Maximizing ServletRequest startAsync Limits for Performance
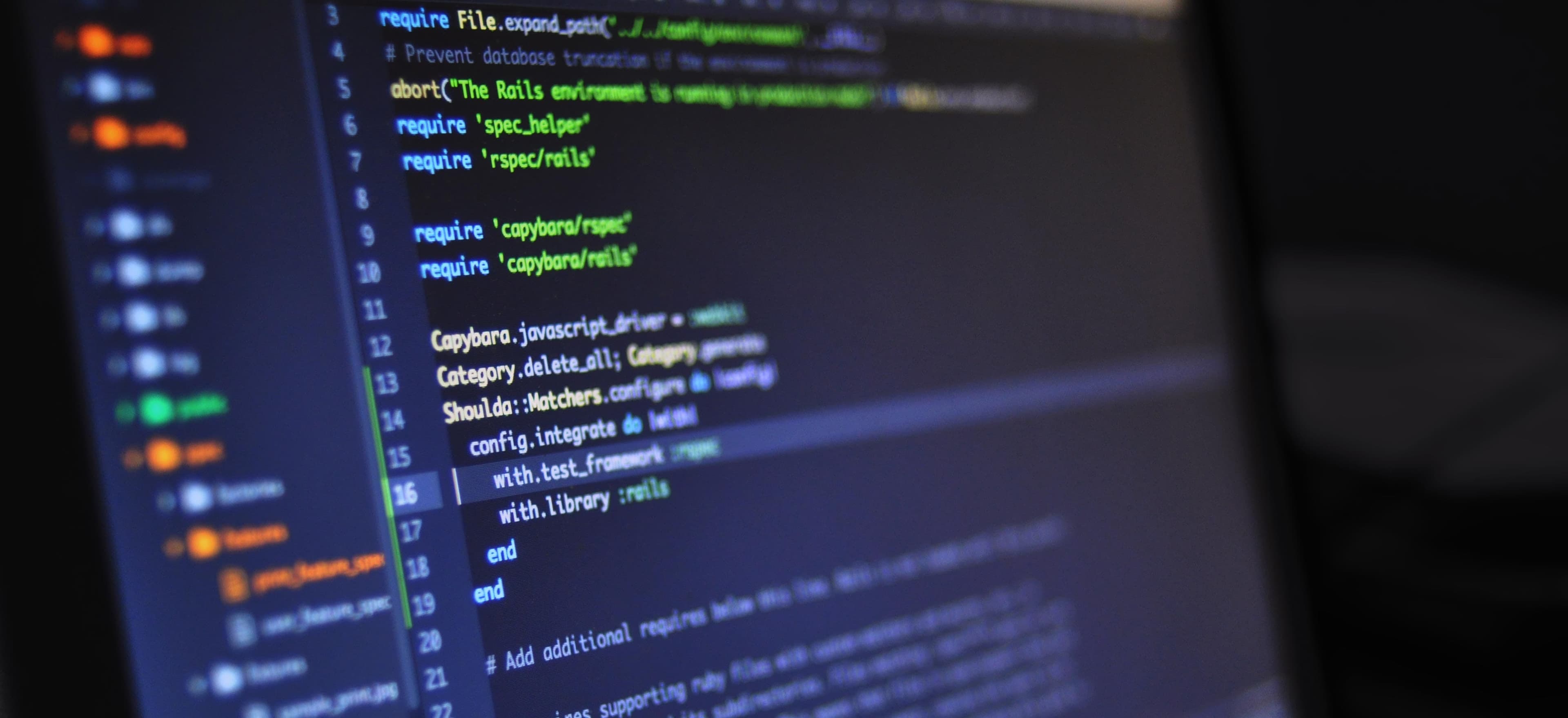
- Published on
Maximizing ServletRequest startAsync Limits for Performance
In the world of Java web application development, optimizing performance is a crucial aspect that every developer should focus on. One of the key features offered by the Java Servlet API is the capability to handle asynchronous requests. This can significantly enhance the performance of your applications, especially under high loads. In this blog post, we will explore the ServletRequest.startAsync()
method, discuss its limits, and share strategies to maximize its benefits.
Understanding Asynchronous Processing in Servlets
Before diving into optimization techniques, it is important to grasp what asynchronous processing means in the context of Servlets. Normally, a servlet responds to client requests in a synchronous manner, meaning that the server cannot process any additional requests until the current one is completed. This can lead to inefficiencies, especially when dealing with long-running tasks.
Asynchronous processing allows a servlet to handle a request in a non-blocking manner. Here’s a brief breakdown of the benefits of using asynchronous processing:
- Improved Resource Utilization: By allowing the server to continue processing other requests while waiting for a long-running task to complete, resources are used more efficiently.
- Enhanced Scalability: Asynchronous processing can scale better under higher load because it frees up threads that would otherwise be blocked.
Invocation of startAsync()
To enable asynchronous processing, you need to call the request.startAsync()
method. This can be done in a servlet as shown below:
@WebServlet(urlPatterns = "/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Start asynchronous processing
AsyncContext asyncContext = request.startAsync();
// Begin processing in a separate thread
asyncContext.start(new Runnable() {
@Override
public void run() {
// Simulate a long-running task
try {
Thread.sleep(5000); // Simulate delay
response.getWriter().println("Async processing complete.");
} catch (InterruptedException | IOException e) {
e.printStackTrace();
} finally {
// Complete the async context
asyncContext.complete();
}
}
});
}
}
Explanation of the Code
- The
@WebServlet
annotation marks the servlet as asynchronous-enabled. - Within the
doGet()
method,startAsync()
is called to switch to async mode. - The
asyncContext.start()
method is used to define the long-running task on a separate thread. - This allows the servlet to finish its work and release the thread to handle other requests immediately.
This simple setup will already improve the responsiveness of the system. However, now let's examine how we can maximize performance by understanding the limits and potential pitfalls.
Maximizing Limits of startAsync()
When dealing with asynchronous processing, it's imperative to manage resources effectively to maintain high performance. Below are some strategies to maximize the benefits of startAsync()
:
1. Thread Pool Management
Utilizing a proper thread pool is critical. If too many threads are spawned, it can lead to resource exhaustion. Consider using the Java Executor framework. Here’s a modified example implementing a thread pool:
@WebServlet(urlPatterns = "/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
private final ExecutorService executorService = Executors.newFixedThreadPool(10);
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
AsyncContext asyncContext = request.startAsync();
executorService.submit(() -> {
try {
Thread.sleep(5000); // Simulate a long-running task
response.getWriter().println("Async processing complete.");
asyncContext.complete();
} catch (InterruptedException | IOException e) {
e.printStackTrace();
}
});
}
@Override
public void destroy() {
executorService.shutdown();
}
}
Why Use a Thread Pool?
Thread pools manage a set of worker threads, optimizing resource allocation and minimizing overhead. Using a pool prevents creating and destroying threads frequently, which is an expensive operation.
2. Timeout Management
Asynchronous processing has its limits. One crucial limit is the timeout. By default, asynchronous contexts have a timeout of 30 seconds. If the processing takes longer than this, an exception is thrown. To handle this, you can configure a timeout:
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
final AsyncContext asyncContext = request.startAsync();
asyncContext.setTimeout(10000); // Set timeout to 10 seconds
executorService.submit(() -> {
try {
// Simulated long processing
Thread.sleep(8000);
response.getWriter().println("Async processing complete.");
asyncContext.complete();
} catch (InterruptedException | IOException e) {
e.printStackTrace();
}
});
}
3. Error Handling
Error handling in asynchronous tasks can be tricky, as exceptions don't propagate back to the servlet directly. Use the AsyncListener
to manage errors:
asyncContext.addListener(new AsyncListener() {
@Override
public void onComplete(AsyncEvent asyncEvent) throws IOException {
// Handle completion
}
@Override
public void onTimeout(AsyncEvent asyncEvent) throws IOException {
response.getWriter().println("Timeout occurred.");
asyncContext.complete();
}
@Override
public void onError(AsyncEvent asyncEvent) throws IOException {
response.getWriter().println("Error occurred: " + asyncEvent.getThrowable().getMessage());
asyncContext.complete();
}
@Override
public void onStartAsync(AsyncEvent asyncEvent) throws IOException {
// Handle start
}
});
4. Keep Request Processing Short
Although startAsync()
allows for long-running tasks, it’s wise to keep the actual processing of requests as minimal as possible. Consider offloading heavy processing to a background service or task:
- Use message queues (like RabbitMQ or Apache Kafka) to process larger tasks asynchronously.
- Invoke services that handle resource-intensive operations (like databases or APIs) within these systems to decouple the request/response cycle.
Closing the Chapter
Implementing ServletRequest.startAsync()
within your Java web applications can significantly enhance performance when correctly managed. By utilizing thread pools, managing timeouts, handling errors properly, and keeping request processing short, you will maximize the benefits of asynchronous processing.
You can learn more about the asynchronous processing model in the Java EE Documentation for further understanding.
Call to Action
Start implementing these strategies in your current projects and see how they improve your application's responsiveness and overall performance! Don't forget to share your experiences and any additional techniques you've found useful in the comments below. Happy coding!
Checkout our other articles