Top Tips to Prevent ClassCastException in Java
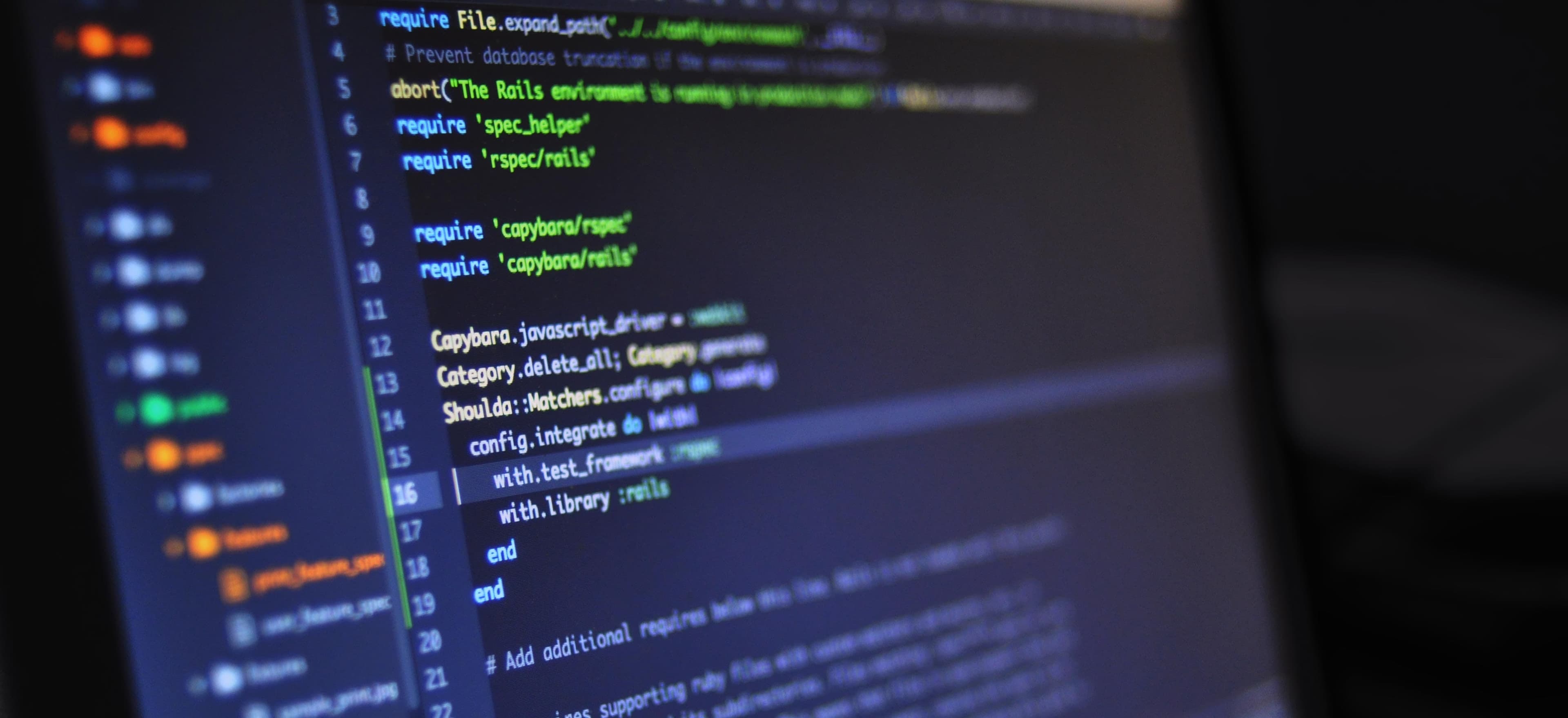
- Published on
Top Tips to Prevent ClassCastException in Java
In the realm of Java programming, dealing with types and casting can be intricate yet vital. One of the issues developers often encounter is the ClassCastException
. This exception typically arises when you try to cast an object to a class of which it is not an instance. Understanding how to prevent this exception can save your application from unexpected crashes and enhance overall code reliability. In this blog post, we will delve into strategies to avoid ClassCastException
, illustrated with code snippets and clear explanations.
Understanding ClassCastException
Before diving into the prevention techniques, let's clarify what ClassCastException
is. This unchecked exception occurs at runtime when:
- You attempt to cast an object to a subclass that it is not an instance of.
For example, consider the following code:
Object obj = new Integer(10);
String str = (String) obj; // This will cause ClassCastException
In this scenario, the cast fails because obj
is an Integer
, not a String
. Java throws a ClassCastException
, indicating that the object cannot be converted.
Tip 1: Use the instanceof
Operator
A straightforward way to prevent ClassCastException
is to utilize the instanceof
operator. This operator checks whether an object is an instance of a specific class or interface before performing the cast.
Example:
Object obj = getSomeObject(); // Assume this returns an Object
if (obj instanceof String) {
String str = (String) obj; // Safe cast
System.out.println(str);
} else {
System.out.println("Object is not a String.");
}
Why Use instanceof
?
Using instanceof
ensures that you only attempt to cast an object that is compatible with the target type. This prevents runtime errors, enhancing the robustness of your code. Remember, however, that overuse of instanceof
can lead to code that is hard to manage and maintain.
Tip 2: Utilize Generics
Generics in Java allow you to define classes, interfaces, and methods with a placeholder for the type of data they operate on. By using generics, you can enforce type checks at compile-time instead of relying on runtime checks.
Example:
import java.util.List;
public class GenericList<T> {
private List<T> items;
public GenericList(List<T> items) {
this.items = items;
}
public T getItem(int index) {
return items.get(index);
}
}
Why Use Generics?
Generics provide strong type safety. If you attempt to add an incorrect type to a GenericList<String>
, the compiler will generate an error. This means you can catch potential ClassCastException
issues during compilation, leading to safer and more reliable code.
Tip 3: Leverage Polymorphism
Polymorphism allows you to invoke methods on an object without needing to know its exact type at compile time. This can often eliminate the need for casting altogether.
Example:
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
void makeSound() {
System.out.println("Bark");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow");
}
}
// Usage
Animal myDog = new Dog();
Animal myCat = new Cat();
myDog.makeSound(); // Bark
myCat.makeSound(); // Meow
Why Use Polymorphism?
By designing your software with polymorphism in mind, you reduce the reliance on casting altogether. Each class will handle its type, allowing flexibility and reducing the risk of ClassCastException
. This principle also adheres to Object-Oriented Programming best practices.
Tip 4: Use Collections with Care
When using Java collections like ArrayList
or HashMap
, always be sure to utilize generics. This reduces casting operations and helps ensure that you are working with data of the expected type.
Example:
List<String> stringList = new ArrayList<>();
stringList.add("Hello");
stringList.add("World");
for (String str : stringList) {
System.out.println(str.toUpperCase()); // No casting needed
}
Why Use Type-Safe Collections?
Using collections with generics ensures that only specific types of objects can be added, preventing ClassCastException
. If a developer mistakenly tried to add an Integer
to a List<String>
, the Java compiler would flag it as an error.
Tip 5: Follow Clear Type Design
A well-defined type hierarchy can help in avoiding type casting issues. If the types in your system are clear and logically structured, the need for casting may decrease.
Example:
interface Vehicle {
void start();
}
class Car implements Vehicle {
public void start() {
System.out.println("Car started");
}
}
class Motorcycle implements Vehicle {
public void start() {
System.out.println("Motorcycle started");
}
}
// Usage
Vehicle myVehicle = new Car();
myVehicle.start(); // polymorphic behavior
Why Have a Clear Type Design?
An efficient type hierarchy reduces complexity. Clear interfaces and abstract classes prevent the need for unnecessary casting. This leads to code that is easier to read, understand, and maintain.
Tip 6: IDE and Static Analysis Tools
Take advantage of Integrated Development Environments (IDEs) and static analysis tools that provide warnings and insights. Tools like IntelliJ IDEA or Eclipse often alert you when you are about to perform a potentially hazardous cast.
Example:
Assuming you create a wrong cast like this in your IDE:
Object obj = "Hello";
Integer num = (Integer) obj; // IDE flags this line
Why Rely on IDE Assistance?
Using IDEs to check code during development can highlight potential casting issues before you even run the application. This practice contributes to the overall quality and stability of your code.
Closing the Chapter
Avoiding ClassCastException
in Java is achievable with a combination of strategies such as using instanceof
, leveraging generics, adhering to polymorphism, and ensuring clear type designs. By following the tips discussed in this blog post, you can enhance your code's reliability and performance.
For more information on Java error handling and best practices, consider checking out the official Java documentation.
Start implementing these strategies in your own projects, and witness a significant reduction in ClassCastException
. Happy coding!
Checkout our other articles