Bridging the Gap: Teaching Functional Programming Effectively
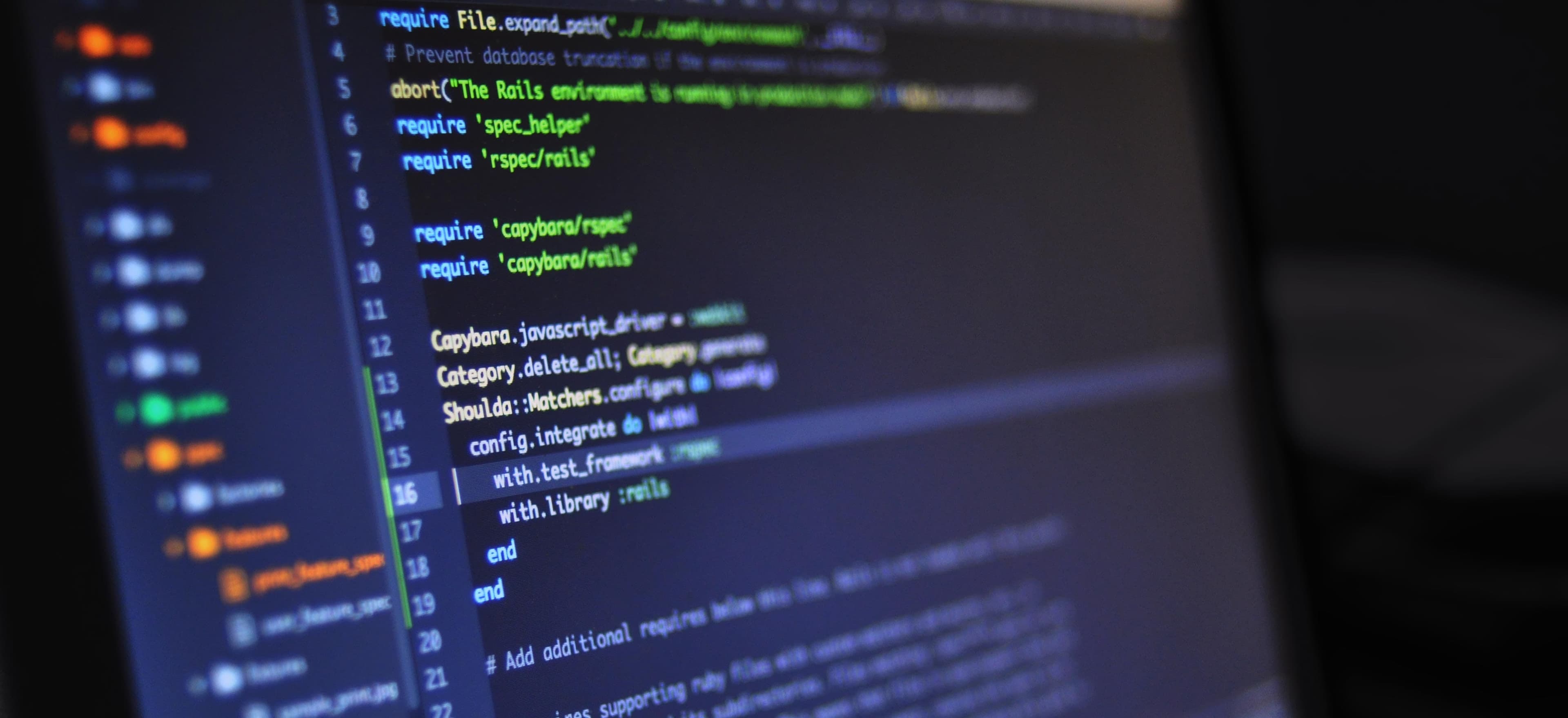
- Published on
Bridging the Gap: Teaching Functional Programming Effectively
Functional programming (FP) has emerged as a powerful paradigm, providing a different perspective from imperative programming. Yet, despite its advantages, many developers find the transition to FP challenging. In this article, we will explore effective methods for teaching functional programming concepts, addressing common misconceptions, practical examples, and providing clear coding illustrations to enhance understanding.
Understanding Functional Programming
Before diving into teaching strategies, let’s clarify what functional programming entails.
Functional programming emphasizes the use of mathematical functions to solve problems. Its key characteristics include:
- Immutability: Data is immutable, meaning it cannot be changed after it's created. This approach helps avoid side effects that can complicate debugging.
- First-Class Functions: Functions can be assigned to variables, passed as arguments, and returned from other functions.
- Higher-Order Functions: Functions that take other functions as parameters or return them as results.
- Pure Functions: Functions that always produce the same output for the same input, without side effects.
Understanding these concepts lays the groundwork for effective teaching.
Step-by-Step Approach to Teaching
1. Start With Real-World Analogies
Analogies can make abstract concepts more tangible. For example:
- Immutability can be compared to a library where books cannot be altered. If you want a different version of a book, you must make a new copy.
- Functions as Recipes: Just like recipes have specific instructions and produce the same dish with the same ingredients, functions, too, yield consistent results when given the same input.
2. Use Clear Code Examples
For learners to grasp FP, practical examples are essential. Let’s illustrate this with code snippets.
Here’s a simple example to demonstrate a pure function.
public int add(int x, int y) {
return x + y;
}
Why? This add
function represents a pure function—it produces the same output (the sum) given the same inputs (x and y), without any side effects.
3. Show the Power of First-Class and Higher-Order Functions
Consider this example, which employs a higher-order function to apply a transformation over an array of integers.
import java.util.Arrays;
public class FunctionalExample {
public static int[] transform(int[] numbers, Function<Integer, Integer> transformer) {
return Arrays.stream(numbers)
.map(transformer::apply)
.toArray();
}
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4};
int[] squared = transform(numbers, x -> x * x);
System.out.println(Arrays.toString(squared));
}
}
Why? The transform
function accepts another function, transformer
, as an argument. Here, learners can see how higher-order functions facilitate powerful transformations on collections.
4. Introduce Functional Concepts Gradually
When diving deeper into functional programming, it’s important to introduce concepts incrementally. Here’s how you can build on foundational principles:
First, understand Pure Functions
Discuss the significance of eliminating side effects. For large applications, side effects can introduce hard-to-track bugs.
Second, explore Functional Composition
Introduce function composition, where multiple functions are combined to create new functionality.
public class FunctionalComposition {
public static Function<Integer, Integer> square() {
return x -> x * x;
}
public static Function<Integer, Integer> increment() {
return x -> x + 1;
}
public static void main(String[] args) {
Function<Integer, Integer> squareIncrement = square().andThen(increment());
System.out.println(squareIncrement.apply(2)); // Output: 5
}
}
Why? This example illustrates how to combine functions into a new function. This concept is vital in structuring complex systems in a manageable way.
5. Pattern Matching with Java Streams
Functional programming shines when using Java Streams for handling collections. This can be daunting for newcomers, so it’s best to introduce it using smaller examples.
import java.util.List;
import java.util.Arrays;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println); // Outputs: Alice
}
}
Why? This example utilizes filter
and forEach
, showcasing the elegance of FP in handling data collections without the boilerplate code associated with loops.
6. Encourage Experimentation
Learning functional programming can be daunting; therefore, encourage learners to experiment with code. Platforms like Replit let learners run Java code snippets in their browsers without any setup.
7. Implement Mini-Projects
Nothing reinforces concepts better than practical application. Consider assigning mini-projects that challenge students to apply functional programming concepts they’ve learned.
Examples include:
- A simple calculator that uses pure functions for operations.
- A string manipulator that leverages higher-order functions for transformation.
8. Foster Discussions
Finally, encourage discussions among learners to share insights and challenge each other's understanding. Use forums like Stack Overflow to facilitate this.
Overcoming Common Misconceptions
One of the biggest hurdles in teaching FP is addressing misconceptions. Let’s consider a few and how to clarify them:
-
"Functional programming is only for small problems." FP scales remarkably well. Encourage exploration of libraries like RxJava for asynchronous programming.
-
"FP is too complex." Start with simple examples and gradually increase complexity. Complexity builds naturally with experience.
The Last Word
Teaching functional programming is a rewarding endeavor. By using real-world analogies, clear examples, and fostering an environment of experimentation and discussion, you can bridge the gap from traditional programming paradigms to the functional approach.
It will require patience and clear explanations, but the rewards of developing cleaner, more maintainable code through functional programming practices will benefit your students and their future careers as developers.
For more in-depth resources, consider checking out Functional Programming in Java by Venkat Subramaniam. Happy coding!
Checkout our other articles