinder();
finder.findAndDisplayAdjacentLines(lines, keyword);
} catch (IOException e) {
System.err.println("Error reading file: " + e);
}
}
Explanation:
- The
main
method begins the program. - You can specify the path to your target file and the keyword for searching.
- The code engages in proper exception handling to ensure that any I/O issues are gracefully reported.
Testing Our Code
- Create a sample text file and populate it with varied data, including instances of your target keyword.
- Run your
AdjacentLinesFinder
to see the output.
Example Input File
INFO: Application started
ERROR: Unable to connect to database
INFO: Retrying connection
ERROR: Database timeout error
INFO: Application closed
Expected Output
Matched Line: ERROR: Unable to connect to database
Previous Line: INFO: Application started
Next Line: INFO: Retrying connection
Matched Line: ERROR: Database timeout error
Previous Line: INFO: Retrying connection
Next Line: INFO: Application closed
Bringing It All Together
In this tutorial, you learned how to read lines from a file in Java, search for matching lines, and effectively display their adjacent lines. This approach can be particularly useful in various applications like log processing, data validation, and performance monitoring.
The code snippets we developed can easily be expanded upon, letting you adapt the logic to suit your specific needs. Consider diving deeper into Java's filesystem libraries like java.nio.file
for more complex operations and explorations.
For more on Java I/O operations, check out the official Java Documentation.
With these fundamentals, you're well on your way to mastering file operations in Java! Happy coding!
Checkout our other articles