Nesting Shell Commands in Java: Handling Process Complexity
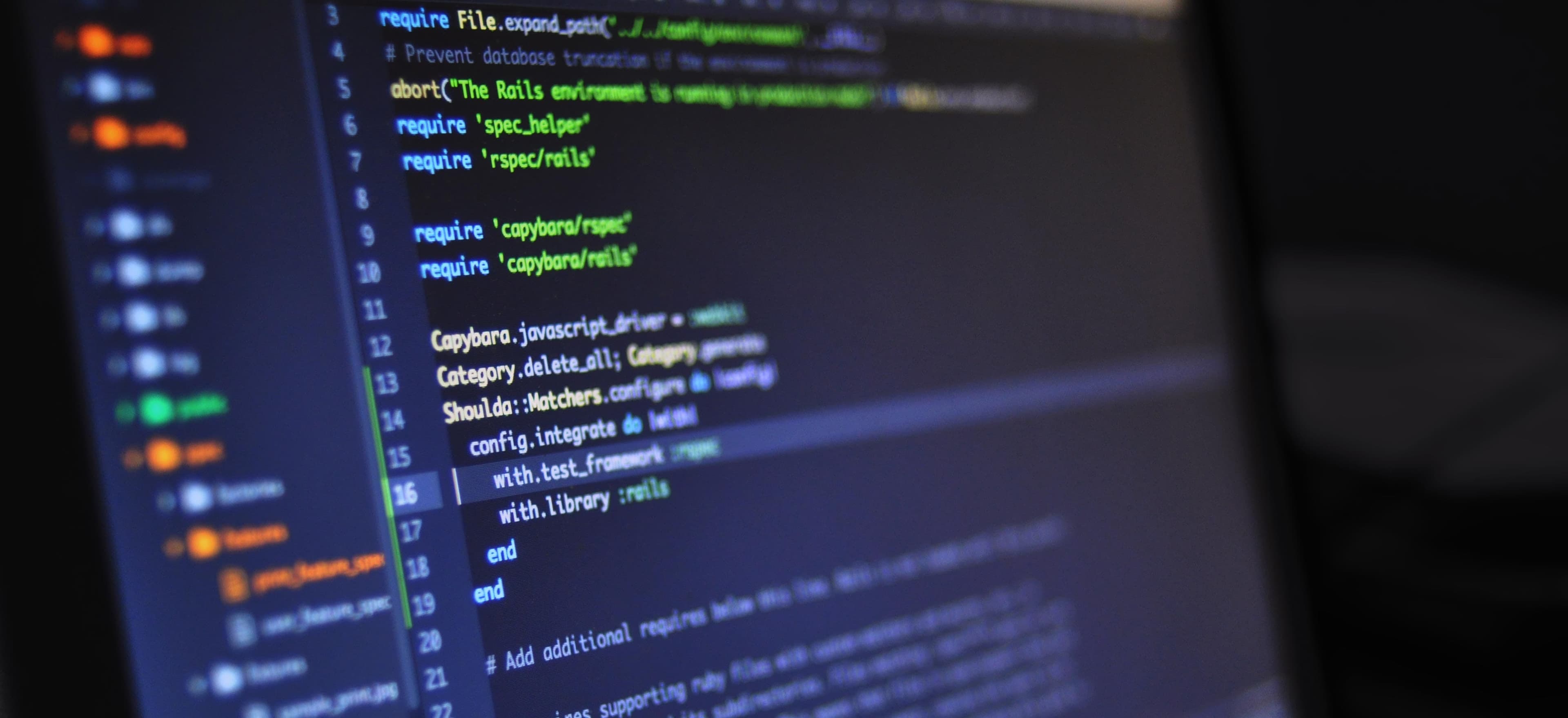
- Published on
Nesting Shell Commands in Java: Handling Process Complexity
In software development, the need to execute shell commands from a Java application is often essential. Whether launching system scripts, processing files, or automating system tasks, the ability to invoke shell commands directly from Java increases the versatility of your programs. This article will explore how to nest shell commands effectively within Java, providing you with code snippets, explanations, and best practices.
Table of Contents
- Understanding ProcessBuilder in Java
- Nesting Shell Commands
- Practical Examples
- Handling Outputs and Errors
- Best Practices
- Conclusion
Understanding ProcessBuilder in Java
Java provides a built-in way to execute operating system commands through the ProcessBuilder
class. This class grants fine control over the input and output streams and allows you to set environment variables and modify the working directory.
Here's a basic example:
ProcessBuilder builder = new ProcessBuilder("ls", "-l");
Process process = builder.start();
In this snippet, the ProcessBuilder
initiates a process to list files in long format. However, executing a single command is hardly enough for complex tasks.
Nesting Shell Commands
Nesting commands allows us to run more complex scripts without creating temporary files. Consider the following scenario where we want to find and count the number of .java
files in a directory:
String command = "find . -name '*.java' | wc -l";
ProcessBuilder builder = new ProcessBuilder("bash", "-c", command);
Process process = builder.start();
Explanation of the Above Code
- Command Breakdown:
find . -name '*.java'
searches for all files with the.java
extension.| wc -l
counts those files.
- Using Bash: The
bash -c
allows us to execute a string as a complex command. - Process Execution:
builder.start()
starts the execution of the provided command.
This command structure encapsulates the power of complex nesting in shell scripting directly inside Java.
Practical Examples
Example 1: Copying Files and Changing Permissions
Suppose we want to copy files from one directory to another and then change their permissions.
String command = "cp source/*.java destination/ && chmod 755 destination/*.java";
ProcessBuilder builder = new ProcessBuilder("bash", "-c", command);
Process process = builder.start();
In this snippet:
- We first copy all
.java
files from thesource
directory to thedestination
. - The
&&
ensures thatchmod
runs only if the copy was successful.
This chaining of commands minimizes the risk of left-behind files with improper permissions, and it flows cleanly into the script's logic.
Example 2: Combining Commands with Variables
You can manage command arguments using Java variables. Here's how you can achieve that:
String sourcePath = "source/*.java";
String destinationPath = "destination/";
String command = String.format("cp %s %s && chmod 755 %s", sourcePath, destinationPath, destinationPath + "*.java");
ProcessBuilder builder = new ProcessBuilder("bash", "-c", command);
Process process = builder.start();
Handling Outputs and Errors
Managing the output and error streams is vital. Java’s Process
object has methods to retrieve these streams. Below is how you can capture output:
String command = "find . -name '*.java' | wc -l";
ProcessBuilder builder = new ProcessBuilder("bash", "-c", command);
Process process = builder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
Explanation of Output Handling
- BufferedReader: Captures the output of the command.
- InputStream: By fetching the process input stream, you can print or process shell output.
When it comes to error handling, you can do this:
BufferedReader errorReader = new BufferedReader(new InputStreamReader(process.getErrorStream()));
while ((line = errorReader.readLine()) != null) {
System.err.println(line);
}
Best Practices
- Sanitize Input: Always sanitize input passed to shell commands to avoid command injection vulnerabilities.
- Use Full Paths: Specify full paths for commands and files to eliminate issues with environment paths.
- Avoid Shell for Simple Commands: If you can achieve your goal using Java's built-in libraries, prefer that over shell commands for better portability and security.
- Graceful Error Handling: Always read the process's error stream to provide feedback when something goes wrong.
- Refer to Resources: If you wish to dive deeper into nesting commands in different contexts, consider reading Mastering the Art of Nesting in Bash Commands.
The Bottom Line
Nesting shell commands within Java using ProcessBuilder
provides significant power and efficiency for automating complex scripting tasks. By following the principles outlined above and learning how to capture outputs effectively, you can enhance your application's capabilities. This mastery of nesting enables developers to handle multifaceted operations with relative ease, merging the worlds of Java and shell scripting harmoniously.
By understanding these strategies and code snippets, you're now better equipped to handle process complexity in your Java applications. Whether you're automating deployment tasks or managing system configurations, effective command handling can be a game changer. So dive in, experiment, and see what you can create!
Checkout our other articles