Overcoming Common Errors in Bean Validation with JAX-RS
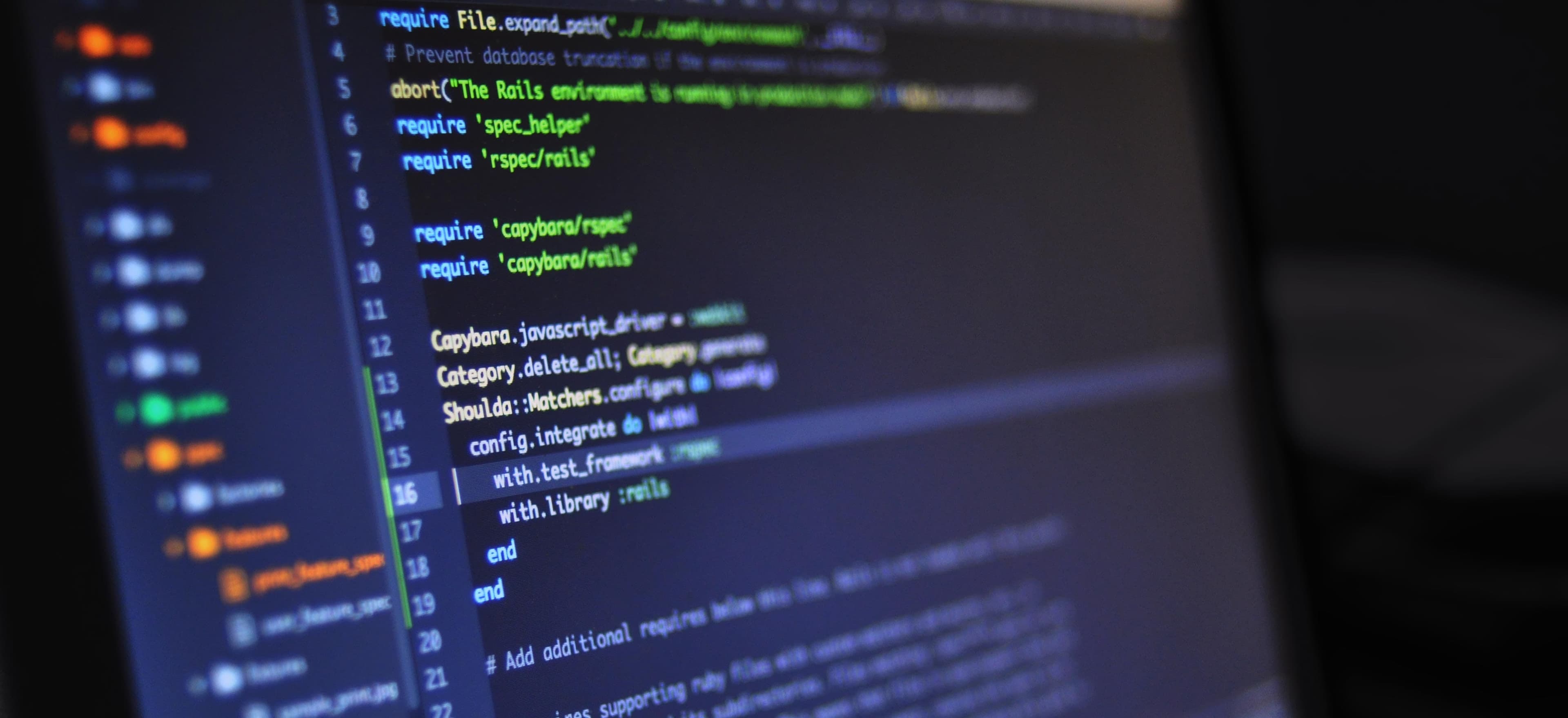
- Published on
Overcoming Common Errors in Bean Validation with JAX-RS
Java developers often encounter various challenges when implementing Bean Validation in RESTful services with JAX-RS. These common errors can lead to frustrating development experiences, reduced productivity, and potentially flawed applications if not handled gracefully. In this blog post, we will explore prevalent issues, provide solutions, and explain essential concepts to enhance your understanding of Bean Validation while streamlining your RESTful service development process.
Understanding Bean Validation and JAX-RS
Before diving into errors and their resolutions, let’s clarify what Bean Validation and JAX-RS entail.
What is Bean Validation?
Bean Validation is a framework for defining and enforcing constraints on JavaBeans. By annotating Java object properties with validation annotations, developers can ensure that the data adheres to specific rules before persisting it in a database or processing it in business logic.
What is JAX-RS?
JAX-RS (Java API for RESTful Web Services) enables developers to create RESTful web services in Java. By using JAX-RS annotations, such as @Path
, @GET
, and @POST
, developers can define a resource's behavior.
Seamlessly integrating Bean Validation with JAX-RS can improve data quality and user experience. To illustrate this integration effectively, we'll analyze a common use case and address some of the errors that may arise.
The Common Use Case: User Registration
Consider a simple user registration form where we collect user's first name, last name, email, and password. We will use JAX-RS to handle the registration endpoint and apply Bean Validation to ensure each field contains valid data.
Setting Up Your Maven Project
Ensure your project has the necessary dependencies in the pom.xml
:
<dependencies>
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
<version>2.0.1.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.32</version>
</dependency>
</dependencies>
Creating the User Registration Model
Here’s the User
model with validation annotations:
import javax.validation.constraints.Email;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
public class User {
@NotBlank(message = "First name is required")
private String firstName;
@NotBlank(message = "Last name is required")
private String lastName;
@Email(message = "Email should be valid")
private String email;
@NotBlank(message = "Password is required")
@Size(min = 8, message = "Password must have at least 8 characters")
private String password;
// Getters and Setters
}
Enhancing the Registration Endpoint with Bean Validation
Create a JAX-RS resource class for the registration endpoint:
import javax.validation.Valid;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/register")
public class UserRegistrationResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response registerUser(@Valid User user) {
// Business logic to save the user
return Response.ok("User registered successfully").build();
}
}
Common Errors and Their Solutions
Now, let’s shed light on common errors faced when implementing Bean Validation in JAX-RS and how to overcome them.
1. ConstraintViolationException: Validation Failures
When the payload does not adhere to the defined constraints, or the @Valid
annotation is missing, JAX-RS will throw a ConstraintViolationException
. If you're not catching this exception, customers may receive unclear errors.
Solution: Implement an Exception Mapper to handle validation errors gracefully.
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
import javax.validation.ConstraintViolationException;
import java.util.HashMap;
import java.util.Map;
@Provider
public class ValidationExceptionMapper implements ExceptionMapper<ConstraintViolationException> {
@Override
public Response toResponse(ConstraintViolationException exception) {
Map<String, String> errors = new HashMap<>();
exception.getConstraintViolations().forEach(violation ->
errors.put(violation.getPropertyPath().toString(), violation.getMessage()));
return Response.status(Response.Status.BAD_REQUEST).entity(errors).build();
}
}
By using the ValidationExceptionMapper
, we can customize the response message returned to the client, including a detailed map of validation errors.
2. Missing Validation Annotations
Another common error is omitted validation annotations, leading to silent failures where inputs may inadvertently pass validation checks.
Solution: Always review your model classes to ensure that the relevant validation annotations are in place. Utilize IDE tools and linters that highlight these practices.
Additional Considerations
Testing the User Registration Endpoint
After implementing the code and error handling mechanisms, it’s essential to test your endpoint. Use a tool like Postman or curl to send various payloads and verify that validations work as expected.
Example payloads to test:
-
Missing first name
{ "lastName": "Doe", "email": "john.doe@example.com", "password": "password123" }
-
Invalid email
{ "firstName": "John", "lastName": "Doe", "email": "invalid-email", "password": "password123!" }
Integrating With Front-End Validation
To enhance user experience, consider implementing client-side validation in addition to backend validation. Tools like React Hook Form or Angular Forms can provide real-time feedback, helping users correct their input before submitting the form.
A Final Look
Integrating Bean Validation with JAX-RS enhances the quality and reliability of your RESTful services. By proactively managing common errors, leveraging exception mapping, and validating input rigorously, you can reduce inconsistencies and streamline the user experience.
While the examples shared are foundational, they provide a critical understanding of handling validation within JAX-RS. For further resources, consider reading Java EE 8 Application Development and Hibernate Validator Documentation.
For more guidance on Java frameworks and best practices in service design, stay tuned to our blog.
By remaining aware of potential challenges and utilizing best practices in your JAX-RS Bean Validation implementation, you can create robust, user-friendly applications with ease. Happy coding!
Checkout our other articles