Overcoming Data Consistency Challenges in Oracle Coherence
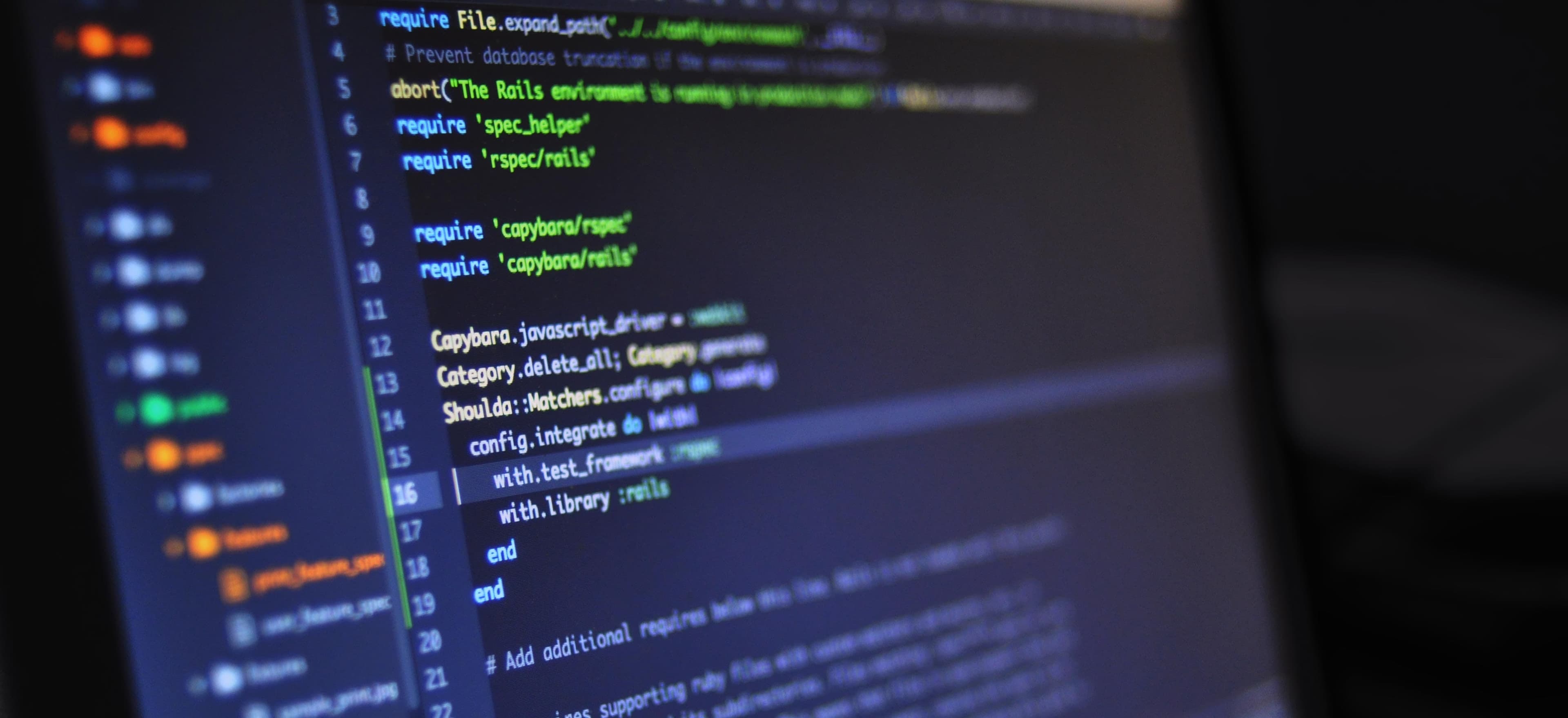
- Published on
Overcoming Data Consistency Challenges in Oracle Coherence
In a world where real-time data processing is paramount, achieving data consistency can be a formidable challenge. This is particularly true in distributed systems like Oracle Coherence. Oracle Coherence is a widely used in-memory data grid solution that helps businesses scale, available through Oracle Cloud. However, with the increased performance and scalability comes the difficulty of maintaining data consistency. In this blog post, we will delve into various approaches to overcome data consistency challenges in Oracle Coherence.
Understanding Data Consistency
Before we explore specific solutions, it's essential to understand what we mean by data consistency. Data consistency refers to the correctness and uniformity of data across the distributed nodes of a system. In Oracle Coherence, which is designed to work seamlessly across multiple nodes, issues may arise when different nodes hold different versions of the same dataset.
Data inconsistency can lead to significant issues such as incorrect application behavior, customer dissatisfaction, and data corruption. Therefore, it is crucial to implement strategies that ensure consistent data across all nodes.
Challenges of Maintaining Data Consistency in Oracle Coherence
Several factors contribute to the challenges of data consistency in Oracle Coherence, including:
- Network Partitions: Nodes can momentarily lose connectivity, leading to updates that are not propagated to all nodes.
- Concurrent Writes: When multiple processes write to the same data simultaneously, it can cause conflicts and inconsistencies.
- Node Failures: Failures can occur due to various reasons including hardware malfunctions or network issues, causing data to go out of sync.
Consistency Strategies in Oracle Coherence
To face these challenges, Oracle Coherence offers various strategies for ensuring consistency. We will discuss the most effective ones below.
1. Replication
Replication involves creating copies of data across multiple nodes. Oracle Coherence supports two types of replication:
- Partitioned: Each partition is assigned to a specific set of nodes. This means that writes are localized, which can simplify data management.
- Backups: A configurable number of backup replicas can be maintained to ensure data is available even during node failures.
Code Example: Configuring Replication
import com.tangosol.net.CacheFactory;
import com.tangosol.net.NamedCache;
public class ReplicationExample {
public static void main(String[] args) {
NamedCache cache = CacheFactory.getCache("myCache");
cache.put("key1", "value1"); // Write to primary cache
// Simulating a failure by not writing to backup
// Thus showcasing how data is preserved in case of node failure
cache.put("key2", "value2");
}
}
Why Use Replication?
Replication provides high availability and fault tolerance. In the event of a node failure, other nodes with backup data ensure no data is lost, thus preserving the integrity of the application.
2. Partitioning
Partitioning divides the data into segments and distributes them across various nodes. This method helps to achieve scalability and can lead to better performance through reduced contention.
Code Example: Configuring Partitioned Cache
import com.tangosol.net.CacheFactory;
import com.tangosol.net.NamedCache;
public class PartitioningExample {
public static void main(String[] args) {
NamedCache cache = CacheFactory.getCache("partitionedCache");
cache.put("key1", "value1");
cache.put("key2", "value2");
// Each node only retrieves partitions it is responsible for
System.out.println(cache.get("key1")); // This would retrieve data from the assigned partition
}
}
Why Use Partitioning?
Partitioning distributes workloads across nodes, reducing bottlenecks associated with high traffic. It also minimizes contention issues, significantly improving throughput and response time.
3. Multicast Associations
In Coherence, multicast is a method of message delivery that uses a "publish-subscribe" pattern. This allows for a real-time distributed environment where all nodes receive the same updates.
Code Example: Using Multicast for Updates
import com.tangosol.net.CacheFactory;
import com.tangosol.net.NamedCache;
import com.tangosol.util.InvocableMap;
public class MulticastExample {
public static void main(String[] args) {
NamedCache cache = CacheFactory.getCache("multicastCache");
// Multicasting an update
cache.invoke("key1", new InvocableMap.AbstractEntryProcessor() {
public Object process(Map.Entry entry) {
entry.setValue("newValue");
return entry.getValue();
}
});
}
}
Why Use Multicasting?
Multicasting ensures that all nodes receive the most current state of data, significantly reducing the risk of inconsistency during updates.
4. Write-Behind Caching
Write-behind caching allows updates to be written to the cache and then asynchronously saved to the database in the background. This can improve performance while maintaining data consistency.
Code Example: Implementing Write-Behind
import com.tangosol.net.CacheFactory;
import com.tangosol.net.NamedCache;
public class WriteBehindExample {
public static void main(String[] args) {
NamedCache cache = CacheFactory.getCache("writeBehindCache");
cache.put("key1", "initialValue");
// Flush changes
cache.flush(); // This represents the async operation performed in the background
}
}
Why Use Write-Behind?
This approach significantly improves application responsiveness by reducing the latency associated with writing data to external storage, while still ensuring that data eventually reaches its destination with proper consistency.
A Final Look
In a distributed environment, such as Oracle Coherence, ensuring data consistency is a multifaceted challenge. Through replication, partitioning, multicast associations, and write-behind caching, organizations can implement strategies to mitigate these challenges effectively.
Choosing the right combination of techniques depends on specific use cases and requirements for performance and reliability. As systems scale, reviewing these strategies becomes important to ensure they continue to meet the evolving needs of your applications.
For more in-depth guidance, you can explore the Oracle Coherence Documentation or check the Oracle Blogs for the latest updates and best practices.
By employing the right strategies, you can ensure that your implementation of Oracle Coherence not only performs well but also maintains rigorous data consistency across all nodes.
Checkout our other articles