Overcoming Common Scaling Challenges in Java EE Applications
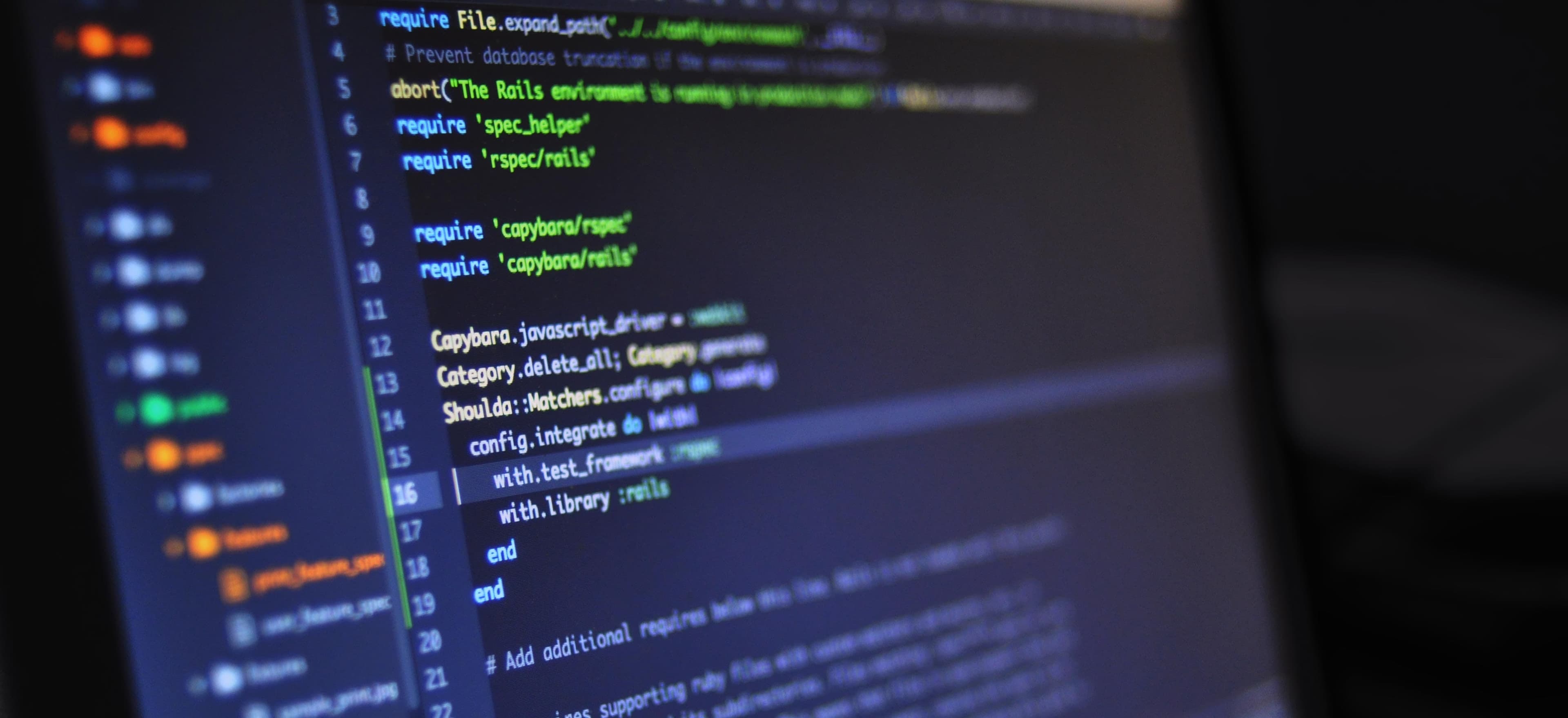
- Published on
Overcoming Common Scaling Challenges in Java EE Applications
Java EE (Enterprise Edition), now known as Jakarta EE, provides a robust framework for building enterprise-level applications. However, as applications grow in complexity and user demands increase, scaling them can pose significant challenges. In this blog post, we'll discuss some common scaling challenges in Java EE applications and explore effective strategies to overcome them.
Understanding Scaling in Java EE
Before we dive into specific challenges, let's clarify what we mean by "scaling." Scaling can primarily be categorized into two types:
- Vertical Scaling: Also called "scaling up," this involves increasing the resources of a single server (e.g., CPU, RAM).
- Horizontal Scaling: Known as "scaling out," this refers to adding more servers to distribute the load.
Both methods have their advantages and disadvantages. However, understanding when and how to implement these strategies can significantly impact the performance of your application.
Common Scaling Challenges
Let's explore some of the most frequent scaling challenges faced by Java EE developers.
1. Session Management
Java EE applications often rely on stateful sessions to manage user sessions. However, as user load increases, maintaining state across multiple servers can result in significant bottlenecks.
Solution: Use Stateless Sessions or Distributed Caches
Using stateless session beans can mitigate these issues, as they allow for easier scaling. When the session is stateless, it does not hold client-specific data, making it simpler to distribute requests across multiple servers.
Here's a simple example of using a stateless session bean:
@Stateless
public class CalculationService {
public int add(int a, int b) {
return a + b;
}
}
This CalculationService
can be deployed across multiple instances, allowing requests to be load-balanced effectively.
If your application demands state management, consider distributed caching solutions like Hazelcast or Infinispan. These solutions reduce the load on the database and keep session data available across a cluster.
Why Use Caching?
Using caches helps eliminate repeated database calls, improving performance and lowering latency.
2. Database Bottleneck
Database access is often a performance bottleneck in Java EE applications. A single database instance can quickly become overwhelmed with read/write requests.
Solution: Database Sharding and Connection Pooling
Database sharding involves partitioning your database into smaller, more manageable pieces. Each shard can be queried separately, reducing the load on any single database.
Database Connection Pooling is equally critical. Instead of creating a new connection for each user request, connection pools allow your application to reuse existing connections.
Here's how to set up a connection pool using HikariCP, a popular lightweight connection pool library:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>4.0.3</version>
</dependency>
Then configure it in your persistence.xml
:
<persistence-unit name="myPU">
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="javax.persistence.jdbc.user" value="user"/>
<property name="javax.persistence.jdbc.password" value="password"/>
<property name="hibernate.hikaricp.minimumIdle" value="5"/>
<property name="hibernate.hikaricp.maximumPoolSize" value="10"/>
<property name="hibernate.hikaricp.idleTimeout" value="30000"/>
</properties>
</persistence-unit>
Why Sharding and Connection Pooling?
These practices allow faster access to the database, legitimatizing the scaling of your application horizontally.
3. High CPU Usage
Application logic and inefficient algorithms can lead to high CPU usage, which can hinder your application’s ability to scale.
Solution: Optimize Algorithms
Always profile your application to identify bottlenecks. Java provides several profilers, such as VisualVM and JProfiler. Once identified, analyze and refactor inefficient code.
For instance, instead of using a basic for-loop for large data sets, consider using Java Streams for parallel processing:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> uppercaseNames = names.parallelStream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Why Optimize?
Optimized code translates to reduced CPU usage, allowing more requests to be processed concurrently.
4. Load Balancing
As traffic increases, a single server may become a bottleneck. Relying on conventional load balancing techniques can result in uneven traffic distribution.
Solution: Use a Reverse Proxy with Smart Load Balancing
Using a reverse proxy like Nginx or HAProxy can efficiently balance loads. These tools can route requests intelligently based on the server's current load, providing better resource utilization.
Why Use a Reverse Proxy?
Reverse proxies cut down latency by caching responses and reducing the load on application servers.
5. Microservices Architecture
Shifting to a microservices architecture can provide redundancy and flexibility but comes with its own set of challenges related to scaling, such as inter-service communication and data consistency.
Solution: Implement Service Discovery and API Gateway
Using tools like Netflix Eureka for service discovery and API Gateway patterns can help your microservices communicate efficiently and manage scaling.
Here's an example configuration for Netflix Eureka:
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Why Microservices?
Microservices allow your application to scale independently. If one service encounters high load, it can be scaled without affecting others.
Final Thoughts
Scaling Java EE applications requires a multifaceted approach, considering both architectural decisions and the optimization of individual components. By adopting stateless designs, optimizing database interactions, managing CPU usage, implementing effective load balancing, and considering a microservices approach, you can build scalable, robust applications.
Additional Resources
- Jakarta EE Specifications
- HikariCP Documentation
- Netflix Eureka
Scaling Java EE applications can be a daunting task, but with the right strategies in place, it is entirely achievable. Start implementing these solutions today and optimize your Java EE applications for better performance and user satisfaction.
Checkout our other articles