Why You Should Delay Switching to Java 8 Today
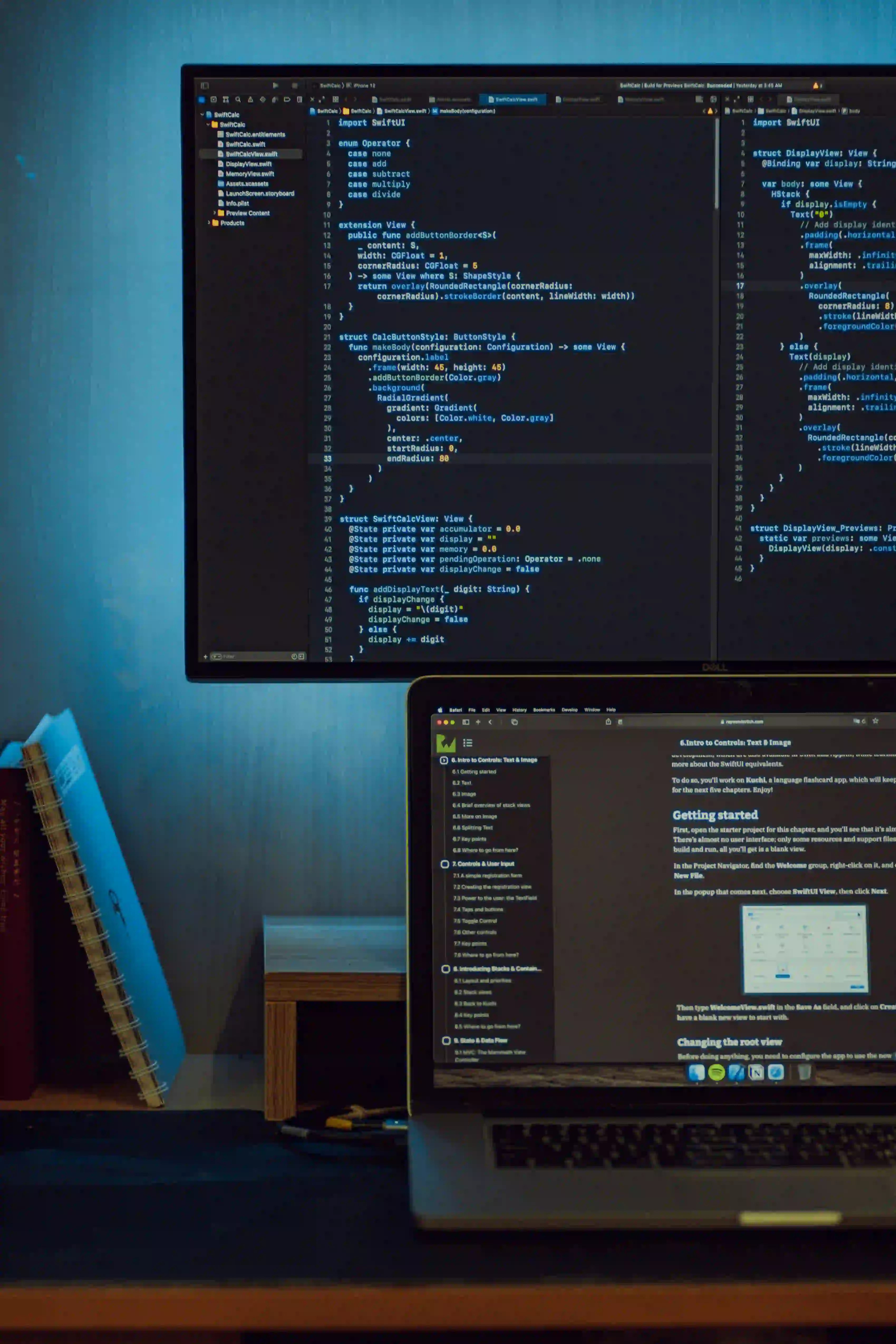
Why You Should Delay Switching to Java 8 Today
Java has long been one of the dominant programming languages, favored for its versatility, position in enterprise environments, and extensive ecosystem. With the introduction of Java 8, significant features were rolled out that bolstered its appeal, especially concerning functional programming, the Stream API, and changes to the Date and Time API. However, before diving headfirst into Java 8, here are several seemingly compelling reasons why it might be wise to hold off on that switch today.
1. Compatibility Concerns
One of the foremost considerations when upgrading any technology stack is compatibility. Legacy systems often depend on a myriad of libraries and frameworks that may not yet support Java 8. If your application relies on third-party dependencies that have not transitioned, you will encounter runtime issues, bugs, and potential system crashes.
Example Snippet: Runtime Exception
public class CompatibilityCheck {
public static void main(String[] args) {
try {
// Assuming some third-party code uses an older version
oldLibraryMethod();
} catch (UnsupportedOperationException e) {
System.out.println("Incompatible library: " + e.getMessage());
}
}
static void oldLibraryMethod() {
throw new UnsupportedOperationException("This library is not compatible with Java 8 features.");
}
}
Why? This snippet illustrates how an UnsupportedOperationException might occur if a method from a library isn't compatible with Java 8.
2. Steep Learning Curve for Developers
Java 8 introduces a host of new features, primarily focused on functional programming paradigms through lambdas and the Stream API. While these features can simplify and make code more expressive, they also come with a learning curve.
For teams that have not adapted to these new coding styles, the transition could yield productivity losses and increased error rates during the learning phase.
Example Snippet: Lambda Expression
import java.util.Arrays;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack");
// Before Java 8, we used anonymous inner classes
names.forEach(name -> System.out.println("Hello, " + name));
}
}
Why? Understanding lambda expressions adds complexity to the learning curve for teams not accustomed to functional programming.
3. Performance Implications
While the Stream API allows for concise and potentially parallel processing of data, it doesn’t guarantee better performance. In fact, if you're working with small data sets, the overhead introduced by streams could lead to performance degradation rather than improvement.
Example Snippet: Stream vs. For-Loop
import java.util.Arrays;
import java.util.List;
public class PerformanceExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using streams
numbers.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
// Using traditional for-loop
for (int n : numbers) {
if (n % 2 == 0) {
System.out.println(n);
}
}
}
}
Why? This example shows the comparison between using streams and traditional for-loops, helping one visually assess the potential performance pitfall.
4. The Java Ecosystem is Still Catching Up
While Java 8 has been around for several years, some frameworks, libraries, and tools have been slow to fully embrace its new features. Before making the switch, it’s worth examining the stability and maturity of your application’s entire ecosystem. In some cases, sticking to a more established version may offer more robust support and fewer headaches.
5. Incomplete Removal of Old Behaviors
When adopting Java 8, some legacy behaviors may still linger, resulting in unexpected bugs and issues. With changes to the Date and Time API, for instance, it can be easy to overlook how certain methods may behave differently than before, creating subtle errors in your application.
Example Snippet: Deprecated Method
import java.util.Date;
public class LegacyDateUsage {
public static void main(String[] args) {
// Old way of using Date (deprecated)
Date date = new Date(System.currentTimeMillis());
System.out.println("Old Date: " + date.toString());
}
}
Why? Transitioning from the old Date class to the new java.time package in Java 8 can lead to deprecated method usages that break backward compatibility.
6. Gradual Transitioning is Preferable
If your organization has established workflows and practices, a gradual transition may be more prudent. Chopping off the old version and replacing it with Java 8 in one go could disrupt your system. By progressively integrating Java 8 features in non-critical applications, you can safeguard your existing systems while providing your team with the opportunity to familiarize themselves with the new features without pressure.
7. Long-Term Support Versions
Rather than jumping into Java 8, consider the implications of committing to Long-Term Support (LTS) versions. It’s vital to gauge how your working environment aligns with Oracle's release and support schedule.
Java 11 is the next LTS version after Java 8, and holding off your transition to Java 8 may allow your team to directly upgrade to a version with continued support and enhancements, minimizing future migration headaches.
Wrapping Up
While Java 8 has undoubtedly propelled Java into new territories with its robust features, a premature switch can have far-reaching consequences. Always weigh the benefits against the potential drawbacks when considering an upgrade. The key is to assess your application’s needs, your team's expertise, and your ecosystem’s readiness.
In light of these considerations, a cautious and well-planned approach may prove to be the best strategy. For further reading, you can check out Official Java Documentation and explore the nuances of Java enhancements.
Remember, technology decisions should always align with your unique organizational goals and project requirements. Sometimes, patience is indeed a virtue in the world of software development.