Overcoming SWT Mouse Click Issues: A Developer's Guide
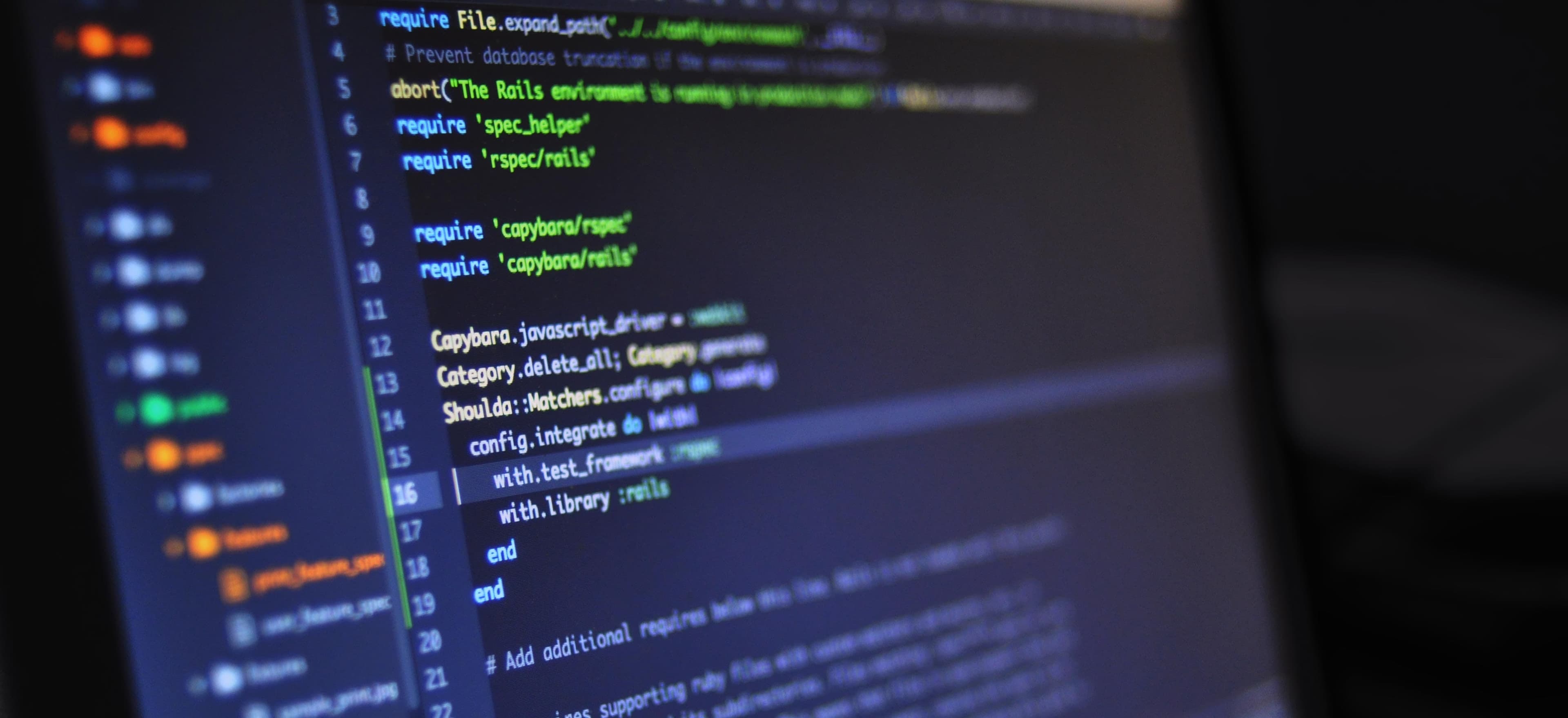
- Published on
Overcoming SWT Mouse Click Issues: A Developer's Guide
The Standard Widget Toolkit (SWT) is a powerful library for developing Java-based graphical user interfaces (GUIs). Although SWT offers rich capabilities to build interactive applications, developers often encounter challenges related to mouse click events. In this post, we'll explore common mouse click issues in SWT, the reasons behind them, and effective solutions to overcome these hurdles.
Understanding SWT Mouse Click Events
In SWT, mouse events are crucial for any interactive application. They allow developers to capture user interactions, such as clicks, drags, and movements. Here’s how you listen for mouse click events:
// Import necessary SWT classes
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.MouseAdapter;
import org.eclipse.swt.events.MouseEvent;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
// Main class for the application
public class MouseClickExample {
public static void main(String[] args) {
// Create a Display and Shell (window)
Display display = new Display();
Shell shell = new Shell(display);
// Set Shell properties
shell.setText("SWT Mouse Click Example");
shell.setSize(300, 200);
// Add MouseListener to handle mouse clicks
shell.addMouseListener(new MouseAdapter() {
@Override
public void mouseDown(MouseEvent e) {
System.out.println("Mouse clicked at: " + e.x + ", " + e.y);
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
Explanation of the Code
-
Display and Shell: These are core components to create an SWT application. The
Display
object manages the connection with the operating system, whileShell
represents a window. -
MouseAdapter: This is an abstract class that you can subclass to listen for mouse events. By overriding
mouseDown
, you can capture the mouse click's x and y coordinates.
Common SWT Mouse Click Issues
Despite SWT's robust framework, developers face several recurring issues related to mouse clicks:
- Multiple Mouse Click Events: Sometimes, a single click triggers multiple events. This can confuse users and lead to undesired outcomes.
- Focus Problems: In certain situations, the focus may not be correctly set, making it so clicks are registered elsewhere or not at all.
- Layered Components: In applications with layered visuals, clicks may not be received by the intended component due to overlapping areas.
Diagnosing the Issues
To tackle these problems, a methodical approach is necessary. Here are steps to diagnose the issues:
-
Check Event Registration: Ensure that your event listeners are not duplicated. Use breakpoints or logging to monitor when events are triggered.
-
Focus Management: Make certain that your Shell or widget has the focus by calling
setFocus()
where appropriate. -
Z-Order Examination: Check the Z-order of your UI components. Use methods like
bringToTop()
orsetBounds()
to control how components layer over one another.
Effective Solutions for Mouse Click Issues
1. Preventing Multiple Clicks
Let's tackle the problem of multiple mouse clicks. One common solution is to debounce mouse click events, ensuring that only one event is processed within a specified time frame.
Here’s an example of debounce logic using a timer:
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class ClickDebounce {
private static long lastClickTime = 0;
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.addMouseListener(new MouseAdapter() {
@Override
public void mouseDown(MouseEvent e) {
long currentTime = System.currentTimeMillis();
if (currentTime - lastClickTime > 300) { // 300 milliseconds threshold
System.out.println("Mouse clicked at: " + e.x + ", " + e.y);
lastClickTime = currentTime;
}
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
Explanation of Debounce Logic
- Timer Check: We store the last click time. When a new click event occurs, it checks whether enough time has passed before processing it. This simple logic can effectively mitigate the problem of repeated events.
2. Ensuring Correct Focus
In SWT, it is critical to have the correct widget in focus. To programmatically ensure focus when a user interacts with a component, you can add:
shell.addMouseListener(new MouseAdapter() {
@Override
public void mouseDown(MouseEvent e) {
shell.setFocus(); // Set focus explicitly
System.out.println("Clicked and focus set on shell.");
}
});
This guarantees that every mouse click will set the appropriate focus, preventing potential confusion over which component is active.
3. Handling Layered Components
When dealing with overlapping components, it’s vital to manage mouse events effectively. Here’s how you can drag a component to the front when clicked:
import org.eclipse.swt.graphics.Color;
public class LayeredClick {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
final Canvas overlappingCanvas = new Canvas(shell, SWT.NONE);
overlappingCanvas.setBackground(new Color(display, 100, 100, 255));
overlappingCanvas.setBounds(50, 50, 100, 100);
// Detect clicks on the overlapping_canvas
overlappingCanvas.addMouseListener(new MouseAdapter() {
@Override
public void mouseDown(MouseEvent e) {
overlappingCanvas.setBounds(50, 50, 100, 100);
overlappingCanvas.bringToTop();
System.out.println("Canvas brought to top.");
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
Explanation of Layering Logic
- Bringing To Top: When clicking on the
Canvas
, we invokebringToTop()
to ensure it is on top of any overlapping components. This act clarifies user interactions while ensuring their clicks register properly.
Bringing It All Together
Mouse click issues in SWT are common challenges faced by many developers. By understanding the basic mechanics behind mouse events and properly diagnosing the issues, you can implement effective solutions. From preventing multiple clicks and managing focus to handling layered components, these strategies will help you enhance the interactivity of your SWT applications significantly.
For further reading, consider checking out the official SWT documentation for comprehensive guidance on mouse events and widget behavior. Or visit Stack Overflow to explore community solutions and share your own challenges.
Embrace these insights, and turn your SWT applications into robust, user-friendly interfaces that solve common issues with ease. Happy coding!
Checkout our other articles