Common Java to LDAP Integration Issues and Fixes
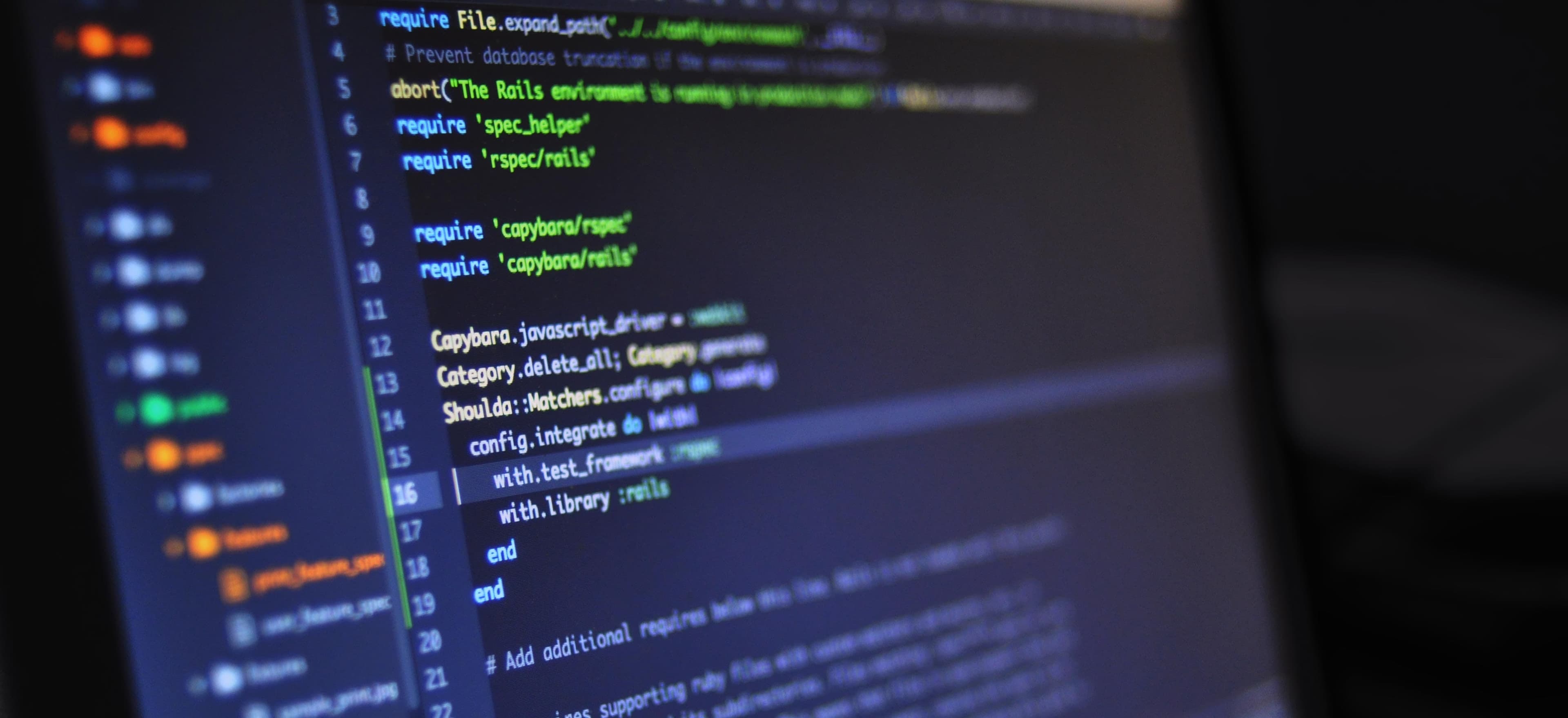
- Published on
Common Java to LDAP Integration Issues and Fixes
Integrating Java applications with an LDAP (Lightweight Directory Access Protocol) directory can be a powerful way to manage user authentication and authorization. However, as with any integration, developers often face challenges. This blog post explores some common issues encountered when integrating Java with LDAP, along with practical fixes. The goal is to equip you with insights and solutions to streamline your development workflow while adhering to best practices.
Understanding LDAP
Before diving into the issues, it’s essential to understand what LDAP is. LDAP is a protocol used to access and manage directory information. Typically, this includes user details, group memberships, and organizational structures. In Java, developers can use the JNDI (Java Naming and Directory Interface) API to work with LDAP servers.
Preparation: Setting Up a Sample Java Project
To effectively illustrate these issues and their resolutions, let’s outline the preparation needed for our Java project.
Maven Dependencies
Make sure you have the following Maven dependencies in your pom.xml
:
<dependency>
<groupId>javax.naming</groupId>
<artifactId>javax.naming-api</artifactId>
<version>1.3.5</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.32</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.32</version>
</dependency>
These dependencies will facilitate logging and provide the necessary tools for directory access.
Common Issues and Their Fixes
1. Authentication Failures
One of the most frequent issues developers face is authentication failures. This occurs when your Java application cannot authenticate a user against the LDAP server.
Why It Happens: This can stem from incorrect credentials, an improperly configured LDAP connection, or issues with the LDAP server itself.
Example Code Snippet:
import javax.naming.*;
import javax.naming.directory.*;
public class LdapAuthentication {
public boolean authenticate(String username, String password) {
String ldapUrl = "ldap://localhost:389";
Hashtable<String, String> env = new Hashtable<>();
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory");
env.put(Context.PROVIDER_URL, ldapUrl);
env.put(Context.SECURITY_AUTHENTICATION, "simple");
env.put(Context.SECURITY_PRINCIPAL, username); // DN of the user
env.put(Context.SECURITY_CREDENTIALS, password); // User's password
try {
new InitialDirContext(env); // Attempt to connect to LDAP
return true; // Authentication was successful
} catch (NamingException e) {
// Log the error
System.err.println("Authentication failed: " + e.getMessage());
return false; // Authentication failed
}
}
}
Fix: Always double-check the format of the username you are passing in. It may need to include base DN or other structural details, depending on your LDAP configuration.
2. Connection Timeout
Connection timeouts can severely affect application performance. When your application tries to connect to an LDAP server and fails to do so within a specified time, it leads to a timeout error.
Why It Happens: This can occur due to network issues, an unreachable LDAP server, or improper socket configurations.
Example Code Snippet:
env.put("java.naming.ldap.connect.timeout", "5000"); // Timeout of 5 seconds
Fix: Set an appropriate timeout duration in your environment settings. Test with various timeout values to find what works best within your network conditions.
3. Improper User Search
Sometimes, applications may unexpectedly fail to find a user in the directory. This can manifest as a user not being authenticated when they should be.
Why It Happens: This may boil down to an incorrectly defined search base or search filter.
Example Code Snippet:
String searchBase = "ou=users,dc=example,dc=com";
String searchFilter = "(uid=" + username + ")";
DirContext ctx = new InitialDirContext(env);
NamingEnumeration<SearchResult> results = ctx.search(searchBase, searchFilter, searchControls);
if (results.hasMoreElements()) {
// User found
}
Fix: Ensure that your search base and filter comply with your LDAP schema. Use tools like ldapsearch to simulate and debug queries directly against your LDAP server.
4. Certificate Issues (SSL/TLS)
In environments where secure connections are required, SSL/TLS certificate-related issues can prevent successful LDAP connections.
Why It Happens: The Java application may not trust the provided SSL certificate, leading to connection failures.
Example Code Snippet:
- Use the following to enable SSL:
System.setProperty("javax.net.ssl.trustStore", "path/to/truststore");
System.setProperty("javax.net.ssl.trustStorePassword", "password");
Fix: Import the LDAP server's SSL certificate into your Java keystore. This step will allow your application to establish secure connections without issues.
5. No Results Found
If your application executes a search and returns no results, it can be frustrating. This could indicate that your search criteria need adjustments.
Why It Happens: The causes may stem from incorrect filters, search bases, or simply because the user indeed does not exist.
Example Code Snippet:
// Adjust search controls to limit results
SearchControls searchControls = new SearchControls();
searchControls.setSearchScope(SearchControls.SUBTREE_SCOPE);
Fix: Examine LDAP entries through an LDAP browser tool and verify the data. Adjust your search based on observed directory structures.
A Final Look
Integrating Java applications with LDAP servers can introduce its own set of challenges, but understanding these issues and knowing how to troubleshoot effectively can enhance your application's robustness.
From authentication issues to connection timeouts and improper user searches, addressing these common problems can help streamline your development process while ensuring a smooth user experience.
For further reading, check out the official Java Naming and Directory Interface tutorial and the LDAP Reference Guide. These resources can provide additional context and advanced usage scenarios.
Feel free to explore the world of LDAP in your Java applications and leverage its capabilities for enhanced user management. Happy coding!
Checkout our other articles