Choosing the Right Garbage Collector: G1 vs CMS vs Parallel
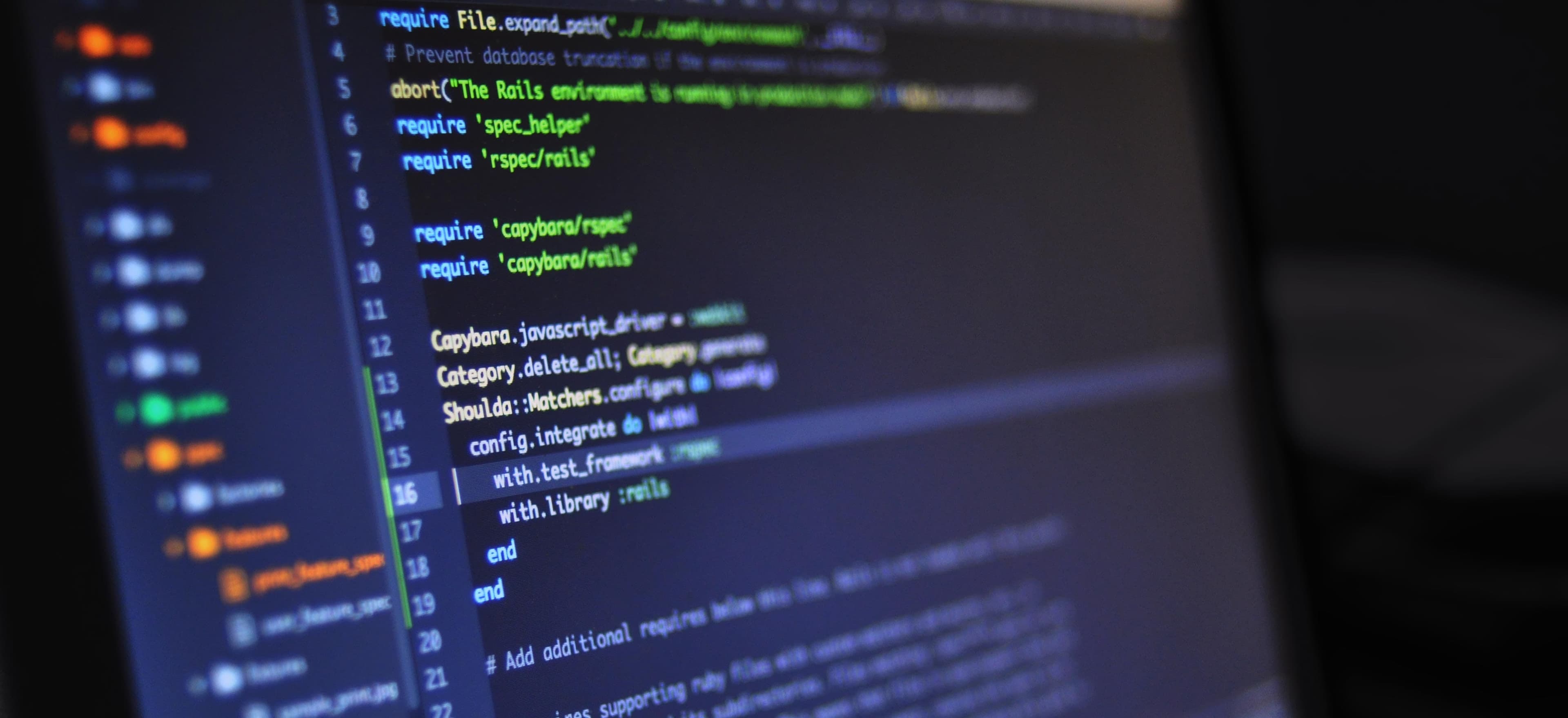
- Published on
Choosing the Right Garbage Collector: G1 vs CMS vs Parallel
Garbage collection is a crucial aspect of Java's memory management. It enables developers to allocate memory dynamically while ensuring that memory leaks are minimized. However, with several garbage collector options available, choosing the right one can be daunting. In this blog post, we’ll explore three popular garbage collectors available in Java: G1 (Garbage-First), CMS (Concurrent Mark-Sweep), and Parallel. By the end, you will have a clearer understanding of their differences, strengths, and weaknesses.
What is Garbage Collection?
Garbage collection refers to the automatic process of identifying and reclaiming unused memory in your application. This process is vital because it helps prevent memory leaks and optimizes memory usage, allowing your Java applications to run efficiently.
Java uses two types of memory: the heap memory pool and the stack memory pool. The heap is where objects are stored, while the stack is used for method calls and local variables. Garbage collectors primarily deal with heap memory, cleaning up unreachable objects left behind after an operation.
Understanding the Different Garbage Collectors
1. G1 (Garbage-First)
The G1 garbage collector was designed with a focus on high throughput and low pause time. It is especially suitable for applications with large heaps and predictable response times.
How G1 Works
G1 splits the heap into multiple regions and collects garbage in a way that prioritizes regions with the most garbage first. It performs this operation in multiple phases:
-
Young Generation Collection: G1 first collects the young generation objects that are no longer reachable. This phase is usually quick and helps reduce the heap quickly.
-
Mixed Garbage Collection: After several collections, G1 will also select some old-generation regions to collect.
-
Full GC: If necessary, G1 can perform a full garbage collection, which is less frequent but may incur longer pause times.
// Example of G1 Garbage Collector setup in Java
java -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -jar YourApplication.jar
This command initiates your Java application using G1 GC and allows you to set a maximum pause time of 200 ms. It's crucial to set a reasonable pause time for applications with real-time requirements.
Advantages and Disadvantages of G1
Advantages:
- Predictable pause times.
- Efficient in managing large heaps.
- Concurrent collections reduce the pause time significantly.
Disadvantages:
- Overhead during marking cycles can have performance implications.
- May require tuning for specific workloads.
2. CMS (Concurrent Mark-Sweep)
The CMS collector focuses on minimizing pause times during garbage collection. It achieves this by performing most of its work concurrently with the application's execution.
How CMS Works
CMS operates in two phases:
-
Initial Mark: This phase marks objects that are directly reachable from the roots (like static variables or stack-local variables).
-
Concurrent Marking: The garbage collector walks through the object graph to identify other reachable objects while the application continues to run.
-
Concurrent Sweep: After marking, CMS clears out unreachable objects from the heap. This phase may also run concurrently.
// Example of CMS Garbage Collector setup in Java
java -XX:+UseConcMarkSweepGC -jar YourApplication.jar
Here you instruct Java to utilize the CMS collector. This setup is more suited for applications requiring low pause times.
Advantages and Disadvantages of CMS
Advantages:
- Low-pause times are great for interactive applications.
- Good for applications requiring large heaps.
Disadvantages:
- Can lead to fragmentation in the heap, leading to OutOfMemory exceptions.
- Requires more system resources due to its concurrent nature, making it less efficient for smaller systems.
3. Parallel Garbage Collector
Parallel garbage collector, also known as the throughput collector, is designed to optimize overall throughput rather than minimizing GC pause times. It uses multiple threads to perform garbage collection, making it highly efficient for CPU-bound applications.
How Parallel GC Works
The parallel garbage collector predominantly operates in a stop-the-world manner but can leverage multiple threads for each phase:
-
Young Generation Collection: Objects in the young generation are collected, which involves a stop-the-world event where all application threads are paused while the garbage collector runs.
-
Old Generation Collection: Similar to the young generation, but this phase can be more expensive in terms of time.
// Example of Parallel Garbage Collector setup in Java
java -XX:+UseParallelGC -jar YourApplication.jar
This command sets your application to use the Parallel GC, balancing efficiency and performance.
Advantages and Disadvantages of Parallel GC
Advantages:
- High throughput for batch processing applications.
- Utilizes multiple CPUs, maximizing the use of available cores.
Disadvantages:
- Long pause times can be detrimental for user-facing applications requiring quick feedback.
- Can be less efficient in environments with heavy allocation and deallocation patterns.
Choosing the Right Garbage Collector
When to Use G1?
If your application emphasizes low pause times while managing a large heap space, G1 is typically the best choice. In addition, if your workload varies significantly, G1's adaptability can provide more benefits.
When to Use CMS?
CMS should be the go-to choice for applications requiring low-latency interactions, such as web servers or game servers. However, be prepared to implement strategies for dealing with potential heap fragmentation.
When to Use Parallel?
Opt for the Parallel Garbage Collector if you are running batch jobs or CPU-intensive applications where throughput is prioritized over low latency. This collector shines in environments with minimal user interaction.
Closing Remarks
Selecting the right garbage collector is essential for ensuring your Java application runs efficiently and effectively. By understanding the capabilities and limitations of G1, CMS, and Parallel, you can make an informed decision that aligns with your application's requirements.
For further reading on Java's garbage collectors and application tuning, check out the following resources:
- Official Java Garbage Collection Tuning Guide
- Understanding Java Garbage Collection: A Beginner’s Guide
By carefully analyzing your specific use case and operational needs, you can ensure optimal performance for your Java applications. Happy coding!
Checkout our other articles