Troubleshooting Common Issues in Spring Boot Pimp Config
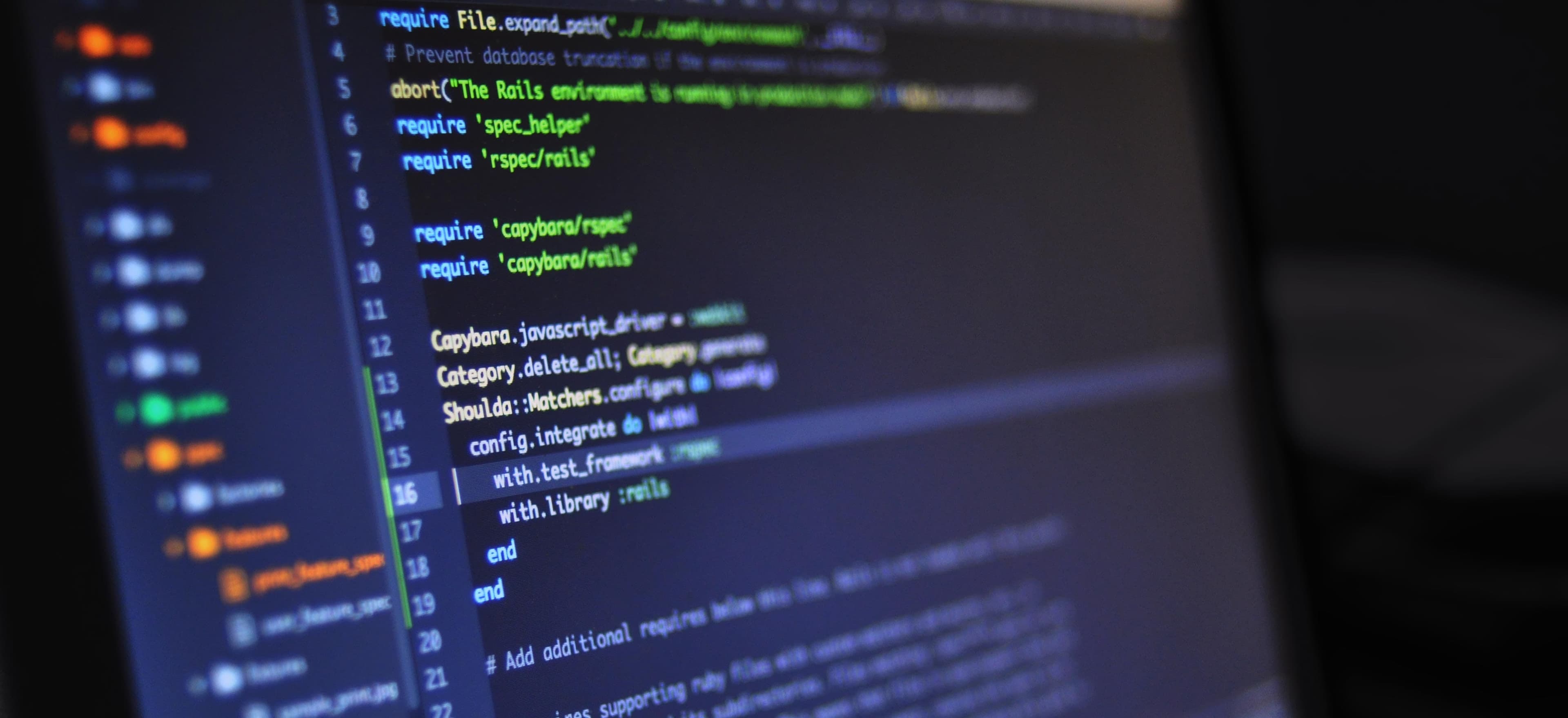
- Published on
Troubleshooting Common Issues in Spring Boot Pimp Config
Spring Boot has revolutionized the way developers build applications by simplifying the setup and configuration process. However, even with its ease of use, developers may encounter issues during configuration, especially when using Pimp Config, a configuration management library for Spring Boot. In this blog post, we will focus on troubleshooting common issues that arise while using Spring Boot Pimp Config and provide practical solutions with code snippets to help you navigate these challenges.
Table of Contents
- Introduction to Pimp Config
- Common Issues in Spring Boot Pimp Config
- Issue 1: Missing Configuration Values
- Issue 2: Incorrect Property Binding
- Issue 3: Connection Issues
- Issue 4: Configuration Profiles Not Applied
- Best Practices for Using Pimp Config
- Conclusion
Starting Off to Pimp Config
Pimp Config is a powerful library that allows developers to manage external configurations in a flexible manner. By integrating Pimp Config with Spring Boot, one can:
- Fetch configurations from various sources (e.g., Git, HTTP, etc.)
- Manage property substitutions seamlessly
- Update configurations at runtime
While Pimp Config provides extensive functionality, issues can arise, particularly in larger applications. Let's explore some common pitfalls and the best practices for overcoming them.
Common Issues in Spring Boot Pimp Config
Issue 1: Missing Configuration Values
One of the most frequent issues encountered is missing configuration values. This typically occurs when the application expects certain properties to be present, but they are not defined in the configuration sources.
Solution
To troubleshoot missing configuration values, you can implement the following approaches:
-
Check Configuration Sources: Ensure that your configuration files or external sources contain the necessary properties.
-
Use Default Values: If a configuration value might be missing, consider defining a default value.
@ConfigurationProperties(prefix = "myapp")
public class MyAppProperties {
private String name = "Default App Name"; // Default value
// Getters and setters
}
In the above code snippet, if myapp.name
is not defined in the configuration source, "Default App Name" will be used instead. This prevents your application from failing due to missing configurations.
Issue 2: Incorrect Property Binding
Another common issue is when the property names in your configuration do not match the structure of your Java class. This can lead to properties not being bound correctly.
Solution
Ensure that the property names in your configuration match the naming conventions of your Java class. Use the @ConfigurationProperties
annotation to bind properties to your class.
@PropertiesPrefix(value = "database")
public class DatabaseProperties {
private String url;
private String username;
private String password;
// Getters and setters
}
For the above code to work, your configuration file (e.g., application.yml
) should look like this:
database:
url: jdbc:mysql://localhost:3306/mydb
username: user
password: password
The naming must be consistent; otherwise, Spring fails to bind these properties correctly. Always refer to the Spring Boot documentation here for a comprehensive understanding of property binding.
Issue 3: Connection Issues
Pimp Config often fetches configurations from remote sources such as Git repositories or configuration servers. Network-related issues can arise, leading to connection problems.
Solution
To address connection issues, follow these debugging steps:
-
Check Network Connectivity: Ensure your application can reach the configured URLs.
-
Configuration Server Details: Verify that your configuration server is up and running with the correct endpoints.
-
Use Retry Logic: Implement retry logic for transient connection failures.
@Configuration
@EnableAutoConfiguration
public class ConfigRetry {
@Bean
public RetryTemplate retryTemplate() {
RetryTemplate retryTemplate = new RetryTemplate();
FixedBackOffPolicy backOffPolicy = new FixedBackOffPolicy();
backOffPolicy.setBackOffPeriod(5000); // 5 seconds
retryTemplate.setBackOffPolicy(backOffPolicy);
return retryTemplate;
}
}
In this example, if a connection fails, the application will automatically retry after 5 seconds. Implementing a robust retry strategy can significantly improve the resilience of your application.
Issue 4: Configuration Profiles Not Applied
When using different profiles in Spring Boot (like dev, test, prod), it's essential that the correct profile is activated, or expected configurations may not be loaded.
Solution
Ensure that you activate the correct profile in your application.properties or use command-line arguments to switch profiles.
spring.profiles.active=dev
Alternatively, you can specify the active profile when starting your application via the command line:
java -jar myapp.jar --spring.profiles.active=prod
Make sure that you have corresponding configuration files for each profile. For example:
- application-dev.yml
- application-prod.yml
This separation allows for different configurations, tailored to the specific needs of each environment. Learn more about profiles here for a deeper understanding.
Best Practices for Using Pimp Config
-
Documentation: Always refer to the official Pimp Config documentation. It is regularly updated and provides useful information.
-
Environment-Specific Files: Keep separate configuration files for different environments to avoid accidental overriding of settings.
-
Testing: Implement unit tests for your configuration classes to ensure properties are bound correctly.
-
Secure Sensitive Information: Use tools like Spring Cloud Config to manage sensitive configurations more securely.
-
Version Control: Keep your external configuration files under version control to track changes and maintain history.
In Conclusion, Here is What Matters
Spring Boot with Pimp Config offers extensive flexibility for managing application configurations. By understanding common issues and implementing the solutions provided in this blog post, you can enhance your debugging skills and ensure that your Spring Boot applications run smoothly. Always keep your configurations organized and adhere to best practices to create robust applications.
If you have any specific issues or have encountered different problems while using Pimp Config, feel free to share in the comments below! Happy coding!