Overcoming Common Challenges in Multilingual Development
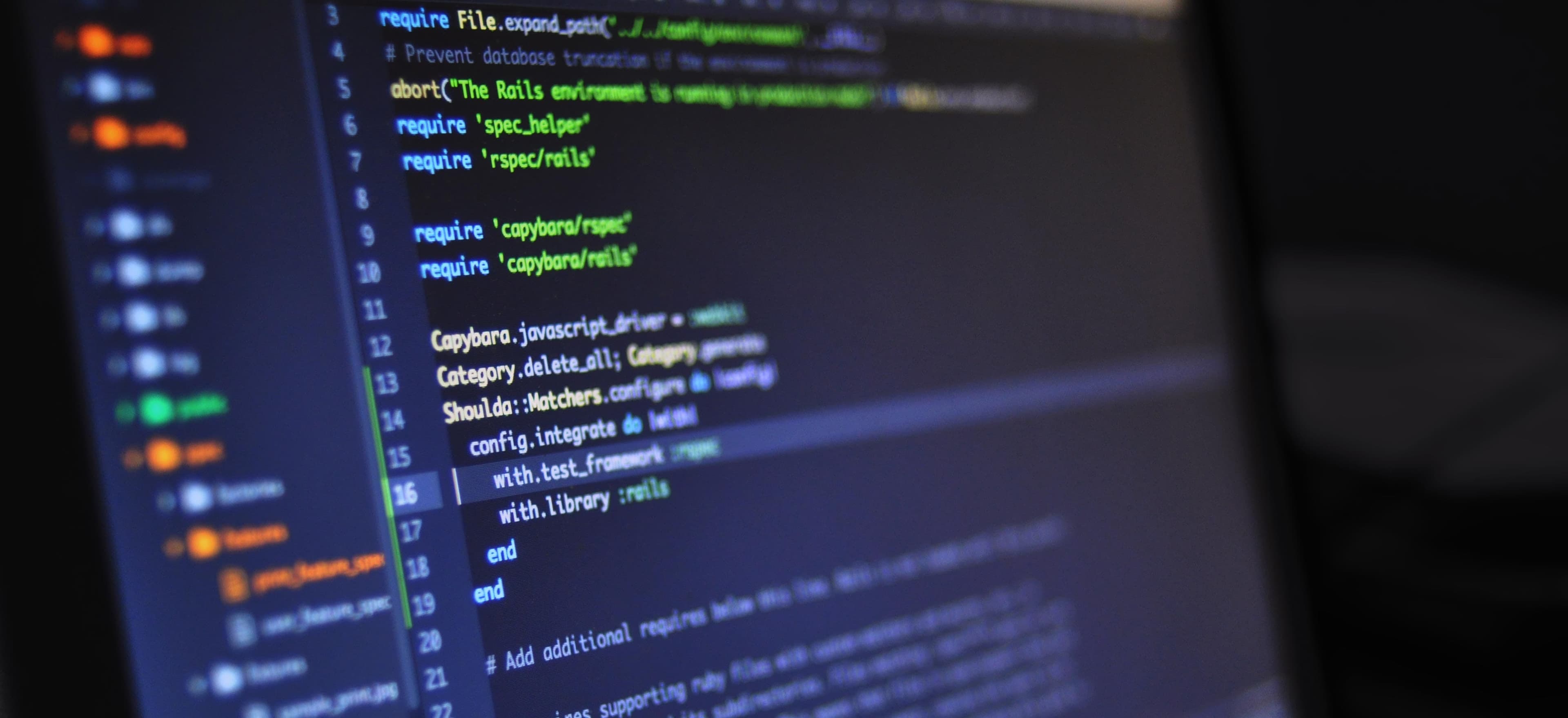
- Published on
Overcoming Common Challenges in Multilingual Development
In an increasingly global market, creating applications that can cater to users across different languages is essential. However, multilingual development comes with its own set of challenges. This blog post will provide insights into common hurdles faced during multilingual development, along with practical solutions and code snippets to overcome them.
Understanding Multilingual Development
Multilingual development involves more than just translating text; it requires accommodating different cultural contexts, regional preferences, and varying technical responsibilities. A well-implemented multilingual application can enhance user experience and broaden reach across diverse demographics.
Common Challenges in Multilingual Development
-
Language Translation
The most evident challenge is accurately translating application content into multiple languages. This involves not just conversion of words but also understanding the nuances of each language. -
Cultural Context
Different cultures can interpret the same information in various ways. It is important to localize content beyond mere words to ensure usability across cultures. -
Text Direction and Formatting
Languages such as Arabic and Hebrew read from right to left, presenting layout challenges. Similarly, certain languages may require different date formats, currency symbols, and numeric systems. -
Dynamic Content
Applications often contain dynamic content that needs to be translated in real-time, making it hard to maintain consistency. -
Testing and Quality Assurance
Ensuring that an application works seamlessly across all languages requires extensive testing, which can be time-consuming and expensive.
Approaches to Overcome Challenges
1. Use of Localization Libraries
Using appropriate localization libraries can significantly ease the translation process. Java, being a powerful platform, offers various libraries like Java ResourceBundle to manage different language resources.
Example Code Snippet
import java.util.Locale;
import java.util.ResourceBundle;
public class MultilingualApp {
public static void main(String[] args) {
Locale currentLocale = new Locale("fr", "FR"); // French - France
ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", currentLocale);
System.out.println(messages.getString("greeting")); // Outputs 'Bonjour'
}
}
Why This Works:
ResourceBundles allow you to store strings in key-value pairs for different languages. By specifying the locale, you can dynamically fetch the right translation based on the user's language preference.
2. Implementing a Localization Strategy
A strategic approach is necessary for ensuring a smooth multilingual experience. Create guides for translators, define terminology, and provide a glossary of terms. These resources can mitigate misunderstandings and ensure consistency across translations.
3. Handling Cultural Context
Understanding cultural nuances is key in localization. For example, colors may have different meanings in various cultures. Different cultures also have varying practices regarding images, symbols, and colors.
Code Snippet for Date Formatting
Using Java's SimpleDateFormat
, you can format dates according to user locale.
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class DateFormattingExample {
public static void main(String[] args) {
Locale locale = Locale.GERMANY; // German locale
SimpleDateFormat format = new SimpleDateFormat("dd.MM.yyyy", locale);
System.out.println("Date in Germany format: " + format.format(new Date()));
}
}
Why This Works:
This code formats the date according to the German conventions, which is critical in providing a localized user experience.
4. Dynamic and Contextual Content
Dynamic content requires a different handling approach. Consider using a translation management system (TMS) that can automatically detect changes and facilitate continuous localization.
5. Comprehensive Testing
Unit tests and automated tests should check for translations, context, and cultural appropriateness. Ensure that your QA team includes members from different cultural backgrounds to cover all angles. Tools like Selenium can assist in testing multilingual applications.
@Test
public void testGreetingTranslation() {
Locale.setDefault(new Locale("es", "ES")); // Spanish - Spain
ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle");
assertEquals("Hola", messages.getString("greeting")); // Check if Spanish greeting is 'Hola'
}
Why This Matters:
Testing ensures your translations are accurate and contextually appropriate. Automated tests can help save time and reduce human error.
Best Practices for Multilingual Development
-
Maintain Separate Resource Files: Different languages should have their own resource files (e.g., MessagesBundle_en.properties, MessagesBundle_fr.properties) to streamline updates.
-
Leverage Community Translation: Consider crowdsourcing translations to tap into native speakers who can provide more culturally relevant translations.
-
Regular Updates: Regularly update your translations to keep them in line with changes in the codebase and content.
-
Integrate real-time translation APIs: Tools such as Google Translate API can help in fetching dynamic content translations on the fly.
The Last Word
Navigating the complexities of multilingual development is no easy feat, but with the right strategies, tools, and awareness of cultural sensitivities, it can be effectively mastered. Whether you are working on a small Django application or a large-scale enterprise solution, quitting is not an option. Embrace these challenges as opportunities to reach a broader audience.
For more in-depth knowledge on localization techniques, check out Internationalization and Localization in Java and Content Localization Best Practices.
By following these tips and being proactive in addressing challenges, you can successfully develop multilingual applications that resonate with users worldwide. Remember, the goal is not just to translate, but to communicate effectively, irrespective of language.