Maximize Efficiency: Tackling ThreadPoolExecutor Overhead
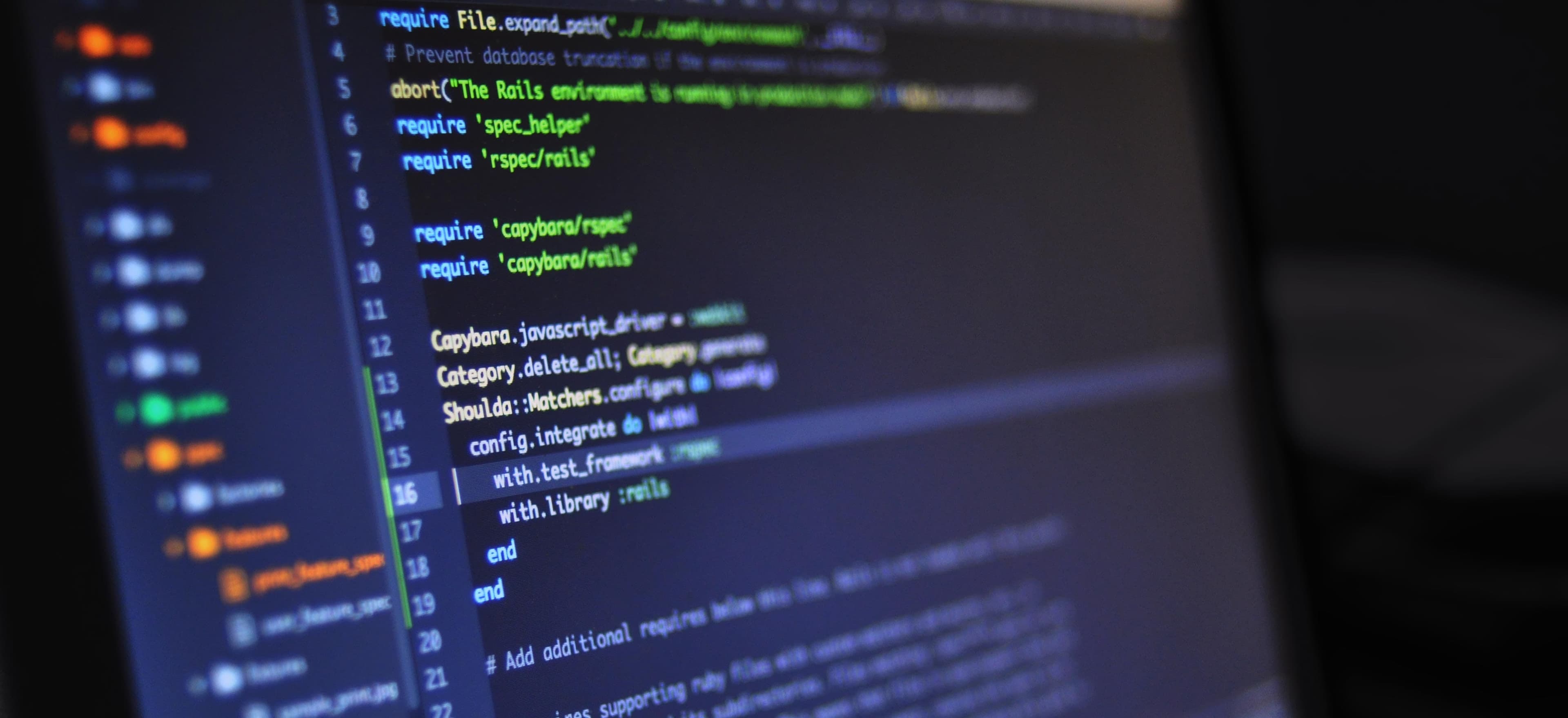
- Published on
Maximize Efficiency: Tackling ThreadPoolExecutor Overhead
In today's fast-paced software landscape, optimizing for performance is crucial. This is particularly true when working with concurrent programming in Java. The ThreadPoolExecutor
class is a vital utility from the java.util.concurrent
package that enables you to manage a pool of threads efficiently. However, improper usage can lead to overhead, which diminishes the expected performance gains. In this blog post, we'll discuss how to maximize the efficiency of ThreadPoolExecutor
by understanding its architecture and how to configure it according to various needs.
What is ThreadPoolExecutor?
ThreadPoolExecutor
is designed to manage a pool of worker threads to execute various tasks asynchronously. Java's concurrent package provides this functionality to simplify the multithreading environment. The executor maintains a pool of threads, which it can reuse for multiple tasks, thereby reducing the cost of thread creation and destruction.
Core Concepts
- Core Pool Size: The number of threads kept in the pool, even if they are idle.
- Maximum Pool Size: The maximum number of threads allowed in the pool.
- Keep Alive Time: When the number of threads exceeds the core pool size, this defines how long excess threads can remain idle before being terminated.
- Work Queue: A queue to hold tasks before they are executed.
- ThreadFactory: Used to create new threads.
Understanding these concepts is essential for effectively leveraging ThreadPoolExecutor
.
Why Optimization Matters
A naive implementation might lead to significant overhead related to thread management, especially in scenarios involving high concurrency and task granularity. High overhead can result in:
- Increased latency
- Inefficient CPU usage
- Longer response times
To put this into context, consider a web server handling numerous requests. If thread management overhead is high, the server will not respond swiftly to incoming requests, leading to potential bottlenecks.
Common Configuration Patterns
1. Choosing the Right Core and Maximum Pool Size
Setting the right core and maximum pool sizes can drastically reduce overhead. The Java Community suggests setting the core pool size based on the number of available processors.
Example Code Snippet
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadPoolExecutor;
public class ThreadPoolExample {
public static void main(String[] args) {
int corePoolSize = Runtime.getRuntime().availableProcessors();
int maxPoolSize = corePoolSize * 2;
ThreadPoolExecutor executor = new ThreadPoolExecutor(
corePoolSize,
maxPoolSize,
60L, // Keep Alive Time
TimeUnit.SECONDS,
new ArrayBlockingQueue<>(100) // Work Queue
);
// Execute some tasks
// executor.execute(new MyRunnable());
}
}
Why this matters: Setting core and maximum sizes according to the machine's capabilities means you can take full advantage of available resources while minimizing latency from thread creation.
2. Selecting an Appropriate Work Queue
The type of work queue you choose can impact performance based on task duration and volume. The most common options are:
- ArrayBlockingQueue: A bounded blocking queue backed by an array.
- LinkedBlockingQueue: An optionally bounded blocking queue backed by linked nodes.
If your tasks are short-lived, consider an unbounded queue like LinkedBlockingQueue
to minimize rejection of tasks. Conversely, for long-running tasks, you might want a bounded queue like ArrayBlockingQueue
.
Example Code Snippet
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class QueueTypeExample {
public static void main(String[] args) {
ThreadPoolExecutor executor = new ThreadPoolExecutor(
10,
20,
60L,
TimeUnit.SECONDS,
new LinkedBlockingQueue<>()
);
// Execute some tasks
// executor.execute(new MyRunnable());
}
}
Why this matters: Choosing the right queue type helps in managing how tasks are stored and executed, directly influencing overall throughput.
3. Implementing a Custom Thread Factory
By default, ThreadPoolExecutor
uses the default thread factory, which doesn't offer much flexibility. Implementing a custom thread factory can provide insights like thread naming, prioritization, and even error logging.
Example Code Snippet
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.ThreadPoolExecutor;
public class CustomThreadFactoryExample {
public static void main(String[] args) {
ThreadFactory threadFactory = new ThreadFactory() {
private int threadCount = 0;
@Override
public Thread newThread(Runnable runnable) {
threadCount++;
return new Thread(runnable, "CustomThread-" + threadCount);
}
};
ThreadPoolExecutor executor = (ThreadPoolExecutor) Executors.newFixedThreadPool(10, threadFactory);
// Execute some tasks
// executor.execute(new MyRunnable());
}
}
Why this matters: Custom threads can help in debugging by giving more context around which threads are executing which tasks, thereby simplifying tracking and resolving issues.
Monitoring and Logging
One of the most beneficial strategies to tackle overhead is by implementing a robust monitoring system. You need to observe metrics like:
- Active thread count
- Completed task count
- Time taken for each task
This allows you to tweak configurations in real-time to find a balance best suited for your specific use case. Java’s ThreadPoolExecutor
provides getTaskCount()
, getCompletedTaskCount()
, and getActiveCount()
methods for monitoring.
Monitoring Code Snippet
import java.util.concurrent.ThreadPoolExecutor;
public class MonitoringExample {
public static void logExecutorStats(ThreadPoolExecutor executor) {
System.out.println("Active Threads: " + executor.getActiveCount());
System.out.println("Completed Tasks: " + executor.getCompletedTaskCount());
System.out.println("Total Tasks: " + executor.getTaskCount());
}
}
Final Thoughts
By optimizing your ThreadPoolExecutor
, you can significantly enhance your application's efficiency and responsiveness. It requires thoughtful consideration of core pool sizes, maximum limits, proper queue selections, and a custom thread factory.
This knowledge translates to lower latencies and better CPU utilization, leading to a smoother user experience. For further reading on Java concurrency, consider exploring Oracle's Java Concurrency Tutorial and Java Concurrency in Practice. These resources offer deeper dives into the intricacies of concurrent programming.
Takeaways
- Configure
ThreadPoolExecutor
thoughtfully. - Monitor performance to tweak settings as needed.
- Implement custom elements like thread factories for clarity.
- Keep learning about concurrency to ensure effective management.
By applying these principles, you can effectively combat the associated overhead and maximize efficiency in your concurrent Java applications.