Overcoming Class Loading Challenges in Evolving APIs
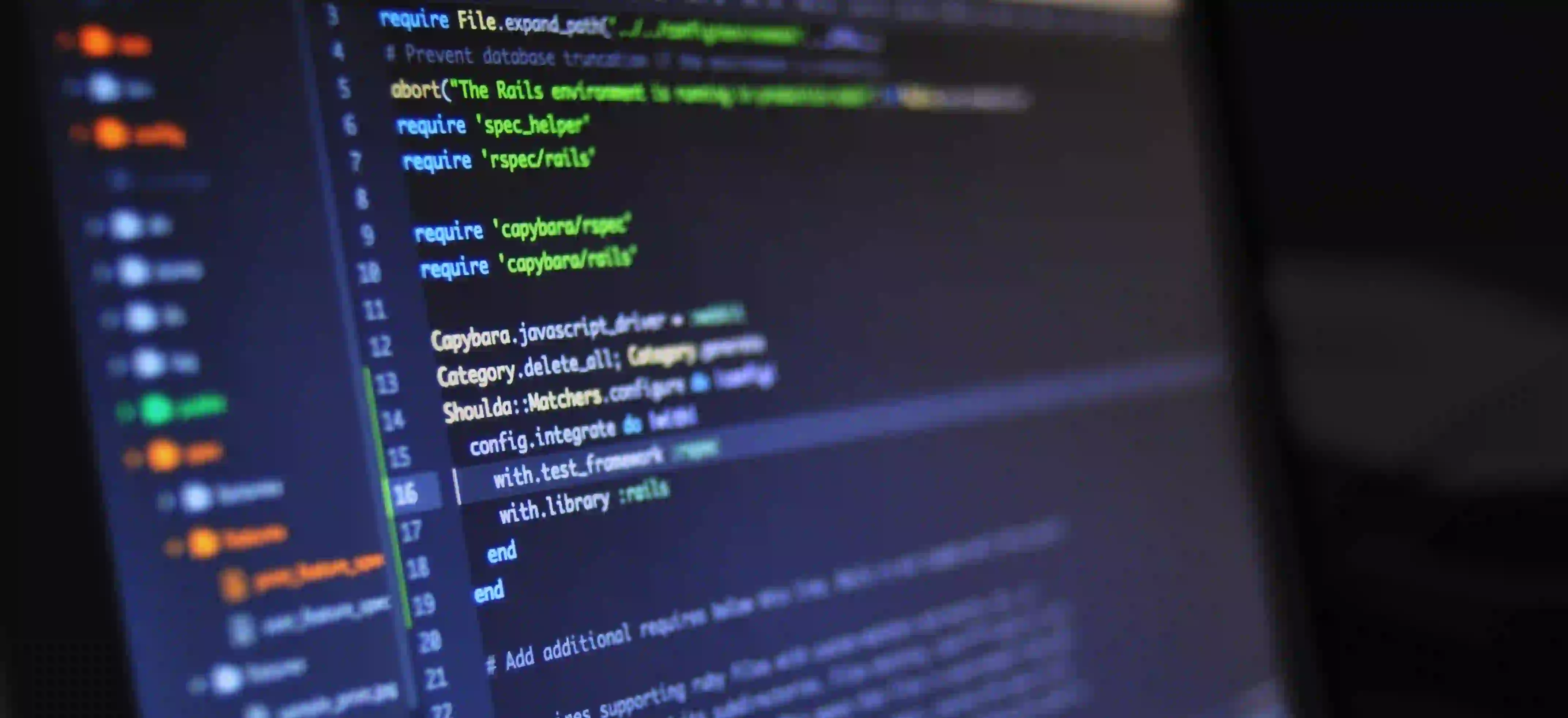
Overcoming Class Loading Challenges in Evolving APIs
In today's fast-paced software development landscape, APIs are constantly evolving. While advancements lead to better functionality and performance, they also present several challenges—especially in terms of class loading in Java. This blog post aims to explore these challenges and provide strategies to navigate the complexities of class loading in evolving APIs.
Understanding Class Loading in Java
Class loading is the process of loading classes into the Java Virtual Machine (JVM). In Java, the class loader is responsible for this task. The JVM uses a hierarchical structure of class loaders, primarily consisting of:
- Bootstrap Class Loader: Loads core Java classes from the Java Runtime Environment (JRE).
- Extension Class Loader: Loads classes from the Java extensions directory.
- System/Application Class Loader: Loads classes from the application classpath.
The Challenges of Evolving APIs
Evolving APIs can wreak havoc on the class loading mechanism. Here are some prominent issues:
- Version Conflicts: Different versions of a library may introduce changes in class definitions, making it challenging to manage dependencies.
- Class Visibility: Classes may not be visible if multiple versions reside in different class loaders.
- Namespace Pollution: Introducing new classes can introduce naming conflicts with existing classes.
- Dynamic Proxies & Reflection: These powerful features can lead to complications when APIs change.
Best Practices to Overcome Class Loading Challenges
To mitigate the issues resulting from evolving APIs, consider the following best practices:
1. Use a Modular Architecture
Java's Java Platform Module System (JPMS) allows you to develop applications as a set of modules. Each module can specify which of its packages are accessible to other modules. This enforces clean boundaries between components.
Example Module Declaration:
module com.example.api {
exports com.example.api.core;
requires com.example.dependency;
}
Why Use Modules?
Modules prevent version conflicts by ensuring that each part of your application operates in its isolated context, reducing the risk of namespace pollution.
2. Utilize Dependency Management Tools
Tools like Maven and Gradle facilitate the management of dependencies. They provide mechanisms to handle transitive dependencies, ensuring that only the required versions are included.
Example Maven Dependency Configuration:
<dependency>
<groupId>org.example</groupId>
<artifactId>api-library</artifactId>
<version>1.0.0</version>
</dependency>
Why Use Dependency Management Tools?
These tools streamline dependency resolution, ensuring compatibility and reducing potential conflicts in complex applications.
3. Class Loader Isolation
In scenarios requiring multiple versions of the same library (e.g., application servers), isolate your class loaders. Custom class loaders can load only certain classes, preventing conflicts.
Example Custom Class Loader:
public class MyCustomClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Load the class data and define it here
}
}
Why Use Custom Class Loaders?
Separating class loaders ensures that different parts of your application can operate independently, minimizing issues that arise from evolving APIs.
4. Versioning APIs
Implement semantic versioning for your APIs. This ensures that breaking changes are communicated effectively, allowing consumers to transition smoothly.
Example Versioning Strategy:
- MAJOR Version: Introduces breaking changes.
- MINOR Version: Adds new functionality in a backward-compatible manner.
- PATCH Version: Implements backward-compatible bug fixes.
Why Version APIs?
Clear versioning helps users understand how changes to the API may impact their applications, making it easier to manage upgrades.
5. Documentation and Communication
Effective documentation is key. Ensure that your API documentation clearly explains changes and their implications. Adopting Swagger helps in creating interactive documentation.
Example Swagger Configuration:
@OpenAPIDefinition
public class ApiDocumentation {
// Annotate API endpoints here
}
Why Document Changes?
Clear documentation helps users understand how to adapt to API changes, ensuring seamless integration and usage.
The Bottom Line
Evolving APIs can introduce class loading challenges that may disrupt development workflows. However, with a modular architecture, effective dependency management, isolated class loaders, semantic versioning, and thorough documentation, you can overcome these hurdles. Implement these strategies in your Java applications to future-proof them against the inevitable changes that come with evolving APIs.
For more information on managing APIs, consider exploring Java's Module System and dependency management tools like Maven and Gradle. They offer powerful features to enhance your development workflow.
By adopting these best practices, you can ensure that your Java applications remain maintainable, robust, and ready to embrace the future of API development.
Happy coding!