Tackling Command Line Challenges with Java ProcessBuilder
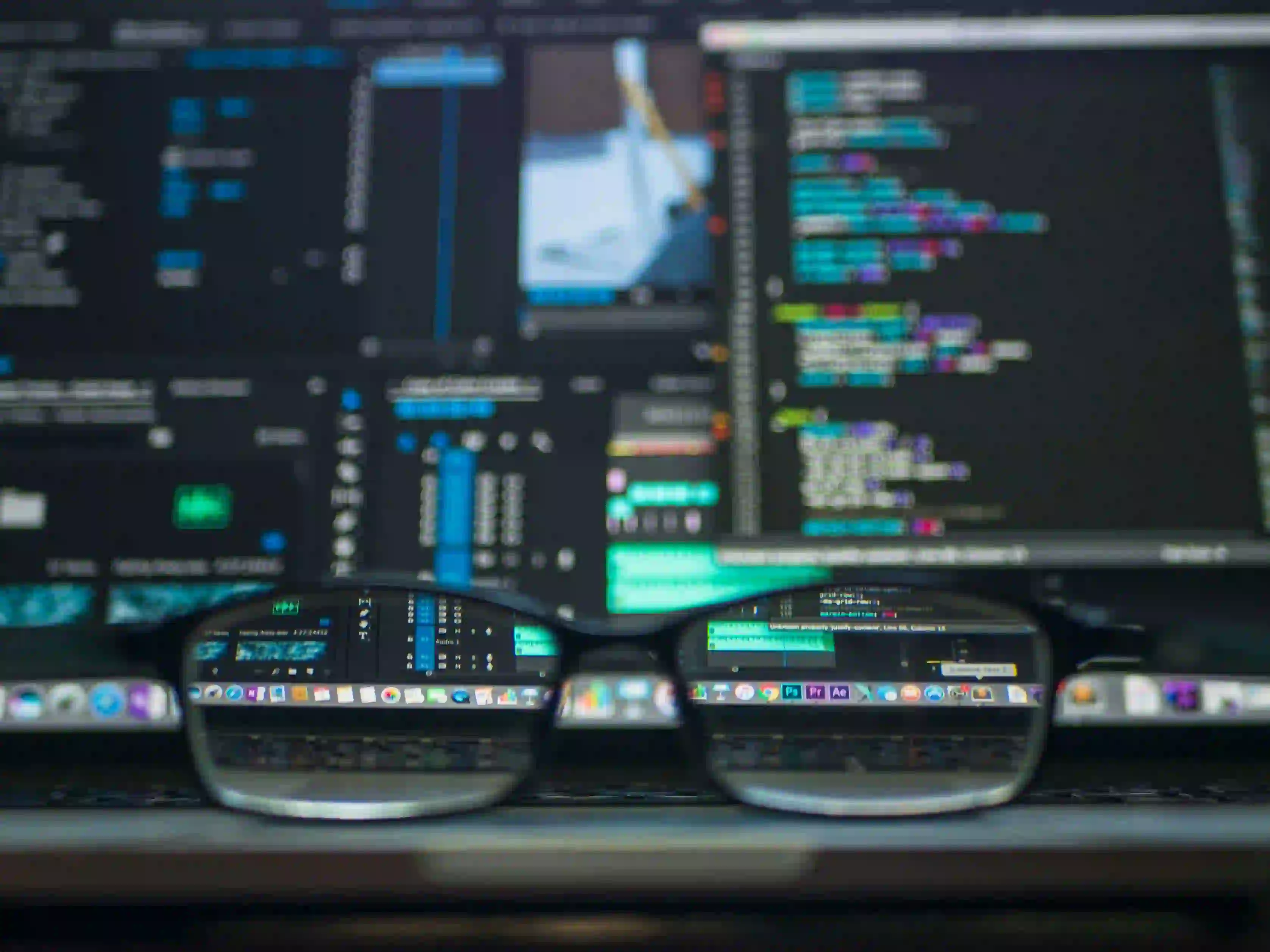
Tackling Command Line Challenges with Java ProcessBuilder
Java has long been a versatile programming language, celebrated for its multithreading capabilities, portability, and robust libraries. One of its lesser-discussed but equally powerful features is the ability to interact with the system command line through the ProcessBuilder
class. This article delves into how to effectively use ProcessBuilder
to tackle various command line challenges.
What is ProcessBuilder?
ProcessBuilder
is a class found in the java.lang
package. It is designed to create operating system processes in Java, facilitating the execution of external commands. This class not only allows you to run system commands as if you were typing them directly in the command line but also enables you to control input and output redirection, manage errors, and manipulate environment variables.
Why Use ProcessBuilder?
- Process Creation and Management: It allows you to spawn processes and control their input/output.
- Error Handling: Handle errors effectively by capturing standard error streams.
- Command-Line Arguments: Easily pass arguments to your commands.
- Environment Variables: Modify the environment variables for the spawned process.
Basic Usage of ProcessBuilder
Let’s start with a simple example of using ProcessBuilder
to run a shell command.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class SimpleProcess {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder();
// Command to be executed
processBuilder.command("echo", "Hello, World!");
try {
Process process = processBuilder.start();
StringBuilder output = new StringBuilder();
// Read the output from the command
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
output.append(line).append("\n");
}
}
// Wait for the process to finish
int exitCode = process.waitFor();
System.out.println("Output: " + output);
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Why This Code Works
- We instantiate
ProcessBuilder
and set up a command using thecommand
method. - The command lists in the form of strings, where "echo" is used to print "Hello, World!".
- We capture the output by reading the process's input stream.
- Finally, we wait for the process to terminate and print its exit code.
The exit code is important as it helps determine if the process was successful (an exit code of 0 generally means success).
Handling Command-Line Arguments
Using ProcessBuilder
, you can easily add command-line arguments to your commands.
public class CommandWithArgs {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder();
// Command with arguments
processBuilder.command("ls", "-l", "/path/to/directory");
try {
Process process = processBuilder.start();
StringBuilder output = new StringBuilder();
// Read the output from the command
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
output.append(line).append("\n");
}
}
// Wait for the process to finish
int exitCode = process.waitFor();
System.out.println("Output: " + output);
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Understanding Command-Line Arguments
Here, we call the ls
command with the -l
argument, which displays detailed directory contents for a specified path. The process receives the arguments as an array of strings, making it easy to build complex commands.
Redirecting Input and Output
In many situations, you may want to redirect the input or output of a command.
import java.io.File;
public class RedirectingOutput {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder();
// Command that generates output
processBuilder.command("ls", "-l");
// Redirect output to a file
processBuilder.redirectOutput(new File("output.txt"));
try {
Process process = processBuilder.start();
int exitCode = process.waitFor();
System.out.println("Process exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Why Redirecting Output is Useful
In this scenario, the output of the ls -l
command is redirected to a file named output.txt
. This is useful for logging outputs or storing data generated from command execution.
Error Handling
Error handling is vital when performing operations like running commands from your application. By capturing standard error, you can get detailed information about what went wrong.
public class ErrorHandlingExample {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder();
// Intentional error: Using a non-existent command
processBuilder.command("non-existent-command");
try {
Process process = processBuilder.start();
StringBuilder output = new StringBuilder();
StringBuilder error = new StringBuilder();
// Reading output stream
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
output.append(line).append("\n");
}
}
// Reading error stream
try (BufferedReader errorReader = new BufferedReader(new InputStreamReader(process.getErrorStream()))) {
String line;
while ((line = errorReader.readLine()) != null) {
error.append(line).append("\n");
}
}
int exitCode = process.waitFor();
System.out.println("Output: " + output);
System.out.println("Error: " + error);
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Error Capture Importance
Here we intentionally run a non-existent command to demonstrate how to capture error messages printed to the error stream. This can help diagnose issues during the execution.
Advanced Usage: Setting Environment Variables
You can also modify the environment variables for the command executed through ProcessBuilder
.
import java.util.Map;
public class EnvironmentVariablesExample {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder();
// Modify environment variable
Map<String, String> env = processBuilder.environment();
env.put("MY_VAR", "Value");
processBuilder.command("bash", "-c", "echo $MY_VAR");
try {
Process process = processBuilder.start();
StringBuilder output = new StringBuilder();
// read output
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
output.append(line).append("\n");
}
}
int exitCode = process.waitFor();
System.out.println("Output: " + output);
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
The Importance of Environment Variables
In this example, we set an environment variable MY_VAR
and then run a bash
command to print its value. This feature allows fine-tuned control over the execution context of your spawned processes.
Wrapping Up
ProcessBuilder
in Java provides a straightforward yet powerful way to tackle command line challenges. Its ability to manage processes, handle command-line arguments, redirect input/output, and set environment variables makes it an essential tool for any Java developer.
For those also interested in enhancing command line skills, I recommend checking out Mastering the Art of Nesting in Bash Commands for a deeper dive.
Hopefully, this guide serves as a springboard into the exciting capabilities of ProcessBuilder
, helping you bridge Java applications and system-level commands seamlessly.