The Challenge of Managing API Complexity in Composition Patterns
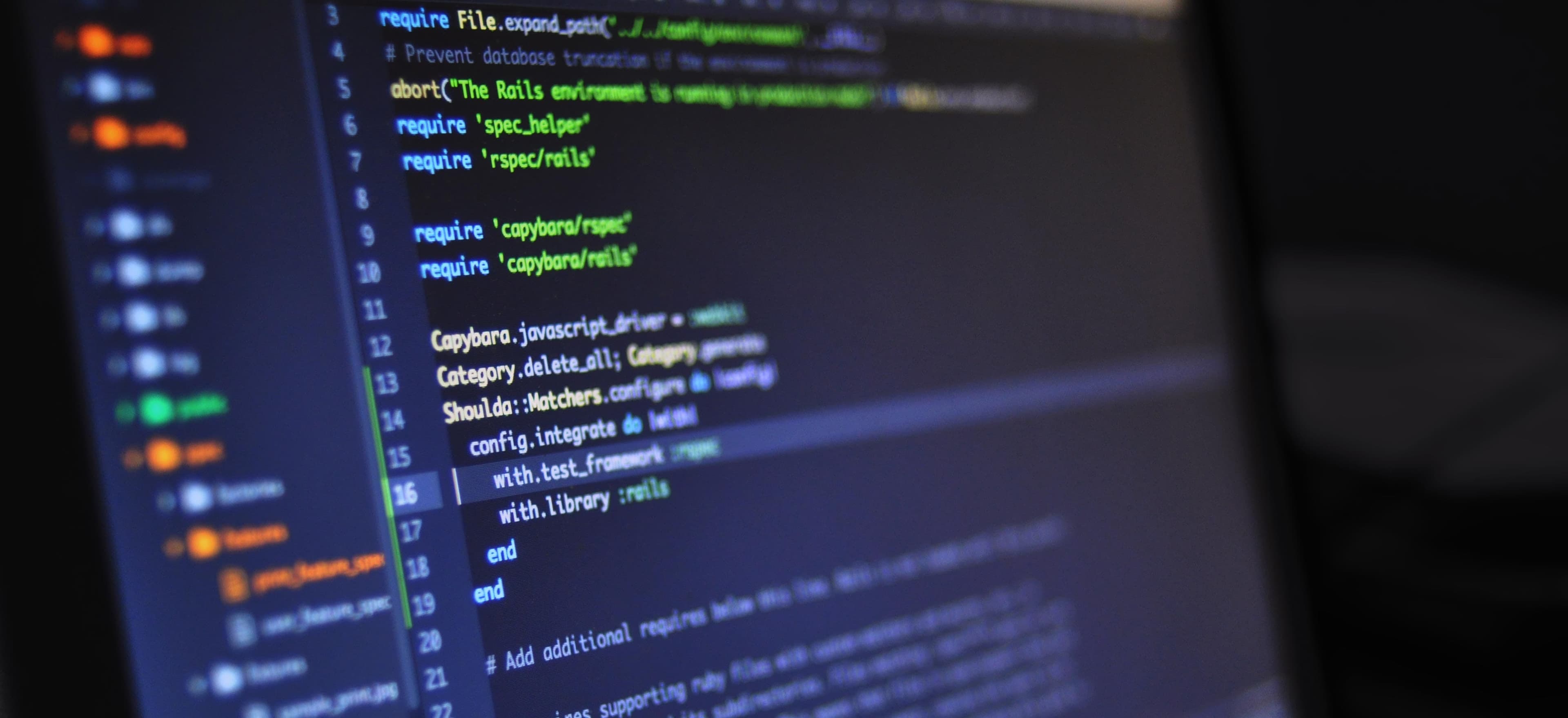
- Published on
The Challenge of Managing API Complexity in Composition Patterns
In today's software development landscape, APIs (Application Programming Interfaces) play a crucial role in enabling different systems to communicate effectively. However, as applications scale and the number of APIs grows, managing this complexity becomes a significant challenge. One way to tackle this complexity is through composition patterns — techniques that allow developers to combine multiple services or components to form a cohesive application. In this blog post, we'll explore the challenges of managing API complexity, delve into different composition patterns, and provide you with practical Java examples.
Understanding API Complexity
APIs are everywhere. From third-party services handling authentication to microservices communicating internally, the number of APIs that a single application interacts with can quickly surpass a manageable number. This multitude creates various challenges, including:
- Increased Latency: Each call to an external API introduces latency. As the number of calls increases, response times can suffer.
- Error Handling: With many APIs in play, the potential for failure rises. Handling these failures requires a robust strategy.
- Versioning: APIs are frequently updated, and managing different versions can lead to integration challenges.
- Documentation: Maintaining up-to-date documentation across various services can become cumbersome.
The challenge lies not just in connecting to these APIs but in doing so efficiently and reliably. This is where composition patterns come into play.
Composition Patterns: An Overview
Composition patterns allow software developers to create systems using smaller, reusable components. Instead of building monolithic applications, developers can compose services to respond to demands quickly and effectively. Here are some widely used composition patterns in API management:
- Service Composition: Orchestrating multiple services to provide a unified response.
- API Gateway: A single entry point to a microservices architecture, managing traffic and aggregating responses.
- Request/Response Aggregation: Combining multiple API calls into a single call to decrease latency.
Service Composition
Service composition involves combining multiple services into a single workflow. It’s practical but can increase complexity if not well managed. Each service often has its own API, leading to orchestration challenges.
Example: Service Composition in Java
Let’s look at a sample use-case in Java where we compose two API calls to fetch user data and their associated posts.
import org.springframework.web.client.RestTemplate;
public class UserService {
private final RestTemplate restTemplate;
public UserService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public UserWithPosts getUserWithPosts(Long userId) {
String userApiUrl = "https://api.example.com/users/" + userId;
String postsApiUrl = "https://api.example.com/users/" + userId + "/posts";
User user = restTemplate.getForObject(userApiUrl, User.class);
List<Post> posts = restTemplate.getForObject(postsApiUrl, List.class);
return new UserWithPosts(user, posts);
}
}
Commentary on the Code
- RestTemplate: A Spring framework utility simplifying RESTful communication.
- Composition: In this example, we seamlessly combine user and their posts retrieval into one method.
In this simple example, handling errors is absent, yet in real-world applications, you'd augment this with robust error handling to ensure reliability.
Error Handling in Service Composition
By integrating error handling into our example, we can react appropriately to API failures:
public UserWithPosts getUserWithPosts(Long userId) {
User user;
List<Post> posts;
try {
user = restTemplate.getForObject(userApiUrl, User.class);
} catch (HttpClientErrorException e) {
throw new UserNotFoundException("User not found", e);
}
try {
posts = restTemplate.getForObject(postsApiUrl, List.class);
} catch (HttpClientErrorException e) {
posts = new ArrayList<>(); // Gracefully handle if the user's posts are not found
}
return new UserWithPosts(user, posts);
}
API Gateway Pattern
An API Gateway serves as the single access point to a microservices architecture. It simplifies interaction with multiple services while providing necessary functionality, including rate limiting, caching, and security.
Using an API gateway can significantly reduce API complexity by decoupling client-side logic from server-side services.
Example: Basic API Gateway Implementation
Below is a conceptual Java implementation of an API gateway using Spring Cloud Gateway:
import org.springframework.cloud.gateway.route.RouteLocator;
import org.springframework.cloud.gateway.builder.RouteLocatorBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
@Component
public class GatewayConfig {
@Bean
public RouteLocator customRoutes(RouteLocatorBuilder builder) {
return builder.routes()
.route("user_route", r -> r.path("/users/**")
.uri("http://user-service:8080"))
.route("post_route", r -> r.path("/posts/**")
.uri("http://post-service:8081"))
.build();
}
}
Commentary on the Gateway
- RouteLocator: It defines routes based on incoming requests.
- Service URL: Each route points to a specific service, abstracting complexity from clients.
With the API Gateway in place, the client does not need to know about the underlying services.
Request/Response Aggregation
Request/response aggregation aims to consolidate multiple API requests into a single request. This method minimizes the number of times data needs to be fetched by collating the necessary information into one call.
Example: Aggregation Pattern
In this example, we will aggregate user and posts retrieval into one endpoint. For this demonstration, we assume we have a method capable of aggregating these requests.
public class AggregatedService {
private final UserService userService;
private final PostService postService;
public AggregatedService(UserService userService, PostService postService) {
this.userService = userService;
this.postService = postService;
}
public UserWithPosts aggregateUserData(Long userId) {
CompletableFuture<User> userFuture = CompletableFuture.supplyAsync(() -> userService.getUser(userId));
CompletableFuture<List<Post>> postsFuture = CompletableFuture.supplyAsync(() -> postService.getUserPosts(userId));
User user = userFuture.join();
List<Post> posts = postsFuture.join();
return new UserWithPosts(user, posts);
}
}
Commentary on Aggregation
- CompletableFuture: A Java feature that allows asynchronous programming; it enables non-blocking calls to the user and post services.
- Efficiency: By waiting for both requests to respond simultaneously, overall latency is reduced.
Bringing It All Together
Managing API complexity in composition patterns presents a myriad of challenges but is attainable with strategic implementation. Understanding patterns such as service composition, API gateways, and request/response aggregation can significantly reduce your application’s complexity and enhance its performance.
When implementing these concepts, ensure you incorporate robust error handling, keep an eye on versioning, and constantly strive for clear documentation. Each piece contributes to a seamless user experience and maintainable codebase.
Resources for Further Learning
- Spring Cloud Documentation: Learn more about building efficient API gateways with Spring.
- Microservices Patterns: A comprehensive guide on effective microservices design.
As you embark on your API composition journey, consider these patterns, and take a structured approach to tackle complexity. Your applications will benefit from a cleaner architecture and smooth interactions with various services.
Checkout our other articles