Java Solutions for Managing Resources in Poultry Systems
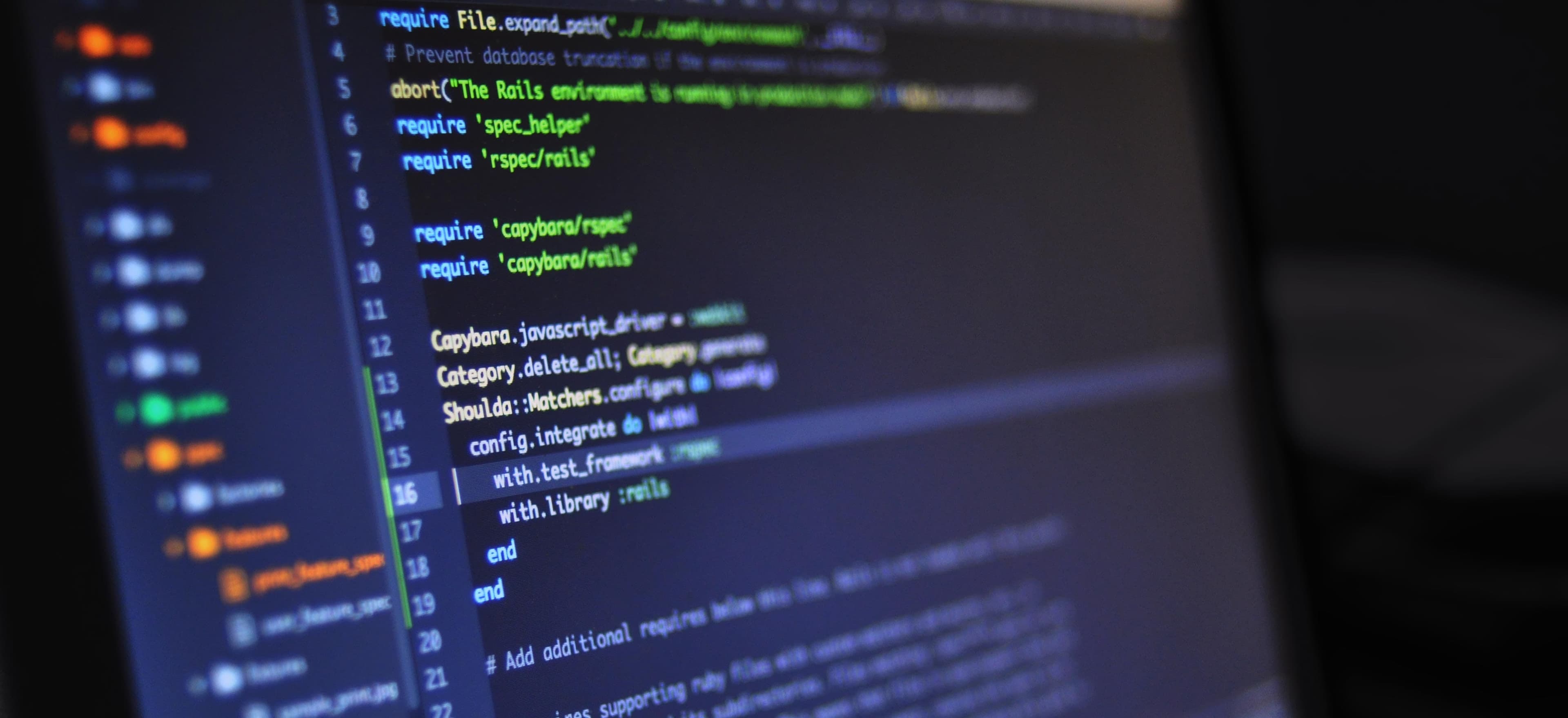
- Published on
Java Solutions for Managing Resources in Poultry Systems
Managing resources in poultry systems is an intricate task that requires precision, efficiency, and adaptability. Just like how brooder overcrowding in chicks needs immediate attention to ensure optimal growth and health (as discussed in this article), managing resource allocation—such as feed, water, and space—is crucial in maintaining a sustainable poultry operation.
Java, a robust and versatile programming language, can play a significant role in streamlining resource management processes. In this blog post, we will explore how Java can be utilized to tackle common challenges in poultry resource management with effective code snippets and commentary. Let’s dive in.
Understanding Resource Management in Poultry Systems
Before diving into the coding aspects, it's essential to understand what resource management in poultry entails. Efficient resource management can include:
- Feed Management: Ensuring that each bird receives the appropriate amount of feed.
- Water Supply: Monitoring and controlling water supply to avoid shortages.
- Space Utilization: Making sure that birds have enough space to grow comfortably without overcrowding.
Given these aspects, let's discuss Java's involvement in streamlining these processes.
Java Classes for Resource Management
When managing resources, we can represent birds and their resources as Java classes. Let’s start with a simple Bird
class.
Bird Class Implementation
public class Bird {
private String name;
private double weight;
private double dailyFeedRequirement;
public Bird(String name, double weight, double dailyFeedRequirement) {
this.name = name;
this.weight = weight;
this.dailyFeedRequirement = dailyFeedRequirement;
}
public String getName() {
return name;
}
public double getWeight() {
return weight;
}
public double getDailyFeedRequirement() {
return dailyFeedRequirement;
}
}
Commentary on the Bird Class
- Constructor: The constructor initializes the bird's name, weight, and daily feed requirement. This is crucial because it lays the groundwork for effective feed management.
- Getters: These methods allow us to access the private fields of the class. This encapsulation helps in maintaining the integrity of our bird objects.
Resource Manager Class
Now that we have a Bird
class, let’s implement a ResourceManager
class. This class will manage the feed allocation for multiple birds.
ResourceManager Class Implementation
import java.util.ArrayList;
import java.util.List;
public class ResourceManager {
private List<Bird> birds;
public ResourceManager() {
birds = new ArrayList<>();
}
public void addBird(Bird bird) {
birds.add(bird);
}
public double calculateTotalFeedRequirement() {
double totalFeed = 0.0;
for (Bird bird : birds) {
totalFeed += bird.getDailyFeedRequirement();
}
return totalFeed;
}
}
Commentary on the ResourceManager Class
- List Initialization: The
birds
variable is anArrayList
that holds all the bird objects. This selection allows for dynamic management of resources as we're likely to add or remove birds frequently. - calculateTotalFeedRequirement Method: This function iterates through all
Bird
objects to calculate the total feed requirement. This is a critical function for ensuring that you know how much feed is necessary each day to avoid shortages.
Example Usage
Let's see how we can use the Bird
and ResourceManager
classes together.
Example Code
public class PoultryManagementApp {
public static void main(String[] args) {
ResourceManager resourceManager = new ResourceManager();
// Adding some birds
resourceManager.addBird(new Bird("Chick1", 1.2, 0.2));
resourceManager.addBird(new Bird("Chick2", 1.5, 0.25));
resourceManager.addBird(new Bird("Chick3", 1.1, 0.15));
// Calculating total feed requirement
double totalFeed = resourceManager.calculateTotalFeedRequirement();
System.out.println("Total Feed Requirement for the day: " + totalFeed + " kg");
}
}
Explanation
- Main Application: The
main
method serves as the entry point of the application. It creates an instance ofResourceManager
and populates it with multipleBird
objects. - Output: The program outputs the total feed requirement, which will help poultry managers prepare feed in advance and avoid running low.
Monitoring Water Supply
Managing water supply is just as vital as feed management. We can create a WaterSupply
class to monitor and manage water allocation.
WaterSupply Class Implementation
public class WaterSupply {
private double totalWaterAvailable;
public WaterSupply(double totalWaterAvailable) {
this.totalWaterAvailable = totalWaterAvailable;
}
public boolean canSupply(double requiredWater) {
return requiredWater <= totalWaterAvailable;
}
public void useWater(double amount) {
if (amount <= totalWaterAvailable) {
totalWaterAvailable -= amount;
} else {
System.out.println("Not enough water available.");
}
}
public double getTotalWaterAvailable() {
return totalWaterAvailable;
}
}
Commentary on the WaterSupply Class
- Water Management Functions: The method
canSupply
checks if there is enough water available. This proactive approach ensures that resources are tracked meticulously. - Water Usage: The
useWater
method allows for decrementing the water supply when utilized, which reflects real-world scenarios accurately.
Integrating Water Management into the Poultry System
Updated Usage Example
Combining both ResourceManager
and WaterSupply
classes would look like this:
public class PoultryManagementApp {
public static void main(String[] args) {
ResourceManager resourceManager = new ResourceManager();
// Adding some birds
resourceManager.addBird(new Bird("Chick1", 1.2, 0.2));
resourceManager.addBird(new Bird("Chick2", 1.5, 0.25));
resourceManager.addBird(new Bird("Chick3", 1.1, 0.15));
// Calculating total feed requirement
double totalFeed = resourceManager.calculateTotalFeedRequirement();
System.out.println("Total Feed Requirement for the day: " + totalFeed + " kg");
// Managing water supply
WaterSupply waterSupply = new WaterSupply(10.0); // 10 liters available
double totalWaterNeeded = resourceManager.calculateTotalFeedRequirement(); // Assume water usage matches feed requirement
if (waterSupply.canSupply(totalWaterNeeded)){
waterSupply.useWater(totalWaterNeeded);
System.out.println("Water supplied successfully.");
} else {
System.out.println("Insufficient water supply.");
}
}
}
Final Thoughts
The integration of Java for poultry resource management shows how programming can assist in automating and optimizing processes that would otherwise take considerable time and effort. With effective classes like Bird
, ResourceManager
, and WaterSupply
, poultry farmers can focus on the health and growth of their stock rather than worrying about resource shortages.
To conclude, tools like Java can be game-changers in poultry management. Effective resource monitoring and allocation are key components in ensuring the well-being of livestock—much like addressing brooder overcrowding in chicks.
For more insights on managing your poultry system, check out Solving Brooder Overcrowding in Chicks for practical tips. Happy farming!
Checkout our other articles