Common Pitfalls When Using log4jdbc for JDBC Logging
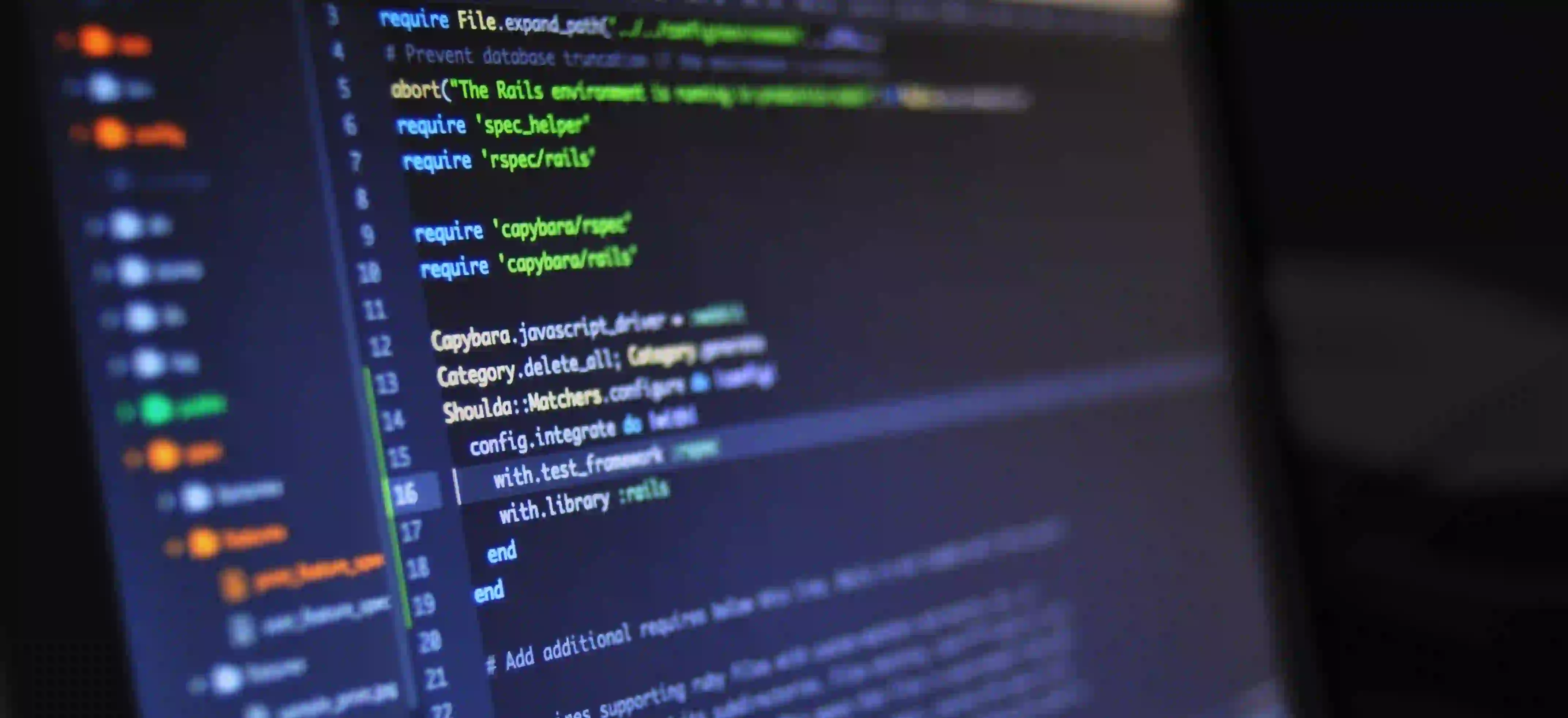
Common Pitfalls When Using log4jdbc for JDBC Logging
In the world of Java development, effective logging is essential for debugging and performance monitoring. One powerful tool in this space is log4jdbc, a library that allows you to log SQL statements and their parameters. While it greatly enhances your ability to track database interactions, there are several common pitfalls developers might encounter when implementing it. In this blog post, we'll explore these pitfalls and provide some best practices to help you avoid them.
What is log4jdbc?
log4jdbc is a wrapper around JDBC that enables logging of all executed SQL statements, parameters, and connection management details. By using this tool, developers can easily monitor what's happening behind the scenes in their database interactions, making debugging a much easier process.
To get started, you need to add the necessary dependencies to your project. Here's how you can include log4jdbc in a Maven project:
<dependency>
<groupId>org.lazyrhinos</groupId>
<artifactId>log4jdbc-log4j2</artifactId>
<version>1.0</version>
</dependency>
With this dependency added, you can start logging JDBC calls by configuring your DataSource
to use the log4jdbc driver.
Common Pitfalls and How to Avoid Them
1. Misconfigured logging level
One of the first issues developers encounter when using log4jdbc is misconfiguration of logging levels. If the logging level is set too high (for example, INFO
or DEBUG
), the logs can become flooded with information that is unnecessary or irrelevant.
Solution: Adjust your logging configurations in log4j2.xml
(or whichever logging framework you are using). Here is an example of how you might set this up:
<Configuration>
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Logger name="jdbc.sqlonly" level="INFO" additivity="false">
<AppenderRef ref="Console"/>
</Logger>
<Root level="WARN">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
By setting specific loggers and using more targeted levels, you can significantly reduce noise in your logs.
2. Not Logging SQL Parameters
Another common pitfall is failing to log SQL parameters. Logging the executed SQL statements without their parameters can lead to confusion, especially when studying how data was sent to the database.
Example Code:
public void executeQuery(String sql, Object... params) {
try (Connection conn = dataSource.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
//Setting parameters
for (int i = 0; i < params.length; i++) {
pstmt.setObject(i + 1, params[i]);
}
ResultSet rs = pstmt.executeQuery();
// Process results...
} catch (SQLException e) {
logger.error("SQL Exception: ", e);
}
}
In this example, ensure that the log4jdbc
configuration is structured to capture parameters. Use the jdbc.sqlonly
logger to log prepared statement parameters, enhancing your ability to troubleshoot later.
3. Performance Overhead
Logging can introduce performance overhead, especially when logging detailed information about slow queries. Continuous logging can slow down application performance due to the extensive output generated.
Solution: Use appropriate filters. For instance, configure log4jdbc to log only slow queries or specific warnings. Below is an example of how this might look:
<Logger name="jdbc.sqlonly" level="ERROR" additivity="false">
<AppenderRef ref="SlowSql"/>
<AppenderRef ref="Console"/>
</Logger>
4. Ignoring Connection Pooling
Many applications utilize connection pooling for efficiency. If log4jdbc is not integrated properly with your connection pool, you may end up with connection leaks or unexpected behavior.
When using a connection pool like HikariCP, ensure that log4jdbc is wrapped around the connection pool’s DataSource
. For HikariCP, this can be done as follows:
HikariDataSource ds = new HikariDataSource();
ds.setJdbcUrl("jdbc:log4jdbc:h2:mem:testdb");
By using the log4jdbc
JDBC URL prefix, you can effectively capture connection pooling behaviors.
5. Not Handling Exceptions
When you are logging SQL statements, ensure that you have proper exception handling. Developers often overlook this, which can lead to failed transactions going unnoticed.
Example Code:
try {
// Execute your SQL commands here
} catch (SQLIntegrityConstraintViolationException e) {
logger.error("Data integrity violation: ", e);
} catch (SQLException e) {
logger.error("SQL Exception caught: ", e);
}
By capturing different types of exceptions, you will have a more comprehensive view of issues that may arise during database interactions.
6. Not Cleaning Up Resources
Failing to clean up resources such as database connections can lead to memory leaks. It’s crucial to always close your Connection
, PreparedStatement
, and ResultSet
objects in a finally
block or use try-with-resources to ensure they get closed properly.
Example Code:
try (Connection conn = dataSource.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
// Your logic here...
} catch (SQLException e) {
logger.error("Error occurred: ", e);
} // Resources are automatically closed here.
7. Ignoring SQL Injection Risks
While logging is critical, it’s vital to remember that logging raw SQL statements can expose vulnerabilities. Logging sensitive information, such as user passwords or sensitive data, can lead to security risks.
Solution: Ensure that you either mask sensitive data in your logs or avoid logging sensitive queries altogether. Make use of prepared statements, as shown earlier, which help mitigate SQL injection risks.
Closing Remarks
log4jdbc can be an invaluable tool for Java developers looking to improve their database logging capabilities. However, it is essential to avoid the common pitfalls discussed in this blog post to reap the full benefits. By configuring logging levels, handling exceptions properly, and cleaning up resources, you can build a logging system that is efficient, informative, and secure.
For more resources on JDBC logging and best practices, consider checking the official log4jdbc documentation or best practices in Java logging.
By paying attention to these details, you can enhance your application's reliability and maintainability effectively. Happy coding!