Mastering Android Custom Grid Views: A Common Pitfall
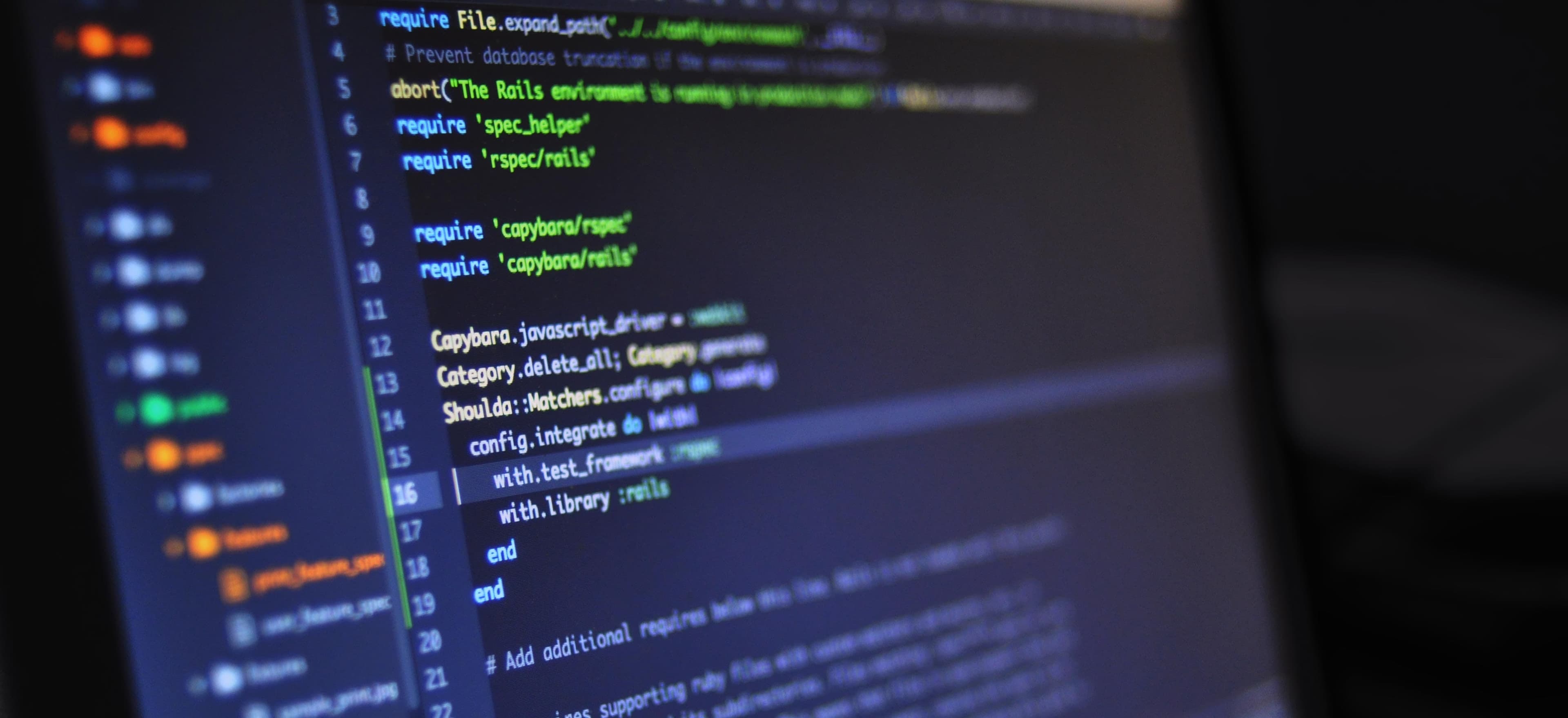
- Published on
Mastering Android Custom Grid Views: A Common Pitfall
Creating a stunning and effective user interface (UI) in Android applications can be challenging, especially when it comes to displaying lists of items gracefully. One of the commonly used layouts is the GridView. While it offers a visually appealing and intuitive way to present data, developers often stumble upon various pitfalls when customizing GridViews.
In this blog post, we'll deep dive into how to master Android custom GridViews by walking through common pitfalls and methodical solutions. We will also provide practical code snippets for clarity and understanding.
What Is a GridView?
A GridView is a view that displays items in a two-dimensional, scrollable grid format. Items are organized in a grid layout with a fixed number of columns. It's commonly used to showcase images, icons, or any content that can be represented in a grid.
Setting Up Your GridView
Before we explore the pitfalls, let's set up a basic GridView in an Android application.
Step 1: Layout File (XML)
First, define your GridView in your activity_main.xml
:
<GridView
android:id="@+id/gridView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:numColumns="2"
android:verticalSpacing="10dp"
android:horizontalSpacing="10dp"
android:stretchMode="columnWidth"
android:gravity="center" />
Step 2: Adapter Class
Next, you need a custom adapter. The adapter serves as a bridge between your data source and your GridView.
public class CustomGridAdapter extends BaseAdapter {
private Context context;
private List<String> items;
public CustomGridAdapter(Context context, List<String> items) {
this.context = context;
this.items = items;
}
@Override
public int getCount() {
return items.size();
}
@Override
public Object getItem(int position) {
return items.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ImageView imageView;
if (convertView == null) {
// If it's not recycled, initialize some attributes
imageView = new ImageView(context);
imageView.setLayoutParams(new GridView.LayoutParams(200, 200));
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imageView.setPadding(8, 8, 8, 8);
} else {
imageView = (ImageView) convertView;
}
// Set the image resource (placeholder)
imageView.setImageResource(R.drawable.placeholder);
return imageView;
}
}
Step 3: Activity Class
Fill the GridView with your custom adapter in MainActivity.java
:
public class MainActivity extends AppCompatActivity {
private GridView gridView;
private CustomGridAdapter adapter;
private List<String> itemList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gridView = findViewById(R.id.gridView);
itemList = new ArrayList<>(Arrays.asList("Item 1", "Item 2", "Item 3"));
adapter = new CustomGridAdapter(this, itemList);
gridView.setAdapter(adapter);
}
}
Common Pitfalls of Using GridView
Pitfall 1: Inefficient View Recycling
One of the most common issues developers face involves improperly managing view recycling in the adapter’s getView
method, leading to performance issues.
Solution: Always check if convertView
is null before inflating a new view. Recycle the views effectively.
Here’s how the example above handles view recycling with appropriate comments:
if (convertView == null) {
imageView = new ImageView(context);
// Set layout parameters and other attributes
} else {
imageView = (ImageView) convertView; // Reuse the existing view
}
Pitfall 2: Lack of Performance Optimization
Creating views in the getView()
method can be computationally expensive, especially when rendering a large dataset. This can lead to laggy scrolling.
Solution: Use the ViewHolder pattern or data binding to store inflated views for reuse. This prevents unnecessary calls to inflate new views each time.
Enhanced ViewHolder Pattern
To implement the ViewHolder pattern in your custom adapter, you can modify your getView()
method as follows:
public class ViewHolder {
ImageView imageView;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
holder = new ViewHolder();
convertView = LayoutInflater.from(context).inflate(R.layout.grid_item, parent, false);
holder.imageView = convertView.findViewById(R.id.image_view);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
// Set your image resource
holder.imageView.setImageResource(R.drawable.placeholder);
return convertView;
}
Pitfall 3: Mismanaged Item Clicks
Another oversight involves managing user engagement with items within the GridView. Often, items do not respond appropriately to user clicks, leading to a frustrating experience.
Solution: Set an OnItemClickListener
.
Here’s how you can do that in MainActivity
:
gridView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// Handle item click
Toast.makeText(getApplicationContext(), "Clicked: " + itemList.get(position), Toast.LENGTH_SHORT).show();
}
});
The Closing Argument
Mastering custom GridViews in Android requires understanding common pitfalls and applying effective solutions. By managing view recycling, optimizing performance with the ViewHolder pattern, and properly handling item clicks, you can create a smooth user experience.
As you develop your applications, remember to keep these best practices in mind, fostering efficiency and great user experiences.
To further enrich your knowledge, you can explore the Android developer documentation on GridView for additional information.
Stay tuned for more articles focusing on advanced Android UI techniques, and happy coding!