Common Pitfalls in Compiler Language Validation
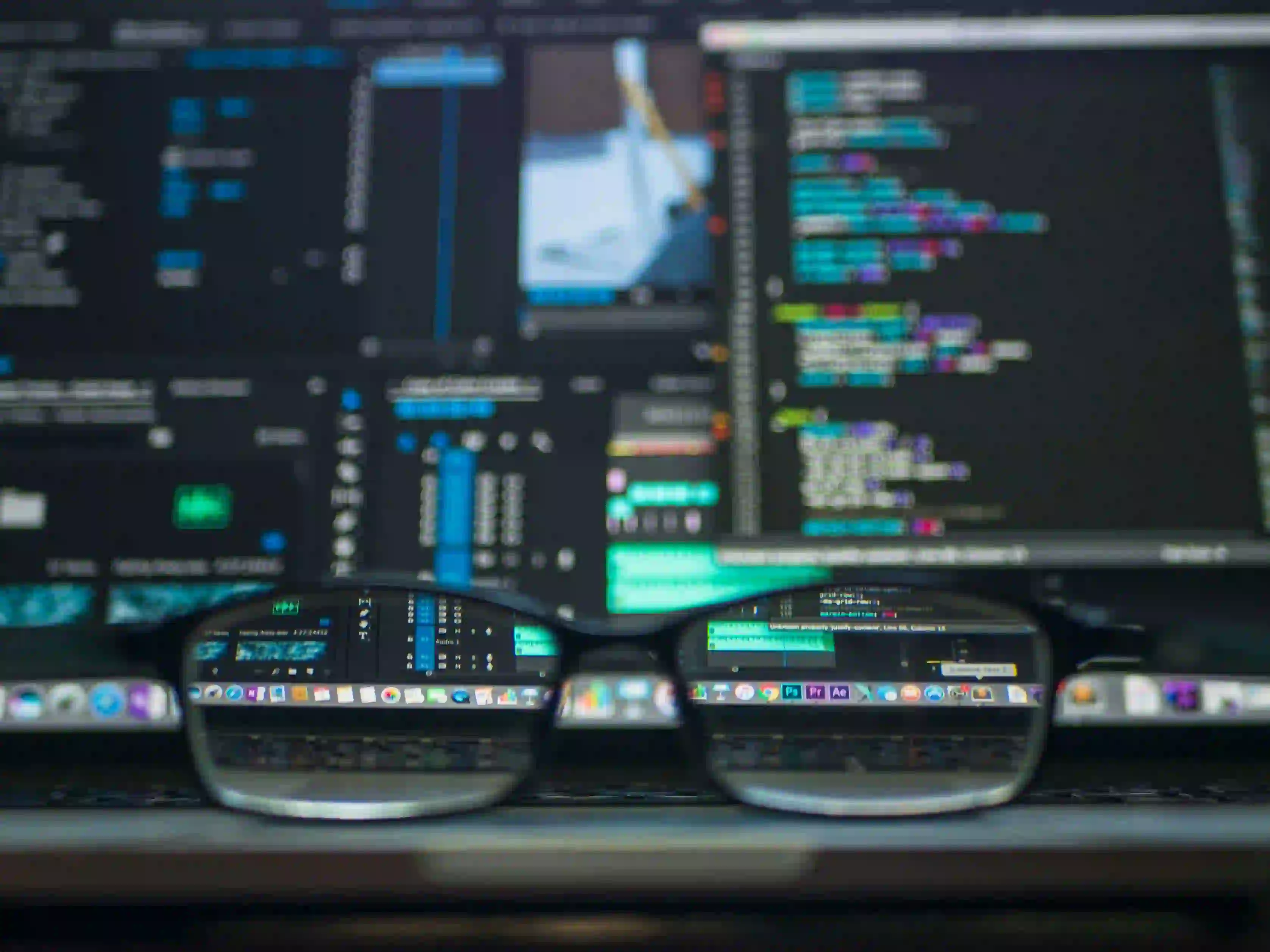
Common Pitfalls in Compiler Language Validation
Compilers are crucial for transforming human-readable code into machine language. But the journey from high-level programming languages to binary isn't straightforward. During this process, validation plays a significant role. The goal is to ensure that the source code adheres to the language's grammatical rules and semantics. However, several pitfalls can arise during compiler language validation. In this blog post, we will explore these pitfalls in detail, offering best practices to avoid them.
Understanding Compiler Language Validation
Before diving into the common challenges, it's essential to comprehend what compiler language validation involves. Validation can be divided into two main components:
- Syntax Checking: This ensures that the code structure conforms to the language's grammar rules.
- Semantic Checking: This verifies the meaning behind the syntax, ensuring that it is logically sound.
While it may seem straightforward, several pitfalls can arise in both syntax and semantic checking.
Common Pitfalls in Syntax Checking
1. Ambiguous Grammar
Ambiguities in grammar can lead to multiple interpretations of the same code. This is particularly problematic in languages that allow for operator overloading or have intricate token definitions.
Example Pitfall
For instance, consider the following expression:
int result = a + b * c;
Is b * c
evaluated first due to operator precedence, or does it depend on the context?
Best Practice
To avoid this pitfall, use Bacchus-Naur Form (BNF) or Extended Backus-Naur Form (EBNF) to define the grammar explicitly. Ensuring clarity in your grammar will prevent ambiguities.
For more details on crafting unambiguous grammars, visit the BNF documentation.
2. Overly Lenient Parsing
Some compilers are too permissive with their syntax checks. They may accept code that is technically valid but does not follow best practices or intended usage. This leniency can lead to confusion down the line.
Example
if (x = 10) {
// do something
}
Here, the intention was likely to compare x
to 10
, but the assignment operator was mistakenly used.
Best Practice
Implement a strict lexicon and grammar that enforces best practices. Utilize linting tools to identify potential errors and maintain code quality.
3. Inconsistent Error Reporting
Inconsistent or vague error messages frustrate developers and can lead them to incorrect assumptions about their code.
Example
Imagine a scenario where a missing semicolon could return a generic "error" without specifying the location or nature of the error.
Best Practice
Standardize error messages and ensure they are informative. Each error should point to the exact line, specify the type, and suggest possible fixes. This transparency aids developers in identifying problems quickly.
Common Pitfalls in Semantic Checking
1. Type Mismatches
Type mismatches are one of the most common semantic errors in programming. A variable may be declared as an integer but assigned a string value, leading to runtime errors.
Example
int number = "42"; // This raises a compilation error
Best Practice
Implement strict type-checking mechanisms and provide meaningful compile-time error messages alongside type descriptions. The use of Generics in Java helps ensure type safety.
2. Unused Variables and Functions
Compilers often miss variables and functions that are declared but never used. While this might not lead to immediate errors, it can generate unnecessary warnings and clutter in the codebase.
Example
int unusedVar; // Declared but never used.
Best Practice
Incorporate a dead code elimination phase in your compiler. This will not only improve performance but also enhance code readability by reducing clutter.
3. Inadequate Scope Resolution
Scope resolution issues arise when variables are mistakenly accessed outside of their intended scopes.
Example
int a = 5;
void someFunction() {
int a = 10; // This shadows the outer 'a'
}
Best Practice
Encourage clear scoping practices through your validation process. Enforce rules that minimize shadowing and provide developers with warnings when scoping conventions are not followed.
Bringing It All Together
Compiler validation is a complex but essential aspect of software development. Recognizing and avoiding common pitfalls can dramatically improve the reliability and quality of the generated code. By focusing on clear grammar definitions, strict error reporting, rigorous type-checking, and efficient scope management, you can construct a robust compiler that guides developers toward writing high-quality code.
Further Reading
For more information on compilers and lexical analysis, consider checking out The Definitive ANTLR 4 Reference which provides in-depth knowledge about building parsers and compilers.
Understanding these common pitfalls will streamline your compiler development process, making it easier for developers to focus on what they do best: writing clean, effective, and efficient code. Happy coding!