Avoiding Common Async Pitfalls in Java's CompletableFuture
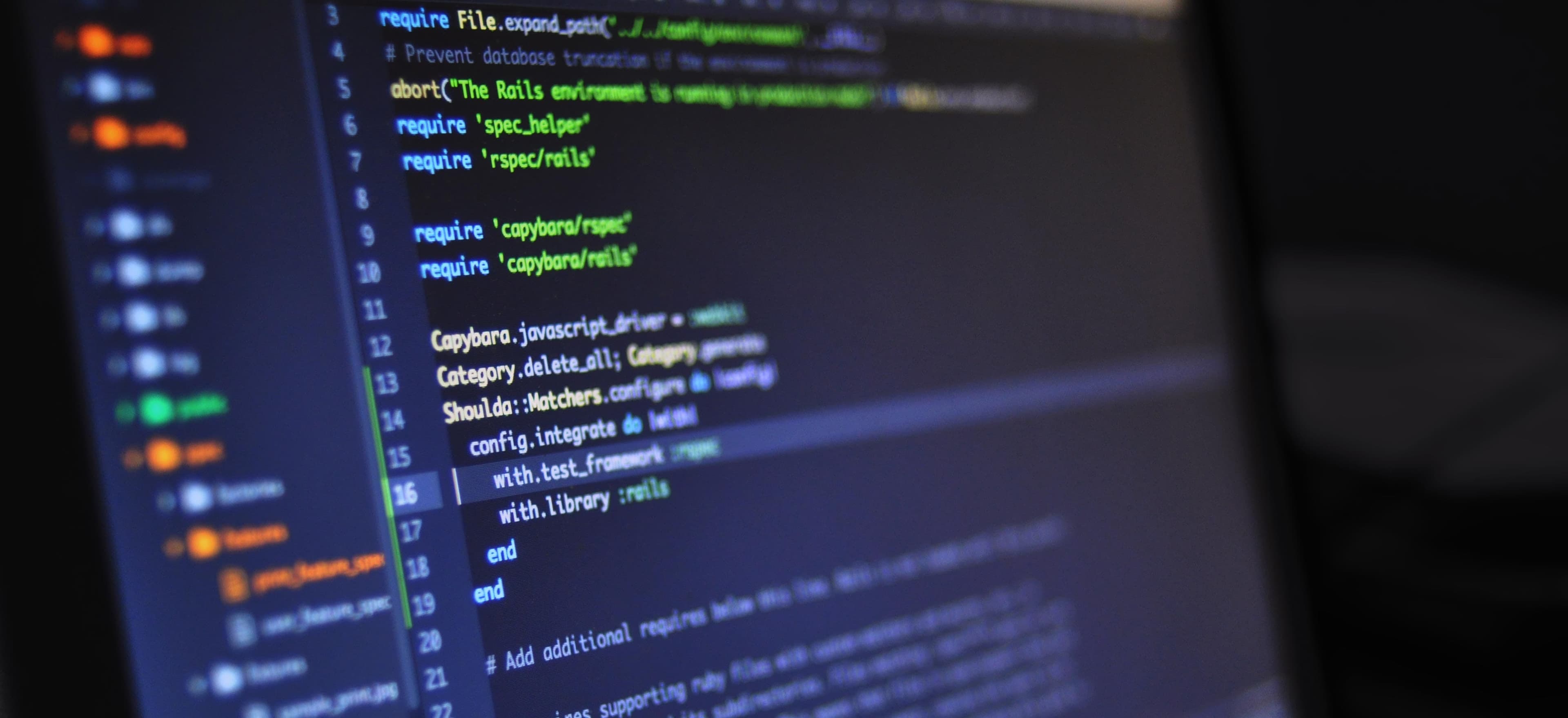
- Published on
Avoiding Common Async Pitfalls in Java's CompletableFuture
As software development evolves, the need for asynchronous programming has grown exponentially, especially in Java applications. With Java's CompletableFuture
, developers can write non-blocking code that is easier to read and maintain. However, there are common pitfalls that can arise when using this powerful feature. In this post, we will explore these pitfalls and provide effective strategies to avoid them, all while enhancing your understanding of asynchronous programming.
What is CompletableFuture?
Introduced in Java 8, CompletableFuture
provides a flexible way to write non-blocking asynchronous tasks. It allows you to compose multiple stages of computations and provides methods to handle results and exceptions efficiently.
Here is a basic example of using CompletableFuture
:
import java.util.concurrent.CompletableFuture;
public class BasicExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
return "Hello, World!";
});
future.thenAccept(result -> {
System.out.println(result); // Prints: Hello, World!
});
}
}
Commentary
In this example, supplyAsync
initiates an asynchronous task using a default executor. The subsequent thenAccept
method consumes the result once it becomes available. This approach prevents blocking the main thread, thus improving the responsiveness of your application.
Common Pitfalls in CompletableFuture
While the API is powerful, misusing CompletableFuture
can lead to issues such as:
- Not Handling Exceptions Properly
- Ignoring Thread Safety
- Creating Memory Leaks via Unbounded Chaining
- Not Using Timeouts
- Too Many Nested Compositions
Let's delve into these pitfalls in detail.
1. Not Handling Exceptions Properly
A significant pitfall is neglecting to handle exceptions in asynchronous tasks. By default, if the underlying computation throws an exception, it will be silently propagated. Consequently, it's vital always to attach an error handler.
Example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
if (true) throw new RuntimeException("Oops");
return "Success";
});
future
.exceptionally(ex -> {
System.out.println("Error: " + ex.getMessage());
return "Fallback";
})
.thenAccept(result -> System.out.println(result));
Commentary
In the example above, the exceptionally
method intercepts any exceptions thrown during the computation, allowing us to handle them gracefully. Without this, the exception would disrupt the flow, leading to unhandled exceptions.
2. Ignoring Thread Safety
Another common mistake is assuming that the code within a CompletableFuture
is safe regarding shared mutability. If you access shared objects without synchronization, it can lead to inconsistent state and race conditions.
Example:
public class ThreadSafety {
private static int count = 0;
public static void main(String[] args) {
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
count++;
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
count++;
});
CompletableFuture.allOf(future1, future2).join();
System.out.println(count); // Might print 0, 1, or 2
}
}
Commentary
In this example, multiple threads attempt to update the shared count
variable concurrently without synchronization. To avoid this, you could utilize atomic classes like AtomicInteger
or synchronize access when incrementing.
3. Creating Memory Leaks via Unbounded Chaining
When chaining multiple CompletableFuture
calls, be cautious of maintaining references to unnecessary intermediate results. If left unbounded, this can lead to memory leaks.
Example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Process")
.thenApply(result -> {
// Some processing
return "Processed " + result;
})
.thenCompose(result -> {
// More async work
return CompletableFuture.supplyAsync(() -> "Final output: " + result);
});
// This remains in memory until it's fully resolved or collected
future.thenAccept(System.out::println);
Commentary
In this case, each stage of processing holds a reference to the previous one. If the chain is extensive and not properly managed, you may end up consuming more memory than needed. Consider clearing references where appropriate or utilizing weak references if necessary.
4. Not Using Timeouts
Asynchronous tasks may sometimes hang indefinitely. Neglecting to set timeout values can lock up your application, leading to significant issues during runtime.
Example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
while (true) {} // Simulates a blocking call
});
// Timeout after 2 seconds
String result = future.orTimeout(2, TimeUnit.SECONDS)
.exceptionally(ex -> {
System.out.println("Timed out: " + ex.getMessage());
return "Fallback";
})
.join();
Commentary
The orTimeout
method allows you to specify a maximum duration for the completion of the future. If the task exceeds this duration, it will throw a TimeoutException
that can be handled gracefully.
5. Too Many Nested Compositions
While composing multiple futures, excessive nesting can lead to convoluted code that is hard to read and maintain. Aim for clarity.
Example:
CompletableFuture<String> result = CompletableFuture.supplyAsync(() -> "Hello")
.thenApply(greeting -> {
return greeting + ", World!";
}).thenCompose(finalGreeting -> {
return CompletableFuture.supplyAsync(() -> {
return finalGreeting + " Async!";
});
});
Commentary
While nested compositions can be concise, they can also reduce readability. Instead, consider breaking down complex logic into simpler methods or actions that flow more naturally.
In Conclusion, Here is What Matters
Mastering CompletableFuture
is a valuable skill for modern Java developers. By being aware of these common pitfalls and applying the discussed strategies, you can write cleaner, safer, and more efficient asynchronous code. If you want to deepen your understanding of asynchronous programming, make sure to check out the article Mastering Async/Await: Common Pitfalls to Avoid.
With practice, you will navigate the landscape of asynchronous programming with ease and confidence. Prioritize proper exception handling, ensure thread safety, manage memory effectively, implement timeouts, and keep your code clean. Happy coding!