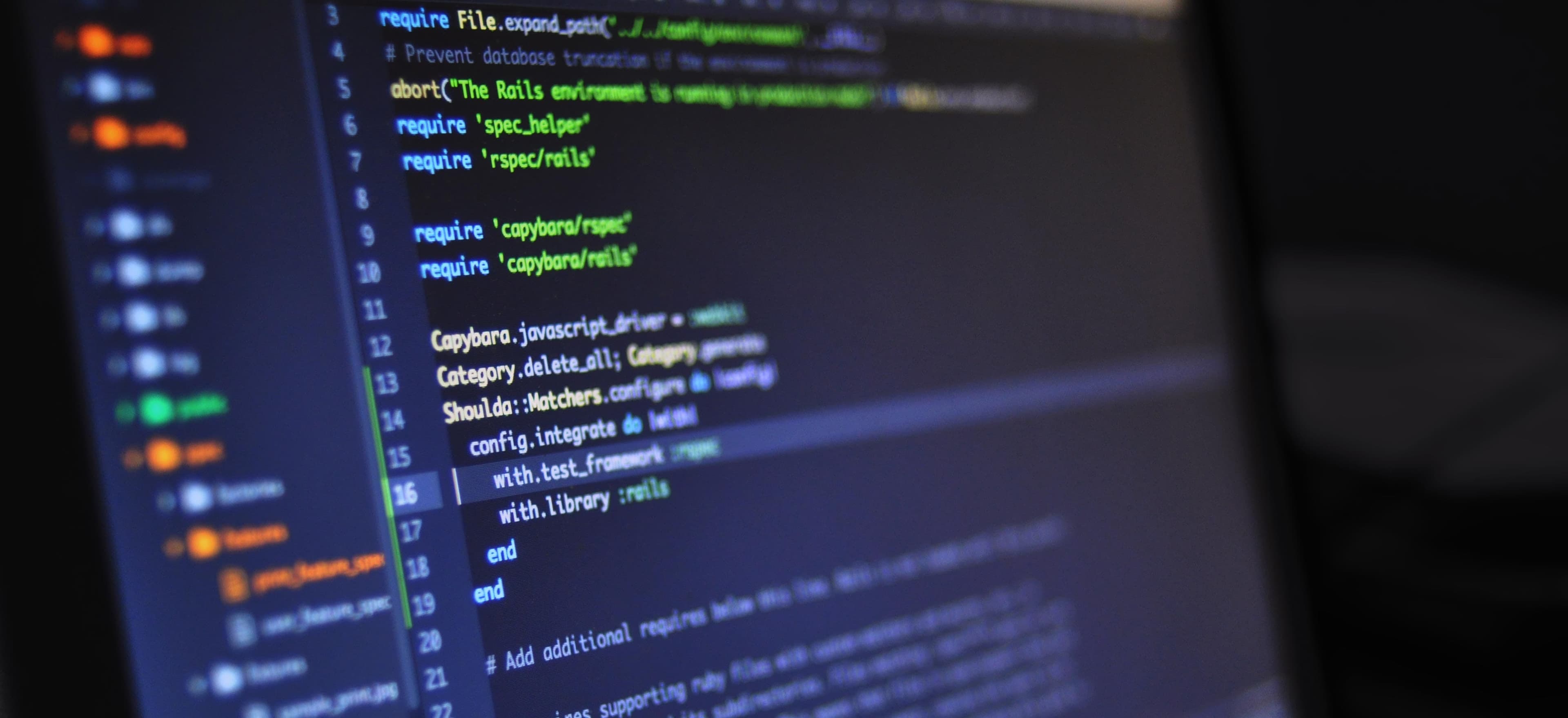
- Published on
Common Pitfalls in Using iOSched for Android UI Development
When it comes to developing robust Android user interfaces, iOSched (an open-source application for managing conference schedules) provides an interesting case study. Despite being crafted primarily for iOS, it offers valuable insights for Android developers looking to build rich UIs. However, it’s essential to be aware of common pitfalls associated with using iOSched as a reference for Android UI development. In this blog post, we will explore these pitfalls while providing solid advice, insightful code snippets, and best practices to help you enhance your Android UI skills.
Understanding iOSched
iOSched is a widely recognized mobile application that helps users keep track of events and schedules in a concise manner. It leverages various UI components, animations, and layouts that might seem appealing to Android developers. You can explore its GitHub repository to see the code in action.
However, while drawing inspiration from iOSched, developers must adapt its concepts to align with the Android design philosophy. Let's dive into some common pitfalls and best practices.
Pitfall 1: Ignoring Platform-Specific Guidelines
Why It Matters
Each mobile platform has its unique design guidelines. iOS leads developers towards a clean, minimalist aesthetic whereas Android is all about versatility and flexibility.
Solution: Follow Material Design Guidelines
As an Android developer, you should adhere to Material Design principles. This ensures that your app feels native to Android users while providing an intuitive experience.
<!-- Example of Material design in Android -->
<Button
android:id="@+id/my_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Press Me"
app:backgroundTint="@color/colorAccent"
android:elevation="8dp" />
Here, we have a button with a background tint and elevation, both of which are crucial for achieving depth in Material Design. This is something that iOSched may miss if directly interpreted for Android.
Pitfall 2: Overcomplicating Navigation
Why It Matters
iOS applications often use a more linear flow in UI navigation. However, Android applications commonly utilize a drawer navigation or tabbed interfaces that can provide a more versatile experience.
Solution: Implement a Navigation Drawer
For Android applications, consider using a navigation drawer—a common and versatile solution.
// Setting up the Navigation Drawer
val drawerLayout = findViewById<DrawerLayout>(R.id.drawer_layout)
val toggle = ActionBarDrawerToggle(this, drawerLayout, R.string.navigation_drawer_open, R.string.navigation_drawer_close)
drawerLayout.addDrawerListener(toggle)
toggle.syncState()
In the above code snippet, we set up a navigation drawer that allows users to access various sections of the application with ease. This enhances user experience and keeps the interface organized.
Pitfall 3: Misusing Layouts
Why It Matters
iOS layouts often rely on fixed sizes and rigid structural hierarchies. Android, however, provides dynamic layouts that can adapt to different screen sizes and orientations.
Solution: Utilize ConstraintLayout
To create flexible and responsive layouts, use ConstraintLayout
. It allows you to create complex layouts with a flat view hierarchy.
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/title"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:text="Hello, Android!" />
<Button
android:id="@+id/start_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@id/title"
app:layout_constraintStart_toStartOf="parent"
android:text="Get Started" />
</androidx.constraintlayout.widget.ConstraintLayout>
In this example, we use ConstraintLayout
to pull elements together based on their relationships rather than relying on hard-coded positions, which is a common mistake drawn from an iOS perspective.
Pitfall 4: Overreliance on Animations
Why It Matters
iOS heavily employs animations, often leading to a smoother user experience. However, overusing animations on Android can hinder performance and distract users. Balancing functionality and flair is a delicate act.
Solution: Use Animation Sparingly
While animations can enhance your UI, ensure they do not detract from usability or degrade performance.
// Using a scale animation on a button
val scaleAnimation = ObjectAnimator.ofFloat(myButton, "scaleX", 0.5f, 1f)
scaleAnimation.duration = 300
scaleAnimation.interpolator = AccelerateDecelerateInterpolator()
scaleAnimation.start()
In the code snippet above, we create a simple scale animation. Keep in mind to use animations to highlight actions (like button presses) rather than overusing them throughout your app.
Pitfall 5: Inadequate Testing Across Devices
Why It Matters
Developers who create for iOS often assume uniformity in device sizes. Android developers must be aware of the multiple screen sizes and resolutions that exist.
Solution: Use Responsive Testing Tools
Utilize tools like Android's Device Emulator or platforms like Firebase Test Lab, which allow you to test your app across different devices and resolutions.
# Example of running tests across various devices
./gradlew connectedAndroidTest -Pdevice=Pixel_4_API_30
Testing is crucial. Identify issues related to layout, performance, and usability before your app goes live.
Lessons Learned
Developing on Android using insights drawn from iOSched can be a double-edged sword. While it offers a wealth of inspiration, it is imperative to acknowledge and negotiate the platform-specific challenges.
Staying aligned with Material Design, utilizing Android’s navigation paradigms, embracing responsive layouts, balancing animations, and rigorously testing cross-device performance will pave the way toward creating a successful Android UI.
In summary, let iOSched serve you as a learning tool rather than a blueprint. Create unique experiences that resonate with Android users while learning from the successes of other platforms. Happy coding!
For further reading on best practices in Android development, check out the official Android developer documentation.
Checkout our other articles