Boosting Java Application Monitoring with Prometheus Alerts
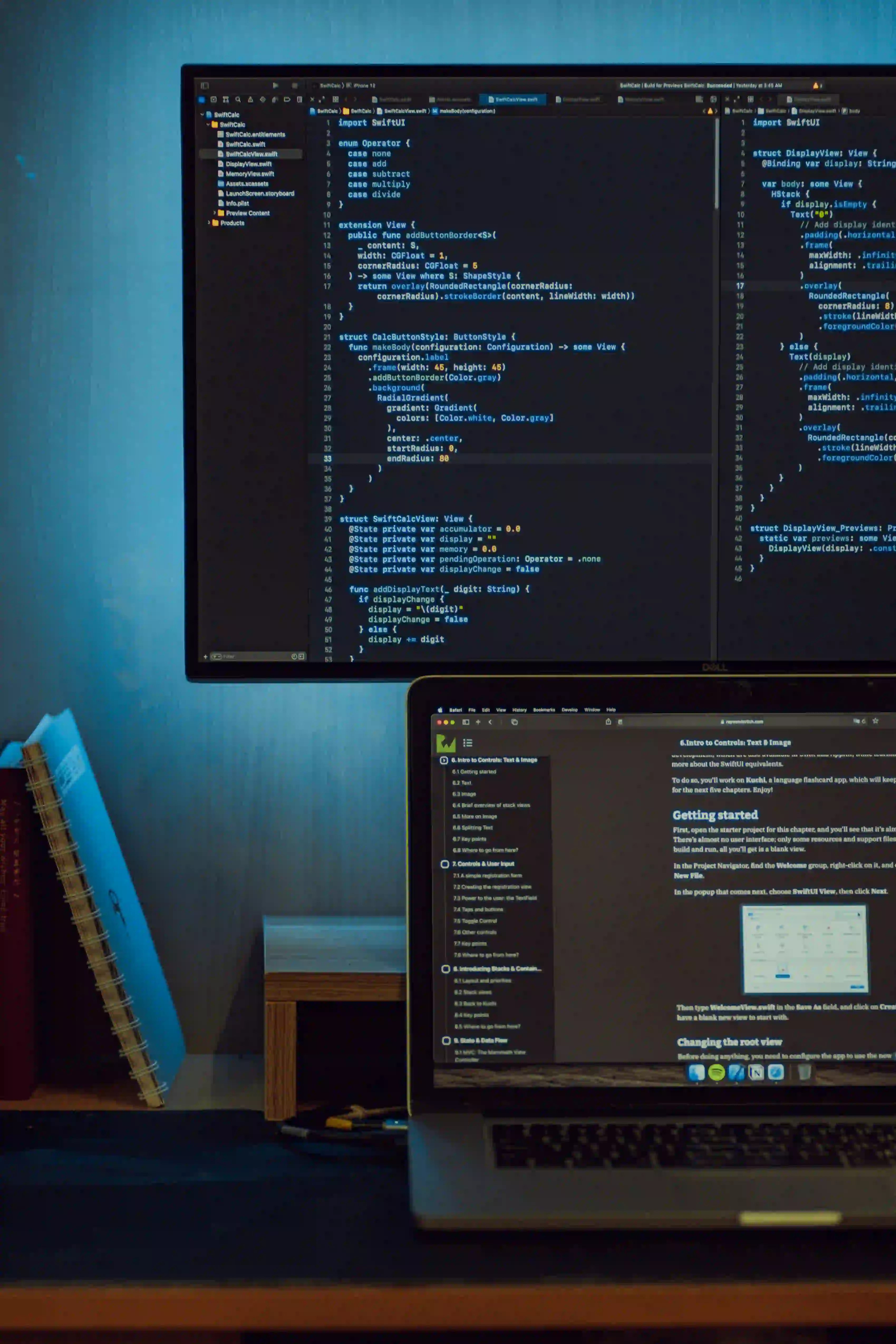
Boosting Java Application Monitoring with Prometheus Alerts
In today's tech landscape, monitoring applications effectively is crucial for maintaining performance, reliability, and user satisfaction. As Java continues to be one of the most prevalent programming languages—especially for enterprise-level applications—integrating robust monitoring capabilities within Java applications is paramount. One of the most effective ways to accomplish this is by leveraging Prometheus for alert notifications.
In this post, we’ll delve into why integrating Prometheus with your Java applications is essential for monitoring, how to implement it effectively, and offer practical code snippets to get you started. Additionally, we’ll reference existing methods to enhance your alert notifications as discussed here.
Understanding Prometheus and Its Role in Monitoring
Prometheus is an open-source monitoring and alerting toolkit that is widely utilized for its robust capabilities. It excels at collecting metrics from configured targets at specified intervals, storing them in its time-series database, and allowing queries to analyze these metrics efficiently. The role of Prometheus becomes even more vital when integrated with Java applications, which often have complex architectures and require granular monitoring.
Key Features of Prometheus:
- Multi-dimensional data model: Supports various metrics types, making it versatile.
- Powerful query language (PromQL): Provides robust capabilities for aggregating and analyzing metrics.
- Alerting capabilities: Enables the setup of alerts based on metrics to proactively address issues.
Why Java Developers Should Be Concerned with Monitoring
Monitoring is not just reserved for production environments. Even in development stages, having visibility into application performance can lead to significant advantages, including:
- Early Detection of Issues: Monitoring helps identify potential bottlenecks and errors early in the development lifecycle.
- Performance Optimization: Understanding how your application behaves under load allows for informed tuning and optimization.
- Resource Management: Assessing resource usage helps manage costs, particularly in cloud environments.
Getting Started with Prometheus in Java Applications
To get started with Prometheus, we'll need to introduce a Prometheus client into our Java application. Apache's Micrometer is the recommended way to do this, as it offers a wide range of instrumentation capabilities that easily integrate with Prometheus.
Step 1: Adding Dependencies
To utilize Micrometer in your Java project, you will first need to include the necessary dependencies. If you are using Maven, here's how to include Micrometer and the Prometheus registry in your pom.xml
:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
<version>1.7.2</version> <!-- Check for the latest version -->
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
<version>1.7.2</version> <!-- Check for the latest version -->
</dependency>
Why: Adding these dependencies allows your Java application to use Micrometer for collecting metrics and exporting them to Prometheus.
Step 2: Setting Up a Metrics Endpoint
Next, you need to expose a metrics endpoint that Prometheus can scrape. Here’s how to set this up in a Spring Boot application:
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class Application {
private final MeterRegistry meterRegistry;
public Application(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@GetMapping("/sample-endpoint")
public String sampleEndpoint() {
// Increment a simple counter every time this endpoint is hit
meterRegistry.counter("hits", "endpoint", "sample-endpoint").increment();
return "Hello, World!";
}
}
Why: By creating a sample endpoint that increments a counter, you can easily see how many times it is accessed. This is a practical way to start collecting metrics.
Step 3: Configuring Prometheus
Once you expose your metrics using Micrometer, the next step is configuring Prometheus to scrape these metrics. Add the following to your prometheus.yml
configuration file:
scrape_configs:
- job_name: 'java_application'
metrics_path: '/actuator/prometheus' # Update this path based on your setup
static_configs:
- targets: ['localhost:8080'] # Update this host and port
Why: This configuration tells Prometheus to scrape the metrics from your Java application. The targets
field should match the host and port where your application is running.
Step 4: Setting Up Alert Notifications
With your Prometheus instance configured to scrape your Java application metrics, the next step is to establish alerting rules. For instance, if you want to alert when the number of requests exceeds a certain threshold, you would create a rule like below in your prometheus.yml
:
groups:
- name: Java Application Alerts
rules:
- alert: HighRequestVolume
expr: sum(rate(hits[1m])) > 100 # Adjust threshold accordingly
for: 5m
labels:
severity: critical
annotations:
summary: "High request volume detected"
description: "Request volume on sample-endpoint has exceeded 100 requests per minute."
Why: These alerting rules ensure that you get notified when your application is under stress, allowing you to take necessary actions quickly.
Improving Alert Notifications
To further enhance your alert notifications, consider implementing best practices discussed in the article titled Improving Prometheus Alert Notifications. These include:
- Personalized Notification Channels: Ensure notifications reach the right teams, using tools like Slack or email notifications.
- Clear and Contextual Messages: Alerts should have clear descriptions and contexts so that the necessary actions can be taken swiftly.
My Closing Thoughts on the Matter
Integrating Prometheus with your Java applications significantly boosts your ability to monitor performance, detect issues, and manage resources. By following the steps outlined above, you can set up a robust monitoring system that provides transparent metrics and alerts to keep your application running smoothly.
As technology evolves, be sure to stay current with developments in monitoring tools and strategies, particularly as they pertain to Java applications. Implementing robust alert notifications and leveraging resources like Improving Prometheus Alert Notifications can significantly enhance your monitoring strategy.
Further Reading
For additional insights on best practices in application monitoring, consider exploring related topics such as:
- Instrumentation for Custom Metrics
- Best Practices for Using Prometheus with Microservices
- Advanced Alert Rules and Dashboards
By adopting these practices, you ensure your Java applications not only remain operational but also meet the performance standards expected in today's fast-paced environments. Happy coding!