Mastering Java Generics: Common Interview Challenges Explained
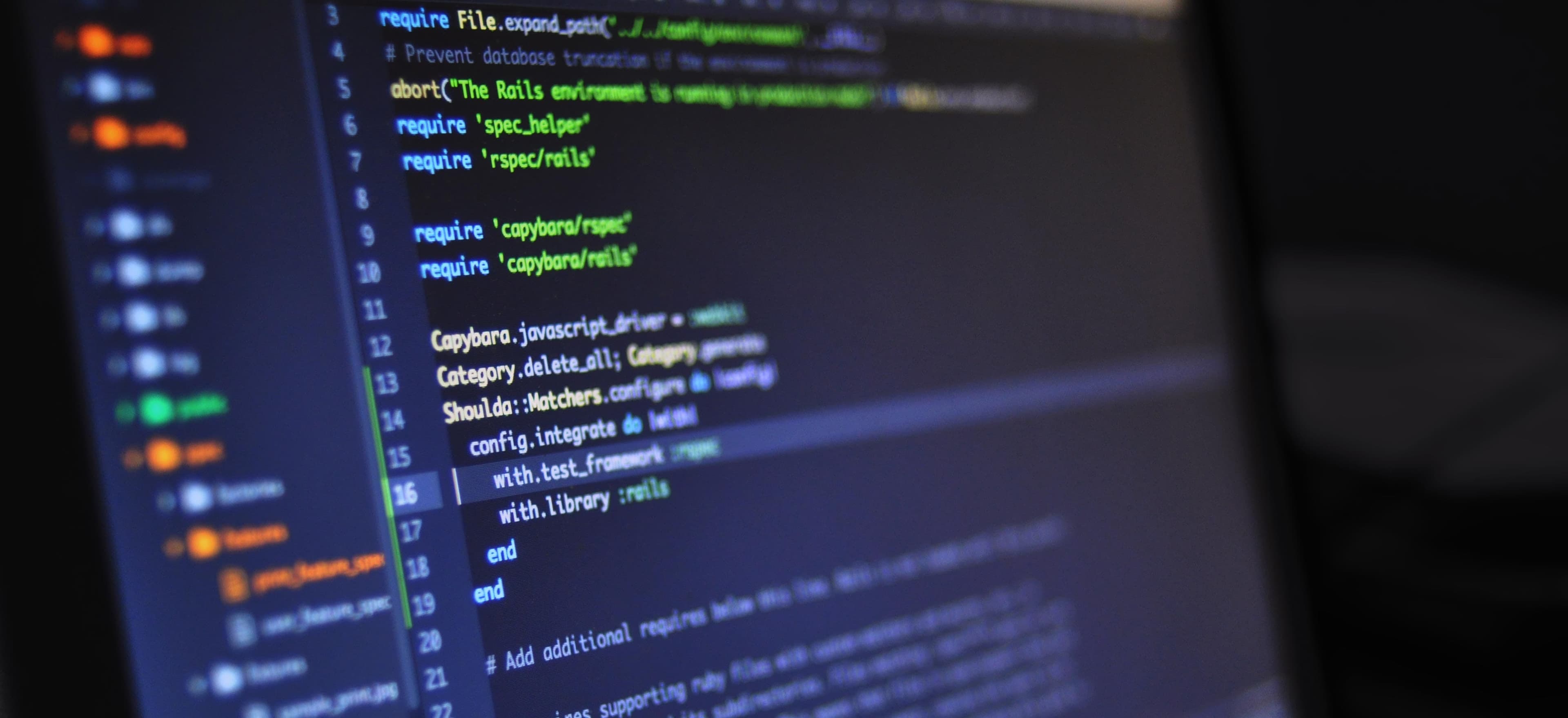
- Published on
Mastering Java Generics: Common Interview Challenges Explained
Java Generics is a powerful feature that enhances type safety and improves code reusability. As a crucial part of the Java programming language, they allow you to define classes, interfaces, and methods with a placeholder for the type of data they operate on. In the competitive job market for Java developers, understanding Generics is essential, especially during technical interviews.
This blog post aims to demystify common challenges and questions around Generics, backed by practical code snippets and real-world use cases.
Understanding Java Generics
Generics are a mechanism in Java that allows developers to specify, with a single method or class definition, the type of objects that can be passed to it. For example, a generic class can work with any data type—Integer
, String
, or even user-defined types.
Syntax of Generics
The syntax for Generics is straightforward. You define a type parameter using angle brackets <T>
. Here’s a simple example of a generic class:
public class GenericBox<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Why Generics?
- Type Safety: Generics ensure that you get compile-time type safety. It helps catch type mismatch errors early in the development process.
- Elimination of Casts: You do not need to cast the object when retrieving it. This reduces boilerplate code and the chances of runtime errors.
- Code Reusability: A single class or method can handle various data types, improving code reusability.
Common Interview Challenges: Explained
1. How do Wildcards Work in Java?
Wildcards are a special feature of Java Generics. They allow more flexibility in using Generics. The wildcard is defined using the question mark ?
.
Types of Wildcards
- Unbounded Wildcards:
<T>
- Upper-bounded Wildcards:
<T extends SomeClass>
- Lower-bounded Wildcards:
<T super SomeClass>
Example
Here’s an example of upper-bounded wildcards:
import java.util.*;
public class WildcardExample {
public static void addNumbers(List<? super Integer> list) {
list.add(10);
list.add(20);
}
public static void main(String[] args) {
List<Number> numberList = new ArrayList<>();
addNumbers(numberList);
System.out.println(numberList); // Outputs: [10, 20]
}
}
In this code:
- The method
addNumbers
accepts a list of type? super Integer
, meaning it can accept a list ofInteger
,Number
, or any super type. - It demonstrates flexibility while maintaining type safety, allowing for various numeric types.
2. What is the Difference Between List<E>
and List<Object>
?
Another common question focuses on the difference between a specific generic type and a raw type. When you use a generic type like List<E>
, it means that the list can only contain elements of the type E
. In contrast, when you use List<Object>
, the list can contain any object, including String
, Integer
, or custom objects.
Example
List<String> stringList = new ArrayList<>();
stringList.add("Hello");
// stringList.add(1); // This will cause a compile-time error
List<Object> objectList = new ArrayList<>();
objectList.add("Hello");
objectList.add(1); // This is perfectly valid
Why is this important?
Understanding these distinctions helps developers make better design decisions. When you design APIs or libraries, using specific generic types enhances type safety and reduces bugs.
3. What Are Type Parameters and Type Erasure?
Type parameters allow you to define a class or method that can work with any data type. You declare these parameters between angle brackets.
Type erasure, on the other hand, is the process of removing generic type information during compilation. After compilation, the generic parameters are replaced with their bounds or Object
if there are no bounds:
- This means
List<String>
andList<Integer>
will both be treated asList<Object>
after compilation.
public class TypeErasureDemo<T> {
public void show(T param) {
System.out.println(param);
}
}
In the above code, during runtime, the type information is lost. This is crucial for understanding some limitations of Generics, such as the inability to create instances of type parameters or arrays of type parameters.
4. Can You Create a Generic Static Method in a Non-generic Class?
Yes! You can create a static generic method within a non-generic class.
Example:
public class GenericStaticMethod {
public static <T> void display(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
public static void main(String[] args) {
Integer[] intArray = {1, 2, 3};
String[] strArray = {"A", "B", "C"};
display(intArray); // Prints: 1 2 3
display(strArray); // Prints: A B C
}
}
This method can accept any type of array, demonstrating the versatility of Generics.
5. Handling Multiple Type Parameters
Generics also support multiple type parameters, making your classes and methods even more flexible.
Example:
public class Pair<K, V> {
private K key;
private V value;
public Pair(K key, V value) {
this.key = key;
this.value = value;
}
public K getKey() {
return key;
}
public V getValue() {
return value;
}
}
public class Main {
public static void main(String[] args) {
Pair<String, Integer> pair = new Pair<>("One", 1);
System.out.println(pair.getKey() + " : " + pair.getValue());
}
}
With this example, you create a Pair
class that can hold a key-value pair of any types.
Additional Resources
For those looking for more in-depth study materials regarding Java Generics, I recommend checking out:
- Java Tutorials on Generics
- Effective Java by Joshua Bloch - This book provides great insights and best practices regarding Java, including Generics.
Closing the Chapter
Mastering Java Generics is a surefire way to impress potential employers in technical interviews. Understanding the various components, such as wildcards, type parameters, and type erasure, will equip you with the knowledge to tackle common challenges.
By embracing the principles of Generics, you will write safer and more flexible Java code, making you a valuable asset to any development team. Keep practicing these concepts, and you'll be well on your way to mastering Java Generics in no time!
Checkout our other articles