Overcoming the Challenges of Google Cloud Bigtable Scalability
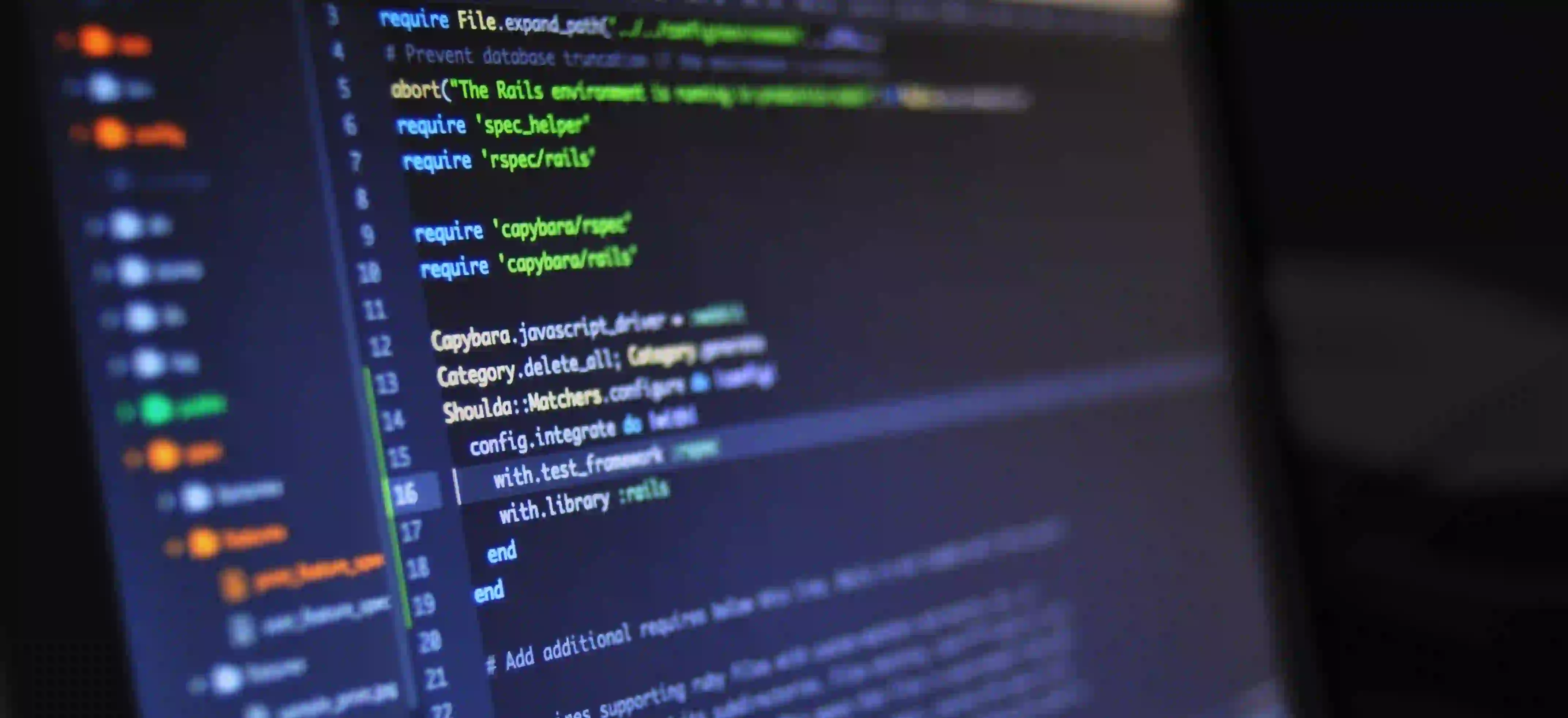
Overcoming the Challenges of Google Cloud Bigtable Scalability
Google Cloud Bigtable is a powerful, distributed storage system designed to manage large-scale, NoSQL data. Its horizontally scalable nature allows for the handling of massive amounts of data with ease, making it a popular choice among businesses that require high performance and low latency. However, the scalability of any database solution comes with its own set of challenges. In this blog post, we will discuss how to overcome the challenges of Google Cloud Bigtable scalability while ensuring optimal performance.
Understanding Google Cloud Bigtable
Before delving into the scalability challenges, it’s essential to understand what Google Cloud Bigtable offers. As a fully managed and scalable database service, Bigtable is suited for real-time analytics, operational workloads, and time-series data. It excels in use cases like:
- IoT Data Storage: Real-time metrics from sensors.
- Finance: Analyzing stock trends and market activity.
- Personalization Engines: Serving recommendations based on user behavior.
To get started with Bigtable, check out this official documentation that provides comprehensive guidelines.
Challenges of Scalability in Bigtable
While the benefits of Google Cloud Bigtable are apparent, challenges may arise as your data grows. Here are some common scalability issues:
- Hotspotting: This occurs when too many requests are directed to a single node, resulting in increased latency and reduced throughput.
- Data Model Complexity: A poorly designed schema can lead to inefficiencies and hinder scaling.
- Write Amplification: Frequent updates can increase storage I/O and affect system performance.
- Cost Management: Scaling can lead to unanticipated costs, especially if not planned properly.
Overcoming Hotspotting
1. Design Schema to Avoid Hotspots
A well-designed schema is critical to preventing hotspots in Bigtable. Avoid using sequential keys. For example, using timestamps as row keys can lead to multiple requests directed towards the latest row, thus creating a hotspot.
Instead, utilize randomization in your row keys. Here’s an example code snippet:
import com.google.cloud.bigtable.data.v2.BigtableDataClient;
import com.google.cloud.bigtable.data.v2.models.RowMutation;
String randomRowKey = "rowkey-" + System.currentTimeMillis() + "-" + UUID.randomUUID();
RowMutation mutation = RowMutation.create("my-table", randomRowKey);
mutation.setCell("cf", "temperature", System.currentTimeMillis(), 25);
bigtableClient.mutateRow(mutation);
Why Randomization?
By adding a UUID or a timestamp, you distribute the writes across multiple nodes. This minimizes the load on any single node.
2. Use Row Keys Wisely
When structuring row keys, use hashing techniques. This helps distribute data evenly across the cluster. For instance:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public String hashKey(String input) {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hash = md.digest(input.getBytes());
return Base64.getEncoder().encodeToString(hash);
}
Why Hashing?
Hashing allows you to segment your data in a manner that's less predictable, effectively spreading the load.
Navigating Data Model Complexity
3. Normalize Data Where Necessary
Normalizing your data can reduce redundancy and improve performance. Understand how your data relates to itself. If certain records are frequently accessed together, consider storing them in the same row.
4. Design with Access Patterns in Mind
In Bigtable, you query data based on row keys. Thus, designing your schema around your access patterns is essential. For example, if your application frequently retrieves data by user ID, ensure that the user ID is a key component of your row key.
String userIdKey = "user-" + userId;
RowMutation mutation = RowMutation.create("my-table", userIdKey);
mutation.setCell("cf", "last_login", System.currentTimeMillis(), new Date());
bigtableClient.mutateRow(mutation);
Why Access Patterns Matter?
Designing a schema based on access patterns ensures that queries can be resolved quickly and efficiently, optimizing read performance.
Reducing Write Amplification
5. Use Batch Writes
Depending on your use cases, employing batch writes can help mitigate write amplification. Instead of writing to the database frequently, accumulate changes and write them in bulk.
import com.google.cloud.bigtable.data.v2.models.MutationBatch;
import java.util.List;
List<RowMutation> mutations = //...collect mutations
bigtableClient.bulkMutate("my-table", mutations);
Why Batch Writes?
Batching reduces the total number of writes and lessens the strain on your I/O capacity.
Managing Costs Efficiently
6. Monitor and Analyze Usage
Understanding how your application interacts with Bigtable will help you manage costs effectively. Use Google Cloud's monitoring tools to gain insights into usage patterns. Implement quotas to limit costs.
- Use Stackdriver Monitoring for detailed metrics.
- Analyze performance through insights from the Bigtable metrics page.
7. Understand Capacity and Pricing
Familiarize yourself with Google Cloud's pricing model. Pay attention to the number of nodes, storage, and usage patterns to optimize costs.
Final Considerations
Overcoming the challenges of scalability in Google Cloud Bigtable may initially seem daunting. However, with careful planning and adherence to best practices, you can harness the full potential of Bigtable. By avoiding hotspots, designing your schema based on access patterns, and utilizing batch writing, you can ensure that your database remains performant and cost-effective.
For a more in-depth exploration of Bigtable capabilities, the Google Cloud Bigtable documentation remains an invaluable resource.
As you implement these strategies, remember that monitoring and optimization are ongoing processes. Stay proactive, and your implementation of Bigtable will scale alongside your business needs.
Additional Resources
- Bigtable vs. Other Databases
- Best Practices for Bigtable
- Google Cloud Pricing Calculator
By following these guidelines, you can create an effective, scalable infrastructure that meets your operational demands while taking full advantage of what Google Cloud Bigtable has to offer.