Improving Java Code Clarity with Effective Boolean Naming
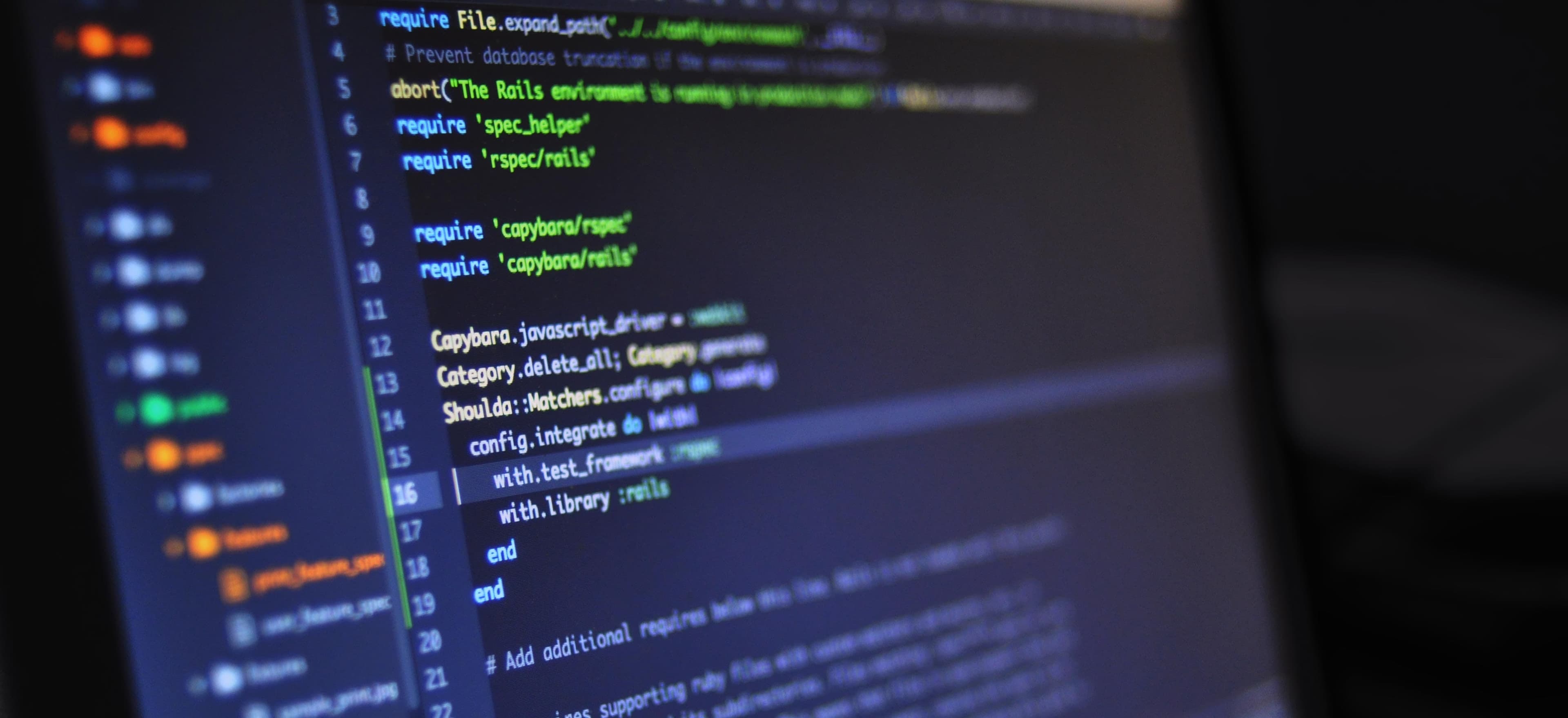
- Published on
Improving Java Code Clarity with Effective Boolean Naming
In the world of programming, clarity is paramount. As developers, we often overlook the subtle yet profound impact that proper naming conventions can have on our code's readability. One of the most critical areas where this is evident is in the naming of Boolean variables. This blog post will explore effective practices for naming Boolean variables in Java to enhance the clarity and maintainability of your code.
Why Boolean Naming Matters
Boolean variables are used to represent true or false conditions. They often control the flow of logic in a program, deciding what actions to take based on certain conditions. Poorly named Boolean variables can lead to confusion, making it difficult for others (or even yourself) to understand the intended use of these variables at a later date.
Consider this scenario:
boolean flag = true;
What does flag
represent? Is it an indicator of success, a trigger for a process, or something entirely different? Without additional context, it's unclear. Proper naming provides that context.
A Real-World Example
Let's take a look at a more meaningful example of Boolean naming. Imagine we're working on a user authentication feature in a web application. Instead of using vague terms like isActive
or flag
, we can use descriptive names.
boolean isUserAuthenticated = true;
Here’s why this name is effective:
- Clarity: It is immediately evident that this Boolean variable indicates whether a user is authenticated.
- Maintains Context: It helps future developers understand its purpose without additional comments or documentation.
Guidelines for Naming Boolean Variables
To promote clarity in your Boolean naming, consider the following guidelines:
1. Use Descriptive Names
Descriptive names provide context to the Boolean variable's purpose. Avoid single-letter or ambiguous names.
Good Example:
boolean isUserLoggedIn = false;
Bad Example:
boolean logged = false;
2. Start with a Verb
Boolean variable names often read better when they start with a verb. This reinforces that the variable represents a state.
Examples:
boolean isCompleted; // Indicates whether a task is completed.
boolean hasPermission; // Indicates whether a user has the required permissions.
3. Use Positive Naming
Using affirmative terms helps to avoid double negatives, which can lead to confusion. Instead of isNotAvailable
, use isAvailable
.
Example:
boolean isPermissionGranted = false;
4. Maintain Consistency
Adopt a consistent naming convention across your codebase. Whether you prefer camelCase, snake_case, or another convention, sticking with one style ensures continuity.
Example:
If you choose camelCase:
boolean isEmailVerified;
5. Reference Existing Patterns
Following established naming patterns can help. For instance, reading articles like Mastering Boolean Variable Names for Readable Code offers insights into industry-standard practices.
Examples in Practice
Now that we have established some guidelines, let's look at real-world Java code examples illustrating effective Boolean naming.
Example 1: User Registration Logic
Imagine a method that checks if a user can proceed with registration based on their email's validity.
public boolean canProceedWithRegistration(String email) {
boolean isEmailValid = isValidEmail(email);
boolean isEmailRegistered = checkIfEmailRegistered(email);
return isEmailValid && !isEmailRegistered;
}
private boolean isValidEmail(String email) {
// Logic to validate email
}
private boolean checkIfEmailRegistered(String email) {
// Logic to verify if the email is already in use
}
Here, we see how isEmailValid
and isEmailRegistered
provide an immediate context about what these variables represent.
Example 2: Game Development
In a gaming context, we may want to track whether a player has unlocked certain achievements.
public void updateAchievements(Player player) {
boolean hasCompletedAllLevels = player.getCompletedLevels() == totalLevels;
boolean hasAcquiredSpecialSkills = player.hasAllSkills();
if (hasCompletedAllLevels && hasAcquiredSpecialSkills) {
player.unlockAchievement("Master Player");
}
}
With hasCompletedAllLevels
and hasAcquiredSpecialSkills
, anyone reading the code can understand the conditions for unlocking an achievement without external documentation.
My Closing Thoughts on the Matter
Effective naming of Boolean variables is not just a matter of personal preference; it's a crucial factor in maintaining a clean, readable codebase. By following the guidelines outlined in this post, developers can significantly improve the clarity of their Java code. This helps foster better collaboration within teams and simplifies the debugging and maintenance process.
For further insight into this topic, I recommend reading Mastering Boolean Variable Names for Readable Code. This resource provides additional strategies and perspectives that can enrich your coding practices.
By taking the time to name your Boolean variables thoughtfully, you invest in the quality of your code now and for the future. After all, clear code is better code.
Checkout our other articles