Fixing Hibernate: Binding Oracle Dates Without Hassle
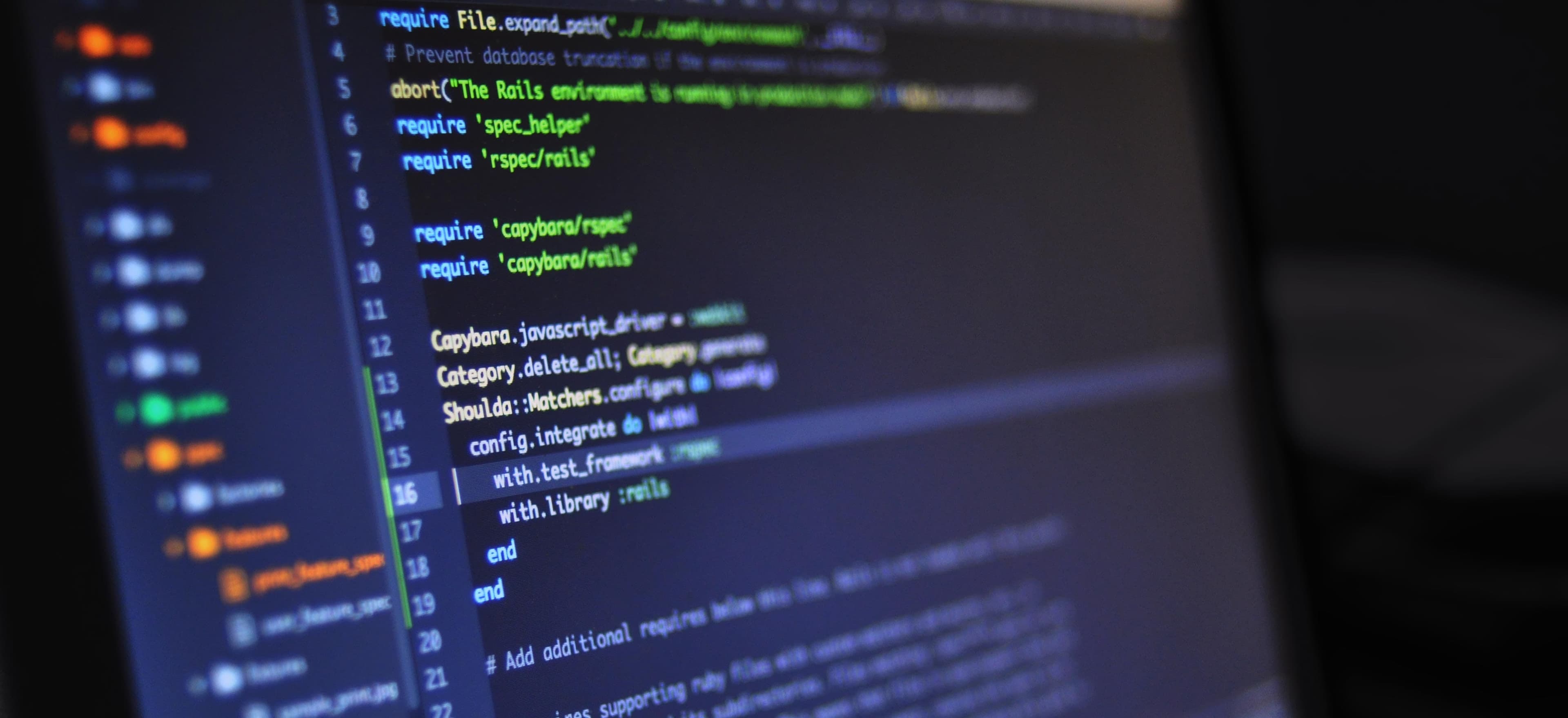
- Published on
Fixing Hibernate: Binding Oracle Dates Without Hassle
When working with databases, a common challenge developers face is effectively binding different data types to their ORM frameworks. In this blog post, we will focus on a specific issue: binding Oracle dates in Hibernate. We will explore the complexities involved and provide you with straightforward solutions. If you have ever struggled with date handling in Hibernate when your database is Oracle, this guide is tailored for you.
Why Oracle Dates Are Different
Oracle databases have their unique way of storing date and time, primarily utilizing the DATE
and TIMESTAMP
data types. These data types capture time down to the second but lack the timezone feature provided by other databases. Consequently, when binding these types in Hibernate, developers often encounter discrepancies in time handling.
Understanding Oracle's Date Types
- DATE: Stores the date and time, with precision to the second.
- TIMESTAMP: Offers higher precision (nanoseconds) but lacks timezone support.
The Problem with Standard Hibernate Configuration
By default, Hibernate expects date types as Java's java.util.Date
or java.time.LocalDateTime
. The disparity between these assumed types and Oracle’s native types can lead to incorrect data being stored or queried.
Setup: Required Dependencies
Before diving into solutions, ensure you have the necessary dependencies in your pom.xml
if you are using Maven.
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.x.Final</version>
</dependency>
<dependency>
<groupId>com.oracle.database.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>19.8.0.0</version>
</dependency>
Make sure to replace the version numbers as per your project’s needs.
Proposed Solutions
1. Custom Hibernate UserType
One of the effective ways to address date binding issues is to create a custom UserType
. This class will define how Hibernate should convert between Oracle’s date types and Java types.
Here’s a simplified version:
import org.hibernate.type.DateType;
import org.hibernate.usertype.UserType;
import java.io.Serializable;
import java.sql.*;
import java.util.Calendar;
import java.util.Date;
public class OracleDateUserType implements UserType {
@Override
public int[] sqlTypes() {
return new int[]{Types.TIMESTAMP};
}
@Override
public Class<?> returnedClass() {
return Date.class;
}
@Override
public boolean equals(Object x, Object y) {
return x == null ? y == null : x.equals(y);
}
@Override
public int hashCode(Object x) {
return x == null ? 0 : x.hashCode();
}
@Override
public Object nullSafeGet(ResultSet rs, String[] names, SharedSessionContractImplementor session, Object owner) throws SQLException {
Timestamp ts = rs.getTimestamp(names[0]);
return ts != null ? new Date(ts.getTime()) : null;
}
@Override
public void nullSafeSet(PreparedStatement st, Object value, int index, SharedSessionContractImplementor session) throws SQLException {
if (value == null) {
st.setNull(index, Types.TIMESTAMP);
} else {
st.setTimestamp(index, new Timestamp(((Date) value).getTime()));
}
}
// Other required methods would go here...
}
Explanation
- nullSafeGet: This method retrieves the date from the database and converts it to a Java Date object.
- nullSafeSet: This method takes a Java Date object and persists it as an Oracle TIMESTAMP.
This custom UserType ensures that when you map the date column in your entity, Hibernate will utilize your custom logic, bridging the gap between Oracle and Java date handling.
2. Using Hibernate Types Library
Alternatively, the Hibernate Types library offers a pre-built solution to manage Oracle Dates and other peculiarities. This library includes a LocalDateTimeType
and other useful types that simplify handling complex mappings.
<dependency>
<groupId>com.vladmihalcea.hibernate</groupId>
<artifactId>hibernate-types-52</artifactId>
<version>2.14.0</version>
</dependency>
Example of Usage
import com.vladmihalcea.hibernate.type.date.LocalDateTimeType;
@Entity
public class ExampleEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Type(LocalDateTimeType.class)
@Column(name = "created_at")
private LocalDateTime createdAt;
// Getters and Setters...
}
3. Adjust the Default Hibernate Dialect
When using Hibernate, it's important to configure the dialect accurately. Oracle databases require the use of Oracle12cDialect
, which is built for managing some of the peculiarities of the Oracle database.
In your application.properties
, specify the dialect:
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.Oracle12cDialect
This simple configuration helps offload much of the date conversion hassle to Hibernate, leveraging its inbuilt capabilities.
Testing Your Configuration
It's vital to run thorough tests to ensure that your new configurations work correctly. Create sample data entries and attempt to retrieve them:
ExampleEntity entity = new ExampleEntity();
entity.setCreatedAt(LocalDateTime.now());
session.save(entity);
ExampleEntity fetchedEntity = session.get(ExampleEntity.class, entity.getId());
System.out.println(fetchedEntity.getCreatedAt());
To Wrap Things Up
Binding Oracle dates in Hibernate doesn’t have to be a headache. By employing a custom UserType
, utilizing the Hibernate Types library, or configuring the correct dialect, you can streamline your application’s interaction with Oracle dates.
Properly managing date types is crucial for ensuring the integrity of your data. Developing familiarity with these tools will make your Java applications more robust and maintainable.
For further reading, check out the official Hibernate Documentation and the aforementioned Hibernate Types library, which provide extensive options for advanced ORM mappings.
Happy coding!
Checkout our other articles