Handling Exceptions in Java 8 ForkJoinPool: A Guide
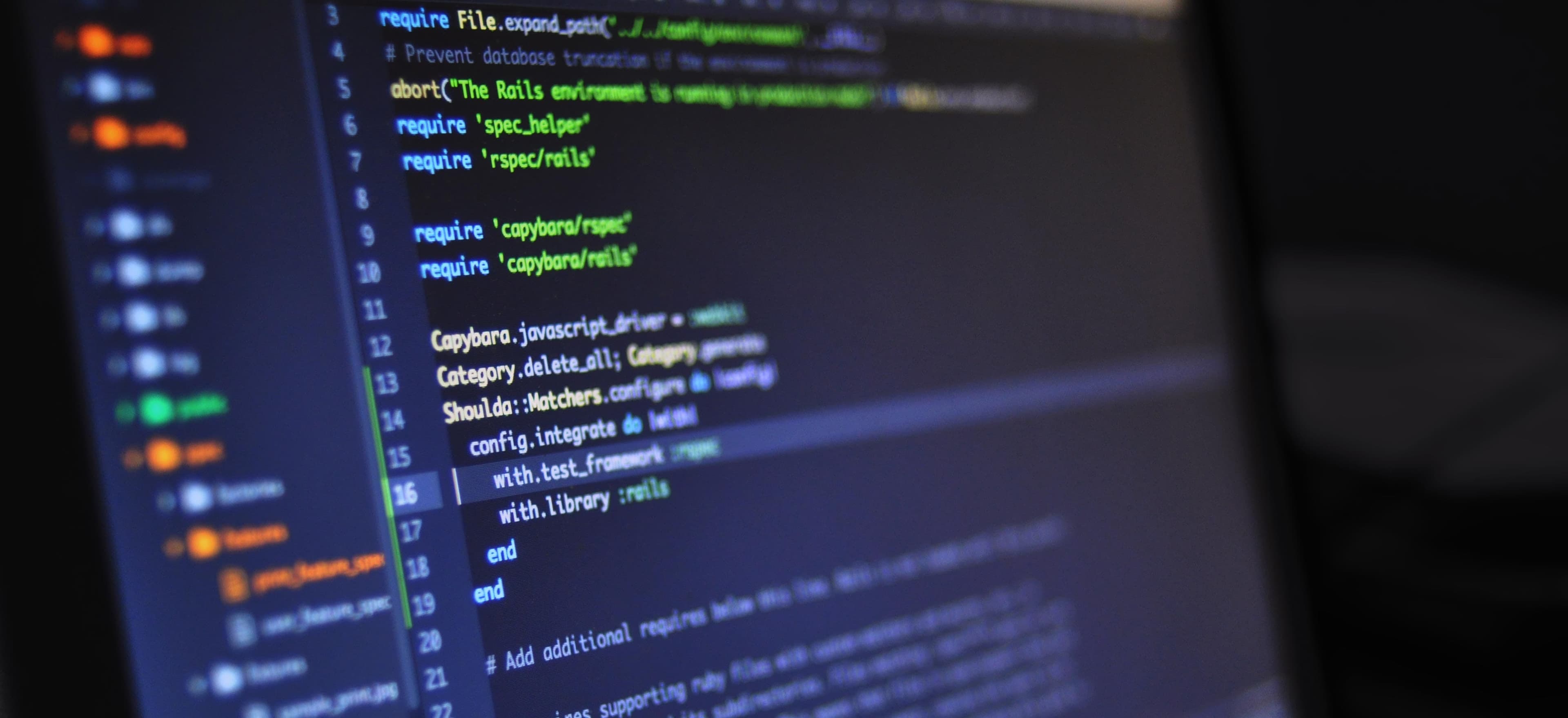
- Published on
Handling Exceptions in Java 8 ForkJoinPool: A Guide
Java has seen tremendous growth in concurrent programming since the introduction of the Fork/Join framework in Java 7. With the advent of Java 8, enhancements such as lambda expressions and Streams have simplified parallel processing tasks. However, handling exceptions in this model can be tricky due to the asynchronous nature of tasks. This blog post aims to provide a comprehensive guide on managing exceptions effectively in a ForkJoinPool
.
Understanding ForkJoinPool
The ForkJoinPool
is part of the Java concurrency framework designed for "divide and conquer" algorithms. It allows you to split a task into smaller subtasks, run them in parallel, and then combine the results. This is ideal for CPU-bound tasks such as mathematical computations or data processing.
Here's a basic example of using a ForkJoinPool
:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
class SumTask extends RecursiveTask<Integer> {
private final int start;
private final int end;
private final int[] data;
public SumTask(int[] data, int start, int end) {
this.data = data;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= 2) { // base case
return data[start] + (start + 1 < end ? data[start + 1] : 0);
}
int mid = (start + end) / 2;
SumTask leftTask = new SumTask(data, start, mid);
SumTask rightTask = new SumTask(data, mid, end);
leftTask.fork(); // asynchronously execute left task
return rightTask.compute() + leftTask.join(); // calculate right task and join results
}
}
public class ForkJoinExample {
public static void main(String[] args) {
int[] data = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(data, 0, data.length);
int result = pool.invoke(task);
System.out.println("Sum: " + result);
}
}
In this example, we define a SumTask
that recursively sums up an array of integers using a ForkJoinPool
.
The Challenge of Exceptions
One of the biggest challenges in parallel processing is dealing with exceptions. When working with ForkJoinPool
, exceptions thrown in a task do not propagate to the main thread like conventional calls. Instead, they are stored in a special ForkJoinTask
that must be explicitly handled. This means when a ForkJoinPool
task fails, it's essential to manage the error appropriately to ensure your application can recover gracefully.
Strategies for Exception Handling
1. Using ForkJoinTask.exceptionally
One straightforward way to handle exceptions is by using the exceptionally
method. This method allows you to provide a fallback result in case the task fails.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ForkJoinPool;
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
int[] data = {1, 2, 3, 4, 5};
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Task failed!");
}, pool);
future.exceptionally(ex -> {
System.out.println("Caught exception: " + ex.getMessage());
return 0; // return a default value
});
// Wait for the future to complete
future.join();
}
In this example, if the task fails, it catches the exception and logs a message, returning a default value instead of failing the program outright. The exceptionally
method is beneficial for handling exceptions in asynchronous tasks.
2. Custom Exception Handling
For more complex situations, you might want to create custom exception handling that either retries the task or performs some cleanup actions.
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
class SummingTask extends RecursiveTask<Integer> {
private final int[] data;
private final int start;
private final int end;
SummingTask(int[] data, int start, int end) {
this.data = data;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
try {
if (end - start <= 2) {
int sum = 0;
for (int i = start; i < end; i++) {
sum += data[i];
}
return sum;
}
int mid = (start + end) / 2;
SummingTask leftTask = new SummingTask(data, start, mid);
SummingTask rightTask = new SummingTask(data, mid, end);
leftTask.fork();
return rightTask.compute() + leftTask.join();
} catch (Exception e) {
System.err.println("Exception in task: " + e.getMessage());
return 0; // Return a default value in case of error
}
}
}
In the SummingTask
class, we wrap the computation inside a try-catch
block. This allows us to manage any exceptions that occur during the computation and log them, thereby preventing the whole task from failing. In such cases, returning a default value or performing an alternative action can keep your application stable and user-friendly.
3. Listener for Task Completion
One can also listen for completion status using ForkJoinTask
listeners. This allows for a structured approach to error handling after the task's execution.
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
int[] data = {1, 2, -3, 4, 5}; // negative number to trigger an exception
RecursiveTask<Integer> task = new RecursiveTask<Integer>() {
@Override
protected Integer compute() {
for (int value : data) {
if (value < 0) throw new RuntimeException("Negative value found.");
}
return 0; // or any valid operations
}
};
task.fork();
task.whenComplete((result, throwable) -> {
if (throwable != null) {
System.err.println("Error: " + throwable.getMessage());
} else {
System.out.println("Result: " + result);
}
});
task.join();
}
In this example, whenComplete
allows you to define behavior depending on whether the task completed successfully or not, offering a clearer separation of success and error handling.
The Last Word
Handling exceptions in Java 8's ForkJoinPool
can seem daunting, but with the right strategies in place, it's entirely manageable. From utilizing exceptionally
clauses for default responses to customizing exception processes and implementing task completion listeners, Java provides robust tools for efficient error management.
Understanding how to handle exceptions effectively will not only make your multi-threaded applications more reliable but also improve your overall programming skills in Java.
For further learning, you can check out the Java Concurrency Tutorial or dive deeper into the Fork/Join Framework documentation. Happy coding!
Checkout our other articles