Overcoming Refactoring Fatigue: Reignite Your Learning Spark
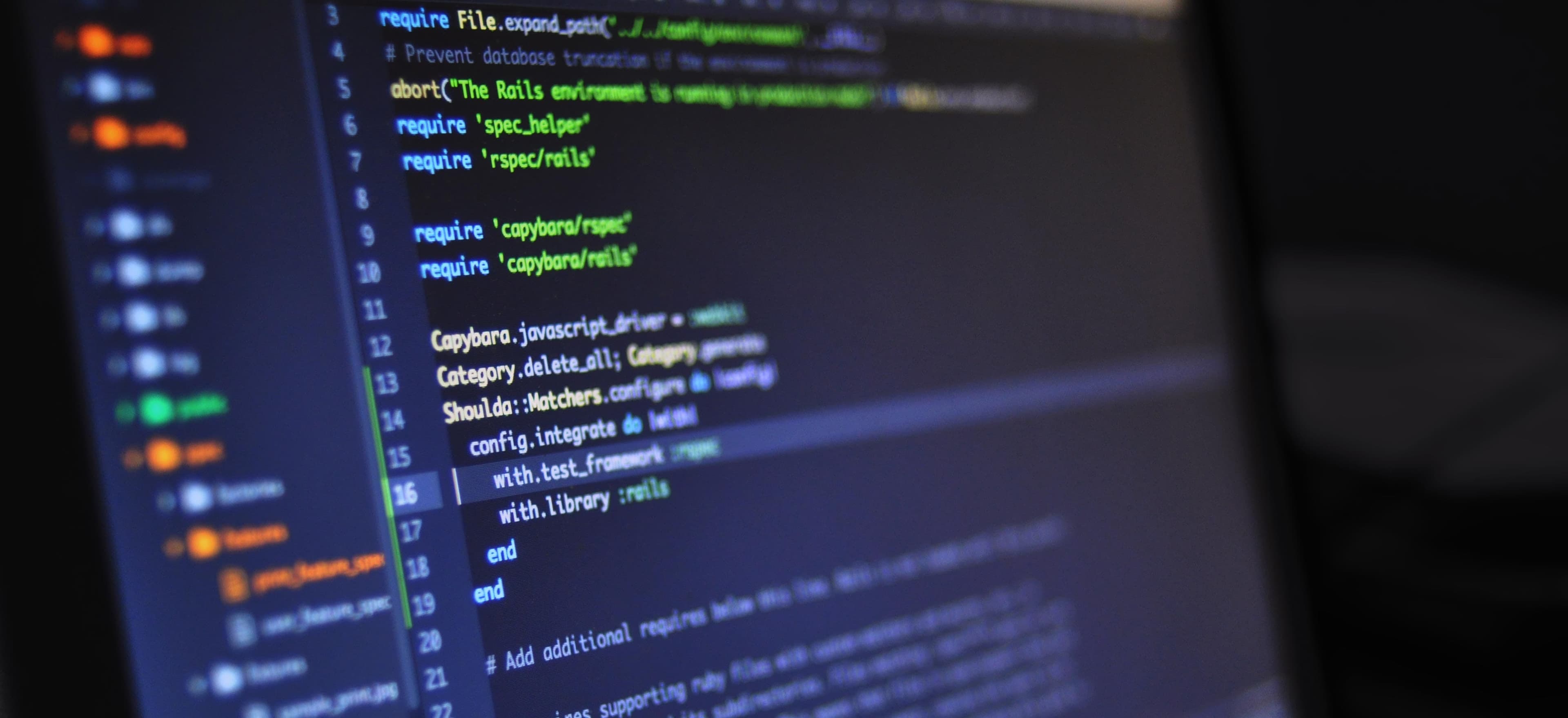
- Published on
Overcoming Refactoring Fatigue: Reignite Your Learning Spark
Refactoring is a crucial aspect of software development in Java and any programming language. It’s essential for maintaining clean, efficient, and understandable code. However, developers often find themselves stuck in a rut, feeling overwhelmed by the relentless need to refactor. This phenomenon is known as “refactoring fatigue.” If you’ve ever been in this position, you’re not alone. This post explores strategies to overcome refactoring fatigue and reignite your passion for learning and improving.
Understanding Refactoring Fatigue
Refactoring fatigue typically sets in when developers repeatedly engage in code revisions without seeing beneficial results. Signs of this fatigue may include:
- Feeling Overwhelmed: Constant changes can create a sense of chaos, making it difficult to focus.
- Loss of Motivation: When improvements seem minimal, the enthusiasm for refactoring can dwindle.
- Perfectionism Pressure: Developers may feel pressure to achieve an unattainable level of quality, leading to dissatisfaction.
It's important to acknowledge these feelings rather than brush them aside.
The Importance of Refactoring
Refactoring is not just about cleaning up code; it enhances its readability, reduces complexity, and promotes maintainability. Well-refactored code is easier to manage and extend, which is crucial in a dynamic environment where requirements change frequently.
Here are some key benefits of refactoring:
- Improved Code Quality: Cleaner code minimizes bugs and technical debt.
- Easier Debugging: Simpler code is less error-prone and easier to troubleshoot.
- Better Team Collaboration: Well-organized code helps team members understand each other’s work faster.
Tips to Overcome Refactoring Fatigue
1. Set Clear Goals
Before embarking on a refactoring session, establish clear and realistic goals. Whether it's cleaning up a specific module or improving the performance of a section of code, having defined objectives helps maintain focus.
Example: Instead of saying, "I will refactor the entire project today," say, "I will refactor the outdated database access methods to use Java Streams."
2. Break It Down
Tackling an entire codebase at once can be overwhelming. Instead, break the task into manageable chunks.
Code Snippet:
import java.util.List;
import java.util.stream.Collectors;
public class UserRepository {
private List<User> users;
public List<User> getUsersAboveAge(int age) {
// Refactoring into a Stream pipeline
return users.stream()
.filter(user -> user.getAge() > age)
.collect(Collectors.toList());
}
}
Commentary: In the above example, the existing method may involve excessive loops and conditions. By shifting to a Java Stream, you improve readability and reduce the cognitive load.
3. Utilize Automated Tools
Incorporate automated tools to help you refactor efficiently. Tools like SonarQube for code quality analysis and IntelliJ IDEA for automated refactoring can save time and reduce errors.
- SonarQube: Offers insights into code quality, suggesting areas that require refactoring.
- IntelliJ IDEA: Provides inline suggestions and quick fixes while coding.
4. Establish Refactoring Patterns
Adopt common refactoring patterns that can be applied across projects. Familiarity with these patterns not only speeds up the process but also boosts confidence.
Common Patterns:
- Extract Method: Move a block of code into a separate method to enhance clarity.
- Rename Variable: Give variables descriptive names to improve code understanding.
For example:
// Before
public void processOrder(Order o) {
// Processing order
}
// After
public void processOrder(OnlineOrder onlineOrder) {
// Processing online order
}
Commentary: By renaming parameters, we improve the semantic understanding of the method, making it clear what type of order is being processed.
5. Reflect on Past Successes
Reflect on previous refactoring successes. Understanding the positive outcomes after refactoring can reignite your motivation.
Ask yourself: What small changes resulted in significant improvements?
Keep a "refactoring log" that documents the benefits gained from earlier efforts. It can serve as a regular source of inspiration.
6. Collaborate with Peers
Pair programming or conducting code reviews with peers can introduce new perspectives. Collaborating helps lighten the refactoring load and offers fresh insights into potential improvements.
Example Activity: Set up a weekly code review session. Each developer presents a piece of code they recently refactored, explaining what challenges they faced and how they overcame them.
7. Embrace Continuous Learning
Stay informed about the latest trends and best practices in Java development. Engaging in continuous learning helps you stay excited and motivated.
- Follow Java blogs and podcasts.
- Participate in community forums like Stack Overflow and Java Reddit communities.
Some noteworthy resources that can help include:
8. Celebrate Small Wins
Celebrate your achievements, no matter how small. Finished refactoring a method? Share this victory with your team. Recognizing these accomplishments fosters a positive environment and reduces feelings of fatigue.
9. Set Boundaries
Set strict time limits for refactoring sessions. Allocate specific time slots in your workday dedicated to these activities. This helps compartmentalize the effort and prevents burnout.
The Closing Argument
Refactoring fatigue is common among developers, but it doesn't have to derail your passion for coding. By implementing clear goals, breaking down tasks, leveraging tools, collaborating with peers, and embracing continuous learning, you can overcome this fatigue effectively. Remember, every small improvement contributes to a more maintainable and efficient codebase. So, ignite your learning spark today, and tackle your refactoring tasks with renewed vitality.
By taking proactive steps, not only will you enhance your skill set, but you'll also positively impact your team's productivity, leading to a more cohesive development environment. Happy coding!
Further Reading:
This blog post aims to provide actionable insights into overcoming refactoring fatigue while simultaneously reinforcing your foundational skills in Java development. As you delve deeper into refactoring practices, remember that each change is a step toward crafting better software.
Checkout our other articles