Common Pitfalls to Avoid in Spring Boot Development
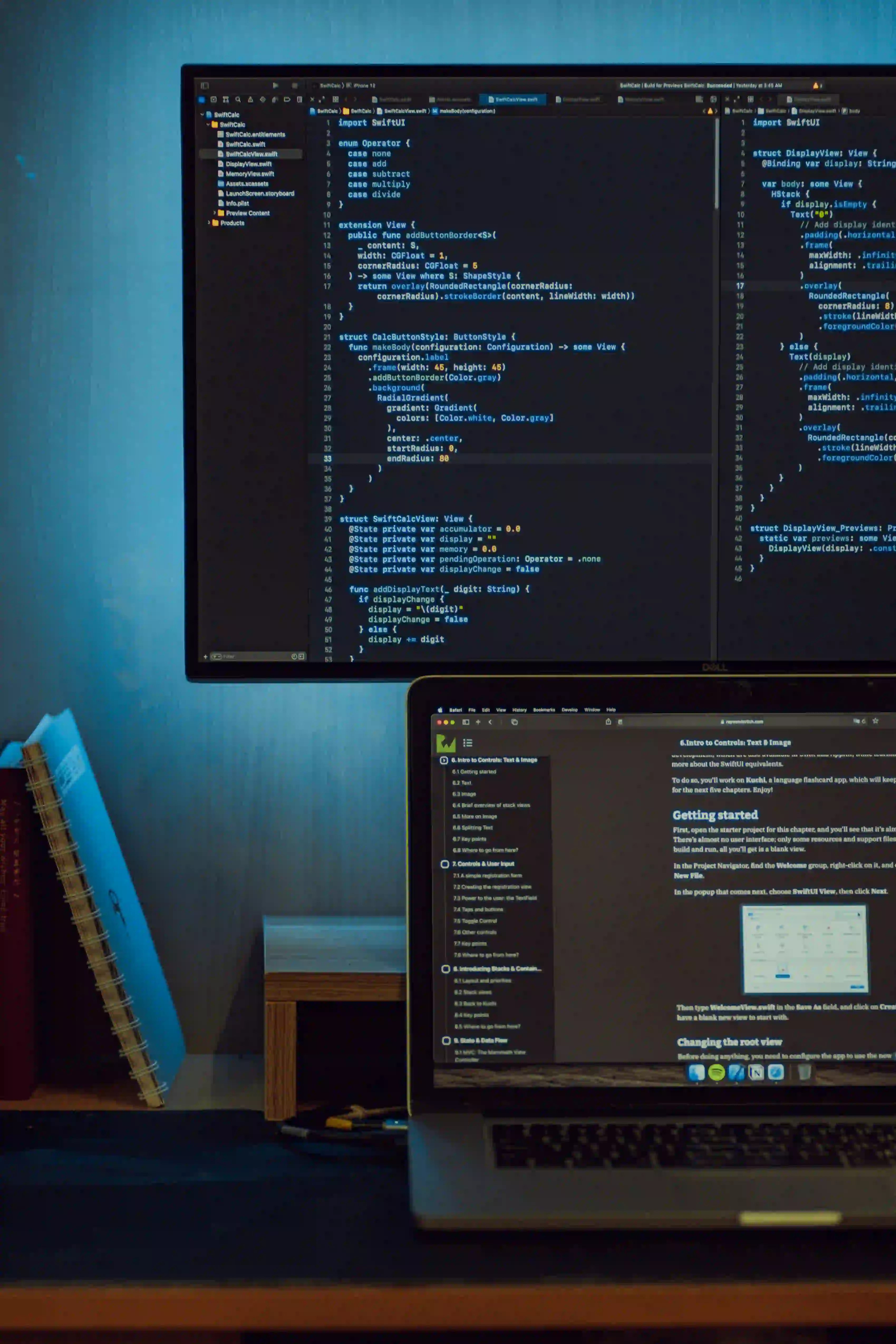
Common Pitfalls to Avoid in Spring Boot Development
Spring Boot has become one of the most popular frameworks for building Java applications. Its ease of use, extensive features, and robust community support make it a favorite among developers. However, just like any powerful tool, Spring Boot comes with its own set of pitfalls that developers, especially those new to the framework, may encounter. In this blog post, we will explore some common mistakes to avoid in Spring Boot development, along with code snippets and tips to help you build more efficient applications.
1. Overusing @Autowired
While Spring's dependency injection is a powerful feature, overusing the @Autowired
annotation can lead to some pitfalls. While it provides convenience, it can also lead to hidden dependencies and make testing difficult.
Example:
@Service
public class MyService {
@Autowired
private MyRepository myRepository; // Consider the implications of this.
public void performAction() {
myRepository.save(new MyEntity());
}
}
Why Avoid Overusing @Autowired?
- Sugar coating the dependencies: Over-reliance on
@Autowired
can obscure the actual dependencies of a class. - Testing difficulty: Classes become harder to test because the actual implementation is hidden behind the framework. Consider using constructor injection instead, as it makes dependencies more explicit.
Better Approach:
@Service
public class MyService {
private final MyRepository myRepository;
public MyService(MyRepository myRepository) {
this.myRepository = myRepository; // Clear dependency injection
}
public void performAction() {
myRepository.save(new MyEntity());
}
}
By utilizing constructor injection, you enhance the clarity of your code and simplify unit testing.
2. Ignoring Profiles
Spring Boot offers profiles to manage configuration. Ignoring this feature can lead to configuration issues when moving from development to production environments.
Example:
# application.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: user
password: pass
Why Profiles Matter?
- Environment Compatibility: Different configurations for development, test, and production environments help maintain application functionality.
- Easier Testing: Spring profiles make it easier to switch between environments without hardcoding configurations.
Using Profiles
# application-dev.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb_dev
# application-prod.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb_prod
Activate a profile using the following command:
java -jar myapp.jar --spring.profiles.active=dev
This helps in keeping your production secrets secure.
3. Poor Error Handling
Ignoring best practices for error handling can lead to unexpected application behavior and poor user experience.
Example of Poor Error Handling:
@GetMapping("/user/{id}")
public User getUser(@PathVariable Long id) {
return userRepository.findById(id).orElse(null); // No meaningful return on failure
}
Why Good Error Handling is Necessary?
- User Experience: Users should receive meaningful feedback instead of a generic error.
- Debugging: Proper logging can help in identifying issues quickly.
Better Error Handling Example:
@GetMapping("/user/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
return userRepository.findById(id)
.map(user -> ResponseEntity.ok(user))
.orElse(ResponseEntity.notFound().build());
}
Using ResponseEntity
, we can convey relevant information about the request's outcome.
4. Inconsistent Naming Conventions
Inconsistent naming of classes, variables, and methods can lead to confusion and harder maintenance.
Common Habit:
public class DataService {
// Inconsistently named methods here
public void addTheData() {}
public User doGetUser() {}
}
Why Consistency Matters?
- Readability: Consistent naming conventions make your code easier to read and understand for others.
- Maintenance: Clear organization of code helps in future maintenance and collaboration.
Suggested Naming Convention:
- Use standard naming conventions such as camelCase for methods and PascalCase for classes.
public class UserService {
public void addData() {}
public User getUser() {}
}
5. Not Leveraging Spring Boot Starters
Developers sometimes build dependencies manually instead of taking advantage of Spring Boot Starters.
Risk of Manual Dependency Management:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
By failing to use Spring Boot's starter packs, you may end up creating a tangled web of dependencies that are hard to maintain.
Recommended Approach:
Always leverage the Spring Boot starters, which encapsulate related dependencies into a single package.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
Benefits of Using Starters
- Simplified Dependency Management: You reduce the risk of missing libraries.
- Easier Configuration: Starters help in setting up default configurations relevant to the library.
6. Misusing @Transactional
Misplacing the @Transactional
annotation can lead to complexities, including unexpected behavior or performance issues.
Common Mistake:
@Transactional
public User getUser(Long id) {
return userRepository.findById(id).orElseThrow();
}
Why is this an Issue?
- Performance: Applying
@Transactional
to methods that do not modify the database defeats its purpose. - Complexity: This can make resource management unintuitive.
Best Practice for @Transactional:
Only use @Transactional
when necessary, particularly on methods that involve data manipulation.
@Transactional
public User createUser(User user) {
return userRepository.save(user);
}
This approach ensures that transactions are appropriately scoped to methods performing database changes.
Final Thoughts
Spring Boot projects can provide significant benefits regarding productivity and ease of development. By avoiding these common pitfalls, you'll create more maintainable, testable, and robust applications.
To stay updated on Spring Boot best practices, consider checking out Spring's official documentation for the latest features and improvements.
By leveraging the provided solutions and maintaining good coding practices, you can fully harness the power of Spring Boot while steering clear of potential issues. Happy coding!