Conquering Technical Debt for Sustained Product Success
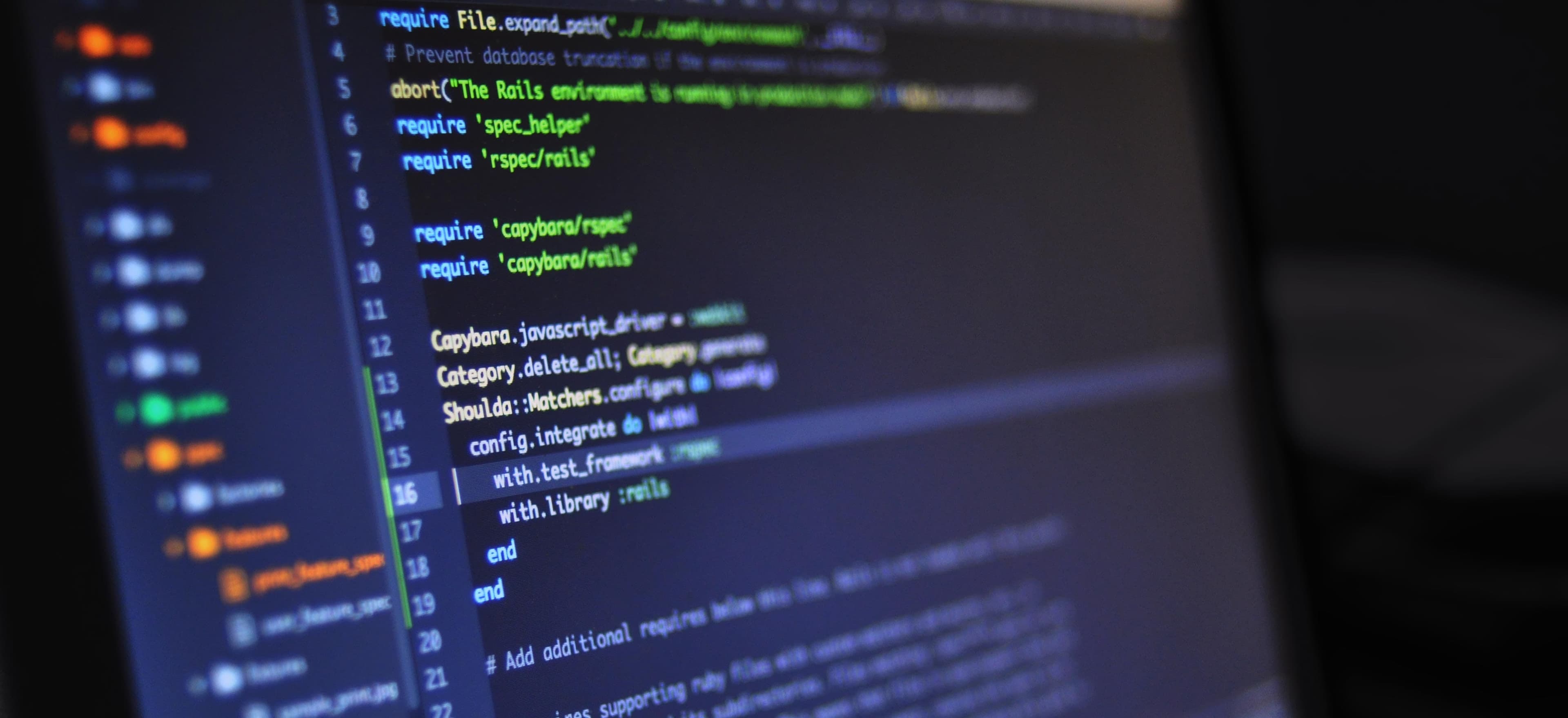
- Published on
Conquering Technical Debt for Sustained Product Success
In the world of software development, the term "technical debt" often surfaces in discussions about product health and longevity. While it may initially seem like a jargon term reserved for industry insiders, understanding and managing technical debt can significantly affect your product's success. This article will delve deeply into what technical debt is, its implications, and practical strategies to conquer it for sustained success.
What is Technical Debt?
Technical debt refers to the implicit cost of rework caused by choosing an easy solution now instead of a better approach that would take longer. It’s not merely bad code; it encompasses design flaws, outdated dependencies, and missed opportunities for optimization.
How Technical Debt Accumulates
Technical debt accumulates in various ways, including:
- Time pressure: Often, teams prioritize delivering features over writing clean code. This urgency leads to shortcuts, increasing long-term costs.
- Evolving requirements: As the project develops, initial designs may become obsolete, creating gaps that can only be rectified through considerable rework.
- Lack of testing: The failure to establish robust testing practices can escalate debt. When tests are absent, code becomes fragile and intertwined, making future changes risky.
Why Technical Debt Matters
It’s easy to dismiss technical debt, but neglecting it can have serious repercussions:
- Reduced Agility: High levels of technical debt hinder the software’s ability to adapt to new requirements.
- Increased Costs: The more technical debt accumulates, the more it costs to address later on. What might be a minor fix today could bloom into a major rewrite tomorrow.
- Diminished Quality: Poor-quality code can lead to bugs and system failures, damaging customer trust and satisfaction.
Measuring Technical Debt
Effective management begins with measurement. Here are several methods to assess your technical debt:
- Code Metrics: Utilize tools like SonarQube to evaluate code quality. These tools provide insights into code complexity, duplication, and vulnerabilities.
- Refactoring Costs: Calculate how long it would take to refactor a piece of code versus its benefit. This estimation requires a keen understanding of the codebase.
- Technical Debt Ratio (TDR): This ratio is defined as the cost to fix the issues in a project divided by the total development cost. This allows teams to visualize the long-term implications of current decisions.
Strategies for Conquering Technical Debt
With a clear understanding of technical debt and its consequences, let's explore actionable strategies for managing it.
1. Code Reviews
Regular code reviews are fundamental to preventing technical debt. Here's a simple code review checklist to get started:
- Adherence to Coding Standards: Are team coding standards followed?
- Complexity Reduction: Does the code solve the problem while minimizing complexity?
- Test Coverage: Are there adequate tests, and do they cover edge cases?
These practices not only catch debt in the making but also encourage knowledge sharing within the team.
2. Prioritize Debt Repayment
Just as you would with financial debt, prioritize which technical debts to pay down. You can categorize your debts in the following manner:
- High Impact, Low Effort: Pay these off quickly as they provide immediate benefits.
- High Impact, High Effort: Scope these out and allocate resources carefully.
- Low Impact: Consider if these debts need fixing at all, or if they can be deferred.
This method helps to systematically tackle the most crucial areas without becoming overwhelmed.
3. Refactoring
Refactoring is both an art and a science. It’s not just about rewriting code; it’s about making it more maintainable and scalable:
public class User {
private String name;
private String email;
// Constructor
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Instead of exposing fields directly, use getter methods
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
In the above code, we encapsulate user properties with getter methods. This encapsulation provides better control over how data is accessed and modified, minimizing vulnerabilities and allowing for easier future updates.
4. Automate Testing
Automated testing is one of the best defenses against technical debt. Implementing unit tests and integration tests as part of your development process ensures that new changes meet existing functionality without causing regression.
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class UserTest {
@Test
public void testUserCreation() {
User user = new User("John Doe", "john@example.com");
assertEquals("John Doe", user.getName());
assertEquals("john@example.com", user.getEmail());
}
}
This Unit Test ensures that our User class works as expected. Automated tests can quickly reveal if new code has inadvertently introduced bugs, which saves time down the road.
5. Invest in Continuous Learning
Technology evolves rapidly. Encourage your team to stay current with the latest programming paradigms, languages, and frameworks. This can be achieved through:
- Workshops
- Online Courses
- Webinars
Investing in continuous learning ensures that your team is equipped with the necessary skills to refactor and improve existing code.
6. Technical Debt Sprints
Similar to feature sprints, consider allocating dedicated sprints to manage technical debt. This focused effort allows teams to prioritize and address debt without the distraction of new feature development.
The Closing Argument
Technical debt is not inherently bad—it's an unavoidable aspect of software development. However, neglecting it can lead to significant long-term costs. By measuring, prioritizing, and systematically addressing technical debt, you pave the way for a more sustainable product.
Remember, conquering technical debt requires an ongoing commitment. By fostering a culture that values quality over speed, you can minimize technical debt's impact and ensure sustained product success.
For more insights into effective product management and software development, check out Martin Fowler’s Blog or Scrum.org for agile methodologies. Happy coding!