Common Mistakes in Using FindBugs with Maven
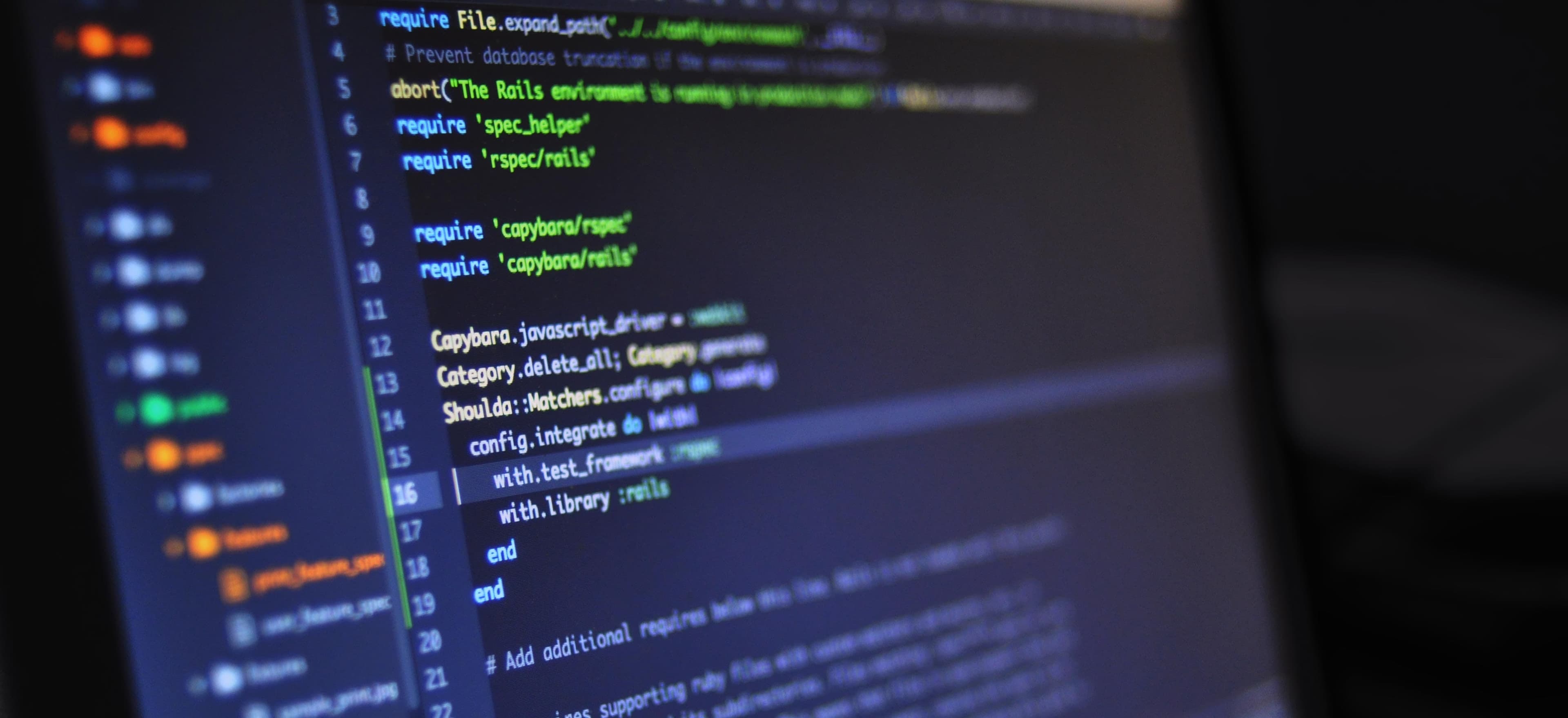
- Published on
Common Mistakes in Using FindBugs with Maven
As the software development ecosystem keeps evolving, ensuring the quality of code remains a pivotal concern. One notable tool in the Java developer's arsenal is FindBugs, a static analysis tool that identifies potential bugs in Java code. When integrated with Maven, a popular build automation tool, FindBugs helps streamline the code analysis process. However, new users often encounter pitfalls that can lead to inefficiencies or even misinterpretations of the analysis results. This blog post delves into common mistakes while using FindBugs with Maven and how to effectively leverage the tool for cleaner code.
What is FindBugs?
FindBugs is a static analysis tool that scans Java bytecode for potential defects. It uses a set of bug patterns that identify common issues such as null pointer dereferences, infinite recursive calls, or resource leaks. The tool can be integrated into a development workflow to provide developers with real-time feedback on code quality.
For more information about FindBugs, check FindBugs GitHub repository.
Setting Up FindBugs with Maven
Before diving into common mistakes, let us first establish how to set up FindBugs with Maven in a Java project. The configuration usually involves adding the FindBugs plugin to the pom.xml
file.
Example Configuration
Here’s a basic setup for adding FindBugs to your Maven project:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>findbugs-maven-plugin</artifactId>
<version>3.0.5</version>
<configuration>
<failOnError>true</failOnError>
<xmlOutput>true</xmlOutput>
<outputDirectory>${project.build.directory}/findbugs</outputDirectory>
</configuration>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Explanation of the Code
- plugin: Defines the FindBugs plugin with the necessary configurations.
- failOnError: When set to true, this parameter ensures the build fails if FindBugs detects serious issues, enforcing better standards.
- xmlOutput: This configuration enables the generation of an XML report, which can be useful for further analysis.
- outputDirectory: Specifies where the results will be stored after the analysis.
By integrating this configuration into your Maven build, you set up a foundation for identifying bugs in your project. However, even with proper setup, developers make mistakes that can compromise the effectiveness of FindBugs.
Common Mistakes
1. Not Running FindBugs Regularly
Mistake Explanation
One of the most frequent errors is neglecting to run FindBugs regularly. Many developers only run static analysis during major releases, which can result in a backlog of issues.
Solution
Incorporate FindBugs into your continuous integration (CI) pipeline. This practice not only ensures that code quality is monitored continuously but also helps future-proof your code. You can effortlessly run the analysis with your build commands:
mvn clean install
2. Ignoring Findings
Mistake Explanation
Another prevalent mistake is overlooking the suggested bug patterns. Many developers might falsely believe some warnings are not relevant to their codebase.
Solution
Take the time to understand each warning generated by FindBugs. Review the FindBugs Bug Descriptions for more context on specific warnings. In some cases, you might need to act on them, while in others, you can safely suppress specific warnings after thoughtful consideration.
3. Misconfiguring the Plugin
Mistake Explanation
Misconfiguration of the FindBugs plugin in the pom.xml
often leads to malformed reports or even inaccuracies in analysis results.
Solution
Ensure your configuration parameters are accurate. Verify the Maven version compatibility with the FindBugs plugin and adjust configurations as per the official FindBugs documentation.
4. Neglecting Custom Rule Sets
Mistake Explanation
FindBugs comes with a predefined set of bug patterns, but overlooking custom rules can hinder your ability to spot issues unique to your codebase.
Solution
Consider creating custom rule sets to address specific concerns in your applications. Here’s how you can include a custom rule set in your Maven configuration:
<configuration>
<excludeFilterFile>path/to/exclude.xml</excludeFilterFile>
<includeFilterFile>path/to/include.xml</includeFilterFile>
</configuration>
This setup allows you to tailor the analysis to match your team's unique coding standards.
5. Not Analyzing Third-Party Libraries
Mistake Explanation
Many developers limit their analysis to their code, overlooking potential Issues in third-party libraries. This oversight can lead to vulnerabilities in the application.
Solution
Include third-party libraries in your FindBugs analysis. Use the dependency:analyze goal to identify issues with transitive dependencies:
mvn dependency:analyze
Also, consider using OWASP Dependency-Check to identify known vulnerabilities in these libraries.
6. Failing to Update FindBugs
Mistake Explanation
Using an outdated version of FindBugs can result in missed out improvements and newer bug detection patterns. Old might not have the latest bug patterns to identify modern coding issues.
Solution
Remind your team to regularly check for updates and modify the version
tag in your pom.xml
file accordingly. The latest versions often come with bug patterns
improvements and bug fixes.
7. Skipping Documentation for Findings
Mistake Explanation
Certain teams undermine the importance of documenting code-quality issues highlighted by FindBugs. This practice can lead to inconsistent understanding and resolution of problems.
Solution
Create a tracking system to log all critical issues found by FindBugs. This can include details about the issues, responsible teams, and timelines for resolution. This process not only improves accountability but also fosters a culture of quality in the team.
Lessons Learned
Integrating FindBugs with Maven is a powerful way to enhance the quality of your Java applications. However, developers must remain vigilant in how they use this tool to ensure they avoid common pitfalls. By utilizing the insights provided by FindBugs, configuring the plugin effectively, and maintaining up-to-date practices, you can significantly strengthen your codebase and reduce the likelihood of defects.
For further reading on Java static analysis, you might also explore tools like SonarQube or PMD, which provide additional layers of code quality insights.
By addressing these common mistakes and following the guidelines suggested in this article, you can leverage FindBugs effectively to maintain clean, high-quality Java code. Happy coding!
Checkout our other articles