Resolving Common Issues with Spring Security Basic Auth in XML
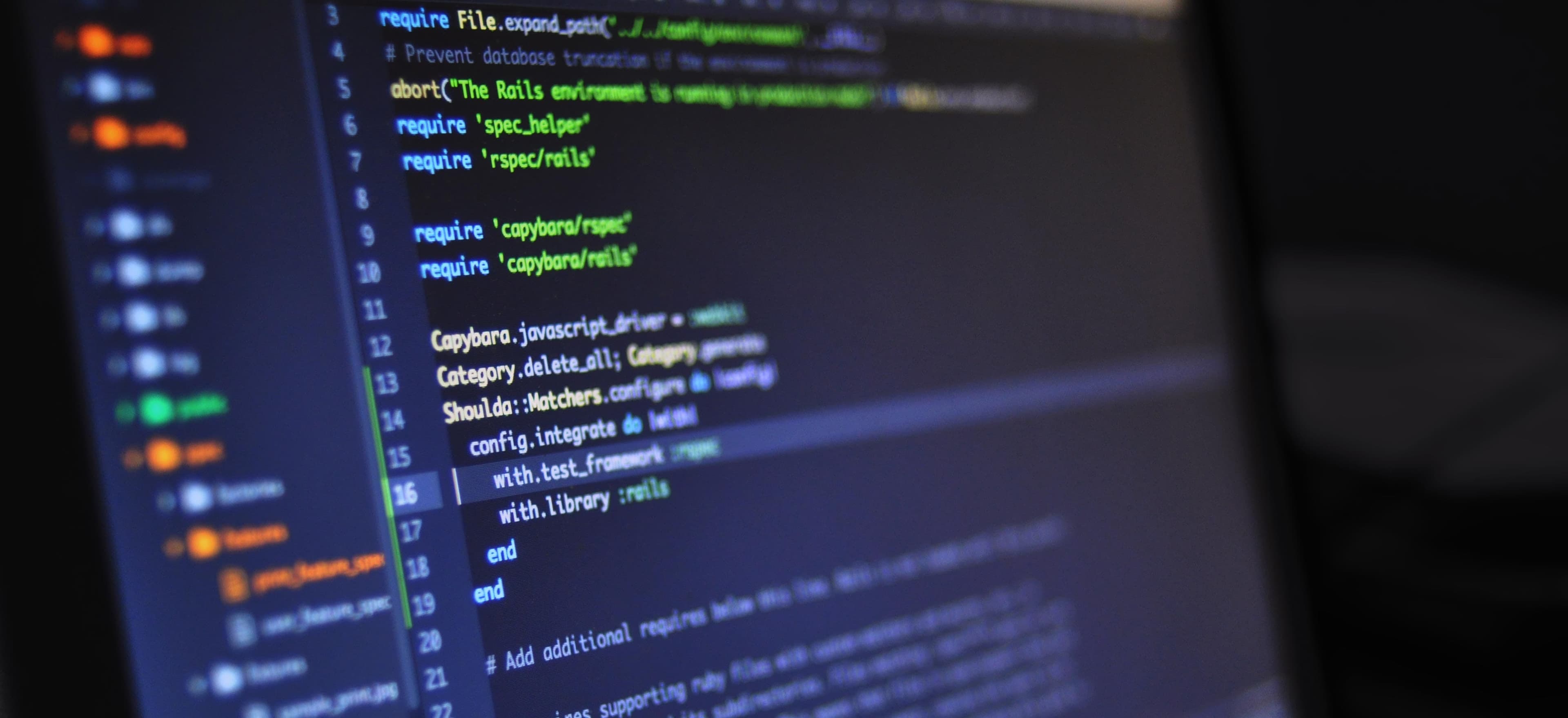
- Published on
Resolving Common Issues with Spring Security Basic Auth in XML
Spring Security is a powerful framework that provides comprehensive security services for Java applications. In the realm of web applications, Basic Authentication is a simple yet effective means of securing your endpoints. However, when configured using XML, it can sometimes lead to some common issues. This blog post aims to delve into these issues and provide you with viable solutions.
Understanding Basic Authentication
Before we dive into resolving issues, let's clarify what Basic Authentication entails. In Basic Authentication, the user's credentials (username and password) are sent in HTTP headers to authenticate a user.
This method is quite secure when combined with HTTPS as it protects the data transferred between client and server. However, proper implementation is crucial to avoid common pitfalls.
Setting Up Spring Security XML Configuration
To employ Basic Authentication using Spring Security, we first need to configure our XML file. Below is an example of a basic configuration:
<beans:beans xmlns="http://www.springframework.org/schema/security"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/security
http://www.springframework.org/schema/security/spring-security.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<http auto-config="true" use-expressions="false">
<intercept-url pattern="/admin/**" access="hasRole('ROLE_ADMIN')" />
<intercept-url pattern="/user/**" access="hasRole('ROLE_USER')" />
<form-login login-page="/login" />
<logout logout-url="/logout" />
<http-basic />
</http>
<authentication-manager>
<authentication-provider>
<password-encoder ref="passwordEncoder" />
<user-service>
<user name="user" password="{noop}password" authorities="ROLE_USER"/>
<user name="admin" password="{noop}admin" authorities="ROLE_ADMIN"/>
</user-service>
</authentication-provider>
</authentication-manager>
<beans:bean id="passwordEncoder" class="org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder"/>
</beans:beans>
In the above configuration:
- We define secured URLs.
- We specify a
<http-basic />
element to enable Basic Authentication. - We create an in-memory user store with usernames and roles.
Common Issues and Their Solutions
1. 401 Unauthorized Error
The most common issue you might encounter is receiving a 401 Unauthorized error. This indicates that authentication has failed.
Solution:
- Ensure that the server is running and the app is accessible.
- Make sure you are sending the correct credentials.
- Check that the specified roles match those defined in the XML configuration.
Here's how to validate the username and password:
// Example of a HTTP client request in Java
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.client.BasicCredentialsProvider;
CloseableHttpClient client = HttpClients.custom()
.setDefaultCredentialsProvider(provider)
.build();
HttpGet request = new HttpGet("http://localhost:8080/user");
HttpResponse response = client.execute(request);
In the above code, ensure that the credentials sent in the request match the configuration.
2. Credentials in Clear Text
Basic Authentication sends credentials encoded in Base64. These are not encrypted, making it vulnerable in transmission. You may note that even if you're connected to HTTPS, a misconfiguration can render it ineffective.
Solution:
- Always ensure your application is served over HTTPS.
Here is how you ensure HTTPS is enabled in your Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.embedded.tomcat.ConfigurableTomcatWebServerFactory;
import org.springframework.boot.web.embedded.tomcat.TomcatServletWebServerFactory;
import org.springframework.boot.web.server.LocalServerPort;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class MySpringSecurityApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringSecurityApplication.class, args);
}
@Bean
public TomcatServletWebServerFactory servletContainer() {
TomcatServletWebServerFactory tomcat = new TomcatServletWebServerFactory();
tomcat.addConnectorCustomizers(
connector -> connector.setScheme("https"),
connector -> connector.setSecure(true)
);
return tomcat;
}
}
By ensuring your server is configured to use HTTPS, you can safeguard your Basic Authentication from exposure.
3. The Application Not Prompting for Credentials
In some cases, the browser or application may not prompt for credentials. This could be due to incorrect configuration.
Solution:
- Verify that you have not customized the
login-page
URL in your XML configuration under the<form-login>
element. In Basic Authentication, the user should be prompted automatically by the browser.
4. CORS Issues with AJAX Requests
If you are integrating with front-end frameworks (like React or Angular) and are facing CORS issues, this is common with Basic Auth.
Solution:
- Enable CORS in your Spring configuration. Below is an example of how you could do it:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*") // Change this in production
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS")
.allowedHeaders("*")
.allowCredentials(true);
}
}
This setup allows your front-end to communicate effectively with the backend, mitigating potential CORS-related problems.
5. Error Handling for Authentication Failures
While developing, you might want to manage errors gracefully.
Solution:
- Implement an error handler in your application to customize the response when authentication fails.
import org.springframework.security.web.AuthenticationEntryPoint;
import org.springframework.stereotype.Component;
import org.springframework.web.HttpRequestMethodNotSupportedException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@Component
public class CustomAuthenticationEntryPoint implements AuthenticationEntryPoint {
@Override
public void commence(HttpServletRequest request, HttpServletResponse response, AuthenticationException authException) throws IOException {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authentication Failed: " + authException.getMessage());
}
}
You can then register this Entry Point in your Spring Security configuration to provide a more user-friendly error response.
Lessons Learned
Setting up Basic Authentication with Spring Security using XML can be straightforward, though it may present several challenges. Understanding these common issues and knowing how to resolve them is paramount in ensuring a seamless user experience.
For further reading, you can explore Spring Security Documentation for extensive details on authentication flows or check out Baeldung's Guide for practical insights on security authentication patterns.
Remember, while Basic Authentication provides a lightweight way to secure your APIs, always accompany it with HTTPS, proper role management, and error handling strategies to protect your applications effectively.
Checkout our other articles