Avoiding Async Pitfalls in Java: Techniques for Success
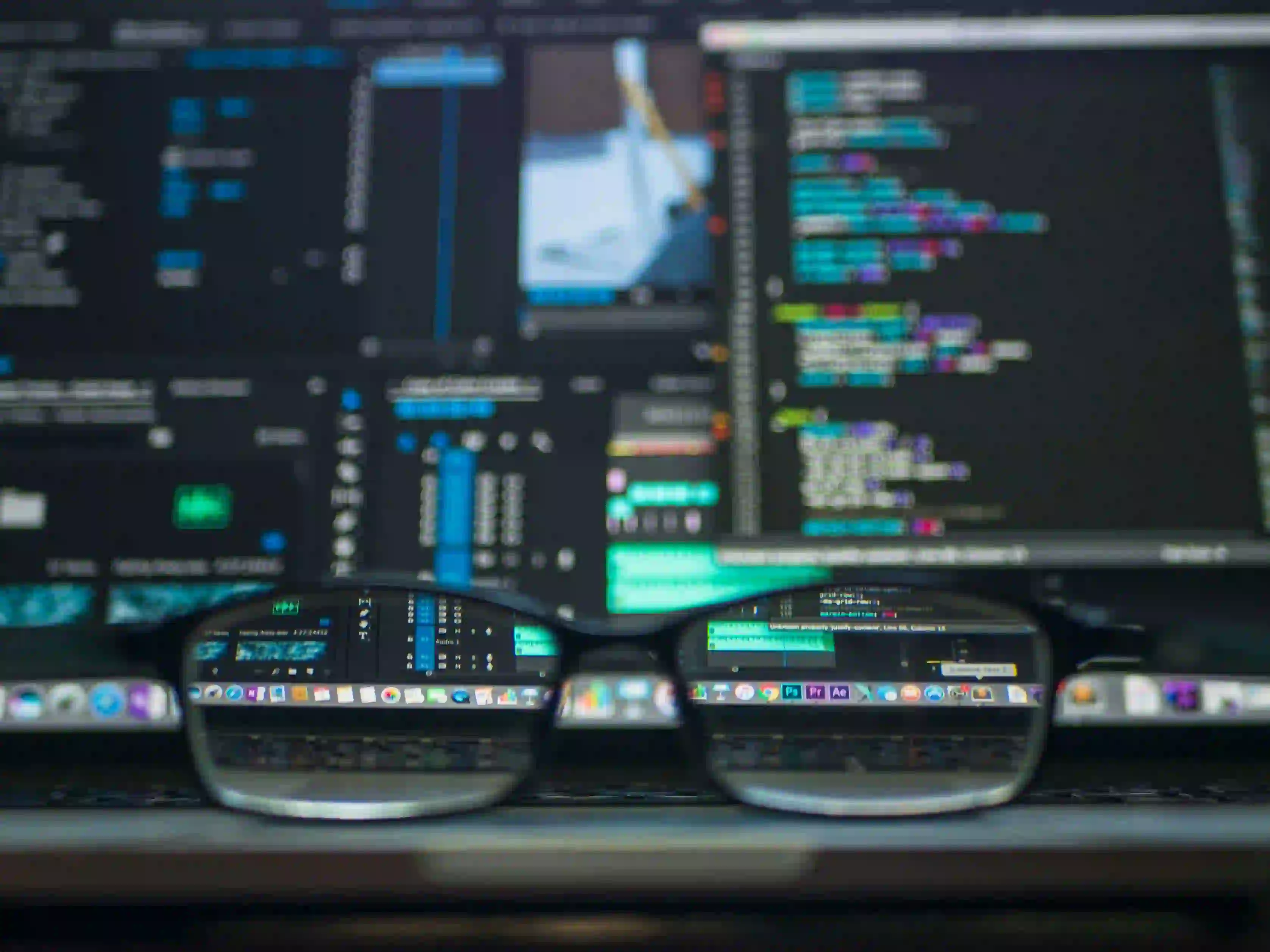
Avoiding Async Pitfalls in Java: Techniques for Success
Asynchronous programming has become an essential paradigm in modern software development, especially in Java. With the rise of frameworks like Spring and Java's native support for asynchronous operations, it's important to understand how to manage these effectively. In this blog post, we will explore common pitfalls related to asynchronous programming in Java and discuss techniques to avoid them.
Whether you're building a web application, a microservice, or any system where responsiveness matter, mastering asynchronous programming will help you deliver high-quality applications.
Understanding Asynchronous Programming in Java
Before we dive into techniques and pitfalls, let's clarify what asynchronous programming is. Asynchronous programming allows a program to initiate a task and move on to the next task without waiting for the previous one to complete. This is particularly useful in applications that perform I/O operations such as reading from a database or making HTTP requests.
Java provides several mechanisms to handle asynchronous operations, including the CompletableFuture
, ExecutorService
, and the reactive programming paradigm via libraries like Project Reactor and RxJava.
Why Use Asynchronous Programming?
- Performance: By not blocking threads, you can handle more requests simultaneously.
- Resource utilization: Improved CPU and memory usage since tasks can run concurrently.
- Responsiveness: Applications, especially UI-based ones, remain responsive to user input.
However, with these advantages come challenges and pitfalls.
Common Pitfalls in Asynchronous Programming
1. Handling Exceptions Properly
When dealing with asynchronous tasks, exceptions may not propagate as they do in synchronous code. This can lead to silent failures if not handled properly.
Example
CompletableFuture.supplyAsync(() -> {
// Simulating a task that may throw an exception
if (new Random().nextBoolean()) {
throw new IllegalStateException("Simulated Exception");
}
return "Completed Successfully";
}).exceptionally(ex -> {
// Handling the exception
System.out.println("Exception: " + ex.getMessage());
return "Fallback result";
});
In this example, we use exceptionally
to handle exceptions that occur during the execution of the CompletableFuture. It's crucial to implement proper error handling to ensure your application remains stable and provides feedback.
2. Thread Management
Improper thread management can lead to performance bottlenecks or resource exhaustion. Creating too many threads or not reusing existing ones can cause overhead.
Example
ExecutorService executorService = Executors.newFixedThreadPool(10);
CompletableFuture.runAsync(() -> {
// Task logic here
}, executorService);
In this snippet, we're using an ExecutorService
to manage thread pools efficiently. The newFixedThreadPool
sets a limit to the number of concurrent threads, reducing the risk of resource exhaustion.
3. Blocking Calls in Asynchronous Threads
Blocking calls in asynchronous contexts negate the benefits of asynchronous programming. Ensure that you do not perform heavy operations that block the thread.
Example
CompletableFuture.supplyAsync(() -> {
// This operation should be non-blocking
return getDataFromDatabase(); // Avoid blocking calls
});
If getDataFromDatabase
uses blocking I/O, replace it with a non-blocking alternative or handle it in a separate thread where blocking is acceptable.
Techniques to Avoid Async Pitfalls
1. Using Non-blocking I/O
One of the most effective ways to avoid pitfalls in asynchronous programming is to use non-blocking I/O whenever possible. Java's java.nio
package provides this capability.
Example
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(Paths.get("example.txt"), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
fileChannel.read(buffer, 0, null, new CompletionHandler<Integer, Void>() {
@Override
public void completed(Integer result, Void attachment) {
System.out.println("Read " + result + " bytes.");
}
@Override
public void failed(Throwable exc, Void attachment) {
System.err.println("Failed to read file: " + exc.getMessage());
}
});
In this example, we utilize an AsynchronousFileChannel
for reading files in a non-blocking way. This ensures that the application can continue processing other tasks without waiting for the file operation to complete.
2. Chaining CompletableFutures
Using thenApply()
, thenAccept()
, and similar methods allows you to chain tasks effectively while maintaining readability.
Example
CompletableFuture.supplyAsync(this::fetchData)
.thenApply(data -> processData(data))
.thenAccept(result -> System.out.println("Final Result: " + result));
This approach makes the code cleaner and easier to understand. It also helps in error handling as you can attach separate exception handling mechanisms to each stage.
3. Context Propagation
When using asynchronous programming, be cautious of losing context (such as security credentials or request information). Use constructs that allow you to propagate the context adequately.
Example
CompletableFuture.supplyAsync(() -> {
// Capture context
UserContext context = UserContext.current();
return processWithContext(context);
});
In this example, we assume that UserContext
contains information that needs to be passed along. Properly propagating context ensures your asynchronous tasks behave as expected.
4. Apply Backpressure in Reactive Programming
In scenarios where the output rate exceeds the consumption rate, implementing backpressure is crucial. Libraries like Project Reactor and RxJava offer built-in mechanisms for managing backpressure.
Example
Flux<Integer> flux = Flux.range(1, 100)
.onBackpressureBuffer(10); // Buffering excess items
flux.subscribe(System.out::println);
This ensures that your application does not overwhelm any consumers, thereby improving reliability.
When Things Go Wrong
Even with the best practices in place, things can still go wrong. Always keep logs and analytics in place to monitor performance and quickly spot errors. As emphasized in the article "Mastering Async/Await: Common Pitfalls to Avoid", recognizing problems before they escalate is vital.
Wrapping Up
Asynchronous programming offers substantial advantages, but it comes with its own set of challenges. By understanding common pitfalls and employing best practices, you can harness the power of asynchronous programming in Java effectively.
We recommend that you stay updated and continuously learn by exploring various resources, including the existing article on async programming pitfalls in Java programming. Remember, the key to success lies in understanding the 'why' and 'how', along with continuous implementation of best practices.
By mastering these concepts and techniques, you can build robust, efficient, and responsive applications that meet user expectations. Happy coding!