Optimizing Java-Based Solutions for Efficient Chick Brooding
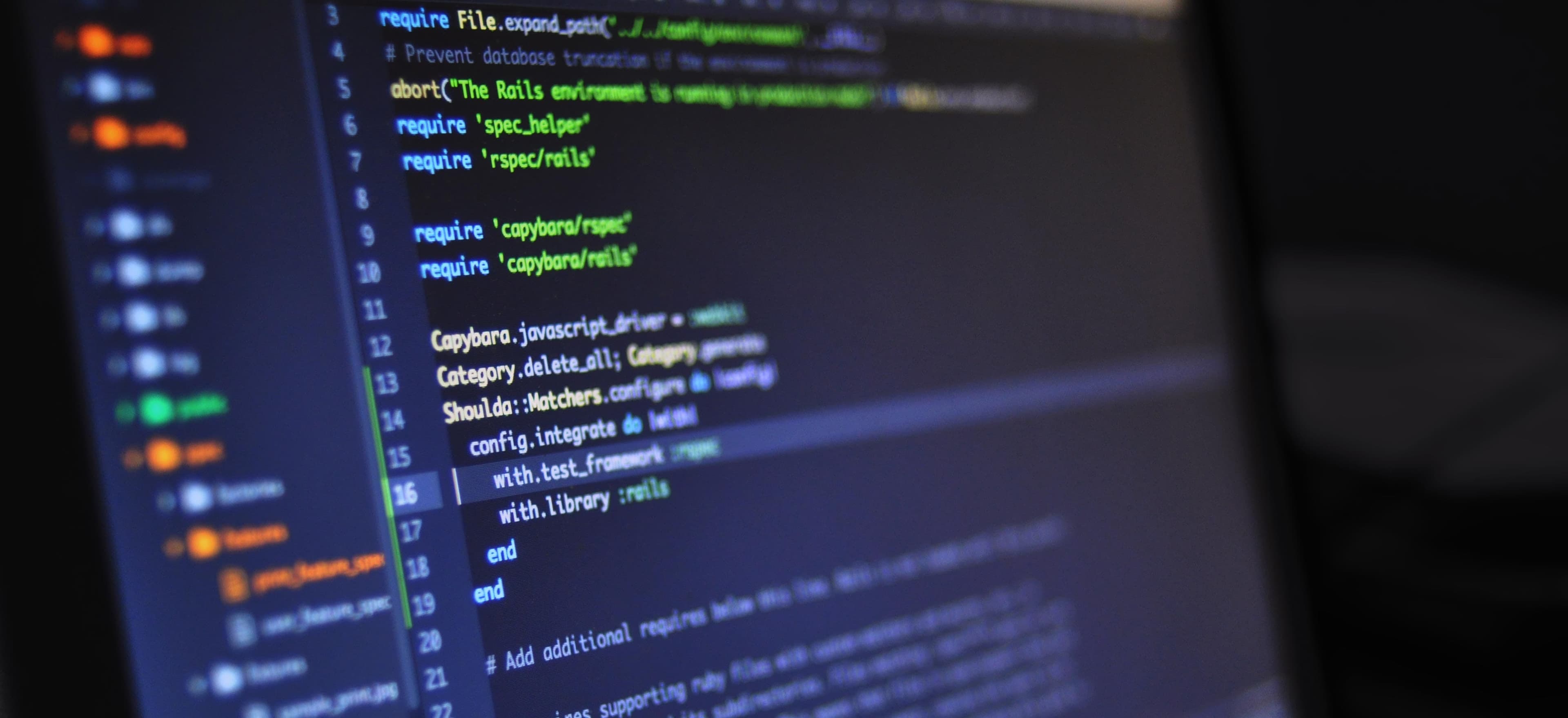
- Published on
Optimizing Java-Based Solutions for Efficient Chick Brooding
Chick brooding is a crucial aspect of poultry farming, ensuring that young chicks have a safe and controlled environment for healthy growth. With the increasing complexities of modern farming, leveraging technology to enhance brooding processes is essential. In this blog post, we’ll explore how Java can be used to create efficient solutions for chick brooding, particularly regarding managing brooder overcrowding. We will include detailed code examples and provide insights into optimizing these solutions for better performance.
The Importance of Automated Solutions
The traditional method of managing brooding environments can often lead to overcrowding challenges. Overcrowded conditions can result in stress, illness, and loss among chicks. Therefore, implementing automated solutions can simplify monitoring and control, ultimately enhancing the welfare of the chicks.
For an in-depth look at chick brooding practices, including overcrowding issues, check out the article Solving Brooder Overcrowding in Chicks.
Using Java to Monitor Environmental Conditions
Java is an excellent language for developing applications that require complex calculations and efficient data handling. In brooding environments, it can be used to monitor factors like temperature, humidity, and light levels to ensure optimal conditions.
Basic Environmental Monitoring System
In this section, we will create a simple Java program to read environmental data from sensors (hypothetical, as we won’t be using actual sensors here) and alert if conditions fall outside of acceptable ranges.
Here's a basic setup:
import java.util.Scanner;
public class EnvironmentalMonitor {
// Acceptable thresholds for temperature and humidity
private static final double MIN_TEMP = 35.0;
private static final double MAX_TEMP = 40.0;
private static final double MIN_HUMIDITY = 60.0;
private static final double MAX_HUMIDITY = 80.0;
public void checkEnvironment(double temperature, double humidity) {
if (temperature < MIN_TEMP || temperature > MAX_TEMP) {
System.out.println("Warning: Temperature " + temperature + "°C is outside acceptable range!");
}
if (humidity < MIN_HUMIDITY || humidity > MAX_HUMIDITY) {
System.out.println("Warning: Humidity " + humidity + "% is outside acceptable range!");
}
}
public static void main(String[] args) {
EnvironmentalMonitor monitor = new EnvironmentalMonitor();
Scanner scanner = new Scanner(System.in);
System.out.print("Enter temperature in °C: ");
double temperature = scanner.nextDouble();
System.out.print("Enter humidity percentage: ");
double humidity = scanner.nextDouble();
monitor.checkEnvironment(temperature, humidity);
scanner.close();
}
}
How the Code Works
-
Threshold Constants: We define acceptable ranges for temperature and humidity as constants for easy adjustments.
-
Environment Check: The
checkEnvironment
method evaluates input against the defined thresholds and issues warnings as necessary. -
User Input: The program prompts users to enter current readings, simulating real-world usage.
This straightforward approach offers a real-time assessment. It's crucial for farmers to monitor these parameters actively since fluctuating conditions can jeopardize the health of their chicks.
Data Collection and Analysis
To enhance our monitoring system, we can incorporate data collection and analysis capabilities. By storing historical data, we can identify trends and make informed decisions about adjustments to brooding environments.
Enhancing the Monitoring System with Data Collection
We will modify the previous program to include a simple way of logging data.
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class EnhancedEnvironmentalMonitor {
private static final double MIN_TEMP = 35.0;
private static final double MAX_TEMP = 40.0;
private static final double MIN_HUMIDITY = 60.0;
private static final double MAX_HUMIDITY = 80.0;
private static final String LOG_FILE = "environment_log.txt";
public void checkEnvironment(double temperature, double humidity) {
String message = "";
if (temperature < MIN_TEMP || temperature > MAX_TEMP) {
message += "Warning: Temperature " + temperature + "°C is outside acceptable range!\n";
}
if (humidity < MIN_HUMIDITY || humidity > MAX_HUMIDITY) {
message += "Warning: Humidity " + humidity + "% is outside acceptable range!\n";
}
logData(temperature, humidity, message);
System.out.print(message);
}
private void logData(double temperature, double humidity, String message) {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(LOG_FILE, true))) {
writer.write("Temperature: " + temperature + "°C, Humidity: " + humidity + "%, " + message);
writer.newLine();
} catch (IOException e) {
System.out.println("Error logging data: " + e.getMessage());
}
}
public static void main(String[] args) {
EnhancedEnvironmentalMonitor monitor = new EnhancedEnvironmentalMonitor();
Scanner scanner = new Scanner(System.in);
System.out.print("Enter temperature in °C: ");
double temperature = scanner.nextDouble();
System.out.print("Enter humidity percentage: ");
double humidity = scanner.nextDouble();
monitor.checkEnvironment(temperature, humidity);
scanner.close();
}
}
Explanation of Enhancements
-
Logging Mechanism: The new
logData
method appends readings to a text file, allowing for long-term data storage. -
Error Handling: Try-catch blocks are used around file operations to gracefully handle errors in logging.
-
Improved User Feedback: The user receives immediate feedback about the environmental conditions, either reassuring them or prompting corrective actions.
By leveraging logged data effectively, farmers can track environmental changes and investigate potential issues. This aspect is invaluable for optimizing brooding conditions and ensuring the health of the chicks.
Integrating with IoT Technologies
To take the monitoring system a step further, integrating Java with IoT technologies can provide real-time monitoring and alerts. Using MQTT or HTTP protocols to send data from environmental sensors to a central server allows for remote monitoring.
Basic MQTT Integration Example
Assuming you have an MQTT broker set up, our Java application can publish environment data as follows:
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
public class IoTEnvironmentalMonitor {
private static final String BROKER_URL = "tcp://yourbrokerurl:1883";
public void sendData(double temperature, double humidity) {
try {
MqttClient client = new MqttClient(BROKER_URL, MqttClient.generateClientId());
client.connect();
String payload = "Temperature: " + temperature + ", Humidity: " + humidity;
MqttMessage message = new MqttMessage(payload.getBytes());
client.publish("chick-brooding/environment", message);
client.disconnect();
} catch (MqttException e) {
System.out.println("Error sending data: " + e.getMessage());
}
}
}
Why Use MQTT?
-
Real-Time Data: MQTT enables real-time data transfer, ensuring that brooding conditions are monitored constantly.
-
Lightweight Protocol: Ideal for resource-constrained devices, MQTT is efficient in terms of bandwidth and energy consumption.
With this integration, you can monitor your brooding environment from anywhere, significantly improving your response times to environmental fluctuations.
Bringing It All Together
Implementing Java-based solutions for efficient chick brooding involves creating monitoring systems that ensure optimal growth conditions. Through environmental monitoring, data collection, and integration with IoT technologies, farmers can significantly enhance their practices.
By leveraging these efficient solutions, poultry farmers can address overcrowding challenges and provide healthier environments for their chicks. For further insights on dealing with chick overcrowding, visit Solving Brooder Overcrowding in Chicks.
As technology continues to evolve, so will the methods we use in agriculture. By adopting these advancements, we can foster a more effective and humane approach to livestock management.
Checkout our other articles