Common Pitfalls in API Testing with RestAssured and Cucumber
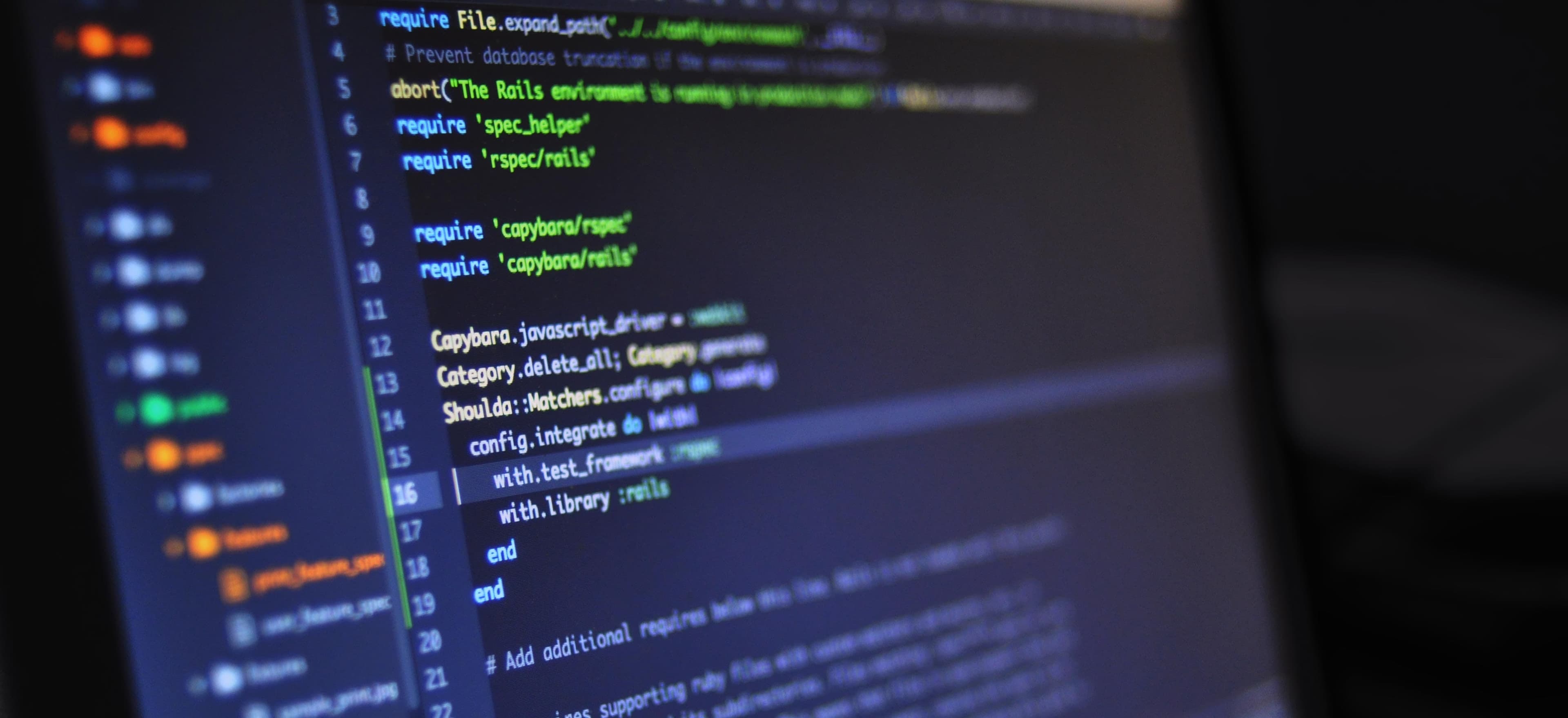
- Published on
Common Pitfalls in API Testing with RestAssured and Cucumber
API testing is a crucial phase in the software development lifecycle, ensuring that the interactions between the frontend and backend systems function seamlessly. With tools like RestAssured for API testing and Cucumber for behavior-driven development (BDD), it becomes easier to write structured and maintainable tests. However, there are common pitfalls that developers often encounter while using these powerful tools together.
In this blog post, we will explore these pitfalls, bolstering our discussion with clear code snippets and explanations. Whether you're a seasoned QA engineer or just starting with API testing, this guide will help you navigate these challenges effectively.
Understanding RestAssured and Cucumber
Before delving into the pitfalls, it is essential to understand what RestAssured and Cucumber offer:
-
RestAssured: A powerful Java-based library for testing REST services. It provides a domain-specific language (DSL) for writing clean and readable tests.
-
Cucumber: A testing framework that supports behavior-driven development, allowing you to write tests in human-readable language (Gherkin) which bridges the gap between non-technical stakeholders and developers.
By combining RestAssured with Cucumber, you can write API tests that are both understandable and maintainable. But with power comes responsibility and troubleshooting.
Common Pitfalls in API Testing
1. Ignoring HTTP Status Codes
One of the primary issues in API testing is neglecting to check the HTTP status codes returned by API endpoints. For instance, a successful API call should return a 200 status code. Forgetting to verify this could lead to false positives in your test results.
Code Example:
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
import org.junit.Test;
public class ApiTest {
@Test
public void testGetUser() {
given()
.pathParam("userId", 1)
.when()
.get("https://api.example.com/users/{userId}")
.then()
.statusCode(200) // Verify that the status code is 200
.body("name", equalTo("John Doe")); // Verifying other response data
}
}
In this code, we assert both the status code and response body. Each part matters; a 200 status code indicates the request was successful, while the body validates the content.
2. Hardcoding Values
A very common mistake is hardcoding values, leading to brittle tests that break easily. For example, using hardcoded URLs or response data can cause issues when the API changes.
Code Improvement: Instead of hardcoding the base URL or user name, consider externalizing these values in a configuration file.
Config Properties File (config.properties
):
base.url=https://api.example.com
default.user=John Doe
Code Example:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class Config {
private static Properties properties = new Properties();
static {
try {
FileInputStream input = new FileInputStream("config.properties");
properties.load(input);
} catch (IOException e) {
e.printStackTrace();
}
}
public static String getBaseUrl() {
return properties.getProperty("base.url");
}
public static String getDefaultUser() {
return properties.getProperty("default.user");
}
}
With the configuration handled separately, any change in the API can easily be managed without updating test code directly.
3. Not Utilizing Tags Effectively in Cucumber
Cucumber allows you to group scenarios with tags, and failing to use them can lead to running unnecessary tests and longer build times.
Example:
@smoke
Scenario: Verify user retrieval
Given I have a user with ID 1
When I request the user
Then I should receive a 200 status code
In your test execution commands, you can specify @smoke
to run only smoke tests, speeding up your feedback loop.
4. Overlooking Negative Testing
It is equally important to test invalid scenarios. Limiting tests to only valid conditions can paint an unrealistically positive picture of your API’s reliability.
Example Code for Negative Testing:
@Test
public void testInvalidUserRetrieval() {
given()
.pathParam("userId", 9999) // Non-existing user ID
.when()
.get("https://api.example.com/users/{userId}")
.then()
.statusCode(404); // Expecting a 404 status code for invalid user
}
In this case, you assert that attempting to retrieve a non-existent user results in a 404 status code. This ensures your API behaves correctly under unexpected conditions.
5. Not Validating Response Time
APIs should not only return valid responses but also do so within a reasonable time frame. Failure to check this could lead to performance issues in production.
Code Snippet:
@Test
public void testResponseTime() {
given()
.pathParam("userId", 1)
.when()
.get("https://api.example.com/users/{userId}")
.then()
.time(lessThan(2000L)); // Expecting the response time to be less than 2000 milliseconds
}
In this snippet, we verify that the response time is less than 2000 milliseconds. Monitoring performance can save you from scalability issues later on.
6. Lack of Detailed Error Handling
Error handling, especially in tests, is often overlooked. Ignoring potential exceptions can lead to either silent failures or misleading results.
@Test
public void testUserCreation() {
try {
given()
.contentType("application/json")
.body("{\"name\":\"John Doe\"}")
.when()
.post("https://api.example.com/users")
.then()
.statusCode(201);
} catch (Exception e) {
// Better reporting of the failure
System.out.println("Exception occurred: " + e.getMessage());
}
}
This code gracefully handles exceptions and logs them, allowing for clearer debugging when tests fail.
7. Neglecting to Use Assertions Wisely
Finally, assertions are your best friend. Using vague assertions can lead to uncertain test outcomes. Always be precise in what you're validating.
Example: Using more meaningful assertions enhances clarity.
.then()
.body("name", equalTo("John Doe"))
.body("age", greaterThan(25));
In this example, we not only check that the name is correct but also that the age is greater than a certain threshold, providing more context.
Closing the Chapter
By being aware of these common pitfalls while using RestAssured and Cucumber for API testing, you can create more robust, reliable, and maintainable tests. Implementing best practices, such as validating HTTP status codes, externalizing configuration, using tags, incorporating negative testing, and monitoring response times, will significantly enhance your testing process.
API testing is not just about the happy path; it’s about ensuring your application behaves as expected under all scenarios. By effectively utilizing the capabilities of RestAssured and Cucumber, you can ensure a smoother development and deployment cycle.
For more detailed insights on API testing techniques and strategies, consider reading these comprehensive guides or exploring the official RestAssured documentation. Keep learning and evolving your API testing practices, and you will reap the benefits in quality and efficiency.
Happy testing!
Checkout our other articles