Top Challenges Users Face with Selenium 4 Features
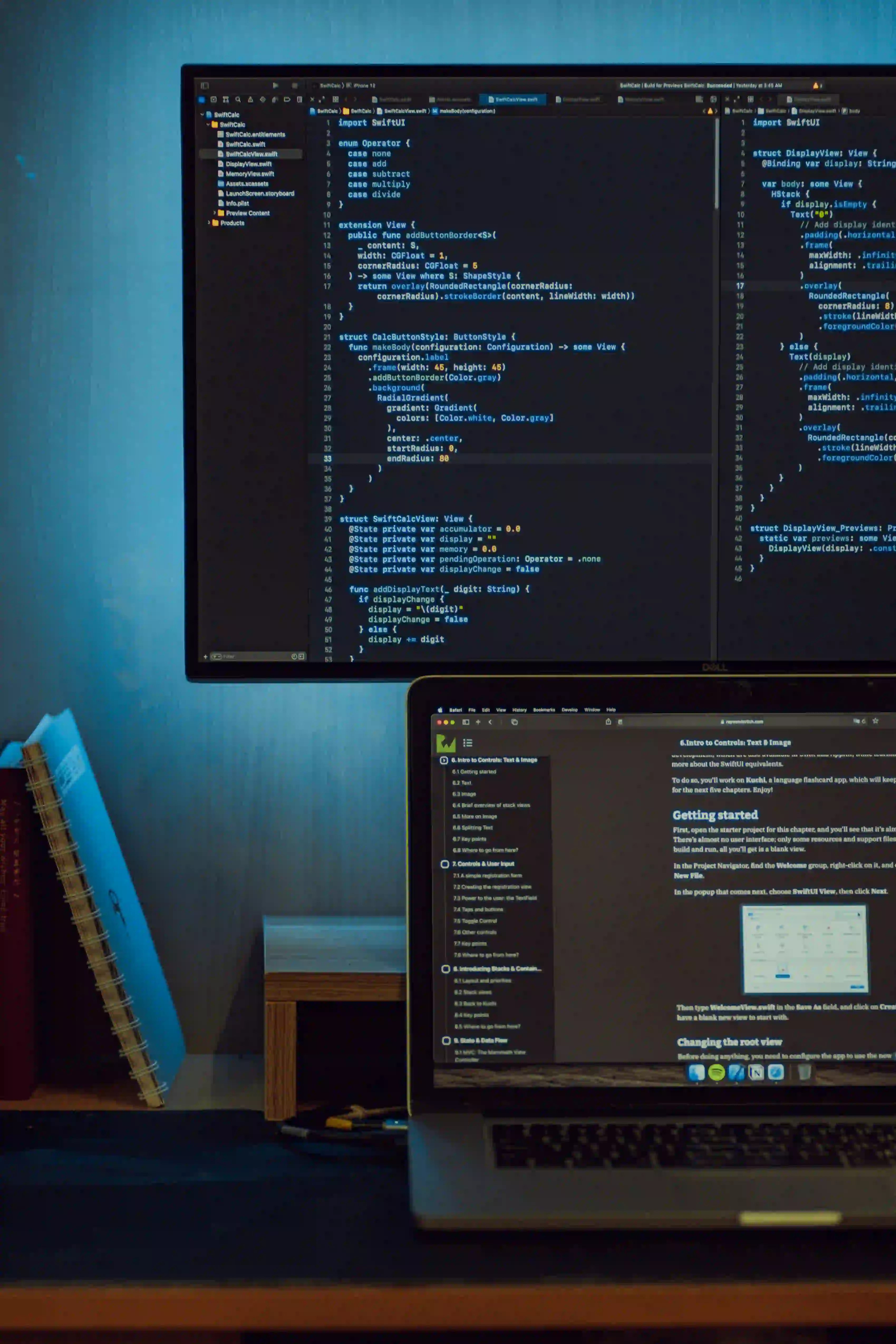
Top Challenges Users Face with Selenium 4 Features
Selenium has long been a go-to tool for web automation. With the advent of Selenium 4, the framework introduced several new features that excited developers and testers alike. However, every new release comes with its own set of challenges. In this blog post, we will delve into the top difficulties users encounter with Selenium 4 features.
A Quick Look to Selenium 4
Selenium 4 has made significant strides in improving the way developers interact with web browsers. Enhanced features such as native events, a full W3C-compliant WebDriver protocol, better support for modern web applications, and improved API for handling timeouts and waits have made it a robust option for automated testing. However, these advantages also introduce complexities that can trip up users.
Challenge 1: W3C Compliance
What's New?
The move to a fully W3C-compliant WebDriver is one of the most significant changes in Selenium 4. This means that developers need to adjust their existing codebase to align with the new standards.
Why Is This a Problem?
If you were accustomed to working in the earlier versions of Selenium, adapting to this shift can be cumbersome. For instance, the way sessions are created and maintained has undergone changes. Here’s a basic example that shows how to start a session in Selenium 3 versus Selenium 4.
// Selenium 3 Example
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Selenium 4 Example
ChromeOptions options = new ChromeOptions();
WebDriver driver = new RemoteWebDriver(new URL("<remote-url>"), options);
driver.get("http://example.com");
In the Selenium 4 example, the addition of RemoteWebDriver
comes into play, especially for remote testing. The transition can cause significant overhead in rewriting existing test scripts.
Challenge 2: Deprecation of Features
What's New?
Selenium 4 has deprecated several features from the previous versions. While these changes are often intended for streamlining the API, they can lead to confusion.
Why Is This a Problem?
Features like DesiredCapabilities
have been replaced by more specific options classes like ChromeOptions
, FirefoxOptions
, etc. This forces users to rethink their automation scripts. A straightforward code snippet illustrates this:
// Selenium 3 Example
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
capabilities.setCapability("someCapability", true);
WebDriver driver = new RemoteWebDriver(new URL("<remote-url>"), capabilities);
// Selenium 4 Example
ChromeOptions options = new ChromeOptions();
options.setCapability("someCapability", true);
WebDriver driver = new RemoteWebDriver(new URL("<remote-url>"), options);
Such changes require users to thoroughly review and modify their code, leading to increased development time and potential for errors.
Challenge 3: Improved Native Events
What's New?
Selenium 4 introduced native events to improve the interaction between the WebDriver and browser. Native events allow for more realistic interactions, simulating user actions more accurately.
Why Is This a Problem?
While the benefits are clear, handling native events requires a deeper understanding of how these events work. For example, if an element is covered by another element in the DOM, the click might not register, causing tests to fail.
// Click using native events
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
actions.moveToElement(targetElement).click().perform();
Users may find themselves debugging issues that didn’t exist in previous versions where Selenium relied on JavaScript click events. This can lead to frustrating setbacks if the underlying HTML structure changes, leading to further complications.
Challenge 4: Context-Sensitive Actions
What's New?
Selenium 4 supports context-sensitive actions that depend on the state of the application. The way you implement these changes can be quite different compared to previous versions.
Why Is This a Problem?
As a user, you will need to be aware of the application’s state to execute actions like dragging and dropping, resizing elements, or performing multiple actions simultaneously. Here is how a drag-and-drop function could look in Selenium 4.
// Selenium 4 Drag and Drop
WebElement sourceElement = driver.findElement(By.id("source"));
WebElement targetElement = driver.findElement(By.id("target"));
Actions actions = new Actions(driver);
actions.clickAndHold(sourceElement)
.moveToElement(targetElement)
.release()
.build()
.perform();
Users may not be accustomed to managing states actively, and such tasks can lead to difficulties in implementing automated test cases.
Challenge 5: Handling Waits
What's New?
Selenium 4 refined how developers handle implicit and explicit waits. This ensures that scripts can run more consistently in dynamic environments.
Why Is This a Problem?
For users who have been leveraging implicit waits, the transition to managing waits can be perplexing, particularly as improper use can lead to flaky tests.
Consider the difference in handling waits:
// Selenium 3 Implicit Wait
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Selenium 4 Improved Handling
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOf(element));
While the explicit wait approach in Selenium 4 provides more control, it also requires users to rethink their strategies for waiting and add more lines of code, which might be overwhelming.
Key Takeaways
While Selenium 4 has introduced several innovations aimed at improving automated web tests, these come with their own set of challenges. From adjusting to W3C compliance to managing native events and handling waits, users need to adapt to a new paradigm of automation testing.
What’s Next?
Users upgrading to Selenium 4 should invest some time in training or practical experimentation to overhaul existing frameworks and take full advantage of the benefits offered. Consider checking out Selenium's official documentation for an in-depth understanding or this guide on upgrading to Selenium 4 to ease the transition.
Understanding and overcoming these challenges will pave the way for seamless automation and improved test robustness. Happy testing!