Mastering Synchronization: Common Pitfalls with Monitor in Guava
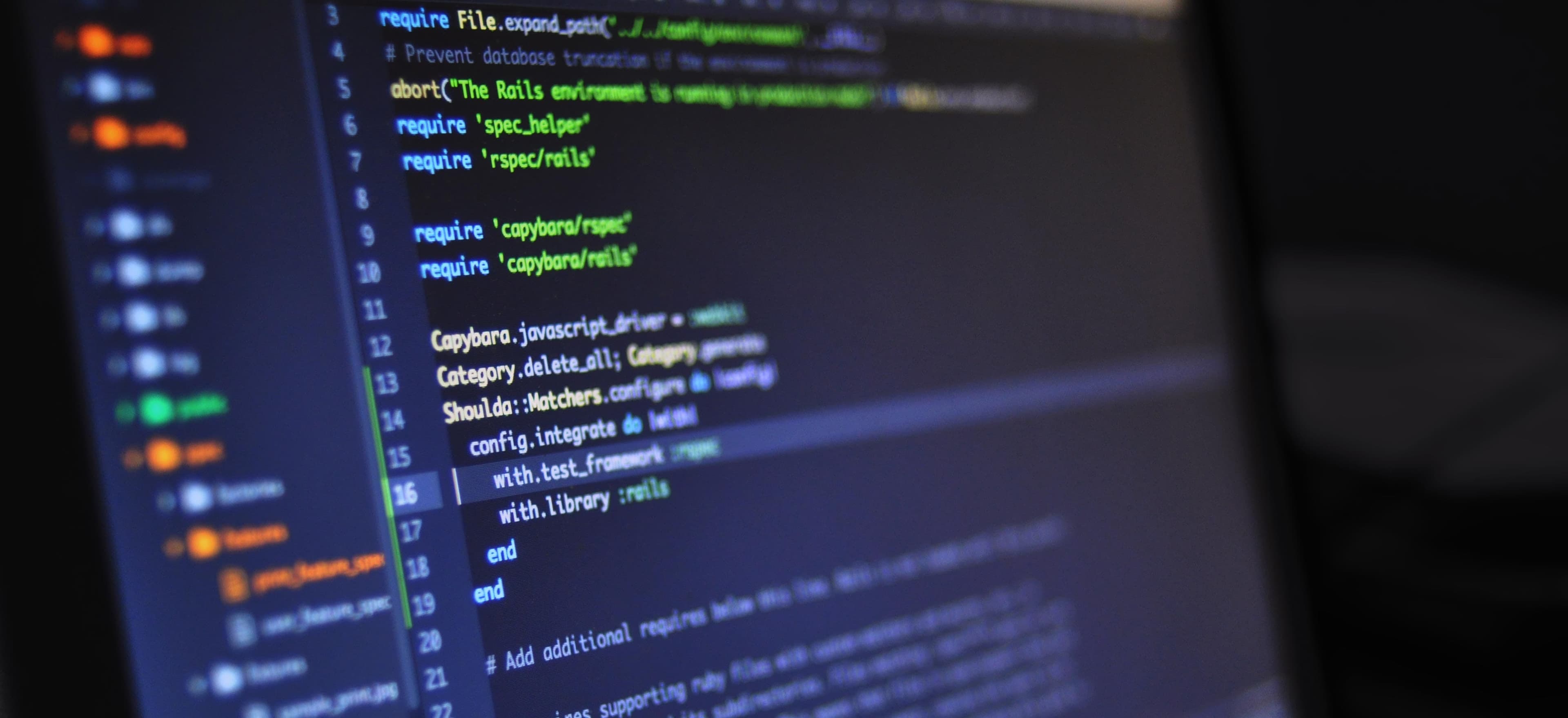
- Published on
Mastering Synchronization: Common Pitfalls with Monitor in Guava
Multithreading is a key component of modern software development, particularly in the Java programming language. Although it can vastly improve performance by executing tasks concurrently, it also introduces complexity, including potential issues such as race conditions and deadlocks. In this blog post, we will explore synchronization in Java, focusing on the Monitor from Google's Guava library, and examine the common pitfalls that developers may encounter.
Understanding Synchronization in Java
Before diving into the specifics of Guava's Monitor, it's essential to grasp the concept of synchronization in Java. Synchronization is the process of coordinating the execution of different threads to ensure that shared resources are accessed in a controlled manner. Java provides several built-in synchronization mechanisms, such as locks, synchronized blocks, and semaphores. However, using these mechanisms properly requires an understanding of their behavior and potential drawbacks.
For a more in-depth exploration of Java concurrency, you can check out the Java Concurrency Tutorial.
The Monitor in Guava
The Monitor class from Guava offers a high-level abstraction for synchronization. It simplifies the locking mechanism by providing methods to handle locks more intuitively than the traditional approach. Here are some features of the Monitor class:
- Monitor.Enter: Locks an object for use by a single thread.
- Monitor.Exit: Releases a lock previously acquired by the same thread.
- Monitor.Guard: Allows a thread to wait until a specific condition is met before proceeding.
- Monitor.Events: Listens for state changes of locks.
Here is a basic usage example of the Monitor:
import com.google.common.util.concurrent.Monitor;
public class MonitorExample {
private final Monitor monitor = new Monitor();
private int sharedResource = 0;
public void increment() {
monitor.enter();
try {
sharedResource++;
} finally {
monitor.leave();
}
}
public int getSharedResource() {
return sharedResource;
}
}
Why Use Monitor?
Using Monitor improves code readability and enhances the safety of resource management. It allows developers to lock resources while ensuring that the lock is released even if exceptions occur—thanks to the finally
block in our example. If you rely solely on native synchronization constructs, missing a lock release can lead to deadlocks, making your program unresponsive.
Common Pitfalls with Monitor
Even with its advantages, Monitor is not foolproof. Below, we discuss some common pitfalls you should be aware of while using Monitor in Guava.
1. Forgetting to Release the Lock
It’s easy to forget to release the lock, especially in more complex methods where multiple paths may be traversed. This can lead to deadlocks. Here's an improved version that ensures locks are released by using a finally
block.
public void safeIncrement() {
monitor.enter();
try {
sharedResource++;
} finally {
monitor.leave();
}
}
By wrapping our increment logic in a try-finally
block, we guarantee that leave
will always execute, even if an exception is thrown.
2. Overusing the Monitor
Using Monitor too frequently can lead to unnecessary contention between threads. This could degrade performance as threads spend more time waiting for locks to be released. Use it judiciously in performance-sensitive sections of your application.
3. Misusing Monitor.Guard
The Monitor.Guard
allows for synchronization based on specific conditions. However, improper use can lead to unintended behavior. Consider the following example:
public void guardExample() {
Monitor.Guard guard = new Monitor.Guard(monitor, () -> sharedResource > 0);
monitor.enterWhen(guard);
try {
// Process when sharedResource is greater than 0
} finally {
monitor.leave();
}
}
In this example, if sharedResource
never becomes greater than 0
, the guard never allows access, potentially leading to thread starvation. Always ensure that your exit conditions are achievable to avoid this pitfall.
4. Failing to Handle InterruptedException
When dealing with thread synchronization, you must also be prepared to handle interruptions. If a thread is waiting for a lock or condition, it can be interrupted. Here’s an improved example:
public void waitWithInterruption() throws InterruptedException {
Monitor.Guard guard = new Monitor.Guard(monitor, () -> sharedResource > 0);
monitor.enterWhen(guard);
try {
// Process when sharedResource is greater than 0
} finally {
monitor.leave();
}
}
Without proper handling of the InterruptedException
, your application may not respond as expected, leading to user experience issues. Therefore, ensure you have appropriate exception handling in place.
5. Not Considering Performance Impact
Finally, it’s essential to consider the performance implications of using locks. Locks can create bottlenecks in high-performance applications. Measure performance and determine if the granularity of your locks can be improved. Perhaps using read-write locks can optimize your synchronization patterns. Guava also offers ReadWriteLock
which might be useful in scenarios where reads are more common than writes.
Closing the Chapter
Mastering synchronization in Java, particularly with the Monitor class from Guava, involves understanding the mechanics of locking and the potential pitfalls associated with them. By recognizing and addressing common issues such as forgetting to release locks, overusing monitors, improperly configuring guards, failing to handle interruptions, and considering performance impacts, you can create more robust applications.
Keep learning, keep coding, and embrace the challenges of multithreading. If you are looking to delve deeper into the complexities of concurrency in Java, you might find Effective Java by Joshua Bloch to be a valuable resource.
Through understanding and careful implementation, you can create applications that are not only efficient and powerful but also safe and coherent. Happy coding!
Checkout our other articles