Mastering Equals and HashCode in Hibernate: Avoiding Pitfalls
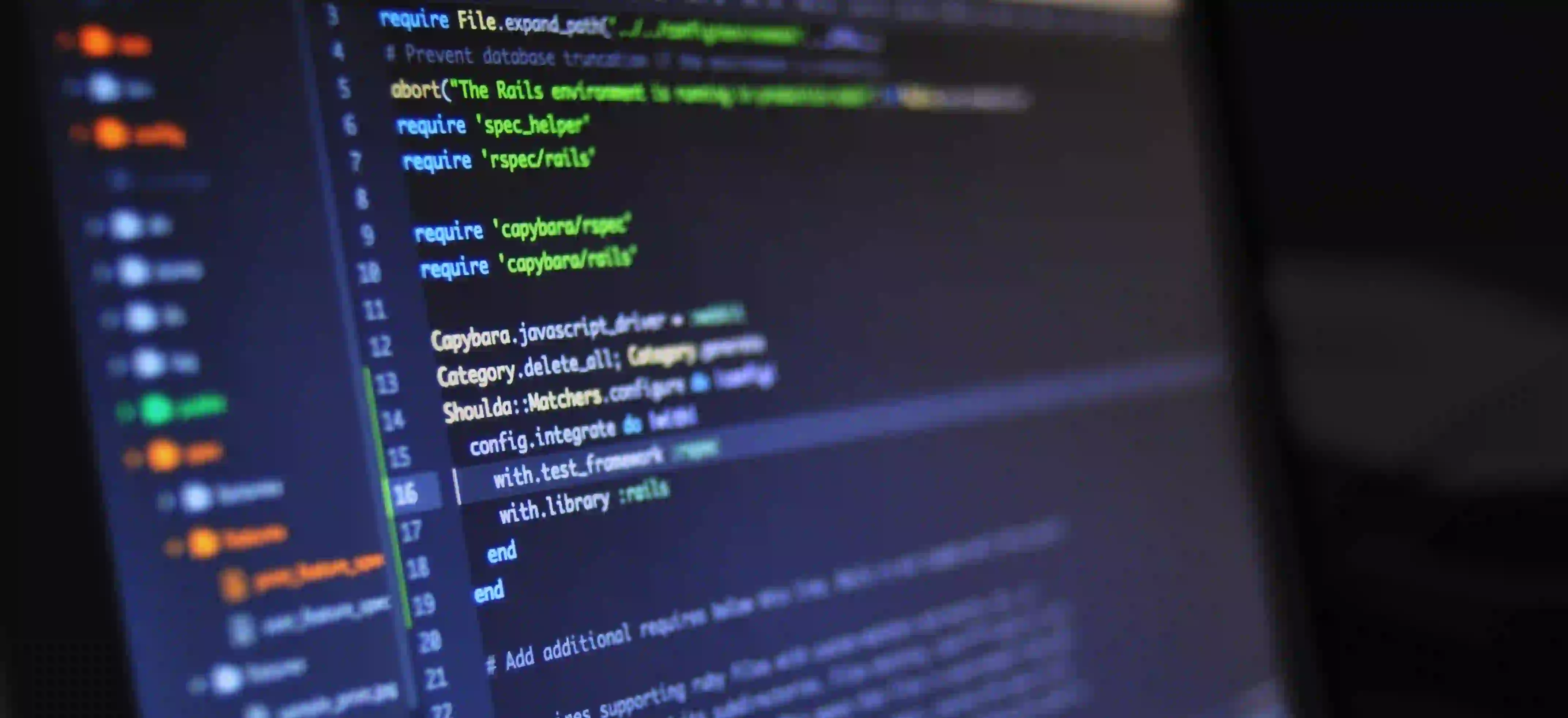
Mastering Equals and HashCode in Hibernate: Avoiding Pitfalls
When working with Java and Hibernate, understanding the implementation of equals()
and hashCode()
is pivotal. Not only do these methods affect object comparison, but they also significantly influence the behavior of entities within collections and the relational database mappings Hibernate manages.
In this blog post, we will delve into the nuances of correctly implementing equals()
and hashCode()
in Hibernate-equipped Java applications. We will highlight common pitfalls and provide clean, well-commented code examples to equip you with the knowledge to avoid these traps.
Why Equals and HashCode Matter
Before we dive into the technical details, let's understand why equals()
and hashCode()
are essential.
-
Object Comparison: The
equals()
method checks if two object references point to the same object or if they represent the same data. When it's not adequately overridden, two distinct objects can be considered equal erroneously. -
Hash-Based Collections: Java collections such as
HashSet
,HashMap
, andHashtable
depend on thehashCode()
method for element management. IfhashCode()
is not implemented alongsideequals()
, it can lead to unexpected behaviors, such as loss of data in collections.
In Hibernate, incorrect implementations of these methods can result in issues like stale data, difficulties in filtering, or even loss of references during application runtime. Thus, mastering them is crucial.
Implementing Equals and HashCode
Basic Contract
Before we write code, it’s crucial to pay attention to the contract defined in the Java documentation:
- equals: If two objects are equal, they must have the same hash code.
- hashCode: If two objects are not equal, they might (or might not) have the same hash code.
Example Entity Class
Below is an example of a simple user entity class integrating the equals()
and hashCode()
methods.
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
public User() {
}
public User(String username) {
this.username = username;
}
// Getters and setters omitted for brevity
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof User)) return false;
User user = (User) o;
return id != null && id.equals(user.id);
}
@Override
public int hashCode() {
return 31; // Default when ID is not yet generated (transient entity)
}
}
Explanation of the Code
In this example, we have:
-
ID is the Identifier: By using the database-generated
id
, we ensure that equality checks rely on an immutable, unique value. This is preferred because the username or other mutable attributes might change. -
Default hashCode Implementation: The method simply returns a constant value (31) if the real hash code cannot be computed since the ID is not generated until the entity is persisted in the database.
Handling Mutable Fields
If your entity schema includes mutable fields, be cautious. Below is an updated version that includes a mutable field, username
.
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof User)) return false;
User user = (User) o;
// Check both ID and username for equality (if both are present)
return id != null && id.equals(user.id) && username.equals(user.username);
}
@Override
public int hashCode() {
// Use both id and username here; however, care must be taken as they can change
int result = id != null ? id.hashCode() : 0;
result = 31 * result + (username != null ? username.hashCode() : 0);
return result;
}
Why This Matters
-
Element Uniqueness: This approach ensures uniqueness in collections. If a user updates their username, despite having the same ID, they will be treated as the same object due to the unique key.
-
Performance: The prime number multiplier (31) is a commonly used practice that helps distribute hash codes effectively and minimizes collisions in hash-based collections.
Pitfalls to Avoid
-
Ignoring Null Values: Always consider nullability. When implementing
equals()
andhashCode()
, check for null to avoidNullPointerExceptions
. -
Changing Fields: Avoid making fields affecting
equals()
orhashCode()
mutable. If they change, stored objects may conduct incorrect comparisons or lose integrity in collections. -
Overriding Only One: Always override both methods together. Failing to do so can lead to bugs in collections or comparison issues.
-
Transient Entities: For transient entities (those not persisted), use a different mechanism for equality checks temporarily, preferably ignoring non-initialized fields.
Final Thoughts
Implementing equals()
and hashCode()
is not just an exercise in fulfilling requirements. It affects the integrity and performance of your Java application with Hibernate. By following the guidelines mentioned, you can avoid common pitfalls and establish reliable object comparisons.
For additional resources, check out the following:
Master these methods, and ensure your applications run smoothly and efficiently in a Hibernate environment!