Java Solutions for Managing Brooder Overcrowding Issues
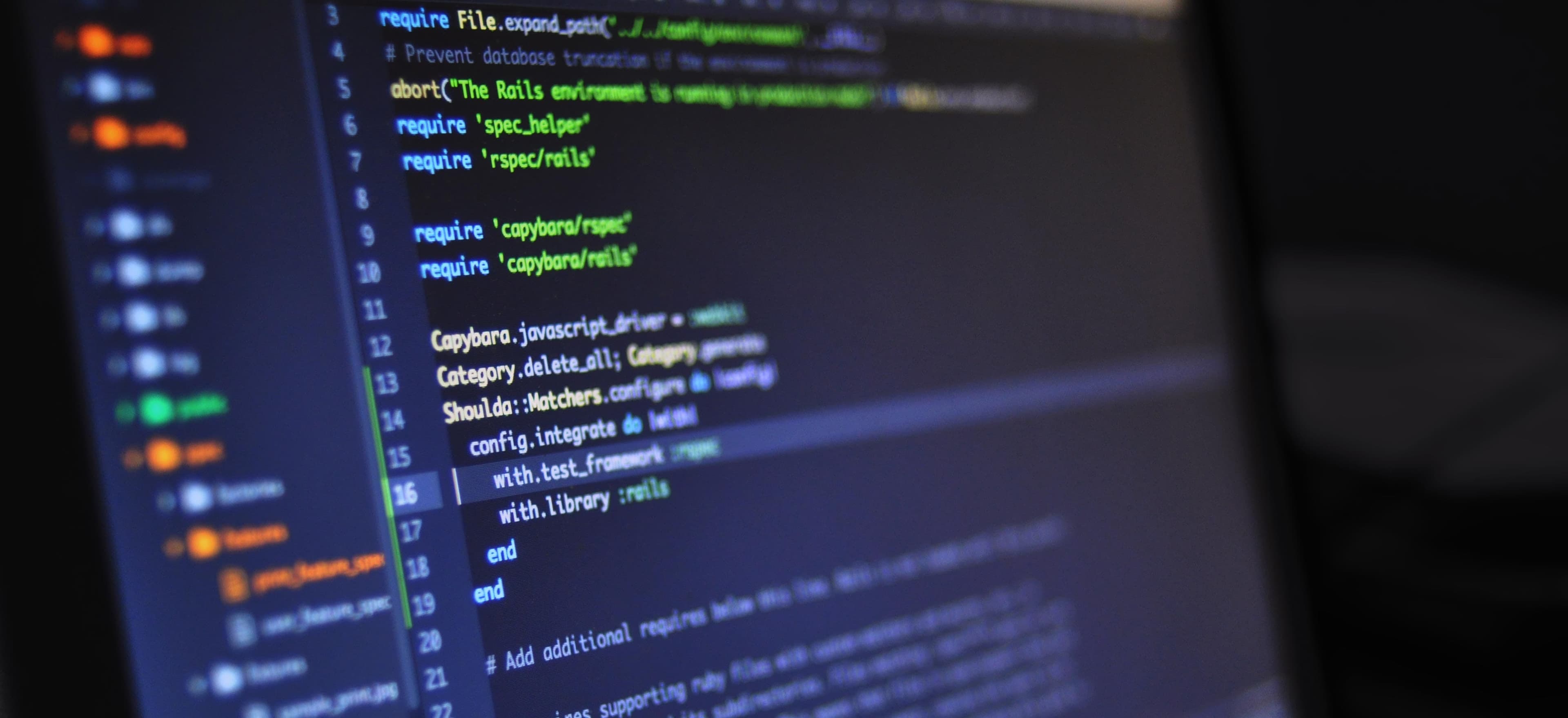
- Published on
Java Solutions for Managing Brooder Overcrowding Issues
Brooder overcrowding can be a critical problem in the care of chicks. Not only can it present significant welfare issues for the animals, it can also result in increased mortality and reduced growth rates. Solutions to these challenges often involve monitoring and adjusting environmental conditions, which can be efficiently managed using programming languages like Java. In this blog post, we will explore how you can use Java to develop a solution for managing brooder overcrowding issues and keep your chicks happy and healthy.
Understanding Brooder Overcrowding
Before we delve into the programming solutions, let's briefly discuss what brooder overcrowding is and why it matters. Brooder overcrowding occurs when too many chicks are placed within a confined space, which can lead to stress, aggressive behavior, and higher susceptibility to disease. The best approach is to monitor the number of chicks relative to the size of the brooder and ensure it is equipped with sufficient resources.
For an in-depth understanding of the issue, consider reading the article "Solving Brooder Overcrowding in Chicks" here.
Setting Up the Environment
To tackle the problem programmatically, we will require a monitoring and management system. This will generally involve the use of Java's object-oriented capabilities. Here's a simple structure of how you might go about setting this up.
Step 1: Create Chick Class
First, we need a basic Chick
class to represent each chick in the brooder.
public class Chick {
private String id;
private double weight; // in grams
private String healthStatus;
public Chick(String id, double weight, String healthStatus) {
this.id = id;
this.weight = weight;
this.healthStatus = healthStatus;
}
public String getId() {
return id;
}
public double getWeight() {
return weight;
}
public String getHealthStatus() {
return healthStatus;
}
}
Why this code? A Chick class allows us to structure the data associated with each chick, giving us a way to manage their individual properties. The properties include a unique identifier, weight for tracking growth, and health status to monitor well-being.
Step 2: Create Brooder Class
Next, we'll set up a Brooder
class. This class will oversee all chick entities and manage the total population and space utilization.
import java.util.ArrayList;
import java.util.List;
public class Brooder {
private List<Chick> chicks;
private double maxWeightCapacity; // maximum weight capacity in grams
private double currentWeight;
public Brooder(double maxWeightCapacity) {
this.maxWeightCapacity = maxWeightCapacity;
this.chicks = new ArrayList<>();
this.currentWeight = 0;
}
public boolean addChick(Chick chick) {
if (canAddChick(chick)) {
chicks.add(chick);
currentWeight += chick.getWeight();
return true;
}
return false;
}
private boolean canAddChick(Chick chick) {
return (currentWeight + chick.getWeight()) <= maxWeightCapacity;
}
public List<Chick> getChicks() {
return chicks;
}
public double getCurrentWeight() {
return currentWeight;
}
}
Why this code? In the Brooder
class, we maintain a list of Chick
instances, monitor the current total weight, and enforce a maximum weight capacity. This mechanism helps prevent overcrowding by restricting the addition of more chicks when the brooder is at capacity.
Step 3: Monitor Conditions
To manage overcrowding, we need functionality to monitor and adjust conditions continuously. To do this, we will create a method that regularly checks the status of chicks.
public void monitorChicks() {
for (Chick chick : chicks) {
if (chick.getHealthStatus().equals("sick")) {
// Take action, e.g., remove or quarantine the chick.
System.out.println("Chick " + chick.getId() + " is sick and needs removal.");
}
}
}
Why this code? Monitoring is crucial to prevent health issues from spreading within the brooder. Identifying sick chicks ensures action can be taken promptly, improving overall welfare and reducing potential losses.
User Interaction
Next, let's implement user interaction to build a simple interface that would allow the user to add chicks and monitor the status.
import java.util.Scanner;
public class BrooderManager {
public static void main(String[] args) {
Brooder brooder = new Brooder(1000); // Max capacity of 1000 grams
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the Brooder Management System!");
while (true) {
System.out.println("1. Add Chick\n2. Monitor Chicks\n3. Exit");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("Enter Chick ID:");
String id = scanner.next();
System.out.println("Enter Chick Weight (grams):");
double weight = scanner.nextDouble();
System.out.println("Enter Health Status:");
String healthStatus = scanner.next();
Chick newChick = new Chick(id, weight, healthStatus);
if (brooder.addChick(newChick)) {
System.out.println("Chick added successfully!");
} else {
System.out.println("Cannot add chick: maximum weight exceeded.");
}
break;
case 2:
brooder.monitorChicks();
break;
case 3:
System.exit(0);
}
}
}
}
Why this code? An interactive console allows users to add chick information and monitor their status easily. This format is user-friendly, reducing the potential for input errors and enhancing usability.
Future Enhancements
While this foundational setup serves to address overflow issues, further enhancements can be integrated to make the system more robust:
- Database Integration: Use a database for persistent storage of chick records, allowing for historical data analysis.
- Data Visualization: Implement graphs to visually depict growth rates and health monitoring over time.
- Alert Notifications: Integrate email or SMS notifications when overcrowding or health issues are detected.
To learn more about poultry care and strategies, please check the article "Solving Brooder Overcrowding in Chicks" here.
Key Takeaways
Effective management of brooder overcrowding is crucial for the health and growth of your chicks. By utilizing Java to build a monitoring and management system, you can proactively address potential issues with overcrowding, ensuring a safe and nurturing environment for your chicks. With further enhancements, your system can become an invaluable tool in your poultry management strategy.
In this blog post, you learned how to create a simple yet effective Java program that can help you manage brooder overcrowding. Start coding today and make raising chicks a joyful and productive experience!
Checkout our other articles