Mastering Data Aggregation in Spring Data MongoDB
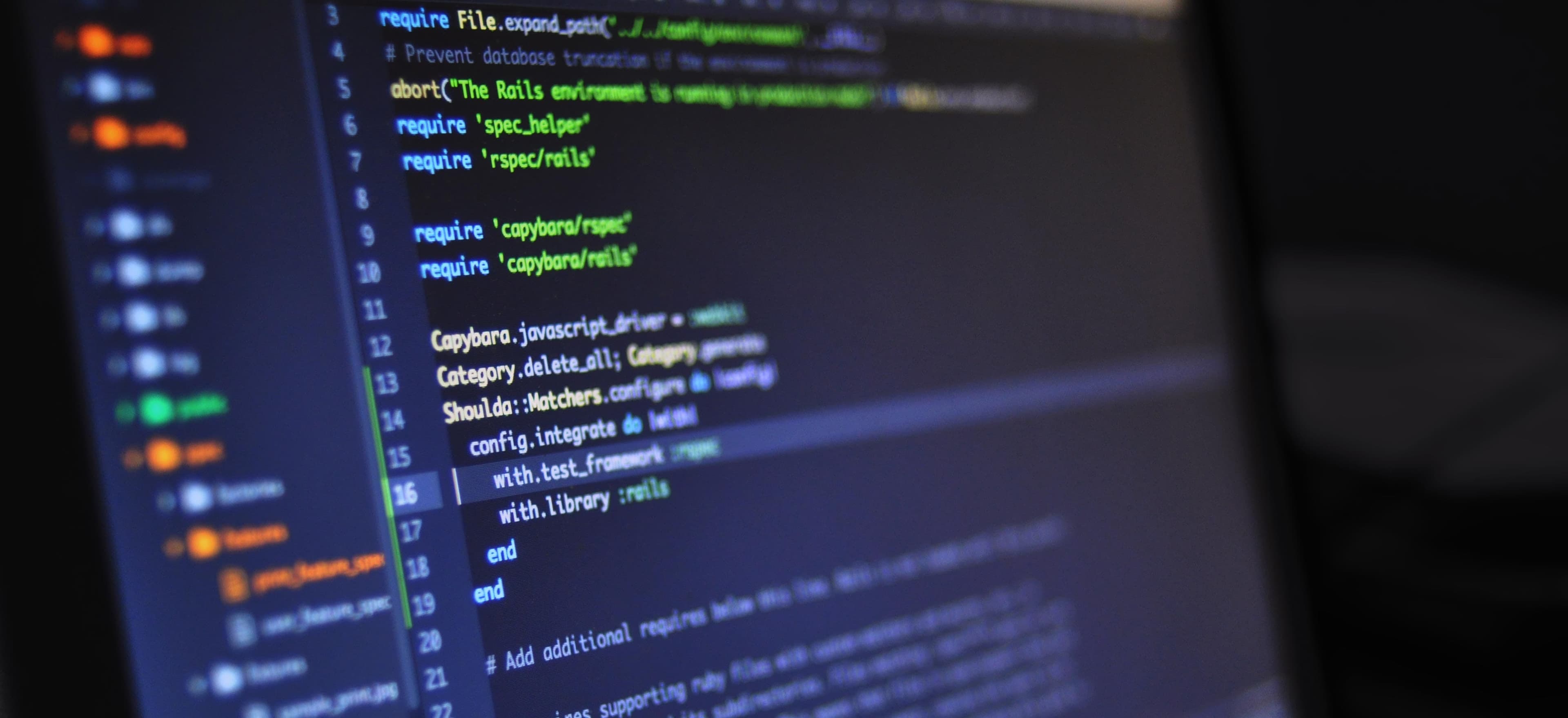
- Published on
Mastering Data Aggregation in Spring Data MongoDB
In today's data-driven world, the ability to efficiently process and analyze large datasets is a must. With the rise of NoSQL databases, MongoDB has gained significant traction for its flexible schema and high performance. In this blog post, we will explore data aggregation using Spring Data MongoDB, a powerful framework that simplifies interactions with MongoDB.
Table of Contents
- Understanding Data Aggregation
- Why Use Spring Data MongoDB?
- Setting Up Your Spring Project
- Basic Aggregation Operations
- Advanced Aggregation Pipeline
- Real-World Example
- Conclusion
Understanding Data Aggregation
Data aggregation is the process of collecting and summarizing data from multiple sources to provide meaningful insights. MongoDB offers a rich set of aggregation frameworks that allow users to transform and combine data in various ways. This can include tasks such as filtering, grouping, and reshaping data.
The core of MongoDB's aggregation framework is the aggregation pipeline. Similar to Unix pipelines, an aggregation pipeline is a series of processing stages where each stage transforms the data. This flexibility allows for complex data manipulations with relative ease.
Why Use Spring Data MongoDB?
Spring Data MongoDB provides several benefits:
- High-Level Abstraction: It offers a simplified interface to work with MongoDB, abstracting away the low-level Java MongoDB driver code.
- Spring Integration: It seamlessly integrates with the Spring ecosystem, allowing for easier configuration and management of database connections.
- Repository Support: Spring Data’s repository support simplifies CRUD operations and enables the creation of custom queries using derived methods.
Setting Up Your Spring Project
1. Maven Dependency
To get started, ensure you have your Spring project set up with Maven. You can include the necessary Spring Data MongoDB dependencies in your pom.xml
.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2. MongoDB Configuration
Configure your application to connect to MongoDB by adding the following properties in application.properties
.
spring.data.mongodb.uri=mongodb://localhost:27017/yourDatabase
Replace yourDatabase
with the name of your MongoDB database.
3. Create Your Domain Model
Define a simple domain model to illustrate our aggregation. For example, let’s say we have a Product
class.
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "products")
public class Product {
@Id
private String id;
private String name;
private int quantity;
private double price;
// Getters and Setters
}
Basic Aggregation Operations
Now that we have our Product
model set, let’s look at some basic aggregation operations. To perform aggregation, we often use the MongoTemplate
class.
Example: Count Products
A common use-case is to count the number of products:
@Autowired
private MongoTemplate mongoTemplate;
public long countProducts() {
return mongoTemplate.count(new Query(), Product.class);
}
Example: Group By Price Range
More complex aggregations can be done using the aggregation framework.
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.AggregationResults;
public Map<String, Long> groupByPriceRange() {
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group("price")
.count().as("totalCount"));
AggregationResults<Map> results = mongoTemplate.aggregate(aggregation, Product.class, Map.class);
return results.getMappedResults();
}
Here, we group products by their price using the group
operation. The count
method counts how many products fall into each price category.
Advanced Aggregation Pipeline
Now, let’s delve deeper into the aggregation pipeline. MongoDB provides various stages like $match
, $group
, $sort
, and $project
that can be combined to perform complex queries.
Example: Find Average Price by Quantity
Suppose you want to find the average price of products grouped by their quantity. Here’s how you can do it:
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.AggregationResults;
public Map<Integer, Double> averagePriceByQuantity() {
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.group("quantity").avg("price").as("averagePrice")
);
AggregationResults<Map> results = mongoTemplate.aggregate(aggregation, Product.class, Map.class);
return results.getMappedResults();
}
Explanation of Each Stage
$group
: This stage groups documents by the specified field, in this case,quantity
. It calculates the average price using theavg
operator.- Output: The output will be a map where keys are quantities and values are average prices.
Real-World Example
Let's consider a situation where you need to generate a report of product sales. We'll create a composite aggregation that counts the number of products over a specified price.
public List<Map> reportHighValueProducts(double thresholdPrice) {
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.match(Criteria.where("price").gte(thresholdPrice)),
Aggregation.group("name").count().as("totalSales"),
Aggregation.sort(Sort.by(Sort.Order.desc("totalSales")))
);
AggregationResults<Map> results = mongoTemplate.aggregate(aggregation, Product.class, Map.class);
return results.getMappedResults();
}
Explanation
$match
: Filters products that are above the given price threshold.$group
: Groups the remaining products by their name and counts them astotalSales
.$sort
: The list is sorted by total sales in descending order.
This allows you to see which products are selling well above your defined price threshold.
Wrapping Up
Mastering data aggregation in Spring Data MongoDB can empower developers to derive insightful analytics from their datasets. The combination of MongoDB's aggregation capabilities with Spring Data's intuitive interface provides a powerful toolset for data manipulation.
Start integrating these concepts into your projects and see the value they bring to your application. If you're interested in diving deeper into Spring Data MongoDB, consider visiting the official documentation here. Also, don't forget to explore aggregation frameworks directly through MongoDB’s aggregation documentation.
By honing your skills in data aggregation, you'll be better equipped to build robust applications that provide meaningful insights from your data. Happy coding!
Checkout our other articles