Managing Shared Libraries for ADF Apps in WebLogic
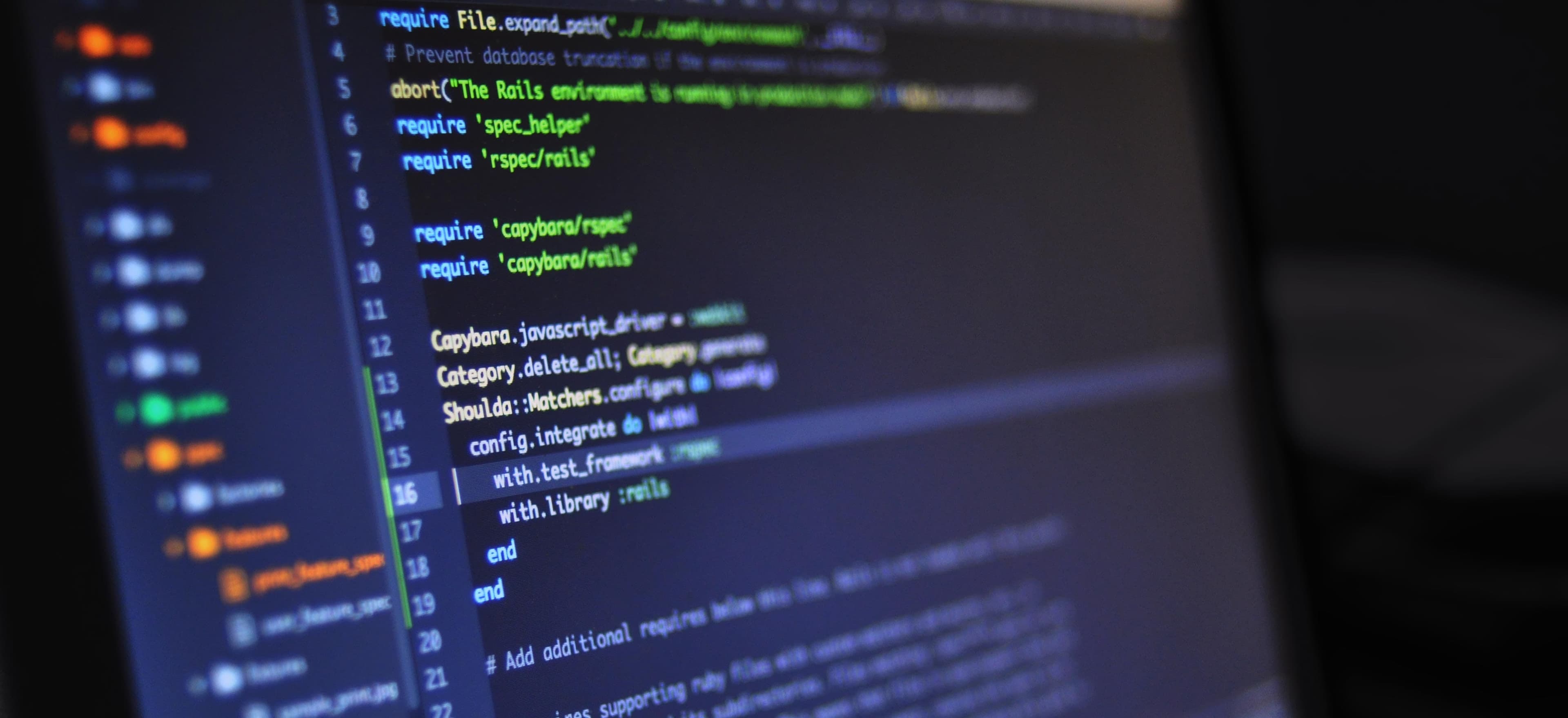
- Published on
Managing Shared Libraries for ADF Apps in WebLogic
Oracle ADF (Application Development Framework) is a powerful framework for building enterprise applications. Leveraging ADF in conjunction with WebLogic Server offers the capability of using shared libraries, optimizing application development, deployment, and maintenance. This blog post aims to provide a comprehensive overview of managing shared libraries for ADF applications in WebLogic.
What are Shared Libraries?
Shared libraries in the context of Java EE applications are typically reusable Java components packaged as JAR files. These libraries enable developers to centralize common functionalities, such as utility functions or specific components, thereby facilitating code reuse and consistency across applications.
Why Use Shared Libraries?
-
Reusability: Code developed once can be reused across multiple applications, leading to reduced duplication of effort.
-
Maintainability: If a bug is found in a shared library, it can be fixed in one location rather than in each application that uses it.
-
Version Control: Shared libraries allow for deploying the same version of a library across applications, leading to fewer version conflicts.
-
Performance Improvement: Shared libraries can reduce the overall size of individual ADF applications since common code will not be packaged into each deployment.
Pre-requisites
Before we dive deeper into managing shared libraries, ensure you have the following:
- A working knowledge of WebLogic Server.
- ADF installed and configured.
- Access to Oracle's documentation for further reference.
- A sample ADF application that can leverage shared libraries.
Creating a Shared Library
Creating a shared library involves several steps. This typically starts with developing a Java utility or application that you want to package and share.
Step 1: Develop Your Java Class
Create your Java class. The example below demonstrates a utility that provides a method to format dates.
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateUtil {
public static String formatDate(Date date) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
return formatter.format(date);
}
}
Why This Code?
The DateUtil
class is straightforward yet reusable. By extracting the date formatting function, we prevent duplicate code in our applications.
Step 2: Package as a JAR
Once your utility class is developed, package it as a JAR file using your IDE (like Eclipse or IntelliJ) or via the command line.
Using Command Line:
javac DateUtil.java
jar cvf DateUtil.jar DateUtil.class
Step 3: Upload JAR to WebLogic Library
-
Log in to the WebLogic Server Administration Console.
-
Navigate to
Deployments
. -
Click on
Install
and upload your JAR file. -
Select the
Library
option when deploying. -
Specify a
name
andtarget
(Choose appropriate servers or clusters). -
Click
Activate Changes
.
Step 4: Configure the ADF Application to Use the Shared Library
In order for your ADF application to utilize the shared library, you need to associate it.
-
Open your ADF application in JDeveloper.
-
Navigate to
Project Properties
. -
Select
Libraries and Classpath
. -
Click on
Add Shared Library
and select the deployed library. -
Build and deploy your application on WebLogic.
Example Usage in ADF
Now that you've deployed your shared library, let's see how we can use the DateUtil
class in a managed bean within an ADF application.
import java.util.Date;
import javax.faces.bean.ManagedBean;
@ManagedBean
public class SampleBean {
private String currentDate;
public SampleBean() {
Date now = new Date();
currentDate = DateUtil.formatDate(now); // Using the shared library
}
public String getCurrentDate() {
return currentDate;
}
}
Why Using Shared Libraries Matters Here:
By using the DateUtil
class within SampleBean
, we maintain clean separation of concerns. This not only enhances readability but also makes modifying the date format simpler without touching the business logic.
Version Management of Shared Libraries
Managing shared libraries over time becomes crucial. Here’s how you can handle versioning effectively.
-
Naming Conventions: Use version numbers in the JAR filenames, like
DateUtil-v1.0.jar
. This helps in identifying which version is deployed. -
Version Tracking: Maintain a changelog to track modifications, bug fixes, and version bumps.
-
Update Deployment: Re-deploy updated libraries in the WebLogic console. Ensure applications are synchronized with the latest library version by updating dependencies and re-deploying.
Handling Compatibility Issues
As existing shared libraries evolve, compatibility issues might arise. Here’s what you can do:
-
Testing: Always implement automated tests to check for potential issues when a shared library is updated.
-
Fallback Versions: While deploying new versions, it might be wise to have old versions available in case rollback is needed.
-
Backward-Compatible Methods: Avoid breaking existing functionality by providing backward-compatible methods when introducing new functionalities.
Troubleshooting Common Issues
Here are some common issues faced when working with shared libraries and how to resolve them.
-
ClassNotFoundException: This often indicates that the shared library isn’t properly deployed or there are classpath issues. Confirm that the library is active and correctly referenced in your ADF application.
-
Version Conflicts: If two libraries contain the same classes or packages, you may run into conflicts. Use unique packages and namespaces when designing libraries.
-
Deployment Failures: If deployment fails, always check the
logs
in WebLogic. They often provide insights into what went wrong and help troubleshoot effectively.
The Closing Argument
Managing shared libraries for ADF applications in WebLogic is a robust technique that facilitates code reuse, enhances maintainability, and streamlines application development. By following the steps outlined above, you can effectively create, deploy, and manage shared libraries, ensuring that your ADF applications are efficient and scalable.
Further reading on WebLogic Server and ADF Framework can provide additional context to deepen your understanding of these powerful tools.
Feel free to share your experiences and challenges in using shared libraries within ADF applications. Happy Coding!